mirror of
https://github.com/topjohnwu/Magisk.git
synced 2024-11-25 11:05:34 +00:00
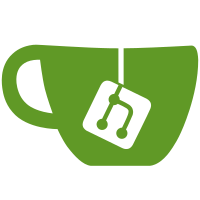
In the current implementation, Magisk will either have to recreate all early mount implementation (for legacy SAR and rootfs devices) or delegate early mount to first stage init (for 2SI devices) to access required partitions for loading sepolicy. It then has to recreate the split sepolicy loading implementation in-house, apply patches, then dump the compiled + patched policies into monolithic format somewhere. Finally, it patches the original init to force it to load the sepolicy file we just created. With the increasing complexity involved in early mount and split sepolicy (there is even APEX module involved in the future!), it is about time to rethink Magisk's sepolicy strategy as rebuilding init's functionality is not scalable and easy to maintain. In this commit, instead of building sepolicy ourselves, we mock selinuxfs with FIFO files connected to a pre-init daemon, waiting for the actual init process to directly write the sepolicy file into MagiskInit. We then patch the file and load it into the kernel. Some FIFO tricks has to be used to hijack the original init process's control flow and prevent race conditions, details are directly in the comments in code. At the moment, only system-as-root (read-only root) support is added. Support for legacy rootfs devices will come with a follow up commit.
64 lines
1.8 KiB
C++
64 lines
1.8 KiB
C++
#pragma once
|
|
|
|
#include <stdlib.h>
|
|
#include <selinux.hpp>
|
|
#include <string>
|
|
|
|
#define ALL nullptr
|
|
|
|
struct policydb;
|
|
|
|
class sepolicy {
|
|
public:
|
|
using c_str = const char *;
|
|
~sepolicy();
|
|
|
|
// Public static factory functions
|
|
static sepolicy *from_data(char *data, size_t len);
|
|
static sepolicy *from_file(c_str file);
|
|
static sepolicy *from_split();
|
|
static sepolicy *compile_split();
|
|
|
|
// External APIs
|
|
bool to_file(c_str file);
|
|
void parse_statement(c_str stmt);
|
|
void load_rules(const std::string &rules);
|
|
void load_rule_file(c_str file);
|
|
|
|
// Operation on types
|
|
bool type(c_str name, c_str attr);
|
|
bool attribute(c_str name);
|
|
bool permissive(c_str type);
|
|
bool enforce(c_str type);
|
|
bool typeattribute(c_str type, c_str attr);
|
|
bool exists(c_str type);
|
|
|
|
// Access vector rules
|
|
bool allow(c_str src, c_str tgt, c_str cls, c_str perm);
|
|
bool deny(c_str src, c_str tgt, c_str cls, c_str perm);
|
|
bool auditallow(c_str src, c_str tgt, c_str cls, c_str perm);
|
|
bool dontaudit(c_str src, c_str tgt, c_str cls, c_str perm);
|
|
|
|
// Extended permissions access vector rules
|
|
bool allowxperm(c_str src, c_str tgt, c_str cls, c_str range);
|
|
bool auditallowxperm(c_str src, c_str tgt, c_str cls, c_str range);
|
|
bool dontauditxperm(c_str src, c_str tgt, c_str cls, c_str range);
|
|
|
|
// Type rules
|
|
bool type_transition(c_str src, c_str tgt, c_str cls, c_str def, c_str obj = nullptr);
|
|
bool type_change(c_str src, c_str tgt, c_str cls, c_str def);
|
|
bool type_member(c_str src, c_str tgt, c_str cls, c_str def);
|
|
|
|
// File system labeling
|
|
bool genfscon(c_str fs_name, c_str path, c_str ctx);
|
|
|
|
// Magisk
|
|
void magisk_rules();
|
|
|
|
// Deprecate
|
|
bool create(c_str name) { return type(name, "domain"); }
|
|
|
|
protected:
|
|
policydb *db;
|
|
};
|