mirror of
https://github.com/oxen-io/session-android.git
synced 2025-01-09 08:53:40 +00:00
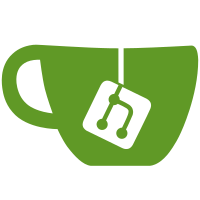
This was a holdover from Signal's origins as a pure SMS app. It causes problems, depends on undefined device specific behavior, and should no longer be necessary now that we have all the information we need to E164 all numbers. // FREEBIE
110 lines
3.6 KiB
Java
110 lines
3.6 KiB
Java
package org.thoughtcrime.securesms.util;
|
|
|
|
import android.content.Context;
|
|
import android.support.annotation.NonNull;
|
|
import android.support.annotation.Nullable;
|
|
import android.util.Log;
|
|
|
|
import org.thoughtcrime.securesms.R;
|
|
import org.thoughtcrime.securesms.database.Address;
|
|
import org.thoughtcrime.securesms.recipients.Recipient;
|
|
import org.thoughtcrime.securesms.recipients.RecipientFactory;
|
|
import org.thoughtcrime.securesms.recipients.Recipients;
|
|
|
|
import java.io.IOException;
|
|
import java.util.LinkedList;
|
|
import java.util.List;
|
|
|
|
import static org.whispersystems.signalservice.internal.push.SignalServiceProtos.GroupContext;
|
|
|
|
public class GroupUtil {
|
|
|
|
private static final String ENCODED_GROUP_PREFIX = "__textsecure_group__!";
|
|
private static final String TAG = GroupUtil.class.getSimpleName();
|
|
|
|
public static String getEncodedId(byte[] groupId) {
|
|
return ENCODED_GROUP_PREFIX + Hex.toStringCondensed(groupId);
|
|
}
|
|
|
|
public static byte[] getDecodedId(String groupId) throws IOException {
|
|
if (!isEncodedGroup(groupId)) {
|
|
throw new IOException("Invalid encoding");
|
|
}
|
|
|
|
return Hex.fromStringCondensed(groupId.split("!", 2)[1]);
|
|
}
|
|
|
|
public static boolean isEncodedGroup(@NonNull String groupId) {
|
|
return groupId.startsWith(ENCODED_GROUP_PREFIX);
|
|
}
|
|
|
|
public static @NonNull GroupDescription getDescription(@NonNull Context context, @Nullable String encodedGroup) {
|
|
if (encodedGroup == null) {
|
|
return new GroupDescription(context, null);
|
|
}
|
|
|
|
try {
|
|
GroupContext groupContext = GroupContext.parseFrom(Base64.decode(encodedGroup));
|
|
return new GroupDescription(context, groupContext);
|
|
} catch (IOException e) {
|
|
Log.w(TAG, e);
|
|
return new GroupDescription(context, null);
|
|
}
|
|
}
|
|
|
|
public static class GroupDescription {
|
|
|
|
@NonNull private final Context context;
|
|
@Nullable private final GroupContext groupContext;
|
|
@Nullable private final Recipients members;
|
|
|
|
public GroupDescription(@NonNull Context context, @Nullable GroupContext groupContext) {
|
|
this.context = context.getApplicationContext();
|
|
this.groupContext = groupContext;
|
|
|
|
if (groupContext == null || groupContext.getMembersList().isEmpty()) {
|
|
this.members = null;
|
|
} else {
|
|
List<Address> adddresses = new LinkedList<>();
|
|
|
|
for (String member : groupContext.getMembersList()) {
|
|
adddresses.add(Address.fromExternal(context, member));
|
|
}
|
|
|
|
this.members = RecipientFactory.getRecipientsFor(context, adddresses.toArray(new Address[0]), true);
|
|
}
|
|
}
|
|
|
|
public String toString(Recipient sender) {
|
|
StringBuilder description = new StringBuilder();
|
|
description.append(context.getString(R.string.MessageRecord_s_updated_group, sender.toShortString()));
|
|
|
|
if (groupContext == null) {
|
|
return description.toString();
|
|
}
|
|
|
|
String title = groupContext.getName();
|
|
|
|
if (members != null) {
|
|
description.append("\n");
|
|
description.append(context.getResources().getQuantityString(R.plurals.GroupUtil_joined_the_group,
|
|
members.getRecipientsList().size(), members.toShortString()));
|
|
}
|
|
|
|
if (title != null && !title.trim().isEmpty()) {
|
|
if (members != null) description.append(" ");
|
|
else description.append("\n");
|
|
description.append(context.getString(R.string.GroupUtil_group_name_is_now, title));
|
|
}
|
|
|
|
return description.toString();
|
|
}
|
|
|
|
public void addListener(Recipients.RecipientsModifiedListener listener) {
|
|
if (this.members != null) {
|
|
this.members.addListener(listener);
|
|
}
|
|
}
|
|
}
|
|
}
|