mirror of
https://github.com/topjohnwu/Magisk.git
synced 2024-11-24 18:45:28 +00:00
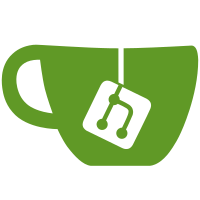
Magisk no longer interferes with any signals/info that were not created or caused by Magisk itself.
58 lines
1.4 KiB
C++
58 lines
1.4 KiB
C++
#include <sys/mount.h>
|
|
|
|
#include <magisk.hpp>
|
|
#include <utils.hpp>
|
|
#include <selinux.hpp>
|
|
#include <resetprop.hpp>
|
|
|
|
#include "magiskhide.hpp"
|
|
|
|
using namespace std;
|
|
|
|
static void lazy_unmount(const char* mountpoint) {
|
|
if (umount2(mountpoint, MNT_DETACH) != -1)
|
|
LOGD("hide: Unmounted (%s)\n", mountpoint);
|
|
}
|
|
|
|
void hide_daemon(int pid) {
|
|
if (fork_dont_care() == 0) {
|
|
hide_unmount(pid);
|
|
// Send resume signal
|
|
kill(pid, SIGCONT);
|
|
_exit(0);
|
|
}
|
|
}
|
|
|
|
#define TMPFS_MNT(dir) (mentry->mnt_type == "tmpfs"sv && str_starts(mentry->mnt_dir, "/" #dir))
|
|
|
|
void hide_unmount(int pid) {
|
|
if (pid > 0 && switch_mnt_ns(pid))
|
|
return;
|
|
|
|
LOGD("hide: handling PID=[%d]\n", pid);
|
|
|
|
vector<string> targets;
|
|
|
|
// Unmount dummy skeletons and /sbin links
|
|
targets.push_back(MAGISKTMP);
|
|
parse_mnt("/proc/self/mounts", [&](mntent *mentry) {
|
|
if (TMPFS_MNT(system) || TMPFS_MNT(vendor) || TMPFS_MNT(product) || TMPFS_MNT(system_ext))
|
|
targets.emplace_back(mentry->mnt_dir);
|
|
return true;
|
|
});
|
|
|
|
for (auto &s : reversed(targets))
|
|
lazy_unmount(s.data());
|
|
targets.clear();
|
|
|
|
// Unmount all Magisk created mounts
|
|
parse_mnt("/proc/self/mounts", [&](mntent *mentry) {
|
|
if (str_contains(mentry->mnt_fsname, BLOCKDIR))
|
|
targets.emplace_back(mentry->mnt_dir);
|
|
return true;
|
|
});
|
|
|
|
for (auto &s : reversed(targets))
|
|
lazy_unmount(s.data());
|
|
}
|