mirror of
https://github.com/oxen-io/session-android.git
synced 2024-11-28 20:45:17 +00:00
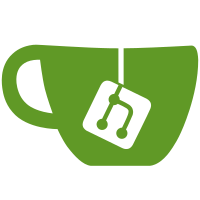
The current activity needs to be finished before calling startActivity. Otherwise, activities with launchMode singleTask (ConversationListActivity) will receive a new Intent instead of getting restarted. And in response to the new Intent, they will run onResume once again and trigger a second restart. Fixes #1292
58 lines
2.0 KiB
Java
58 lines
2.0 KiB
Java
package org.thoughtcrime.securesms.util;
|
|
|
|
import android.app.Activity;
|
|
import android.content.Intent;
|
|
import android.os.Build;
|
|
import android.preference.PreferenceManager;
|
|
|
|
import org.thoughtcrime.securesms.ApplicationPreferencesActivity;
|
|
import org.thoughtcrime.securesms.ConversationActivity;
|
|
import org.thoughtcrime.securesms.ConversationListActivity;
|
|
import org.thoughtcrime.securesms.R;
|
|
|
|
public class DynamicTheme {
|
|
|
|
private int currentTheme;
|
|
|
|
public void onCreate(Activity activity) {
|
|
currentTheme = getSelectedTheme(activity);
|
|
activity.setTheme(currentTheme);
|
|
}
|
|
|
|
public void onResume(Activity activity) {
|
|
if (currentTheme != getSelectedTheme(activity)) {
|
|
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) {
|
|
activity.recreate();
|
|
} else {
|
|
Intent intent = activity.getIntent();
|
|
activity.finish();
|
|
OverridePendingTransition.invoke(activity);
|
|
activity.startActivity(intent);
|
|
OverridePendingTransition.invoke(activity);
|
|
}
|
|
}
|
|
}
|
|
|
|
private static int getSelectedTheme(Activity activity) {
|
|
String theme = TextSecurePreferences.getTheme(activity);
|
|
|
|
if (theme.equals("light")) {
|
|
if (activity instanceof ConversationListActivity) return R.style.TextSecure_LightTheme_NavigationDrawer;
|
|
else if (activity instanceof ConversationActivity) return R.style.TextSecure_LightTheme_ConversationActivity;
|
|
else return R.style.TextSecure_LightTheme;
|
|
} else if (theme.equals("dark")) {
|
|
if (activity instanceof ConversationListActivity) return R.style.TextSecure_DarkTheme_NavigationDrawer;
|
|
else if (activity instanceof ConversationActivity) return R.style.TextSecure_DarkTheme_ConversationActivity;
|
|
else return R.style.TextSecure_DarkTheme;
|
|
}
|
|
|
|
return R.style.TextSecure_LightTheme;
|
|
}
|
|
|
|
private static final class OverridePendingTransition {
|
|
static void invoke(Activity activity) {
|
|
activity.overridePendingTransition(0, 0);
|
|
}
|
|
}
|
|
}
|