mirror of
https://github.com/oxen-io/session-android.git
synced 2024-11-28 12:35:17 +00:00
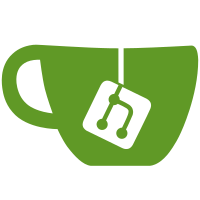
1) Remove all our PDU code and switch to the PDU code from the klinker library 2) Switch to using the system Lollipop MMS library by default, and falling back to our own custom library if that fails. 3) Format SMIL differently, using code from klinker instead of what we've pieced together. 4) Pull per-carrier MMS media constraints from the XML config files in the klinker library, instead of hardcoding it at 280kb. Hopefully this is an improvement, but given that MMS is involved, it will probably make things worse instead.
99 lines
2.9 KiB
Java
99 lines
2.9 KiB
Java
package org.thoughtcrime.securesms.jobs;
|
|
|
|
import android.content.Context;
|
|
import android.util.Log;
|
|
import android.util.Pair;
|
|
|
|
import com.google.android.mms.pdu_alt.GenericPdu;
|
|
import com.google.android.mms.pdu_alt.NotificationInd;
|
|
import com.google.android.mms.pdu_alt.PduHeaders;
|
|
import com.google.android.mms.pdu_alt.PduParser;
|
|
|
|
import org.thoughtcrime.securesms.ApplicationContext;
|
|
import org.thoughtcrime.securesms.database.DatabaseFactory;
|
|
import org.thoughtcrime.securesms.database.MmsDatabase;
|
|
import org.thoughtcrime.securesms.recipients.RecipientFactory;
|
|
import org.thoughtcrime.securesms.recipients.Recipients;
|
|
import org.thoughtcrime.securesms.util.Util;
|
|
import org.whispersystems.jobqueue.JobParameters;
|
|
|
|
public class MmsReceiveJob extends ContextJob {
|
|
|
|
private static final long serialVersionUID = 1L;
|
|
|
|
private static final String TAG = MmsReceiveJob.class.getSimpleName();
|
|
|
|
private final byte[] data;
|
|
private final int subscriptionId;
|
|
|
|
public MmsReceiveJob(Context context, byte[] data, int subscriptionId) {
|
|
super(context, JobParameters.newBuilder()
|
|
.withWakeLock(true)
|
|
.withPersistence().create());
|
|
|
|
this.data = data;
|
|
this.subscriptionId = subscriptionId;
|
|
}
|
|
|
|
@Override
|
|
public void onAdded() {
|
|
|
|
}
|
|
|
|
@Override
|
|
public void onRun() {
|
|
if (data == null) {
|
|
Log.w(TAG, "Received NULL pdu, ignoring...");
|
|
return;
|
|
}
|
|
|
|
PduParser parser = new PduParser(data);
|
|
GenericPdu pdu = null;
|
|
|
|
try {
|
|
pdu = parser.parse();
|
|
} catch (RuntimeException e) {
|
|
Log.w(TAG, e);
|
|
}
|
|
|
|
if (isNotification(pdu) && !isBlocked(pdu)) {
|
|
MmsDatabase database = DatabaseFactory.getMmsDatabase(context);
|
|
Pair<Long, Long> messageAndThreadId = database.insertMessageInbox((NotificationInd)pdu, subscriptionId);
|
|
|
|
Log.w(TAG, "Inserted received MMS notification...");
|
|
|
|
ApplicationContext.getInstance(context)
|
|
.getJobManager()
|
|
.add(new MmsDownloadJob(context,
|
|
messageAndThreadId.first,
|
|
messageAndThreadId.second,
|
|
true));
|
|
} else if (isNotification(pdu)) {
|
|
Log.w(TAG, "*** Received blocked MMS, ignoring...");
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public void onCanceled() {
|
|
// TODO
|
|
}
|
|
|
|
@Override
|
|
public boolean onShouldRetry(Exception exception) {
|
|
return false;
|
|
}
|
|
|
|
private boolean isBlocked(GenericPdu pdu) {
|
|
if (pdu.getFrom() != null && pdu.getFrom().getTextString() != null) {
|
|
Recipients recipients = RecipientFactory.getRecipientsFromString(context, Util.toIsoString(pdu.getFrom().getTextString()), false);
|
|
return recipients.isBlocked();
|
|
}
|
|
|
|
return false;
|
|
}
|
|
|
|
private boolean isNotification(GenericPdu pdu) {
|
|
return pdu != null && pdu.getMessageType() == PduHeaders.MESSAGE_TYPE_NOTIFICATION_IND;
|
|
}
|
|
}
|