mirror of
https://github.com/oxen-io/session-android.git
synced 2025-01-12 17:33:39 +00:00
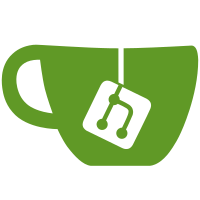
Strip all EXIF metadata from all JPEGs by re-encoding the JPEG. This will keep all of the necessary visual effects of the tags (by encoding them directly in the image data) while stripped the EXIF tags themselves.
84 lines
3.2 KiB
Java
84 lines
3.2 KiB
Java
package org.thoughtcrime.securesms.jobs;
|
|
|
|
import android.content.Context;
|
|
import android.support.annotation.NonNull;
|
|
|
|
import org.thoughtcrime.securesms.BuildConfig;
|
|
import org.thoughtcrime.securesms.TextSecureExpiredException;
|
|
import org.thoughtcrime.securesms.attachments.Attachment;
|
|
import org.thoughtcrime.securesms.crypto.MasterSecret;
|
|
import org.thoughtcrime.securesms.database.AttachmentDatabase;
|
|
import org.thoughtcrime.securesms.database.DatabaseFactory;
|
|
import org.thoughtcrime.securesms.mms.MediaConstraints;
|
|
import org.thoughtcrime.securesms.mms.MediaStream;
|
|
import org.thoughtcrime.securesms.mms.MmsException;
|
|
import org.thoughtcrime.securesms.transport.UndeliverableMessageException;
|
|
import org.thoughtcrime.securesms.util.MediaUtil;
|
|
import org.thoughtcrime.securesms.util.Util;
|
|
import org.whispersystems.jobqueue.JobParameters;
|
|
|
|
import java.io.IOException;
|
|
import java.util.LinkedList;
|
|
import java.util.List;
|
|
|
|
public abstract class SendJob extends MasterSecretJob {
|
|
|
|
@SuppressWarnings("unused")
|
|
private final static String TAG = SendJob.class.getSimpleName();
|
|
|
|
public SendJob(Context context, JobParameters parameters) {
|
|
super(context, parameters);
|
|
}
|
|
|
|
@Override
|
|
public final void onRun(MasterSecret masterSecret) throws Exception {
|
|
if (Util.getDaysTillBuildExpiry() <= 0) {
|
|
throw new TextSecureExpiredException(String.format("TextSecure expired (build %d, now %d)",
|
|
BuildConfig.BUILD_TIMESTAMP,
|
|
System.currentTimeMillis()));
|
|
}
|
|
|
|
onSend(masterSecret);
|
|
}
|
|
|
|
protected abstract void onSend(MasterSecret masterSecret) throws Exception;
|
|
|
|
protected void markAttachmentsUploaded(long messageId, @NonNull List<Attachment> attachments) {
|
|
AttachmentDatabase database = DatabaseFactory.getAttachmentDatabase(context);
|
|
|
|
for (Attachment attachment : attachments) {
|
|
database.markAttachmentUploaded(messageId, attachment);
|
|
}
|
|
}
|
|
|
|
protected List<Attachment> scaleAndStripExifFromAttachments(@NonNull MediaConstraints constraints,
|
|
@NonNull List<Attachment> attachments)
|
|
throws UndeliverableMessageException
|
|
{
|
|
AttachmentDatabase attachmentDatabase = DatabaseFactory.getAttachmentDatabase(context);
|
|
List<Attachment> results = new LinkedList<>();
|
|
|
|
for (Attachment attachment : attachments) {
|
|
try {
|
|
if (constraints.isSatisfied(context, attachment)) {
|
|
if (MediaUtil.isJpeg(attachment)) {
|
|
MediaStream stripped = constraints.getResizedMedia(context, attachment);
|
|
results.add(attachmentDatabase.updateAttachmentData(attachment, stripped));
|
|
} else {
|
|
results.add(attachment);
|
|
}
|
|
} else if (constraints.canResize(attachment)) {
|
|
MediaStream resized = constraints.getResizedMedia(context, attachment);
|
|
results.add(attachmentDatabase.updateAttachmentData(attachment, resized));
|
|
} else {
|
|
throw new UndeliverableMessageException("Size constraints could not be met!");
|
|
}
|
|
} catch (IOException | MmsException e) {
|
|
throw new UndeliverableMessageException(e);
|
|
}
|
|
}
|
|
|
|
return results;
|
|
}
|
|
}
|