mirror of
https://github.com/portapack-mayhem/mayhem-firmware.git
synced 2025-07-12 05:58:33 +00:00
Update links to point to the new repo
parent
669d2f3a86
commit
00734dff52
@ -1,18 +1,18 @@
|
||||
|
||||
## About
|
||||
|
||||
Now we’re going to dive into something a little more complex and bring radios into the mix. If you’re not familiar with the LPC43xx please read up on the [Firmware Architecture](https://github.com/eried/portapack-mayhem/wiki/Firmware-Architecture) before continuing.
|
||||
Now we’re going to dive into something a little more complex and bring radios into the mix. If you’re not familiar with the LPC43xx please read up on the [Firmware Architecture](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Firmware-Architecture) before continuing.
|
||||
|
||||
So far we’ve only been dealing with application code with is ran on the M0 of the LPC43xx. Now we’re going to start working with the baseband side of the codebase which is ran on the LPC43xx’s M4 processor. Both of these processors use 8k worth of shared memory from `0x1008_8000` to `0x1008_a000` to pass messages to and from each other. The M0 controls **ALL** operations of the portapack while the M4 mostly handles the DSP and radio functions.
|
||||
|
||||
Complexitys aside with the two processors, accessing the HackRF's radio hardware has been simplified with helper classes such as the [`TransmitterModel`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/transmitter_model.cpp) and [`ReceiverModel`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/receiver_model.cpp). Both of these classes interface with the M4 baseband processes and gives us a more piratical way to control the radio.
|
||||
Complexitys aside with the two processors, accessing the HackRF's radio hardware has been simplified with helper classes such as the [`TransmitterModel`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/transmitter_model.cpp) and [`ReceiverModel`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/receiver_model.cpp). Both of these classes interface with the M4 baseband processes and gives us a more piratical way to control the radio.
|
||||
|
||||
Other classes and structs such as [`baseband api`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/baseband_api.cpp) and [`SharedMemory`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/portapack_shared_memory.hpp) also bridge the gap between the M0 and M4. Even though the M4's primary responsability is to handle DSP with the radio hardware the M0 can still be used to decode data. For example, classes found in `firmware/application/protocols/` like [`encoders`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/protocols/encoders.cpp) still send data too and from the two processors but also encodes and decodes messages at the higher level protocols.
|
||||
Other classes and structs such as [`baseband api`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/baseband_api.cpp) and [`SharedMemory`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/portapack_shared_memory.hpp) also bridge the gap between the M0 and M4. Even though the M4's primary responsability is to handle DSP with the radio hardware the M0 can still be used to decode data. For example, classes found in `firmware/application/protocols/` like [`encoders`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/protocols/encoders.cpp) still send data too and from the two processors but also encodes and decodes messages at the higher level protocols.
|
||||
|
||||
|
||||
## TX
|
||||
|
||||
The code bellow is an example OOK TX application using [`TransmitterModel`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/transmitter_model.cpp), [`encoders`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/protocols/encoders.cpp), and [`baseband`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/baseband_api.cpp).
|
||||
The code bellow is an example OOK TX application using [`TransmitterModel`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/transmitter_model.cpp), [`encoders`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/protocols/encoders.cpp), and [`baseband`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/baseband_api.cpp).
|
||||
|
||||
#### ui_newapp.hpp
|
||||
|
||||
@ -109,13 +109,13 @@ The code bellow is an example OOK TX application using [`TransmitterModel`](http
|
||||
|
||||
## RX
|
||||
|
||||
Building from the example code for TX lets talk about how the baseband processes are started on the M4. The application code on the M0 uses the baseband api `baseband::run_image` to tell the M4 to run a process. The baseband images are defined in [`spi_image.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/spi_image.hpp) as the struct `image_tag_t`. These structs and have a 4 char array tag being used as an ID. Below is an example `image_tag_t` for AFSK RX.
|
||||
Building from the example code for TX lets talk about how the baseband processes are started on the M4. The application code on the M0 uses the baseband api `baseband::run_image` to tell the M4 to run a process. The baseband images are defined in [`spi_image.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/spi_image.hpp) as the struct `image_tag_t`. These structs and have a 4 char array tag being used as an ID. Below is an example `image_tag_t` for AFSK RX.
|
||||
|
||||
```
|
||||
constexpr image_tag_t image_tag_afsk_rx { 'P', 'A', 'F', 'R' };
|
||||
```
|
||||
|
||||
Under [`firmware/baseband/CMakeLists.txt`](https://github.com/eried/portapack-mayhem/blob/next/firmware/baseband/CMakeLists.txt) the following code snippet shows how the baseband processes are linked to the images defined in [`spi_image.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/spi_image.hpp).
|
||||
Under [`firmware/baseband/CMakeLists.txt`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/baseband/CMakeLists.txt) the following code snippet shows how the baseband processes are linked to the images defined in [`spi_image.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/spi_image.hpp).
|
||||
|
||||
```
|
||||
### AFSK RX
|
||||
@ -125,7 +125,7 @@ set(MODE_CPPSRC
|
||||
DeclareTargets(PAFR afskrx)
|
||||
```
|
||||
|
||||
In `firmware/baseband`, process or "proc" code for the M4 processor like [`proc_afskrx.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/baseband/proc_afskrx.cpp) for example can be found here. These proc classes are ran by [`BasebandThread`](https://github.com/eried/portapack-mayhem/blob/next/firmware/baseband/baseband_thread.cpp). All proc classes inherent [`BasebandProcessor`](https://github.com/eried/portapack-mayhem/blob/next/firmware/baseband/baseband_processor.hpp) and must include the parent functions.
|
||||
In `firmware/baseband`, process or "proc" code for the M4 processor like [`proc_afskrx.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/baseband/proc_afskrx.cpp) for example can be found here. These proc classes are ran by [`BasebandThread`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/baseband/baseband_thread.cpp). All proc classes inherent [`BasebandProcessor`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/baseband/baseband_processor.hpp) and must include the parent functions.
|
||||
|
||||
#### baseband_processor.hpp
|
||||
```
|
||||
@ -153,7 +153,7 @@ private:
|
||||
};
|
||||
```
|
||||
|
||||
Now that we have a better idea how M0 can drive the M4 lets talk about the Messaging between the two processors. The [`Message`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/message.hpp) class found under `firmware/common/`. Common code is used both by application (M0) and baseband (M4). Messages are handled by EventDispatcher found in [`event_m4.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/baseband/event_m4.cpp) for the baseband code and [`event_m0.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/event_m0.cpp) for the application code. Within the same file [`firmware/commen/message.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/message.hpp) you can find definitions for spacific message classes and ID. Bellow is an example message class for AFSK RX.
|
||||
Now that we have a better idea how M0 can drive the M4 lets talk about the Messaging between the two processors. The [`Message`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/message.hpp) class found under `firmware/common/`. Common code is used both by application (M0) and baseband (M4). Messages are handled by EventDispatcher found in [`event_m4.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/baseband/event_m4.cpp) for the baseband code and [`event_m0.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/event_m0.cpp) for the application code. Within the same file [`firmware/commen/message.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/message.hpp) you can find definitions for spacific message classes and ID. Bellow is an example message class for AFSK RX.
|
||||
|
||||
|
||||
#### message.hpp
|
||||
@ -217,7 +217,7 @@ class AFSKDataMessage : public Message {
|
||||
};
|
||||
```
|
||||
|
||||
[`SharedMemory`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/portapack_shared_memory.hpp) found in `firmware/common/` is used to pass data inbetween the application code (M0) to the baseband code (M4). Below is an example from [`proc_afskrx.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/baseband/proc_afskrx.cpp) on how data is sent back to the application [`AFSKRxView`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/apps/ui_afsk_rx.cpp).
|
||||
[`SharedMemory`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/portapack_shared_memory.hpp) found in `firmware/common/` is used to pass data inbetween the application code (M0) to the baseband code (M4). Below is an example from [`proc_afskrx.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/baseband/proc_afskrx.cpp) on how data is sent back to the application [`AFSKRxView`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/apps/ui_afsk_rx.cpp).
|
||||
|
||||
#### proc_afskrx.cpp
|
||||
```
|
||||
|
@ -52,6 +52,6 @@ Its important to note all parameters must be delimited by a single space.
|
||||
010203040507 190953445220426c7565746f6f7468204c6f7720456e65726779 500
|
||||
|
||||
# References:
|
||||
BLE Rx App: https://github.com/eried/portapack-mayhem/wiki/Bluetooth-Low-Energy-Receiver
|
||||
BLE Rx App: https://github.com/portapack-mayhem/mayhem-firmware/wiki/Bluetooth-Low-Energy-Receiver
|
||||
|
||||
Advertisement PDU Types: https://novelbits.io/bluetooth-low-energy-advertisements-part-1/
|
@ -1,4 +1,4 @@
|
||||
The [Capture](https://github.com/eried/portapack-mayhem/wiki/Capture) app records into C16 files, and the Replay app read those files. _(Support for C8 format has since been added to firmware, but this chapter assumes that files were captured in C16 format; most instructions below apply to C8 files as well by just replacing the 16 with 8.)_
|
||||
The [Capture](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Capture) app records into C16 files, and the Replay app read those files. _(Support for C8 format has since been added to firmware, but this chapter assumes that files were captured in C16 format; most instructions below apply to C8 files as well by just replacing the 16 with 8.)_
|
||||
|
||||
As described in an [issue](https://github.com/sharebrained/portapack-hackrf/issues/139) in PortaPack's repo, this format consist of a tuple of 16 bits signed integers. The first number is I and second Q, forming a complex number. As a result, you get a tridimensional representation of the capture: the real and the imaginary parts in the file (I and Q) versus the time (defined by the sample rate, in this case in an adjacent TXT file with the same filename as the C16).
|
||||
|
||||
@ -36,7 +36,7 @@ As seen in the image above you'll need to bring in the original C16 file using t
|
||||
|
||||
The reason that the value 32768.0 is used to normalize the C16 capture is because int16 has a range of -32768 to +32767. 2^15 is 32768.0, so dividing int16 value by 2^15 gives a number that is normalized between -1 and +1.
|
||||
|
||||
This GRC script can be found at [`firmware/tools/convert_C16_to_complex.grc`](https://github.com/eried/portapack-mayhem/blob/next/firmware/tools/convert_C16_to_complex.grc)
|
||||
This GRC script can be found at [`firmware/tools/convert_C16_to_complex.grc`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/tools/convert_C16_to_complex.grc)
|
||||
|
||||
|
||||
|
||||
|
10
Capture.md
10
Capture.md
@ -12,7 +12,7 @@ The Key Items on the App that can be selected with the cursor and changed with t
|
||||
* **Step Size:** The selected step size of frequency adjustment carried out by the Rotary encoder.
|
||||
* **Gain:** Amp (0dB or 14dB), LNA(IF) (0-40),VGA(Baseband)(0-62)
|
||||
* **Rate:** The sample rate, which by its nature set the set bandwidth of capture. This is shown in the markers around the centre line of the waterfall display. The sample rate is variable from 12k5 to 2750K (*) in many steps. You need to ensure that the sample rate is more than twice the bandwidth of the signal you want to capture see [(Nyquist Principle / also called Shannon’s Law) ](https://en.wikipedia.org/wiki/Nyquist%E2%80%93Shannon_sampling_theorem)
|
||||
* **Format:** The user can select two file formats, of the recorded IQ data 16 / 8 bits : C16 (complex 16) or C8 (complex 8), and its related file extension ".C16" or "C8". (By default we are preselecting [C16](https://github.com/eried/portapack-mayhem/wiki/C16-format)).
|
||||
* **Format:** The user can select two file formats, of the recorded IQ data 16 / 8 bits : C16 (complex 16) or C8 (complex 8), and its related file extension ".C16" or "C8". (By default we are preselecting [C16](https://github.com/portapack-mayhem/mayhem-firmware/wiki/C16-format)).
|
||||
* **Trim:** If checked, the capture will attempt to be automatically trimmed to only contain the signal part. This is not reversable. Consider using the IQ Trim utility instead if you're not sure you can capture the signal again.
|
||||
|
||||
Note (*) : Currently , **for correct reliable Replay application ,you should ONLY use Capture App selecting any Bandwidth capture <= 1Mhz (but 500Khz is the recommended for majority micro SD cards compatibility because it requires a quite common average write speed in our system >2MB/sec,C16)**. From 600khz till 1Mhz (and 1.25 MHz) , you will need more fast and good quality micro SD card (with min average write speed in our system >3MB/sec (for BW=750khz,C16) , and >4MB/sec (for BW=1Mhz ,C16), (>5MB/sec for BW=1.25Mhz ,C16) and with as small as possible write random latency. (In the GUI , those correct bandwidth capture options appear with the Normal usual "REC" icon Background color, as user recommended BW capture options). If you face too much % dropped samples when recording , you can retry it reducing C16 to C8 , that also reduces the needed average write speed :2 (example 1Mhz rec will need average sd interface speed of 4MB/sec in C16 or 2MB/sec in C8).
|
||||
@ -28,7 +28,7 @@ Above 1.25Mhz till 5.5Mhz bandwith options (with YELLOW REC button background Ic
|
||||
|
||||
* **Record Button:** The red button shows as Rec or Stop. If record is selected then it will record the I/Q file. To the side of the Record Button is additional information that is shown for the recording file, % of Dropped Samples, total Recording Time Remaining. (As we mentioned above, for correct Replay operatons, please make sure to select a proper Capture bandwith option , with normal black background "REC" icon , not the yellow one.)
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
* File Name (as above example , BBD_0109.C16, based on the selected format C16)
|
||||
@ -39,12 +39,12 @@ From nightly 23-08-19 onwards, we revised and extended a good functionality of
|
||||
If you adjust correctly the GAIN and LNA and center freq. it is possible to capture good bit streams in C16 / C8 format ,
|
||||
Pls. find attached some screenshot examples , capturing the same tuned AM MF band broadcasting with a BW aprox of 16Khz , using an upconverter ,
|
||||
|
||||

|
||||

|
||||

|
||||

|
||||
|
||||
Depending on the tuned frequency, (mainly visible in 25k, 32k, 50k and 75k) we may got a random strange left picture effect -on the screen, it seems not affecting to the recorded files.C16 or .C8 - with “vertical stripes” , this can be easily corrected, just readjusting the center frequency some Hz up or down , till trying to have full convergence of these vertical red carriers to the central unique one , with +-10Hz steps.
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
Please check the video below for HackRF PortaPack Capture/Replay functionality.
|
||||
|
@ -1,16 +1,16 @@
|
||||
There are severals ways to compile the firmware. As the traditional way, check the original [Building from Source](https://github.com/furrtek/portapack-havoc/wiki/Building-from-source) document, however, Docker is recommended because it provides a very clean way to go from source to a `.bin` file.
|
||||
|
||||
[Using Docker and Kitematic](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#using-docker-hub-and-kitematic)
|
||||
[Using Docker and Kitematic](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#using-docker-hub-and-kitematic)
|
||||
|
||||
[Docker command-line reference](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#docker---command-line-reference)
|
||||
[Docker command-line reference](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#docker---command-line-reference)
|
||||
|
||||
[Using Buddyworks and other CI platforms](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#using-buddyworks-and-other-ci-platforms)
|
||||
[Using Buddyworks and other CI platforms](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#using-buddyworks-and-other-ci-platforms)
|
||||
|
||||
[Notes for Buddy.Works (and other CI platforms)](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#notes-for-buddyworks-and-other-ci-platforms)
|
||||
[Notes for Buddy.Works (and other CI platforms)](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#notes-for-buddyworks-and-other-ci-platforms)
|
||||
|
||||
[Using ARM on Debian host](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#using-arm-on-debian)
|
||||
[Using ARM on Debian host](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#using-arm-on-debian)
|
||||
|
||||
[All in one script for ARM on Debian host](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#all-in-one-script-for-arm-on-debian-host)
|
||||
[All in one script for ARM on Debian host](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#all-in-one-script-for-arm-on-debian-host)
|
||||
|
||||
# Using Docker Hub and Kitematic
|
||||
|
||||
@ -58,7 +58,7 @@ Make sure they look like this:
|
||||
|
||||
After that click run!
|
||||
|
||||
_Note: Come across a `/usr/bin/env: ‘python\r’: No such file or directory` error? RTFM and go back to step 2 https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#step-2-clone-the-repository_
|
||||
_Note: Come across a `/usr/bin/env: ‘python\r’: No such file or directory` error? RTFM and go back to step 2 https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#step-2-clone-the-repository_
|
||||
|
||||
|
||||
## Step 4: Compile!
|
||||
@ -74,7 +74,7 @@ If you have additional questions, please check [this guide](Using-MAC-OS).
|
||||
If you are inclined for using the command line, you can try the following:
|
||||
* Clone the repository and submodules:
|
||||
```
|
||||
git clone https://github.com/eried/portapack-mayhem.git
|
||||
git clone https://github.com/portapack-mayhem/mayhem-firmware.git
|
||||
cd portapack-mayhem
|
||||
git submodule update --init --recursive
|
||||
```
|
||||
@ -135,7 +135,7 @@ You can use the following _yml _as your template for your CI platform (pipeline
|
||||
|
||||
## Notes for Buddy.Works (and other CI platforms)
|
||||
|
||||
If you decide to [ignore this guide](https://github.com/eried/portapack-mayhem/issues/75) and use the command line instead, you will need to include submodules
|
||||
If you decide to [ignore this guide](https://github.com/portapack-mayhem/mayhem-firmware/issues/75) and use the command line instead, you will need to include submodules
|
||||
|
||||
git clone --recurse-submodules --remote-submodules <url>
|
||||
|
||||
@ -192,7 +192,7 @@ some compiling errors to check it's better to call it without '-j 8')
|
||||
## 4. Clone the eried's mayhem repository from GitHub (if not done before) into /opt
|
||||
|
||||
cd /opt
|
||||
sudo git clone --recurse-submodules https://github.com/eried/portapack-mayhem.git
|
||||
sudo git clone --recurse-submodules https://github.com/portapack-mayhem/mayhem-firmware.git
|
||||
|
||||
## 5. Give permission for the portapack-mayhem directory to your user
|
||||
|
||||
@ -221,4 +221,4 @@ If you want to have all these commands in one go, go to https://github.com/GullC
|
||||
# Other Toolsets
|
||||
- [Linux Aarch64 gcc-arm-none-eabi-9-2020-q2-update-aarch64-linux.tar.bz2](https://developer.arm.com/-/media/Files/downloads/gnu-rm/9-2020q2/gcc-arm-none-eabi-9-2020-q2-update-aarch64-linux.tar.bz2?revision=7166404a-b4f5-4598-ac75-e5f8b90abb09&rev=7166404ab4f54598ac75e5f8b90abb09&hash=07EE5CACCCE77E97A1A8C049179D77EACA5AD4E5)
|
||||
- [Toolsets archive](https://developer.arm.com/downloads/-/gnu-rm)
|
||||
- [Compilation error after changing toolsets](https://github.com/eried/portapack-mayhem/issues/420)
|
||||
- [Compilation error after changing toolsets](https://github.com/portapack-mayhem/mayhem-firmware/issues/420)
|
@ -42,7 +42,7 @@ _This will automatically add the path of arm toolchain to the ``PATH`` of your d
|
||||
# 3. Clone your repo to local and satisfying the sub-module
|
||||
```
|
||||
cd ~
|
||||
git clone https://github.com/eried/portapack-mayhem.git
|
||||
git clone https://github.com/portapack-mayhem/mayhem-firmware.git
|
||||
cd portapack-mayhem
|
||||
git submodule update --init --recursive
|
||||
```
|
||||
|
@ -43,7 +43,7 @@ Upon the successful downloading and combining of your custom map refer to the fo
|
||||
* And the package "Pillow" installed (`pip install Pillow`)
|
||||
|
||||
## Coding
|
||||
To see your actual custom map, you need to do a change/edit of code. This change would occur in line 197 of file ui_geomap.cpp which is located at /firmware/application/ui/ or [here](https://github.com/eried/portapack-mayhem/blob/f161e85f960cff0c166173f4f7a4244b8c625375/firmware/application/ui/ui_geomap.cpp#L197) for ease of reference. The function that's being changed is `void GeoMap::move(const float lon, const float lat)`.
|
||||
To see your actual custom map, you need to do a change/edit of code. This change would occur in line 197 of file ui_geomap.cpp which is located at /firmware/application/ui/ or [here](https://github.com/portapack-mayhem/mayhem-firmware/blob/f161e85f960cff0c166173f4f7a4244b8c625375/firmware/application/ui/ui_geomap.cpp#L197) for ease of reference. The function that's being changed is `void GeoMap::move(const float lon, const float lat)`.
|
||||
|
||||
What I replaced this function with is as follow:
|
||||
|
||||
|
@ -61,7 +61,7 @@ The following structure is the base of any application. Following the general st
|
||||
|
||||
## Entry in the main menu
|
||||
|
||||
For triggering your new app, you need to add an entry on the main menu. This menu resides on [`firmware\application\ui_navigation.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/ui_navigation.cpp). Check the current entries, and add a new one in a section you think is suitable for your new app.
|
||||
For triggering your new app, you need to add an entry on the main menu. This menu resides on [`firmware\application\ui_navigation.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/ui_navigation.cpp). Check the current entries, and add a new one in a section you think is suitable for your new app.
|
||||
|
||||
#### firmware\application\ui_navigation.cpp
|
||||
```
|
||||
@ -98,12 +98,12 @@ set(CPPSRC
|
||||
)
|
||||
```
|
||||
|
||||
In this moment you should be able to compile and test the app in your device. For your reference here's the link to the [Compile-firmware](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware) wiki. The new app should appear in the menu you picked in `ui_navigation.cpp`.
|
||||
In this moment you should be able to compile and test the app in your device. For your reference here's the link to the [Compile-firmware](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware) wiki. The new app should appear in the menu you picked in `ui_navigation.cpp`.
|
||||
|
||||
## Adding Widgets and Basic Functionality
|
||||
|
||||
Widgets are the elements that compose the UI of your custom app. Widgets are defined inside [`firmware\common\ui_widget.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui_widget.hpp) and widget functions can be found inside [`firmware\common\ui_widget.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui_widget.cpp). In order to be able to use them, you must `#include "ui_widget.hpp"` into your app .hpp file.
|
||||
There are different type of widget. here you will find a list of available widget, with their respecting constructor. For all the methods available, you should go and see the [ui_widget.hpp](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui_widget.hpp) file.
|
||||
Widgets are the elements that compose the UI of your custom app. Widgets are defined inside [`firmware\common\ui_widget.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui_widget.hpp) and widget functions can be found inside [`firmware\common\ui_widget.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui_widget.cpp). In order to be able to use them, you must `#include "ui_widget.hpp"` into your app .hpp file.
|
||||
There are different type of widget. here you will find a list of available widget, with their respecting constructor. For all the methods available, you should go and see the [ui_widget.hpp](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui_widget.hpp) file.
|
||||
|
||||
### Attach a Generic Widget to Your Application
|
||||
|
||||
@ -121,7 +121,7 @@ add_children({
|
||||
});
|
||||
```
|
||||
|
||||
There are several different widgets that we're going to use for our new app. A more complete list can be found on the [Widgets](https://github.com/eried/portapack-mayhem/wiki/Widgets) wiki. More might be added so you should always go and check whether new widgets have been added or not.
|
||||
There are several different widgets that we're going to use for our new app. A more complete list can be found on the [Widgets](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Widgets) wiki. More might be added so you should always go and check whether new widgets have been added or not.
|
||||
|
||||
|
||||
#### Button
|
||||
@ -162,7 +162,7 @@ Labels my_label{
|
||||
};
|
||||
```
|
||||
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui.hpp).
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui.hpp).
|
||||
|
||||
In `apps/ui_newapp.cpp` you'll need to add the `my_label` pointer to add_child() or add_children():
|
||||
```
|
||||
|
@ -1,23 +1,23 @@
|
||||
**This is intended for maintainers only**
|
||||
|
||||
## Setting up the repo
|
||||
To create a prod/stable release, first go to https://github.com/eried/portapack-mayhem/tree/next/.github/workflows and edit the `past_version.txt` and the `version.txt` files.
|
||||
To create a prod/stable release, first go to https://github.com/portapack-mayhem/mayhem-firmware/tree/next/.github/workflows and edit the `past_version.txt` and the `version.txt` files.
|
||||
|
||||
* `past_version.txt` needs to be the current release (I.E if you are creating a new release and the old/current stable release is `v1.0.0` and you want the new version to be `v1.1.0`, then past version needs to be `v1.0.0`).
|
||||
* `version.txt` needs to be the version you want for your new release. So from the example above, that would be `v1.1.0`.
|
||||
|
||||
## Running the pipeline
|
||||
Once that is done then you need to create the draft stable release. You can do this by running the stable release pipeline on `next` branch https://github.com/eried/portapack-mayhem/actions/workflows/create_stable_release.yml
|
||||
Once that is done then you need to create the draft stable release. You can do this by running the stable release pipeline on `next` branch https://github.com/portapack-mayhem/mayhem-firmware/actions/workflows/create_stable_release.yml
|
||||
|
||||
This should take around 7-15 minutes.
|
||||
|
||||
## Editing the draft release
|
||||
This then create a draft release that you should be able to see in releases at the top https://github.com/eried/portapack-mayhem/releases
|
||||
This then create a draft release that you should be able to see in releases at the top https://github.com/portapack-mayhem/mayhem-firmware/releases
|
||||
|
||||
Next, make sure you test it on your own device before going any further. This is to ensure it created it correctly and that there are no last minute bugs.
|
||||
|
||||
|
||||
**Below is no longer needed after https://github.com/eried/portapack-mayhem/pull/1022**
|
||||
**Below is no longer needed after https://github.com/portapack-mayhem/mayhem-firmware/pull/1022**
|
||||
|
||||
~~Once tested, you then need to manually update the files in the `mayhem_nightly_X_COPY_TO_SDCARD.zip` folder. This is because there are some files (like the world map) that are too big to host on the GitHub repo, so they need to be manually added into the zip folder. So to do this, download `mayhem_nightly_X_COPY_TO_SDCARD.zip` from the draft release and then download `mayhem_nightly_PREVIOUS_RELEASE_X_COPY_TO_SDCARD.zip` from the previous stable release. Copy the files from the previous to the new release making sure to not overwrite any files (As we are just wanting to add the ones missing).~~
|
||||
|
||||
|
@ -4,4 +4,4 @@ To partially work around a dead or missing coin cell battery, persistent memory
|
||||
|
||||
Those with an H2 model and soldering skills might want to consider adding a larger CR2032 battery holder such as the one below (attached with double-stick foam tape), to lengthen the time that the coin battery lasts by about 6X:
|
||||
|
||||

|
||||

|
||||
|
@ -337,7 +337,7 @@ Before a microcontroller’s SWD port is serviceable, an initialization sequence
|
||||
|
||||
(1) We need to buy a MULTI PURPOSE USB TO UART FT232H MODULE (RS232, RS422 o RS485), USB a FIFO, USB a FT1248, USB a JTAG, USB a SPI, USB a I2C))
|
||||
|
||||

|
||||

|
||||
|
||||
Features
|
||||
Chip: FT232H
|
||||
@ -359,7 +359,7 @@ Pin Number: 24 Pins
|
||||
|
||||
(2) And prepare the proper interface cables to connect 4 wires + GND from the Hackrf connector .
|
||||
|
||||

|
||||

|
||||
|
||||
From this 10 pin Hackrf JTAG connector , we need to connect (4 wires + GND) to the FT232H module. (the yellow pins, in below JTAG table pinout)
|
||||
|
||||
@ -375,23 +375,23 @@ From this 10 pin Hackrf JTAG connector , we need to connect (4 wires + GND) to
|
||||
|
||||
* GND - GND
|
||||
|
||||

|
||||

|
||||
|
||||
I used a bridge Hackrf female cable connector PCB , to make those easy connections .
|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
(3) Make sure to have installed the OpenOCD package.
|
||||
@ -424,7 +424,7 @@ Info : Listening on port 3334 for gdb connections"
|
||||
|
||||
It is matching with the expected M0, M4 JTAG ID,
|
||||
|
||||

|
||||

|
||||
|
||||
then , now you are ready to proceed with vscode debug integration,
|
||||
|
||||
@ -434,7 +434,7 @@ then , now you are ready to proceed with vscode debug integration,
|
||||
|
||||
Note , it can be added to the previous SWD Jeff debug module block , so in this example , my launch.json file , will have 3 config blocks , and every time before debugging , you will need to connect Jeff SWD or FT232H JTAG , and select the proper one, and click play
|
||||
|
||||

|
||||

|
||||
|
||||
When you select M4 debug
|
||||
→ you will need to update in the launch.json file the proper .elf file , according to the selected proc_m4_file.ccp to be debug. (example : "program": "${workspaceRoot}/build/firmware/baseband/baseband_weather.elf",)
|
||||
|
10
Debug.md
10
Debug.md
@ -9,13 +9,13 @@ Gives information on the peripherals:
|
||||
* SI5351C
|
||||
* WM8731 or Ak4951 (depending on the IC audio codec detected in your PP brd)
|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||
Note : Recently (Manufacturing year: 2023) GSG introduced the new r9 Hackrf board version (already compatible with Hackrf and Portapack-Mayhem fw's).
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
|
||||
@ -27,7 +27,7 @@ Starting with HackRF One r6, hardware revisions are detected by firmware and rep
|
||||
|
||||
Thanks to GSG developers and our Mayhem git admin , we merged their commit about all those r9 hw support in our Portapack Mayhem Debug menu tool. And now you can also easily detect that r9 version without disassembling the boards :
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
## Temperature
|
||||
@ -36,7 +36,7 @@ Data is provided by the MAX 2837 (or MAX 2839) on chip digital temperature sens
|
||||
## Buttons Test
|
||||
This shows when either the buttons are pressed, the encode knob is turned or the screen is touched. It can also show if the encoder when turned is cleanly stepping the states as it is turned. Encoder sensitivity is now adjustable in Settings, and the encoder can be desoldered and replaced with a better-quality version if it has issues. The test screen also has an option for testing the "long press" feature which is applicable to the directional keys and the DFU switch only.
|
||||
|
||||

|
||||

|
||||
|
||||
## Touch Test
|
||||
Allows testing the Touch Screen calibration (and your artistic skill) by drawing on the screen using a stylus. The following controls are available:
|
||||
|
@ -9,26 +9,26 @@ Try boot into DFU mode by:
|
||||
|
||||
3. Check Windows device manager and see if you have LPC showing up
|
||||
|
||||

|
||||

|
||||
|
||||
4. If it shows up then make sure you download the latest version of **mayhem_vx.x.x_FIRMWARE.zip** from GitHub here https://github.com/eried/portapack-mayhem/releases/latest. You can then run "dfu_hackrf_one.bat". Now double check the file name as they look quite similar.
|
||||
4. If it shows up then make sure you download the latest version of **mayhem_vx.x.x_FIRMWARE.zip** from GitHub here https://github.com/portapack-mayhem/mayhem-firmware/releases/latest. You can then run "dfu_hackrf_one.bat". Now double check the file name as they look quite similar.
|
||||
5. Once it opens, press enter on your keyboard and you should see the following:
|
||||
|
||||

|
||||

|
||||
|
||||
6. Once that is done, you need to check device manager and see if you can now see a new device showing up
|
||||
|
||||

|
||||

|
||||
|
||||
7. If this device is showing up, then you can now run "flash_portapack_mayhem.bat" and press enter. You should now see the following:
|
||||
|
||||

|
||||

|
||||
|
||||
8. Once that is done, you should be able to press the reset button and your device will boot up. If not, then please refer to the wiki here: [Won't boot](https://github.com/eried/portapack-mayhem/wiki/Won't-boot
|
||||
8. Once that is done, you should be able to press the reset button and your device will boot up. If not, then please refer to the wiki here: [Won't boot](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Won't-boot
|
||||
|
||||
|
||||
|
||||
### Unknown device
|
||||
If you see **Unknown device** then you will need to install your device drivers.
|
||||
|
||||

|
||||

|
@ -1,4 +1,4 @@
|
||||
The **H1** is the [original](https://github.com/eried/portapack-mayhem/tree/master/hardware/portapack_h1) design, which is well documented and in the public domain.
|
||||
The **H1** is the [original](https://github.com/portapack-mayhem/mayhem-firmware/tree/master/hardware/portapack_h1) design, which is well documented and in the public domain.
|
||||
|
||||
PortaPack **H2** is a modification based on the last (from an unknown origin, no public design documentation available) incorporating some enhancements:
|
||||
|
||||
@ -40,14 +40,14 @@ This is a non trivial difference, compared against H1 design where the speaker o
|
||||
|
||||
Since the firmware is shared between H1 and H2, on PortaPack H2 you end up powering TWO speaker amplifiers: The one inside AK4951 CODEC IC, and the extra 3W speaker amplifier.
|
||||
|
||||
## H2+ [Variations](https://github.com/eried/portapack-mayhem/wiki/PortaPack-Versions)
|
||||
## H2+ [Variations](https://github.com/portapack-mayhem/mayhem-firmware/wiki/PortaPack-Versions)
|
||||
At least some of the PortaPack "H2+" variations have the following audio differences:
|
||||
|
||||
A **WM8731** audio CODEC may be installed in place of the **AK4951**. The WM8731 IC has no speaker amplifier, for one difference.
|
||||
|
||||
An **INS8002E** or **LTK8002D** audio amplifier may be installed in place of the **CS8122S**.
|
||||
|
||||
On some H2+ variations, audio volume is low, and inserting a headphone plug does not electrically disable the speaker output (without [additional hardware modifications](https://github.com/eried/portapack-mayhem/wiki/H2-Plus-speaker-modifications)).
|
||||
On some H2+ variations, audio volume is low, and inserting a headphone plug does not electrically disable the speaker output (without [additional hardware modifications](https://github.com/portapack-mayhem/mayhem-firmware/wiki/H2-Plus-speaker-modifications)).
|
||||
|
||||
# Power Supply
|
||||
|
||||
|
@ -18,7 +18,7 @@ Selecting certain types of files launch a viewer for the content. Use the right
|
||||
|
||||
## Buttons
|
||||
|
||||

|
||||

|
||||
|
||||
When an item has been selected, the following commands are available in the toolbar.
|
||||
- Rename - rename the file or folder.
|
||||
@ -53,8 +53,8 @@ When an item has been selected, the following commands are available in the tool
|
||||
- SETTINGS - holds saved App Settings (.ini files), PMEM_FILEFLAG and pmem_settings (only if persistent memory is being saved on the SD card), DATE_FILEFLAG (for incrementing pseudo-date only when coin cell battery is dead), and blacklist (list of apps to be disabled [1.8.0 firmware])
|
||||
- SPLASH - holds example splash screen files. To select a new splash screen, open a BMP file with FileMan and press the Right button to copy it to the root directory as your new splash.bmp file ("Show Splash" also needs to be enabled on the Settings->User Interface page).
|
||||
|
||||

|
||||

|
||||

|
||||

|
||||
|
||||
|
||||
## Known Issues
|
||||
|
@ -10,13 +10,13 @@ There is a Discord server ["HackRF PortaPack Mayhem"](https://discord.gg/tuwVMv3
|
||||
# Power on/off
|
||||
The original H1 powers instantly when you plug a power supply to the USB port. To turn it off, just unplug it. Similar to the issues with some USB cables while [upgrading the firmware](Update-firmware), the quality of your cable might affect the performance.
|
||||
|
||||
To power on/off the H2, you need to hold the middle button (knob or pushbutton) for few seconds. See more details [here](https://github.com/eried/portapack-mayhem/wiki/Powering-the-PortaPack).
|
||||
To power on/off the H2, you need to hold the middle button (knob or pushbutton) for few seconds. See more details [here](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Powering-the-PortaPack).
|
||||
|
||||
For some H2+, click the knob to power on, double-click the knob to power off.
|
||||
|
||||
# Extra functionality (H2 and H2+)
|
||||
## Charging
|
||||
This version can charge the internal lipo battery via the USB. There is a led indicator that turns off when the charging is done, but it might flicker.On some models (H2+) there are 4 leds below the knob that represent the state of the battery charge 25%,50%,75%,100%.When charging one will flash dependant on the current charge state of the battery. See more details [here](https://github.com/eried/portapack-mayhem/wiki/Powering-the-PortaPack).
|
||||
This version can charge the internal lipo battery via the USB. There is a led indicator that turns off when the charging is done, but it might flicker.On some models (H2+) there are 4 leds below the knob that represent the state of the battery charge 25%,50%,75%,100%.When charging one will flash dependant on the current charge state of the battery. See more details [here](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Powering-the-PortaPack).
|
||||
|
||||
## Battery life
|
||||
An internal battery between 1000 and 2500 mAh should last several hours of use, depending App use. The standby consumption is [very low](https://github.com/eried/Research/blob/master/HackRF/PortaPack/h2_standby_consumption.jpg), around 52 µA, so you do not need to worry to remove/disconnect the battery in normal circumstances.
|
||||
@ -29,4 +29,4 @@ to preserve settings and time calendar between usages, some versions a high prec
|
||||
|
||||
Although the Mayhem firmware gives you the possibility to directly use a lot of functions on the field standalone , without a computer, it also provides a “HackRF mode” which enables the user to start a version of the original GSG firmware and use your HackRF via USB, controlled by a computer. However if you separate the two boards, you wont be able to use the menu GUI and enable “HackRF mode”, so if you want to use your HackRF board alone (detached), that way you'll need to flash it with the GSG firmware.
|
||||
|
||||
In case you bought a PortaPack separately or want to upgrade your firmware, check out our [Update firmware](https://github.com/eried/portapack-mayhem/wiki/Update-firmware) page!
|
||||
In case you bought a PortaPack separately or want to upgrade your firmware, check out our [Update firmware](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Update-firmware) page!
|
@ -2,7 +2,7 @@ This application allows you to install a new firmware on your PortaPack. The Fl
|
||||
|
||||
# The Flash Utility
|
||||
|
||||
* **Step 1:** Compile or download the image. For example the latest nightly build from https://github.com/eried/portapack-mayhem/releases. If you download and unzip the latest mayhem_v#.#.#_COPY_TO_SDCARD.zip contents to your SD card, the latest firmware image will be found in the FIRMWARE folder (this method also updates the external app files in the APPS folder as well as data files needed for other apps).
|
||||
* **Step 1:** Compile or download the image. For example the latest nightly build from https://github.com/portapack-mayhem/mayhem-firmware/releases. If you download and unzip the latest mayhem_v#.#.#_COPY_TO_SDCARD.zip contents to your SD card, the latest firmware image will be found in the FIRMWARE folder (this method also updates the external app files in the APPS folder as well as data files needed for other apps).
|
||||
* **Step 2:** If you download only the file mayhem_v#.#.#_FIRMWARE.zip, extract the portapack-h1_h2-mayhem.bin file and place it in the FIRMWARE folder of your SD card. (The SD card may be physically plugged into your computer, or left installed in the PortaPack using the "SD over USB" app and connected with a USB cable.) See note below regarding external apps.
|
||||
* **Step 3:** Start the Flash Utility and select the new .bin file.
|
||||
|
||||
|
@ -2,7 +2,7 @@ Many PortaPacks on AliExpress come with a metal or plastic enclosure. Enclosure
|
||||
|
||||
You can 3D-print the following enclosure for your Portapack (click the pictures to get the design files):
|
||||
|
||||
[<img src="https://raw.githubusercontent.com/eried/portapack-mayhem/master/docs/images/h2_front.jpg" height="300">
|
||||
[<img src="https://raw.githubusercontent.com/portapack-mayhem/mayhem-firmware/master/docs/images/h2_front.jpg" height="300">
|
||||
](https://www.thingiverse.com/thing:4260973)
|
||||
|
||||
Encoder knob:
|
||||
@ -11,7 +11,7 @@ Encoder knob:
|
||||
|
||||
And the following magnetic cover (consider that most of the commercial cases that clone the design of the enclosure include plastic screws, hence this wont work):
|
||||
|
||||
[<img src="https://raw.githubusercontent.com/eried/portapack-mayhem/master/docs/images/h2_cover.jpg" height="300">](https://www.thingiverse.com/thing:4278961)
|
||||
[<img src="https://raw.githubusercontent.com/portapack-mayhem/mayhem-firmware/master/docs/images/h2_cover.jpg" height="300">](https://www.thingiverse.com/thing:4278961)
|
||||
|
||||
If you do not own a 3d printer, you have other options:
|
||||
|
||||
|
@ -1,3 +1,3 @@
|
||||
The HackRF is the board under your screen (unless you are using it standalone) designed by [Great Scott Gadgets](https://greatscottgadgets.com/). Check the versions on the official [HackRF documentation](https://hackrf.readthedocs.io/en/latest/list_of_hardware_revisions.html).
|
||||
|
||||
For the Mayhem firmware, check the [PortaPack versions](https://github.com/eried/portapack-mayhem/wiki/PortaPack-Versions).
|
||||
For the Mayhem firmware, check the [PortaPack versions](https://github.com/portapack-mayhem/mayhem-firmware/wiki/PortaPack-Versions).
|
@ -2,7 +2,7 @@
|
||||
|
||||
<img src="img/hw_overview_h2_front.png" height="500"> <img src="img/hw_overview_h2_back.png" height="500">
|
||||
|
||||
1. [Antenna](https://github.com/eried/portapack-mayhem/wiki/Antennas#types-of-antenna) (the connector is a _female SMA_, so the antenna needs to be _male SMA_, and not _RPSMA_)
|
||||
1. [Antenna](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Antennas#types-of-antenna) (the connector is a _female SMA_, so the antenna needs to be _male SMA_, and not _RPSMA_)
|
||||
2. Encoder thumb wheel (on the H1 has a different layout, but the same functionality), notice that pushing the wheel down has the same effect as pressing the center push button
|
||||
3. Directional pushbuttons and Enter/ON/OFF in the center
|
||||
4. [CLK IN](https://hackrf.readthedocs.io/en/latest/external_clock_interface.html)
|
||||
|
2
Home.md
2
Home.md
@ -1,4 +1,4 @@
|
||||
Thanks for buying your HackRF+PortaPack device. _That_ is what I would say if I was actually the one selling them as the intro line for the documentation, but I am not. I am just trying to document the great work of a lot of people involved in this project, listed [here](https://github.com/eried/portapack-mayhem/blob/c9eb2b16ca1b8cc50935c1b72af8f5438f582268/firmware/application/apps/ui_about_simple.cpp#L19).
|
||||
Thanks for buying your HackRF+PortaPack device. _That_ is what I would say if I was actually the one selling them as the intro line for the documentation, but I am not. I am just trying to document the great work of a lot of people involved in this project, listed [here](https://github.com/portapack-mayhem/mayhem-firmware/blob/c9eb2b16ca1b8cc50935c1b72af8f5438f582268/firmware/application/apps/ui_about_simple.cpp#L19).
|
||||
|
||||
The following documentation is split in two parts. The User manual intends to emulate a typical instruction manual for the end user, while the Developer manual documents all the apis available in order to write custom apps.
|
||||
|
||||
|
@ -26,7 +26,7 @@ So, setup the environment and compile the code as instructed below. Modify somet
|
||||
|
||||
### Fork the repository
|
||||
1. Download and install [Github desktop](https://desktop.github.com/)
|
||||
2. Log to Github, open the [repository](https://github.com/eried/portapack-mayhem) and click **Fork** on the top right
|
||||
2. Log to Github, open the [repository](https://github.com/portapack-mayhem/mayhem-firmware) and click **Fork** on the top right
|
||||
3. In your Fork, Click **Clone** and **Open in Desktop**
|
||||
4. Follow the rest of instructions in Github desktop
|
||||
|
||||
|
@ -1,6 +1,6 @@
|
||||
_**Note: This is for Windows only**_
|
||||
|
||||
The steps on the [Compile-firmware](https://github.com/eried/portapack-mayhem/wiki/Compile-firmware#step-3-prepare-the-docker-container) page work fine for compiling, but you may find it takes around 35 minutes each time.
|
||||
The steps on the [Compile-firmware](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Compile-firmware#step-3-prepare-the-docker-container) page work fine for compiling, but you may find it takes around 35 minutes each time.
|
||||
In this guide I will show you how to get that time down to 2 seconds - 1 minute depending on your hardware.
|
||||
|
||||
|
||||
@ -44,7 +44,7 @@ After Step 5 do this:
|
||||
|
||||
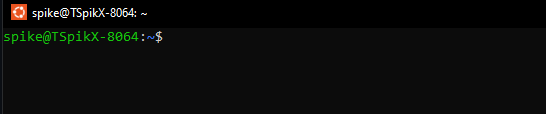
|
||||
|
||||
**Step 5.2)** Do a sudo git clone --recurse-submodules `sudo git clone --recurse-submodules https://github.com/eried/portapack-mayhem.git`
|
||||
**Step 5.2)** Do a sudo git clone --recurse-submodules `sudo git clone --recurse-submodules https://github.com/portapack-mayhem/mayhem-firmware.git`
|
||||
|
||||
_(Note if it still doesn't compile, you might need to run ```git submodule update --init --recursive```)_
|
||||
|
||||
|
@ -1,6 +1,6 @@
|
||||
IQ Trim allows you to trim "radio silence" from the beginning and end of a C16 or C8 capture file.
|
||||
|
||||

|
||||

|
||||
|
||||
## UI Components
|
||||
|
||||
|
@ -61,4 +61,4 @@ After a crash, pressing the DFU button will display the M0 core's stack contents
|
||||
## More useful Information
|
||||
|
||||
* [How to debug a HardFault on an ARM Cortex-M on interrupt.memfault.com](https://interrupt.memfault.com/blog/cortex-m-hardfault-debug)
|
||||
* You can read the related PR with instructions [here](https://github.com/eried/portapack-mayhem/pull/830)
|
||||
* You can read the related PR with instructions [here](https://github.com/portapack-mayhem/mayhem-firmware/pull/830)
|
||||
|
@ -31,7 +31,7 @@ It should be noted in the PortaPack, **the RF settings are called either “Amp
|
||||
|
||||
A good default setting for RX is to start with is RF (Amp=0) i.e. RF amp is off, IF=16, Baseband=16. Increase or decrease the IF and baseband gain controls roughly equally to find the best settings for your situation. Turn on the RF amp if you need help picking up weak signals. If your gain settings are too low, your signal may be buried in the noise. If one or more of your gain settings is too high, you may see distortion (look for unexpected frequencies that pop up when you increase the gain) or the noise floor may be amplified more than your signal.
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
To get the optimal level use the radio saturation monitor in the radio section of the [DFU Overlay](DFU-overlay).
|
||||
|
@ -1,4 +1,4 @@
|
||||
This tool creates [airlines.db](https://github.com/eried/portapack-mayhem/blob/next/sdcard/ADSB/airlines.db).
|
||||
This tool creates [airlines.db](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/sdcard/ADSB/airlines.db).
|
||||
This database is used to find the _airline name_ and _country_ based on the three-letter ICAO code.
|
||||
This database is currently only used by ADS-B receiver application.
|
||||
|
||||
@ -7,6 +7,6 @@ https://raw.githubusercontent.com/kx1t/planefence-airlinecodes/main/airlinecodes
|
||||
|
||||
## Build database
|
||||
* Download the latest version from location above.
|
||||
* Download script [make_airlines_db.py](https://github.com/eried/portapack-mayhem/blob/next/firmware/tools/make_airlines_db/make_airlines_db.py).
|
||||
* Download script [make_airlines_db.py](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/tools/make_airlines_db/make_airlines_db.py).
|
||||
* Put them in the same folder and run script. Note: Python 3 required.
|
||||
* Copy database to /ADSB folder on sdcard.
|
||||
|
@ -1,4 +1,4 @@
|
||||
This tool creates [icao24.db](https://github.com/eried/portapack-mayhem/blob/next/sdcard/ADSB/icao24.db).
|
||||
This tool creates [icao24.db](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/sdcard/ADSB/icao24.db).
|
||||
|
||||
This database is used to find aircraft-related information based on the 24-bit ICAO transponder code.
|
||||
This database is currently only used by ADS-B receiver application.
|
||||
@ -8,5 +8,5 @@ https://opensky-network.org/datasets/metadata/aircraftDatabase.csv
|
||||
|
||||
## Build database
|
||||
* Copy file from: https://opensky-network.org/datasets/metadata/aircraftDatabase.csv
|
||||
* Run Python 3 [script](https://github.com/eried/portapack-mayhem/blob/next/firmware/tools/make_airlines_db/make_icao24_db.py): `./make_icao24_db.py`
|
||||
* Run Python 3 [script](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/tools/make_airlines_db/make_icao24_db.py): `./make_icao24_db.py`
|
||||
* Copy file to /ADSB folder on SDCARD
|
||||
|
@ -3,7 +3,7 @@ Checking Portapack-Havoc repository , we can see , that this excellent app was i
|
||||
|
||||
Later on , thanks to many other great sw developers , gradually it has been added many more nice functionalities (VOX control, Roger Beep,...) ,and improving it day by day ...
|
||||
And from Sept -2020, it was also added the support of multi analogue mic Modulation types in half duplex TX / RX, highly appreciated specially by all ham amateur radio community. Those mod types are widely used in LF, HF , VHF, 2m band , maritime communications ,airport airband communications, UHF PMR446,...
|
||||
And since them we are currently supporting those following ones (valid from , [Nightly Release - 2022-10-17](https://github.com/eried/portapack-mayhem/releases/tag/nightly-tag-2022-10-17) onwards) :
|
||||
And since them we are currently supporting those following ones (valid from , [Nightly Release - 2022-10-17](https://github.com/portapack-mayhem/mayhem-firmware/releases/tag/nightly-tag-2022-10-17) onwards) :
|
||||
|
||||
* Narrow and normal band FM (NBFM/FM),
|
||||
* Wide band FM (WBFM),
|
||||
@ -22,7 +22,7 @@ Note, this feature is not working once we activate below "Rx audio listen" ; but
|
||||
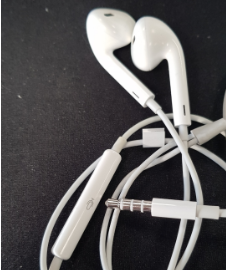
|
||||
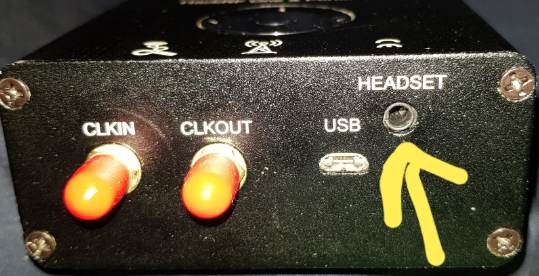
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
* **MIC. GAIN:** Cursor selection and use rotary encoder is used to select a fixed gain of x0.5, x1.0, x1.5, x2.0. The setting needs to be selected based, on the Microphone used which is connected via the Headset/ Microphone socket (standard smartphone 4 segment 3.5mm connector).
|
||||
@ -82,7 +82,7 @@ We have added five user "**Boost**" options , activating on/off , the mic-boost
|
||||
|
||||
From latest [Nightly Release - 2023-10-21] onwards it has been added a new check box (next to Roger beep) to the user to be able to select Separated (default) ) / Common freq. RX tuning control respect TX freq., and also another one "Hear Mic" (see attached new updated GUI pictures):
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
* **F:** This field set the TX Frequency for the transmitter. Use encoder dial to adjust by Step value (uses a default step value, or step value saved in SETTINGS\tx_mic.ini file if "Load App Settings" is enabled). A long press of the Select button allows adjusting frequency digits individually.
|
||||
|
2
Morse.md
2
Morse.md
@ -17,5 +17,5 @@ The Key Items on the App that can be selected with the cursor and changed with t
|
||||
* **Start:** This button starts the transmission and if pressed again can stop the transmission.
|
||||
|
||||
# Important note
|
||||
Due to the way it is designed, the first 2 characters are somewhat not sent. If you encounter the problem, try prefixing your message with two characters, like 'E' and space. Example: "E MyMessageStartsHere" (see issue [!303](https://github.com/eried/portapack-mayhem/issues/303) if you think you can help)
|
||||
Due to the way it is designed, the first 2 characters are somewhat not sent. If you encounter the problem, try prefixing your message with two characters, like 'E' and space. Example: "E MyMessageStartsHere" (see issue [!303](https://github.com/portapack-mayhem/mayhem-firmware/issues/303) if you think you can help)
|
||||
|
||||
|
@ -2,7 +2,7 @@ Views text files and supports very basic editing.
|
||||
|
||||
## The interface
|
||||
|
||||
<img width="240" alt="Notepad" src="https://github.com/eried/portapack-mayhem/assets/21130052/f7591165-9965-441f-b8cf-1e4f2dc04566">
|
||||
<img width="240" alt="Notepad" src="https://github.com/portapack-mayhem/mayhem-firmware/assets/21130052/f7591165-9965-441f-b8cf-1e4f2dc04566">
|
||||
|
||||
The menu button on the bottom right opens and closes the main menu. On start, this button will immediately show the file picker.
|
||||
|
||||
|
@ -5,9 +5,9 @@ More technical details can be found by following the links in the References sec
|
||||
|
||||
# UI Overview
|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||
### Settings
|
||||
- **Frequency**: Sets the frequency to receive pager messages on. Can be adjusted with the [encoder thumb wheel](Hardware-overview), on-screen numpad, or loaded from frequencies saved on an SD card. 439.9875 MHz is the most popular worldwide frequency used by Amateur radio for POCSAG. Amateur radio POCSAG uses 1200bps.
|
||||
@ -32,7 +32,7 @@ More technical details can be found by following the links in the References sec
|
||||
|
||||
## Config
|
||||
|
||||

|
||||

|
||||
|
||||
- **Enable Log**: Logs messages to the SD Card at "LOGS/POCSAG.TXT"
|
||||
- **Log Raw Data**: Logs the batch codewords as hexadecimal. Useful for debugging decoder bugs.
|
||||
|
@ -2,7 +2,7 @@ In order to get your PR merged into the code base, there are a few checks that n
|
||||
|
||||
* At least one code approver needs to approve your code.
|
||||
* If any code reviewer left PR comments, then all those PR comments left by reviewers need to be marked as resolved.
|
||||
* All 3 code format gate checks need to complete successfully. (don't know how to format your code? Check out how to do it here https://github.com/eried/portapack-mayhem/wiki/Code-formatting)
|
||||
* All 3 code format gate checks need to complete successfully. (don't know how to format your code? Check out how to do it here https://github.com/portapack-mayhem/mayhem-firmware/wiki/Code-formatting)
|
||||
|
||||
Once these steps are all complete, if you are an approved contributor you will notice you have a green merge button available, then means you are good to go and merge your code into the code base. Everyone else will see that tests have passed and will need to ask an approved contributor to merge it in for them (You can as the dev that approved the changes).
|
||||
|
||||
|
@ -2,7 +2,7 @@ This is part of the **RTC (Real Time Clock) subsystem**, inside the LPC43xx chip
|
||||
|
||||
# RTC Subsystem
|
||||
|
||||
The RTC subsystem keeps the actual clock (date / time) running, powered by a back up coin battery (which needs to be [installed](https://github.com/eried/portapack-mayhem/wiki/Hardware-overview#portapack-internals) on the portapack board),even when the unit is not connected to an USB power source.
|
||||
The RTC subsystem keeps the actual clock (date / time) running, powered by a back up coin battery (which needs to be [installed](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Hardware-overview#portapack-internals) on the portapack board),even when the unit is not connected to an USB power source.
|
||||
And on top of this RTC functionality, the same coin battery will keep persistent a small region of VBAT-maintained SRAM (0x40041000, 256 bytes) for saving system state settings when the device is powered off.
|
||||
|
||||
The persistent memory map is defined at `/firmware/chibios-portapack/os/hal/platforms/LPC43xx/lpc43xx.inc`:
|
||||
|
@ -1 +1 @@
|
||||
This app was combined with the [Replay app](https://github.com/eried/portapack-mayhem/wiki/Replay) after 1.7.2.
|
||||
This app was combined with the [Replay app](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Replay) after 1.7.2.
|
@ -70,8 +70,8 @@ A: Check the hints on the top of this page.
|
||||
* Has new CPLD code so the reboot button doesn't freeze the device unlike some of the other H2's on the market.
|
||||
|
||||
## H2 (old OpenSourceSDRLab version)
|
||||

|
||||

|
||||

|
||||

|
||||
|
||||
|
||||
### Differences:
|
||||
@ -109,13 +109,13 @@ A: Check the hints on the top of this page.
|
||||
|
||||
<img src="https://user-images.githubusercontent.com/4393979/170891015-6c9517bf-ee0b-49b9-b7bf-8d9f4049c721.png" height="300">
|
||||
|
||||

|
||||

|
||||
|
||||
### Differences
|
||||
* Similar to the H2+R3 except it now uses the AG256SL100 IC as well as the 28 pin QFN WM8731L instead of the 38 pin QFN AK4951. Marked as "PCB v3.6 mmdvm.club".
|
||||
* 3W LTK8002D SOP8 Class D amplifier for the speaker (INS8002e clone).
|
||||
* Power IC IP5306 SOP8.
|
||||
* Inserting headphone plug doesn't [disable the speaker](https://github.com/eried/portapack-mayhem/wiki/H2-Plus-speaker-modifications).
|
||||
* Inserting headphone plug doesn't [disable the speaker](https://github.com/portapack-mayhem/mayhem-firmware/wiki/H2-Plus-speaker-modifications).
|
||||
|
||||
## H2+ R5
|
||||
Bascially H2 with internal microphone and a independent power switch (but only pad/hole exist in some of the boards.)
|
||||
|
@ -15,7 +15,7 @@ If you identified the problem correctly, you can try to solve it by discarding t
|
||||
|
||||
With this battery charging module, even using a powerbank, the interference due to the old noisy charging IC is gone.
|
||||
|
||||
See the following pictures for more informations, and take a look at the [related issue](https://github.com/eried/portapack-mayhem/issues/898)
|
||||
See the following pictures for more informations, and take a look at the [related issue](https://github.com/portapack-mayhem/mayhem-firmware/issues/898)
|
||||
|
||||
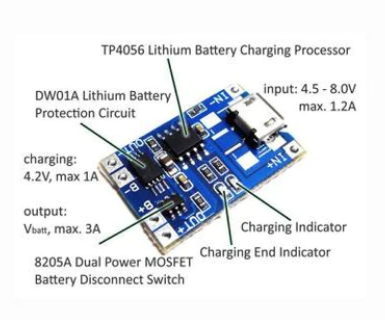
|
||||
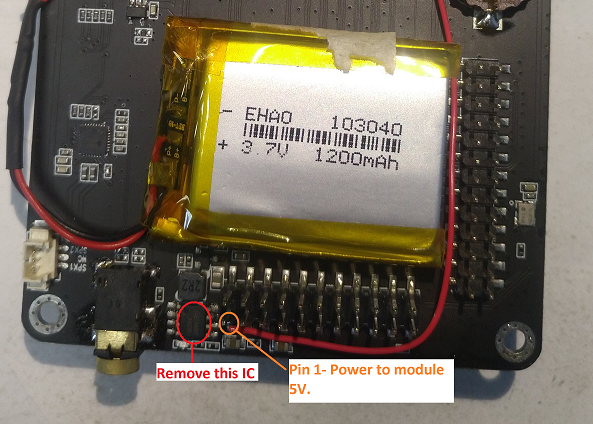
|
||||
|
@ -2,7 +2,7 @@ The MGA-81563 can be damaged easily, but you can buy (relatively cheap: https://
|
||||
|
||||
The previous link has a very good reference picture:
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
# RX problems
|
||||
@ -17,9 +17,9 @@ It is possible to replace the damaged IC with simple tools (soldering iron, sold
|
||||
|
||||
The same component is used for TX, which also can be damaged. Below is an example of the replacement procedure. First remove and clean the pads. This can be done with hot air or normal soldering iron:
|
||||
|
||||

|
||||

|
||||
|
||||
For the orientation, you could use the text of the chip itself. It should be "up", pointing to the antenna jack of the HackRF:
|
||||
|
||||

|
||||

|
||||
|
||||
|
@ -16,7 +16,7 @@ The Receivers Apps have a variable sensitivity to radio signals, and it should b
|
||||
These specifications can be used to roughly determine the suitability of HackRF for a given application. Testing is required to finely measure performance in an application. Performance can typically be enhanced significantly by selecting an appropriate antenna, external amplifier, and/or external filter for the application.
|
||||
|
||||
## What is the Receive Power of HackRF?
|
||||
The maximum RX power of HackRF One is -5 dBm. Exceeding -5 dBm can result in permanent damage! This is often seen, see notes on [Preamplifier IC replacement](https://github.com/eried/portapack-mayhem/wiki/Preamplifier-IC-replacement).
|
||||
The maximum RX power of HackRF One is -5 dBm. Exceeding -5 dBm can result in permanent damage! This is often seen, see notes on [Preamplifier IC replacement](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Preamplifier-IC-replacement).
|
||||
|
||||
In theory, HackRF One can safely accept up to 10 dBm with the front-end RX amplifier disabled. **However, a simple software or user error could enable the amplifier, resulting in permanent damage.** It is better to use an external attenuator than to risk damage.
|
||||
|
||||
|
12
Recon.md
12
Recon.md
@ -1,6 +1,6 @@
|
||||
# Recon App
|
||||
|
||||

|
||||

|
||||
- [Recon App](#recon-app)
|
||||
* [Important note](#important-note)
|
||||
* [Introduction](#introduction)
|
||||
@ -94,9 +94,9 @@ More:
|
||||
* Check that 'input: load freqs' and 'input: load ranges' are checked
|
||||
|
||||
# Main Screens
|
||||

|
||||

|
||||

|
||||

|
||||

|
||||

|
||||
|
||||
Buttons and information description, from top to bottom, and left to right. [NAME] is used to mark a button / gui element that the user can change, else it's a description of an onscreen information.
|
||||
|
||||
@ -130,7 +130,7 @@ Buttons and information description, from top to bottom, and left to right. [NAM
|
||||
|
||||
# CONFIG
|
||||
## Main RECON settings page
|
||||

|
||||

|
||||
|
||||
* input file => File from which we will load frequencies or ranges to search (default FREQMAN/RECON.TXT)
|
||||
* output file => File into which we will save frequency using STORE button or autosave mode (default FREQMAN/RECON_RESULTS.TXT)
|
||||
@ -141,7 +141,7 @@ Buttons and information description, from top to bottom, and left to right. [NAM
|
||||
* clear output at start => If checked then the output file is blanked at app start. If you're using that feature and want to keep one of your search results, do not forgot to go into filemanager to rename the file before starting the Recon app one more time
|
||||
|
||||
## More CONFIG settings page
|
||||

|
||||

|
||||
|
||||
One of the first two options have to be checked else nothing will be loaded at all and only manual range search will be available
|
||||
* load freq => allow load of frequencies
|
||||
|
@ -1,7 +1,7 @@
|
||||
The remote app allows you to create a custom remote UI and bind buttons to captures.
|
||||
|
||||

|
||||

|
||||

|
||||

|
||||
|
||||
## Main UI
|
||||
|
||||
|
@ -2,7 +2,7 @@ This app allows captured signals to be replayed.
|
||||
|
||||
# The UI
|
||||
|
||||

|
||||

|
||||
|
||||
Top controls
|
||||
* The top line is the name of the currently selected capture file to play (*.C8 or *.C16) .
|
||||
|
@ -4,7 +4,7 @@ Reading and writing to the SD card is a great way for your application to save p
|
||||
|
||||
## File Class
|
||||
|
||||
Most of the heavy lifting for working with files on the SD Card is done by the [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp) class. This helper class which simplifies the `FatFs - Generic FAT file system module` which can found at [`firmware/chibios-portapack/ext/fatfs/src/ff.c`](https://github.com/eried/portapack-mayhem/blob/next/firmware/chibios-portapack/ext/fatfs/src/ff.c). This wiki will go over some examples on how to read and write files to the SD card.
|
||||
Most of the heavy lifting for working with files on the SD Card is done by the [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp) class. This helper class which simplifies the `FatFs - Generic FAT file system module` which can found at [`firmware/chibios-portapack/ext/fatfs/src/ff.c`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/chibios-portapack/ext/fatfs/src/ff.c). This wiki will go over some examples on how to read and write files to the SD card.
|
||||
|
||||
Continuing with the [Create a Simple App](Create-a-simple-app) the code bellow will outline what is required to manipulate the file system. The first thing you'll need to do is include the File class and SD Card helper functions to your application's hpp file.
|
||||
|
||||
@ -17,7 +17,7 @@ Continuing with the [Create a Simple App](Create-a-simple-app) the code bellow w
|
||||
|
||||
### Check SD Card
|
||||
|
||||
Before reading and writing from the SD Card it's ideal to do a quick check to see if the SD card is mounted. There is error handing working in the background that keeps things from crashing however this will give people a better idea if there's a problem or not. The function below dose a quick check to see if the SD Card is showing a status of "Mounted". This will return true if sd_card::status() returns "Mounted" or false if sd_card::status() returns any other status. SD Card statuses are defined in [firmware/application/sd_card.hpp](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/sd_card.hpp).
|
||||
Before reading and writing from the SD Card it's ideal to do a quick check to see if the SD card is mounted. There is error handing working in the background that keeps things from crashing however this will give people a better idea if there's a problem or not. The function below dose a quick check to see if the SD Card is showing a status of "Mounted". This will return true if sd_card::status() returns "Mounted" or false if sd_card::status() returns any other status. SD Card statuses are defined in [firmware/application/sd_card.hpp](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/sd_card.hpp).
|
||||
|
||||
|
||||
#### ui_newapp.cpp
|
||||
@ -41,7 +41,7 @@ if(check_sd_card()) { // Check to see if S
|
||||
|
||||
### List Directory Contents
|
||||
|
||||
To make things easier to use we're going to use a method from [firmware/application/apps/ui_fileman.cpp](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/apps/ui_fileman.cpp) to list the contents of the SD Card. First thing we'll need to do is create a Struct that outlines the pertinent information of files and directories. Each struct will include the file path, size, and if the item is a directory or not.
|
||||
To make things easier to use we're going to use a method from [firmware/application/apps/ui_fileman.cpp](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/apps/ui_fileman.cpp) to list the contents of the SD Card. First thing we'll need to do is create a Struct that outlines the pertinent information of files and directories. Each struct will include the file path, size, and if the item is a directory or not.
|
||||
|
||||
#### ui_newapp.hpp
|
||||
```
|
||||
@ -94,7 +94,7 @@ std::vector<file_entry> NewAppView::list_dir(const std::filesystem::path& path)
|
||||
|
||||
### Create Directory
|
||||
|
||||
To create a directory we can use the `make_new_directory()` function from [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp). The helper function below will return true if `make_new_directory()` succeeds (Success from `make_new_directory()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`.
|
||||
To create a directory we can use the `make_new_directory()` function from [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp). The helper function below will return true if `make_new_directory()` succeeds (Success from `make_new_directory()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`.
|
||||
|
||||
#### ui_newapp.cpp
|
||||
```
|
||||
@ -122,7 +122,7 @@ if(check_sd_card()) { // Check to see if SD Card is mounted
|
||||
|
||||
### Create File
|
||||
|
||||
To create a file we can use the `create()` function from [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp) class. The helper function below will return true if `create()` succeeds (Success from `create()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`.
|
||||
To create a file we can use the `create()` function from [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp) class. The helper function below will return true if `create()` succeeds (Success from `create()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`.
|
||||
|
||||
#### ui_newapp.cpp
|
||||
```
|
||||
@ -155,12 +155,12 @@ In both mode a hardware limit is preventing any buffered read higher than blocks
|
||||
|
||||
**!! While you can and will do buffered read/write, the size of the buffer must not be higher than 512 !!**
|
||||
|
||||
See the [related issue discussion](https://github.com/eried/portapack-mayhem/issues/1103)
|
||||
See the [related issue discussion](https://github.com/portapack-mayhem/mayhem-firmware/issues/1103)
|
||||
|
||||
|
||||
### Read File
|
||||
|
||||
To read to a file the `read_file()` function from the [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp) class can be used. The helper function below will return true if the class object `File` opens the file successfully. Success from this open function will have a `is_valid()` function which will return a 0, other values means failure. Inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
To read to a file the `read_file()` function from the [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp) class can be used. The helper function below will return true if the class object `File` opens the file successfully. Success from this open function will have a `is_valid()` function which will return a 0, other values means failure. Inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
|
||||
**Note: The below sample code dose not handle large files, memory management needs to be implemented.**
|
||||
|
||||
@ -202,7 +202,7 @@ if(check_sd_card()) { // Check to see if
|
||||
|
||||
### Write File
|
||||
|
||||
To write to a file the `write_line()` or `write()` function from the [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp) class can be used. The helper function below will return true if the class object `File` appends to the target file successfully. Success from this append function will have a `is_valid()` function which will return a 0, other values means failure. Inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
To write to a file the `write_line()` or `write()` function from the [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp) class can be used. The helper function below will return true if the class object `File` appends to the target file successfully. Success from this append function will have a `is_valid()` function which will return a 0, other values means failure. Inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
|
||||
#### ui_newapp.cpp
|
||||
```
|
||||
@ -234,7 +234,7 @@ if(check_sd_card()) { // Check to see if
|
||||
|
||||
### Rename File or Directory
|
||||
|
||||
To rename a file or directory the `rename_file()` function from [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp) can be used. The helper function below will return true if `rename_file()` succeeds (Success from `rename_file()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
To rename a file or directory the `rename_file()` function from [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp) can be used. The helper function below will return true if `rename_file()` succeeds (Success from `rename_file()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
|
||||
#### ui_newapp.cpp
|
||||
```
|
||||
@ -260,7 +260,7 @@ if(check_sd_card()) { // Check to see if S
|
||||
|
||||
### Delete File or Directory
|
||||
|
||||
To delete a file or directory the `delete_file()` function from [File](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/file.cpp) can be used. The helper function below will return true if `delete_file()` succeeds (Success from `make_new_directory()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
To delete a file or directory the `delete_file()` function from [File](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/file.cpp) can be used. The helper function below will return true if `delete_file()` succeeds (Success from `make_new_directory()` returns a 0, other values means failure) and takes two variable inputs for file path and directory name. Again the input for the file path is a `std::filesystem::path` which can be `UTF-16 string literal`. The root of the SD Card is `u""` and any directory beyond that is `u"DIRECTORY/SUB_DIRECTORY"`
|
||||
|
||||
#### ui_newapp.cpp
|
||||
```
|
||||
|
@ -1,4 +1,4 @@
|
||||
The SD Card provides a memory resource that can be tailored to the specific user. Technical details of the card are given [here.](https://github.com/eried/portapack-mayhem/wiki/SD-Card-(DEV)) The SD card Standard image is supplied as part of the standard firmware download. Instructions for its use are supplied elsewhere. The SD card holds information in relation to Specific Apps or as general list of items such as frequencies follows:
|
||||
The SD Card provides a memory resource that can be tailored to the specific user. Technical details of the card are given [here.](https://github.com/portapack-mayhem/mayhem-firmware/wiki/SD-Card-(DEV)) The SD card Standard image is supplied as part of the standard firmware download. Instructions for its use are supplied elsewhere. The SD card holds information in relation to Specific Apps or as general list of items such as frequencies follows:
|
||||
|
||||
* **ADSB:** The ADBS Folder has databases of Airlines, IACO and is where the world map is located. See separate page on how to generate the map.
|
||||
* **AIS:** Holds the AIS database.
|
||||
|
@ -73,5 +73,5 @@ Follow the instructions below to use Scanner as a Broadcast FM Radio Tuner:
|
||||
6. In future listening sessions, just load the same file (step 1) and Scanner will loop through the saved frequencies, spending "Wsa" delay time on each saved station. To stay on a saved station indefinitely, press `<PAUSE>`. To manually change stations, move focus to the `<PAUSE>`/`<RESUME>` button and use the tuning dial. Additional stations may be added in the future by pressing `SRCH`, then `ADD FQ` as desired (step 5). Consider renaming the frequency file to SCANNER.TXT if you wish it to be loaded automatically whenever the app is started.
|
||||
|
||||
## Also see: Recon app
|
||||
Advanced users may want to try the [Recon](https://github.com/eried/portapack-mayhem/wiki/Recon) app, which is a more fully-featured Scanner application.
|
||||
Advanced users may want to try the [Recon](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Recon) app, which is a more fully-featured Scanner application.
|
||||
|
||||
|
@ -10,12 +10,12 @@ In the radio section there are three options,
|
||||
|
||||
> Note 2 : In all previous r1 to r8 Hackrf platforms , as we are using Si5351C, we do not have that limitation , and user can change the CLKOUT frequency between 4 kHz to 60000 kHz; press OK when the frequency is highlighted to select which digit position to modify and then use the encoder to scroll through the digit values. (it works with both clock references, the internal Hackrf (25Mhz) and the external -when available- from Portapack (TCXO 10Mhz ). Once enabled the CLK_out , the new introduced frequency will be updated to the CLK_out port, as soon as you press below SAVE button.
|
||||
|
||||
> 
|
||||
> 
|
||||
|
||||
> Note 3 : Zooming previous picture , when the unit detects that external CLOCK_in -usually 10Mhz reference- (from Portapack or from external source) , it is indicated in the top title CLK_in icon
|
||||
> with some top arrow below the icon (right picture) . In case of no detection , it is indicated with some "X" below the icon (left picture),and in that case, the system takes the internal 25Mhz Hackrf clock reference.
|
||||
|
||||
> 
|
||||
> 
|
||||
|
||||
|
||||
> Warning note : be awared that some of current market Portapack boards may have an integrated low ppm TCXO 10Mhz clock generator mounted, and when it is built in, it is connected in parallel to the external Hackrf CLK_in port connector. So in that special case , that internal PP clock signal is present always in the SMA CLK_in connector (this s a strange case, because in those devices, CLK_in is behaving as real embedded ref. output of internal TCXO 10Mhz clock) , and you should better not connect any other external signal generator there (unless you dissassembly the Portapack from Hackrf) , because otherwise, you will connect two clock signal generators in parallel -the embedded one to the external one -, and you may damage that Portapack TCXO clock IC circuit.
|
||||
@ -23,7 +23,7 @@ In the radio section there are three options,
|
||||
> Here below , you can see two different examples of the embedded TCXO 10Mhz ref. clock,
|
||||
> in a PP H1 brd (left side ) , PP H2 brd (right side) boards :
|
||||
|
||||
> 
|
||||
> 
|
||||
|
||||
|
||||
|
||||
@ -96,7 +96,7 @@ Set persistent memory from/to sd card options. It's particularly useful to keep
|
||||
* !reset p.mem, load defaults! : reset the persistent memory to defaults
|
||||
|
||||
## FreqCorrect
|
||||

|
||||

|
||||
|
||||
Set TX and or RX Frequency correction in Hz.
|
||||
|
||||
|
@ -17,13 +17,13 @@ The Spectrum Painter app allows to "paint" an image or text in a way that it app
|
||||
* Use a power of 2 for the width for optimal result. (eg. 512 / 1024 / 2048 / ...)
|
||||
|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||
### Text
|
||||
* Messages up to 300 characters are supported
|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
18
Title-Bar.md
18
Title-Bar.md
@ -12,12 +12,12 @@ The sections of the title bar, along with their functionality, are (from left to
|
||||
|-----|--------|----------|
|
||||
|Back |Back arrow to previous screen/Return to menu| Yes |
|
||||
|Name|Application name, when click -> same with Back| Yes |
|
||||
||Take [screenshot](screenshots) | Yes |
|
||||
||Activate [sleep mode](sleep-mode) | Yes |
|
||||
||Toggle [stealth mode](stealth-mode). Green if selected | Yes |
|
||||
| / |Toggle [Up conversion or Down conversion](https://github.com/eried/portapack-mayhem/wiki/Settings#converter). Green if activated. | Yes |
|
||||
| /  |Toggle DC power on antenna port. Yellow if selected | Yes |
|
||||
||Indicate status of external clock. Green if selected | Yes |
|
||||
| / |Enable/mute audio (speaker & headphone)|Yes|
|
||||
| /  |Enable speaker output on PortaPack with AK4951 codec. Green if activated. Warning: Do not enable if speaker already works when disabled, as on OpenSourceSDRLab PortaPack H2.| Yes |
|
||||
||Indicate presence of SD card. Green if SD card was found | No |
|
||||
||Take [screenshot](screenshots) | Yes |
|
||||
||Activate [sleep mode](sleep-mode) | Yes |
|
||||
||Toggle [stealth mode](stealth-mode). Green if selected | Yes |
|
||||
| / |Toggle [Up conversion or Down conversion](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Settings#converter). Green if activated. | Yes |
|
||||
| /  |Toggle DC power on antenna port. Yellow if selected | Yes |
|
||||
||Indicate status of external clock. Green if selected | Yes |
|
||||
| / |Enable/mute audio (speaker & headphone)|Yes|
|
||||
| /  |Enable speaker output on PortaPack with AK4951 codec. Green if activated. Warning: Do not enable if speaker already works when disabled, as on OpenSourceSDRLab PortaPack H2.| Yes |
|
||||
||Indicate presence of SD card. Green if SD card was found | No |
|
||||
|
@ -10,12 +10,12 @@ Any serial terminal client can be used to connect like PuTTY, minicom, screen, [
|
||||
|
||||
The terminal exposes the ChibiOS/RT Shell:
|
||||
|
||||

|
||||

|
||||
|
||||
# Available Commands
|
||||
* `help`: lists all available commands.
|
||||
|
||||

|
||||

|
||||
* `info`: shows the ChibiOS/RT system details.
|
||||
* `systime`: shows the uptime in ms.
|
||||
* `reboot`: reboots the PortaPack. This will also work on devices where the reset button is not working.
|
||||
@ -25,7 +25,7 @@ The terminal exposes the ChibiOS/RT Shell:
|
||||
* `flash`: This is the [Flash Utility](Flash-Utility).
|
||||
* `screenshot`: Takes a screenshot.
|
||||
|
||||

|
||||

|
||||
* `cmd_screenframe`: Replies with the screen's content. Format is 1 line / screen line, and for each pixel HEX (2 char) in the R,G,B order. So one line will be 720 + newline.
|
||||
* `cmd_screenframeshort`: Replies with the screen's content. Format is 1 line / screen line, and for each pixel you'll get 1 character. Format is '00RRGGBB' + 32. So for black you'll get 32 (' '). For white 95 ('_').
|
||||
* `gotgps`: You can send apps your current position. Format `gotgps lat lon <alt> <speed>`. Lat, lon is mandantory with '.' as a decimal separator.
|
||||
@ -34,7 +34,7 @@ The terminal exposes the ChibiOS/RT Shell:
|
||||
* `appstart`: You can start apps from the list given by the `applist` command. 1 paremeter needed, the app's short name. It'll stop any running apps.
|
||||
* `write_memory`: Writes arbitrary memory locations.
|
||||
|
||||

|
||||

|
||||
* `read_memory`: Reads arbitrary memory locations.
|
||||
* `button`: Simulates a button press
|
||||
* button 1: Right
|
||||
@ -62,7 +62,7 @@ The terminal exposes the ChibiOS/RT Shell:
|
||||
> there is a faster binary read option: `frb`
|
||||
* `fwrite`: Writes bytes from the currently opened file.
|
||||
|
||||

|
||||

|
||||
> [!TIP]
|
||||
> there is a faster binary write option: `fwb`
|
||||
* `pmemreset`: Sets all values in PMEM to default. Pass the "yes" as a parameter, that indicates you know what are you doing.
|
||||
|
@ -2,7 +2,7 @@ One of the main sources of problems while [updating the firmware](Update-firmwar
|
||||
|
||||
## Black or white screen?
|
||||
|
||||
If you get a black or white screen after updating, please check the wiki here: [Won't boot](https://github.com/eried/portapack-mayhem/wiki/Won't-boot)
|
||||
If you get a black or white screen after updating, please check the wiki here: [Won't boot](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Won't-boot)
|
||||
|
||||
## An application is not starting anymore?
|
||||
|
||||
|
@ -3,11 +3,11 @@ In theory, it is impossible to brick the device, since you can always try the DF
|
||||
# Normal procedure
|
||||
|
||||
## Firmware
|
||||
Get the latest firmware from the [](https://github.com/eried/portapack-mayhem/releases/latest) page. Please check the [FAQ](https://github.com/eried/portapack-mayhem#frequently-asked-questions) if you have any additional question.
|
||||
Get the latest firmware from the [](https://github.com/portapack-mayhem/mayhem-firmware/releases/latest) page. Please check the [FAQ](https://github.com/portapack-mayhem/mayhem-firmware#frequently-asked-questions) if you have any additional question.
|
||||
|
||||
### HackRF/PortaPack itself via Flash Utility
|
||||
|
||||
The [Flash Utility](https://github.com/eried/portapack-mayhem/wiki/Flash-Utility) can also be used to program new firmware from a bin file stored on a MicroSD card, mentioned below. The Flash utility is the update method of choice for users that may not want to run an app on their PC, or may want to switch between firmware versions when in the field. If you download and unzip the latest mayhem_v#.##_COPY_TO_SDCARD.zip contents to your SD card, the latest firmware image will be found in the FIRMWARE folder (this method also updates the external apps in the APPS folder, mentioned below).
|
||||
The [Flash Utility](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Flash-Utility) can also be used to program new firmware from a bin file stored on a MicroSD card, mentioned below. The Flash utility is the update method of choice for users that may not want to run an app on their PC, or may want to switch between firmware versions when in the field. If you download and unzip the latest mayhem_v#.##_COPY_TO_SDCARD.zip contents to your SD card, the latest firmware image will be found in the FIRMWARE folder (this method also updates the external apps in the APPS folder, mentioned below).
|
||||
|
||||
### Windows
|
||||
|
||||
@ -40,7 +40,7 @@ _HackRF CLI tools for MacOS available through MacPorts or Homebrew_
|
||||
|
||||
Your PortaPack has a slot for a memory card. You need to provide a MicroSD with enough space (16GB is recommended, over 32GB will be omitted due the limits of the FAT32). This is necessary for certain functionality, like the world map, GPS simulator, external apps, and others.
|
||||
|
||||
Get the latest files from the [](https://github.com/eried/portapack-mayhem/releases/latest) page. You need to uncompress (using [7-zip](https://www.7-zip.org/download.html)) the files from `mayhem_vX.Y.Z_COPY_TO_SDCARD.7z` to a FAT32 formatted MicroSD card.
|
||||
Get the latest files from the [](https://github.com/portapack-mayhem/mayhem-firmware/releases/latest) page. You need to uncompress (using [7-zip](https://www.7-zip.org/download.html)) the files from `mayhem_vX.Y.Z_COPY_TO_SDCARD.7z` to a FAT32 formatted MicroSD card.
|
||||
> [!NOTE]
|
||||
> How to put into sdcard?
|
||||
> Extract the root directory of the 7z archive into the root directory of your sdcard.
|
||||
|
@ -1,10 +1,10 @@
|
||||
> This guide is a temporal entry.
|
||||
|
||||
1. Navigate into **github.com** (Create account if not have already) get your own fork of **eried/portapack-mayhem**
|
||||
1. Navigate into **github.com** (Create account if not have already) get your own fork of **portapack-mayhem/mayhem-firmware**
|
||||
|
||||
1. Download and install Github Desktop **https://desktop.github.com**
|
||||
|
||||
1. Next, start github desktop, get into your account and clone forked eried/portapack-mayhem into local folder (i.e. **/Users/enrique/Documents/GitHub/portapack-mayhem**)
|
||||
1. Next, start github desktop, get into your account and clone forked portapack-mayhem/mayhem-firmware into local folder (i.e. **/Users/enrique/Documents/GitHub/portapack-mayhem**)
|
||||
|
||||
1. Download and install Docker Desktop for Mac **https://hub.docker.com/editions/community/docker-ce-desktop-mac/**
|
||||
|
||||
|
@ -1,8 +1,8 @@
|
||||
* Freq manager
|
||||
* File manager
|
||||
* [Signal gen](https://github.com/eried/portapack-mayhem/wiki/Signal-Generator)
|
||||
* [Signal gen](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Signal-Generator)
|
||||
* Wave viewer
|
||||
* [Antenna Length](https://github.com/eried/portapack-mayhem/wiki/antennas)
|
||||
* [Antenna Length](https://github.com/portapack-mayhem/mayhem-firmware/wiki/antennas)
|
||||
* [Wipe SD card](Wipe-SD-Card)
|
||||
* [Flash Utility](Flash-Utility)
|
||||
* [SD over USB](SD-Over-USB)
|
12
Widgets.md
12
Widgets.md
@ -1,7 +1,7 @@
|
||||
## About
|
||||
|
||||
Widgets are the elements that compose the UI of your custom app. Widgets are defined inside [`firmware\common\ui_widget.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui_widget.hpp) and widget functions can be found inside [`firmware\common\ui_widget.cpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui_widget.cpp). In order to be able to use them, you must `#include "ui_widget.hpp"` into your app .hpp file.
|
||||
There are different type of widget. here you will find a list of available widget, with their respecting constructor. For all the methods available, you should go and see the [ui_widget.hpp](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui_widget.hpp) file.
|
||||
Widgets are the elements that compose the UI of your custom app. Widgets are defined inside [`firmware\common\ui_widget.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui_widget.hpp) and widget functions can be found inside [`firmware\common\ui_widget.cpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui_widget.cpp). In order to be able to use them, you must `#include "ui_widget.hpp"` into your app .hpp file.
|
||||
There are different type of widget. here you will find a list of available widget, with their respecting constructor. For all the methods available, you should go and see the [ui_widget.hpp](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui_widget.hpp) file.
|
||||
|
||||
## Attach a Generic Widget to Your Application
|
||||
|
||||
@ -75,7 +75,7 @@ Labels my_label{
|
||||
};
|
||||
```
|
||||
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui.hpp).
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui.hpp).
|
||||
|
||||
In `apps/ui_newapp.cpp` you'll need to add the `my_label` pointer to add_child() or add_children():
|
||||
```
|
||||
@ -321,7 +321,7 @@ Image my_Image_widget{
|
||||
};
|
||||
```
|
||||
|
||||
Images need to be a Bitmap object before they can be displayed. Bellow is an example of the code needed to create a Bitmap from [`firmware/application/bitmap.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/application/bitmap.hpp).
|
||||
Images need to be a Bitmap object before they can be displayed. Bellow is an example of the code needed to create a Bitmap from [`firmware/application/bitmap.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/application/bitmap.hpp).
|
||||
```
|
||||
static constexpr uint8_t bitmap_stripes_data[] = {
|
||||
0xFF, 0x03, 0xC0,
|
||||
@ -347,7 +347,7 @@ Image my_image(
|
||||
Color::black() // Color Background
|
||||
);
|
||||
```
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui.hpp)
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui.hpp)
|
||||
|
||||
|
||||
In `apps/ui_newapp.cpp` you'll need to add the `my_image` pointer to add_child() or add_children():
|
||||
@ -478,7 +478,7 @@ Waveform my_waveform(
|
||||
Color::white() // Sine wave color
|
||||
);
|
||||
```
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/eried/portapack-mayhem/blob/next/firmware/common/ui.hpp).
|
||||
**Note:** Colors are defined in [`firmware/common/ui.hpp`](https://github.com/portapack-mayhem/mayhem-firmware/blob/next/firmware/common/ui.hpp).
|
||||
|
||||
|
||||
The data being displayed by `my_waveform` needs to be a `int16_t` array. You can declare this variable in `apps/ui_newapp.hpp`:
|
||||
|
@ -101,7 +101,7 @@ Then ,
|
||||
|
||||
|
||||
## Explanation
|
||||
Firmware starting at version 1.5.4 and onward contain the <a href="https://github.com/eried/portapack-mayhem/pull/662">Pull Request 662</a> that uses the persistent memory to test and store the hardware and LCD config settings . That memory uses the same back up voltage than the Real Time Clock calendar, both needs a healthy cell battery button voltage. Sometimes, in a re flashing process , although we got good battery cell button voltage, the unit seems to be badly initializing those persistent bytes and we got strange black screen. Doing those above steps probably reset the persistent memory. That's just a guess.
|
||||
Firmware starting at version 1.5.4 and onward contain the <a href="https://github.com/portapack-mayhem/mayhem-firmware/pull/662">Pull Request 662</a> that uses the persistent memory to test and store the hardware and LCD config settings . That memory uses the same back up voltage than the Real Time Clock calendar, both needs a healthy cell battery button voltage. Sometimes, in a re flashing process , although we got good battery cell button voltage, the unit seems to be badly initializing those persistent bytes and we got strange black screen. Doing those above steps probably reset the persistent memory. That's just a guess.
|
||||
|
||||
### Additional notes :
|
||||
I used to have many frequent “black LCD boot brick” when exchanging binaries compiled with different gcc-arm… version , from 9.4 to 10.3 or 12 . But thanks to @u–foka‘s PR fix pmem -> make backup_ram_t data members volatile [#1135](https://github.com/eried/portapack-mayhem/pull/1135), all those problems are gone , and now I do not have any persistent memory boot problems , so I do not need to go back to any old version 1.4.3 anymore.
|
||||
I used to have many frequent “black LCD boot brick” when exchanging binaries compiled with different gcc-arm… version , from 9.4 to 10.3 or 12 . But thanks to @u–foka‘s PR fix pmem -> make backup_ram_t data members volatile [#1135](https://github.com/portapack-mayhem/mayhem-firmware/pull/1135), all those problems are gone , and now I do not have any persistent memory boot problems , so I do not need to go back to any old version 1.4.3 anymore.
|
||||
|
@ -1 +1 @@
|
||||
### [Moved to here: https://github.com/eried/portapack-mayhem/wiki/Won't-boot](https://github.com/eried/portapack-mayhem/wiki/Won't-boot)
|
||||
### [Moved to here: https://github.com/portapack-mayhem/mayhem-firmware/wiki/Won't-boot](https://github.com/portapack-mayhem/mayhem-firmware/wiki/Won't-boot)
|
Loading…
x
Reference in New Issue
Block a user