mirror of
https://github.com/oxen-io/session-android.git
synced 2024-11-24 02:25:19 +00:00
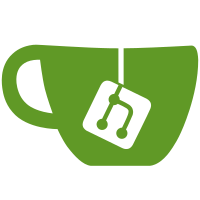
* Fix the build.gradle file * Use the correct VisibleForTesting class * Fix the Android test setUp() logic * Enable a test in DeliveryReceiptJobTest.java where the @Test anotation was missing It should be noted that the tests in AttachmentDatabaseTest.java fail. Fixes #3474 Closes #5882
71 lines
2.8 KiB
Java
71 lines
2.8 KiB
Java
package org.thoughtcrime.securesms.database;
|
|
|
|
import android.net.Uri;
|
|
|
|
import org.thoughtcrime.securesms.TextSecureTestCase;
|
|
import org.thoughtcrime.securesms.attachments.AttachmentId;
|
|
import org.thoughtcrime.securesms.attachments.DatabaseAttachment;
|
|
import org.thoughtcrime.securesms.crypto.MasterSecret;
|
|
|
|
import java.io.FileNotFoundException;
|
|
import java.io.InputStream;
|
|
|
|
import static org.mockito.Matchers.any;
|
|
import static org.mockito.Matchers.anyFloat;
|
|
import static org.mockito.Matchers.eq;
|
|
import static org.mockito.Mockito.doNothing;
|
|
import static org.mockito.Mockito.doReturn;
|
|
import static org.mockito.Mockito.mock;
|
|
import static org.mockito.Mockito.never;
|
|
import static org.mockito.Mockito.spy;
|
|
import static org.mockito.Mockito.verify;
|
|
import static org.mockito.Mockito.when;
|
|
|
|
public class AttachmentDatabaseTest extends TextSecureTestCase {
|
|
private static final long ROW_ID = 1L;
|
|
private static final long UNIQUE_ID = 2L;
|
|
|
|
private AttachmentDatabase database;
|
|
|
|
@Override
|
|
public void setUp() {
|
|
super.setUp();
|
|
database = spy(DatabaseFactory.getAttachmentDatabase(getInstrumentation().getTargetContext()));
|
|
}
|
|
|
|
public void testTaskNotRunWhenThumbnailExists() throws Exception {
|
|
final AttachmentId attachmentId = new AttachmentId(ROW_ID, UNIQUE_ID);
|
|
|
|
when(database.getAttachment(attachmentId)).thenReturn(getMockAttachment("x/x"));
|
|
|
|
doReturn(mock(InputStream.class)).when(database).getDataStream(any(MasterSecret.class), any(AttachmentId.class), eq("thumbnail"));
|
|
database.getThumbnailStream(mock(MasterSecret.class), attachmentId);
|
|
|
|
// XXX - I don't think this is testing anything? The thumbnail would be updated asynchronously.
|
|
verify(database, never()).updateAttachmentThumbnail(any(MasterSecret.class), any(AttachmentId.class), any(InputStream.class), anyFloat());
|
|
}
|
|
|
|
public void testTaskRunWhenThumbnailMissing() throws Exception {
|
|
final AttachmentId attachmentId = new AttachmentId(ROW_ID, UNIQUE_ID);
|
|
|
|
when(database.getAttachment(attachmentId)).thenReturn(getMockAttachment("image/png"));
|
|
doReturn(null).when(database).getDataStream(any(MasterSecret.class), any(AttachmentId.class), eq("thumbnail"));
|
|
doNothing().when(database).updateAttachmentThumbnail(any(MasterSecret.class), any(AttachmentId.class), any(InputStream.class), anyFloat());
|
|
|
|
try {
|
|
database.new ThumbnailFetchCallable(mock(MasterSecret.class), attachmentId).call();
|
|
throw new AssertionError("didn't try to generate thumbnail");
|
|
} catch (FileNotFoundException fnfe) {
|
|
// success
|
|
}
|
|
}
|
|
|
|
private DatabaseAttachment getMockAttachment(String contentType) {
|
|
DatabaseAttachment attachment = mock(DatabaseAttachment.class);
|
|
when(attachment.getContentType()).thenReturn(contentType);
|
|
when(attachment.getDataUri()).thenReturn(Uri.EMPTY);
|
|
|
|
return attachment;
|
|
}
|
|
}
|