mirror of
https://github.com/oxen-io/session-android.git
synced 2025-02-17 18:48:27 +00:00
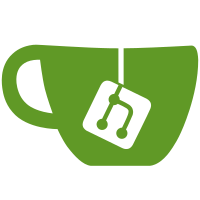
No sticker packs are available for use yet, but we now have the latent ability to send and receive.
6779 lines
243 KiB
Java
6779 lines
243 KiB
Java
// Generated by the protocol buffer compiler. DO NOT EDIT!
|
|
// source: Backups.proto
|
|
|
|
package org.thoughtcrime.securesms.backup;
|
|
|
|
public final class BackupProtos {
|
|
private BackupProtos() {}
|
|
public static void registerAllExtensions(
|
|
com.google.protobuf.ExtensionRegistry registry) {
|
|
}
|
|
public interface SqlStatementOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional string statement = 1;
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
boolean hasStatement();
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
java.lang.String getStatement();
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
com.google.protobuf.ByteString
|
|
getStatementBytes();
|
|
|
|
// repeated .signal.SqlStatement.SqlParameter parameters = 2;
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
java.util.List<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter>
|
|
getParametersList();
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter getParameters(int index);
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
int getParametersCount();
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
java.util.List<? extends org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder>
|
|
getParametersOrBuilderList();
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder getParametersOrBuilder(
|
|
int index);
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.SqlStatement}
|
|
*/
|
|
public static final class SqlStatement extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements SqlStatementOrBuilder {
|
|
// Use SqlStatement.newBuilder() to construct.
|
|
private SqlStatement(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private SqlStatement(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final SqlStatement defaultInstance;
|
|
public static SqlStatement getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public SqlStatement getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private SqlStatement(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 10: {
|
|
bitField0_ |= 0x00000001;
|
|
statement_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 18: {
|
|
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
parameters_ = new java.util.ArrayList<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter>();
|
|
mutable_bitField0_ |= 0x00000002;
|
|
}
|
|
parameters_.add(input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.PARSER, extensionRegistry));
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
parameters_ = java.util.Collections.unmodifiableList(parameters_);
|
|
}
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.class, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<SqlStatement> PARSER =
|
|
new com.google.protobuf.AbstractParser<SqlStatement>() {
|
|
public SqlStatement parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new SqlStatement(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<SqlStatement> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
public interface SqlParameterOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional string stringParamter = 1;
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
boolean hasStringParamter();
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
java.lang.String getStringParamter();
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
com.google.protobuf.ByteString
|
|
getStringParamterBytes();
|
|
|
|
// optional uint64 integerParameter = 2;
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
boolean hasIntegerParameter();
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
long getIntegerParameter();
|
|
|
|
// optional double doubleParameter = 3;
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
boolean hasDoubleParameter();
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
double getDoubleParameter();
|
|
|
|
// optional bytes blobParameter = 4;
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
boolean hasBlobParameter();
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
com.google.protobuf.ByteString getBlobParameter();
|
|
|
|
// optional bool nullparameter = 5;
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
boolean hasNullparameter();
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
boolean getNullparameter();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.SqlStatement.SqlParameter}
|
|
*/
|
|
public static final class SqlParameter extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements SqlParameterOrBuilder {
|
|
// Use SqlParameter.newBuilder() to construct.
|
|
private SqlParameter(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private SqlParameter(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final SqlParameter defaultInstance;
|
|
public static SqlParameter getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public SqlParameter getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private SqlParameter(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 10: {
|
|
bitField0_ |= 0x00000001;
|
|
stringParamter_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 16: {
|
|
bitField0_ |= 0x00000002;
|
|
integerParameter_ = input.readUInt64();
|
|
break;
|
|
}
|
|
case 25: {
|
|
bitField0_ |= 0x00000004;
|
|
doubleParameter_ = input.readDouble();
|
|
break;
|
|
}
|
|
case 34: {
|
|
bitField0_ |= 0x00000008;
|
|
blobParameter_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 40: {
|
|
bitField0_ |= 0x00000010;
|
|
nullparameter_ = input.readBool();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_SqlParameter_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_SqlParameter_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.class, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<SqlParameter> PARSER =
|
|
new com.google.protobuf.AbstractParser<SqlParameter>() {
|
|
public SqlParameter parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new SqlParameter(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<SqlParameter> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional string stringParamter = 1;
|
|
public static final int STRINGPARAMTER_FIELD_NUMBER = 1;
|
|
private java.lang.Object stringParamter_;
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public boolean hasStringParamter() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public java.lang.String getStringParamter() {
|
|
java.lang.Object ref = stringParamter_;
|
|
if (ref instanceof java.lang.String) {
|
|
return (java.lang.String) ref;
|
|
} else {
|
|
com.google.protobuf.ByteString bs =
|
|
(com.google.protobuf.ByteString) ref;
|
|
java.lang.String s = bs.toStringUtf8();
|
|
if (bs.isValidUtf8()) {
|
|
stringParamter_ = s;
|
|
}
|
|
return s;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getStringParamterBytes() {
|
|
java.lang.Object ref = stringParamter_;
|
|
if (ref instanceof java.lang.String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
stringParamter_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
|
|
// optional uint64 integerParameter = 2;
|
|
public static final int INTEGERPARAMETER_FIELD_NUMBER = 2;
|
|
private long integerParameter_;
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
public boolean hasIntegerParameter() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
public long getIntegerParameter() {
|
|
return integerParameter_;
|
|
}
|
|
|
|
// optional double doubleParameter = 3;
|
|
public static final int DOUBLEPARAMETER_FIELD_NUMBER = 3;
|
|
private double doubleParameter_;
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
public boolean hasDoubleParameter() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
public double getDoubleParameter() {
|
|
return doubleParameter_;
|
|
}
|
|
|
|
// optional bytes blobParameter = 4;
|
|
public static final int BLOBPARAMETER_FIELD_NUMBER = 4;
|
|
private com.google.protobuf.ByteString blobParameter_;
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
public boolean hasBlobParameter() {
|
|
return ((bitField0_ & 0x00000008) == 0x00000008);
|
|
}
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString getBlobParameter() {
|
|
return blobParameter_;
|
|
}
|
|
|
|
// optional bool nullparameter = 5;
|
|
public static final int NULLPARAMETER_FIELD_NUMBER = 5;
|
|
private boolean nullparameter_;
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
public boolean hasNullparameter() {
|
|
return ((bitField0_ & 0x00000010) == 0x00000010);
|
|
}
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
public boolean getNullparameter() {
|
|
return nullparameter_;
|
|
}
|
|
|
|
private void initFields() {
|
|
stringParamter_ = "";
|
|
integerParameter_ = 0L;
|
|
doubleParameter_ = 0D;
|
|
blobParameter_ = com.google.protobuf.ByteString.EMPTY;
|
|
nullparameter_ = false;
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeBytes(1, getStringParamterBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeUInt64(2, integerParameter_);
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
output.writeDouble(3, doubleParameter_);
|
|
}
|
|
if (((bitField0_ & 0x00000008) == 0x00000008)) {
|
|
output.writeBytes(4, blobParameter_);
|
|
}
|
|
if (((bitField0_ & 0x00000010) == 0x00000010)) {
|
|
output.writeBool(5, nullparameter_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(1, getStringParamterBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt64Size(2, integerParameter_);
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeDoubleSize(3, doubleParameter_);
|
|
}
|
|
if (((bitField0_ & 0x00000008) == 0x00000008)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(4, blobParameter_);
|
|
}
|
|
if (((bitField0_ & 0x00000010) == 0x00000010)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBoolSize(5, nullparameter_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.SqlStatement.SqlParameter}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_SqlParameter_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_SqlParameter_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.class, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
stringParamter_ = "";
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
integerParameter_ = 0L;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
doubleParameter_ = 0D;
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
blobParameter_ = com.google.protobuf.ByteString.EMPTY;
|
|
bitField0_ = (bitField0_ & ~0x00000008);
|
|
nullparameter_ = false;
|
|
bitField0_ = (bitField0_ & ~0x00000010);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_SqlParameter_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter result = new org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.stringParamter_ = stringParamter_;
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
result.integerParameter_ = integerParameter_;
|
|
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
|
|
to_bitField0_ |= 0x00000004;
|
|
}
|
|
result.doubleParameter_ = doubleParameter_;
|
|
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
|
|
to_bitField0_ |= 0x00000008;
|
|
}
|
|
result.blobParameter_ = blobParameter_;
|
|
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
|
|
to_bitField0_ |= 0x00000010;
|
|
}
|
|
result.nullparameter_ = nullparameter_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.getDefaultInstance()) return this;
|
|
if (other.hasStringParamter()) {
|
|
bitField0_ |= 0x00000001;
|
|
stringParamter_ = other.stringParamter_;
|
|
onChanged();
|
|
}
|
|
if (other.hasIntegerParameter()) {
|
|
setIntegerParameter(other.getIntegerParameter());
|
|
}
|
|
if (other.hasDoubleParameter()) {
|
|
setDoubleParameter(other.getDoubleParameter());
|
|
}
|
|
if (other.hasBlobParameter()) {
|
|
setBlobParameter(other.getBlobParameter());
|
|
}
|
|
if (other.hasNullparameter()) {
|
|
setNullparameter(other.getNullparameter());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional string stringParamter = 1;
|
|
private java.lang.Object stringParamter_ = "";
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public boolean hasStringParamter() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public java.lang.String getStringParamter() {
|
|
java.lang.Object ref = stringParamter_;
|
|
if (!(ref instanceof java.lang.String)) {
|
|
java.lang.String s = ((com.google.protobuf.ByteString) ref)
|
|
.toStringUtf8();
|
|
stringParamter_ = s;
|
|
return s;
|
|
} else {
|
|
return (java.lang.String) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getStringParamterBytes() {
|
|
java.lang.Object ref = stringParamter_;
|
|
if (ref instanceof String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
stringParamter_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public Builder setStringParamter(
|
|
java.lang.String value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
stringParamter_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public Builder clearStringParamter() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
stringParamter_ = getDefaultInstance().getStringParamter();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string stringParamter = 1;</code>
|
|
*/
|
|
public Builder setStringParamterBytes(
|
|
com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
stringParamter_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional uint64 integerParameter = 2;
|
|
private long integerParameter_ ;
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
public boolean hasIntegerParameter() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
public long getIntegerParameter() {
|
|
return integerParameter_;
|
|
}
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
public Builder setIntegerParameter(long value) {
|
|
bitField0_ |= 0x00000002;
|
|
integerParameter_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint64 integerParameter = 2;</code>
|
|
*/
|
|
public Builder clearIntegerParameter() {
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
integerParameter_ = 0L;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional double doubleParameter = 3;
|
|
private double doubleParameter_ ;
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
public boolean hasDoubleParameter() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
public double getDoubleParameter() {
|
|
return doubleParameter_;
|
|
}
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
public Builder setDoubleParameter(double value) {
|
|
bitField0_ |= 0x00000004;
|
|
doubleParameter_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional double doubleParameter = 3;</code>
|
|
*/
|
|
public Builder clearDoubleParameter() {
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
doubleParameter_ = 0D;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional bytes blobParameter = 4;
|
|
private com.google.protobuf.ByteString blobParameter_ = com.google.protobuf.ByteString.EMPTY;
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
public boolean hasBlobParameter() {
|
|
return ((bitField0_ & 0x00000008) == 0x00000008);
|
|
}
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString getBlobParameter() {
|
|
return blobParameter_;
|
|
}
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
public Builder setBlobParameter(com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000008;
|
|
blobParameter_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional bytes blobParameter = 4;</code>
|
|
*/
|
|
public Builder clearBlobParameter() {
|
|
bitField0_ = (bitField0_ & ~0x00000008);
|
|
blobParameter_ = getDefaultInstance().getBlobParameter();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional bool nullparameter = 5;
|
|
private boolean nullparameter_ ;
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
public boolean hasNullparameter() {
|
|
return ((bitField0_ & 0x00000010) == 0x00000010);
|
|
}
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
public boolean getNullparameter() {
|
|
return nullparameter_;
|
|
}
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
public Builder setNullparameter(boolean value) {
|
|
bitField0_ |= 0x00000010;
|
|
nullparameter_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional bool nullparameter = 5;</code>
|
|
*/
|
|
public Builder clearNullparameter() {
|
|
bitField0_ = (bitField0_ & ~0x00000010);
|
|
nullparameter_ = false;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.SqlStatement.SqlParameter)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new SqlParameter(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.SqlStatement.SqlParameter)
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional string statement = 1;
|
|
public static final int STATEMENT_FIELD_NUMBER = 1;
|
|
private java.lang.Object statement_;
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public boolean hasStatement() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public java.lang.String getStatement() {
|
|
java.lang.Object ref = statement_;
|
|
if (ref instanceof java.lang.String) {
|
|
return (java.lang.String) ref;
|
|
} else {
|
|
com.google.protobuf.ByteString bs =
|
|
(com.google.protobuf.ByteString) ref;
|
|
java.lang.String s = bs.toStringUtf8();
|
|
if (bs.isValidUtf8()) {
|
|
statement_ = s;
|
|
}
|
|
return s;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getStatementBytes() {
|
|
java.lang.Object ref = statement_;
|
|
if (ref instanceof java.lang.String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
statement_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
|
|
// repeated .signal.SqlStatement.SqlParameter parameters = 2;
|
|
public static final int PARAMETERS_FIELD_NUMBER = 2;
|
|
private java.util.List<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter> parameters_;
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public java.util.List<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter> getParametersList() {
|
|
return parameters_;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public java.util.List<? extends org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder>
|
|
getParametersOrBuilderList() {
|
|
return parameters_;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public int getParametersCount() {
|
|
return parameters_.size();
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter getParameters(int index) {
|
|
return parameters_.get(index);
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder getParametersOrBuilder(
|
|
int index) {
|
|
return parameters_.get(index);
|
|
}
|
|
|
|
private void initFields() {
|
|
statement_ = "";
|
|
parameters_ = java.util.Collections.emptyList();
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeBytes(1, getStatementBytes());
|
|
}
|
|
for (int i = 0; i < parameters_.size(); i++) {
|
|
output.writeMessage(2, parameters_.get(i));
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(1, getStatementBytes());
|
|
}
|
|
for (int i = 0; i < parameters_.size(); i++) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(2, parameters_.get(i));
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.SqlStatement}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.class, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
getParametersFieldBuilder();
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
statement_ = "";
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
if (parametersBuilder_ == null) {
|
|
parameters_ = java.util.Collections.emptyList();
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
} else {
|
|
parametersBuilder_.clear();
|
|
}
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SqlStatement_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement result = new org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.statement_ = statement_;
|
|
if (parametersBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
parameters_ = java.util.Collections.unmodifiableList(parameters_);
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
}
|
|
result.parameters_ = parameters_;
|
|
} else {
|
|
result.parameters_ = parametersBuilder_.build();
|
|
}
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance()) return this;
|
|
if (other.hasStatement()) {
|
|
bitField0_ |= 0x00000001;
|
|
statement_ = other.statement_;
|
|
onChanged();
|
|
}
|
|
if (parametersBuilder_ == null) {
|
|
if (!other.parameters_.isEmpty()) {
|
|
if (parameters_.isEmpty()) {
|
|
parameters_ = other.parameters_;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
} else {
|
|
ensureParametersIsMutable();
|
|
parameters_.addAll(other.parameters_);
|
|
}
|
|
onChanged();
|
|
}
|
|
} else {
|
|
if (!other.parameters_.isEmpty()) {
|
|
if (parametersBuilder_.isEmpty()) {
|
|
parametersBuilder_.dispose();
|
|
parametersBuilder_ = null;
|
|
parameters_ = other.parameters_;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
parametersBuilder_ =
|
|
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
|
|
getParametersFieldBuilder() : null;
|
|
} else {
|
|
parametersBuilder_.addAllMessages(other.parameters_);
|
|
}
|
|
}
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional string statement = 1;
|
|
private java.lang.Object statement_ = "";
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public boolean hasStatement() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public java.lang.String getStatement() {
|
|
java.lang.Object ref = statement_;
|
|
if (!(ref instanceof java.lang.String)) {
|
|
java.lang.String s = ((com.google.protobuf.ByteString) ref)
|
|
.toStringUtf8();
|
|
statement_ = s;
|
|
return s;
|
|
} else {
|
|
return (java.lang.String) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getStatementBytes() {
|
|
java.lang.Object ref = statement_;
|
|
if (ref instanceof String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
statement_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public Builder setStatement(
|
|
java.lang.String value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
statement_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public Builder clearStatement() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
statement_ = getDefaultInstance().getStatement();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string statement = 1;</code>
|
|
*/
|
|
public Builder setStatementBytes(
|
|
com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
statement_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// repeated .signal.SqlStatement.SqlParameter parameters = 2;
|
|
private java.util.List<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter> parameters_ =
|
|
java.util.Collections.emptyList();
|
|
private void ensureParametersIsMutable() {
|
|
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
parameters_ = new java.util.ArrayList<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter>(parameters_);
|
|
bitField0_ |= 0x00000002;
|
|
}
|
|
}
|
|
|
|
private com.google.protobuf.RepeatedFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder> parametersBuilder_;
|
|
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public java.util.List<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter> getParametersList() {
|
|
if (parametersBuilder_ == null) {
|
|
return java.util.Collections.unmodifiableList(parameters_);
|
|
} else {
|
|
return parametersBuilder_.getMessageList();
|
|
}
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public int getParametersCount() {
|
|
if (parametersBuilder_ == null) {
|
|
return parameters_.size();
|
|
} else {
|
|
return parametersBuilder_.getCount();
|
|
}
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter getParameters(int index) {
|
|
if (parametersBuilder_ == null) {
|
|
return parameters_.get(index);
|
|
} else {
|
|
return parametersBuilder_.getMessage(index);
|
|
}
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder setParameters(
|
|
int index, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter value) {
|
|
if (parametersBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
ensureParametersIsMutable();
|
|
parameters_.set(index, value);
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.setMessage(index, value);
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder setParameters(
|
|
int index, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder builderForValue) {
|
|
if (parametersBuilder_ == null) {
|
|
ensureParametersIsMutable();
|
|
parameters_.set(index, builderForValue.build());
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.setMessage(index, builderForValue.build());
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder addParameters(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter value) {
|
|
if (parametersBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
ensureParametersIsMutable();
|
|
parameters_.add(value);
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.addMessage(value);
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder addParameters(
|
|
int index, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter value) {
|
|
if (parametersBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
ensureParametersIsMutable();
|
|
parameters_.add(index, value);
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.addMessage(index, value);
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder addParameters(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder builderForValue) {
|
|
if (parametersBuilder_ == null) {
|
|
ensureParametersIsMutable();
|
|
parameters_.add(builderForValue.build());
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.addMessage(builderForValue.build());
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder addParameters(
|
|
int index, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder builderForValue) {
|
|
if (parametersBuilder_ == null) {
|
|
ensureParametersIsMutable();
|
|
parameters_.add(index, builderForValue.build());
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.addMessage(index, builderForValue.build());
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder addAllParameters(
|
|
java.lang.Iterable<? extends org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter> values) {
|
|
if (parametersBuilder_ == null) {
|
|
ensureParametersIsMutable();
|
|
super.addAll(values, parameters_);
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.addAllMessages(values);
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder clearParameters() {
|
|
if (parametersBuilder_ == null) {
|
|
parameters_ = java.util.Collections.emptyList();
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.clear();
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public Builder removeParameters(int index) {
|
|
if (parametersBuilder_ == null) {
|
|
ensureParametersIsMutable();
|
|
parameters_.remove(index);
|
|
onChanged();
|
|
} else {
|
|
parametersBuilder_.remove(index);
|
|
}
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder getParametersBuilder(
|
|
int index) {
|
|
return getParametersFieldBuilder().getBuilder(index);
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder getParametersOrBuilder(
|
|
int index) {
|
|
if (parametersBuilder_ == null) {
|
|
return parameters_.get(index); } else {
|
|
return parametersBuilder_.getMessageOrBuilder(index);
|
|
}
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public java.util.List<? extends org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder>
|
|
getParametersOrBuilderList() {
|
|
if (parametersBuilder_ != null) {
|
|
return parametersBuilder_.getMessageOrBuilderList();
|
|
} else {
|
|
return java.util.Collections.unmodifiableList(parameters_);
|
|
}
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder addParametersBuilder() {
|
|
return getParametersFieldBuilder().addBuilder(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.getDefaultInstance());
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder addParametersBuilder(
|
|
int index) {
|
|
return getParametersFieldBuilder().addBuilder(
|
|
index, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.getDefaultInstance());
|
|
}
|
|
/**
|
|
* <code>repeated .signal.SqlStatement.SqlParameter parameters = 2;</code>
|
|
*/
|
|
public java.util.List<org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder>
|
|
getParametersBuilderList() {
|
|
return getParametersFieldBuilder().getBuilderList();
|
|
}
|
|
private com.google.protobuf.RepeatedFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder>
|
|
getParametersFieldBuilder() {
|
|
if (parametersBuilder_ == null) {
|
|
parametersBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameter.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.SqlParameterOrBuilder>(
|
|
parameters_,
|
|
((bitField0_ & 0x00000002) == 0x00000002),
|
|
getParentForChildren(),
|
|
isClean());
|
|
parameters_ = null;
|
|
}
|
|
return parametersBuilder_;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.SqlStatement)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new SqlStatement(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.SqlStatement)
|
|
}
|
|
|
|
public interface SharedPreferenceOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional string file = 1;
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
boolean hasFile();
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
java.lang.String getFile();
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
com.google.protobuf.ByteString
|
|
getFileBytes();
|
|
|
|
// optional string key = 2;
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
boolean hasKey();
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
java.lang.String getKey();
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
com.google.protobuf.ByteString
|
|
getKeyBytes();
|
|
|
|
// optional string value = 3;
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
boolean hasValue();
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
java.lang.String getValue();
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
com.google.protobuf.ByteString
|
|
getValueBytes();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.SharedPreference}
|
|
*/
|
|
public static final class SharedPreference extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements SharedPreferenceOrBuilder {
|
|
// Use SharedPreference.newBuilder() to construct.
|
|
private SharedPreference(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private SharedPreference(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final SharedPreference defaultInstance;
|
|
public static SharedPreference getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public SharedPreference getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private SharedPreference(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 10: {
|
|
bitField0_ |= 0x00000001;
|
|
file_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 18: {
|
|
bitField0_ |= 0x00000002;
|
|
key_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 26: {
|
|
bitField0_ |= 0x00000004;
|
|
value_ = input.readBytes();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SharedPreference_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SharedPreference_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.class, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<SharedPreference> PARSER =
|
|
new com.google.protobuf.AbstractParser<SharedPreference>() {
|
|
public SharedPreference parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new SharedPreference(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<SharedPreference> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional string file = 1;
|
|
public static final int FILE_FIELD_NUMBER = 1;
|
|
private java.lang.Object file_;
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public boolean hasFile() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public java.lang.String getFile() {
|
|
java.lang.Object ref = file_;
|
|
if (ref instanceof java.lang.String) {
|
|
return (java.lang.String) ref;
|
|
} else {
|
|
com.google.protobuf.ByteString bs =
|
|
(com.google.protobuf.ByteString) ref;
|
|
java.lang.String s = bs.toStringUtf8();
|
|
if (bs.isValidUtf8()) {
|
|
file_ = s;
|
|
}
|
|
return s;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getFileBytes() {
|
|
java.lang.Object ref = file_;
|
|
if (ref instanceof java.lang.String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
file_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
|
|
// optional string key = 2;
|
|
public static final int KEY_FIELD_NUMBER = 2;
|
|
private java.lang.Object key_;
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public boolean hasKey() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public java.lang.String getKey() {
|
|
java.lang.Object ref = key_;
|
|
if (ref instanceof java.lang.String) {
|
|
return (java.lang.String) ref;
|
|
} else {
|
|
com.google.protobuf.ByteString bs =
|
|
(com.google.protobuf.ByteString) ref;
|
|
java.lang.String s = bs.toStringUtf8();
|
|
if (bs.isValidUtf8()) {
|
|
key_ = s;
|
|
}
|
|
return s;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getKeyBytes() {
|
|
java.lang.Object ref = key_;
|
|
if (ref instanceof java.lang.String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
key_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
|
|
// optional string value = 3;
|
|
public static final int VALUE_FIELD_NUMBER = 3;
|
|
private java.lang.Object value_;
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public boolean hasValue() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public java.lang.String getValue() {
|
|
java.lang.Object ref = value_;
|
|
if (ref instanceof java.lang.String) {
|
|
return (java.lang.String) ref;
|
|
} else {
|
|
com.google.protobuf.ByteString bs =
|
|
(com.google.protobuf.ByteString) ref;
|
|
java.lang.String s = bs.toStringUtf8();
|
|
if (bs.isValidUtf8()) {
|
|
value_ = s;
|
|
}
|
|
return s;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getValueBytes() {
|
|
java.lang.Object ref = value_;
|
|
if (ref instanceof java.lang.String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
value_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
|
|
private void initFields() {
|
|
file_ = "";
|
|
key_ = "";
|
|
value_ = "";
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeBytes(1, getFileBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeBytes(2, getKeyBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
output.writeBytes(3, getValueBytes());
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(1, getFileBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(2, getKeyBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(3, getValueBytes());
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.SharedPreference}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SharedPreference_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SharedPreference_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.class, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
file_ = "";
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
key_ = "";
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
value_ = "";
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_SharedPreference_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference result = new org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.file_ = file_;
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
result.key_ = key_;
|
|
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
|
|
to_bitField0_ |= 0x00000004;
|
|
}
|
|
result.value_ = value_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance()) return this;
|
|
if (other.hasFile()) {
|
|
bitField0_ |= 0x00000001;
|
|
file_ = other.file_;
|
|
onChanged();
|
|
}
|
|
if (other.hasKey()) {
|
|
bitField0_ |= 0x00000002;
|
|
key_ = other.key_;
|
|
onChanged();
|
|
}
|
|
if (other.hasValue()) {
|
|
bitField0_ |= 0x00000004;
|
|
value_ = other.value_;
|
|
onChanged();
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional string file = 1;
|
|
private java.lang.Object file_ = "";
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public boolean hasFile() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public java.lang.String getFile() {
|
|
java.lang.Object ref = file_;
|
|
if (!(ref instanceof java.lang.String)) {
|
|
java.lang.String s = ((com.google.protobuf.ByteString) ref)
|
|
.toStringUtf8();
|
|
file_ = s;
|
|
return s;
|
|
} else {
|
|
return (java.lang.String) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getFileBytes() {
|
|
java.lang.Object ref = file_;
|
|
if (ref instanceof String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
file_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public Builder setFile(
|
|
java.lang.String value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
file_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public Builder clearFile() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
file_ = getDefaultInstance().getFile();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string file = 1;</code>
|
|
*/
|
|
public Builder setFileBytes(
|
|
com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
file_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional string key = 2;
|
|
private java.lang.Object key_ = "";
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public boolean hasKey() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public java.lang.String getKey() {
|
|
java.lang.Object ref = key_;
|
|
if (!(ref instanceof java.lang.String)) {
|
|
java.lang.String s = ((com.google.protobuf.ByteString) ref)
|
|
.toStringUtf8();
|
|
key_ = s;
|
|
return s;
|
|
} else {
|
|
return (java.lang.String) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getKeyBytes() {
|
|
java.lang.Object ref = key_;
|
|
if (ref instanceof String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
key_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public Builder setKey(
|
|
java.lang.String value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
key_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public Builder clearKey() {
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
key_ = getDefaultInstance().getKey();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string key = 2;</code>
|
|
*/
|
|
public Builder setKeyBytes(
|
|
com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
key_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional string value = 3;
|
|
private java.lang.Object value_ = "";
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public boolean hasValue() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public java.lang.String getValue() {
|
|
java.lang.Object ref = value_;
|
|
if (!(ref instanceof java.lang.String)) {
|
|
java.lang.String s = ((com.google.protobuf.ByteString) ref)
|
|
.toStringUtf8();
|
|
value_ = s;
|
|
return s;
|
|
} else {
|
|
return (java.lang.String) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getValueBytes() {
|
|
java.lang.Object ref = value_;
|
|
if (ref instanceof String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
value_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public Builder setValue(
|
|
java.lang.String value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000004;
|
|
value_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public Builder clearValue() {
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
value_ = getDefaultInstance().getValue();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string value = 3;</code>
|
|
*/
|
|
public Builder setValueBytes(
|
|
com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000004;
|
|
value_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.SharedPreference)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new SharedPreference(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.SharedPreference)
|
|
}
|
|
|
|
public interface AttachmentOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional uint64 rowId = 1;
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
boolean hasRowId();
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
long getRowId();
|
|
|
|
// optional uint64 attachmentId = 2;
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
boolean hasAttachmentId();
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
long getAttachmentId();
|
|
|
|
// optional uint32 length = 3;
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
boolean hasLength();
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
int getLength();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Attachment}
|
|
*/
|
|
public static final class Attachment extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements AttachmentOrBuilder {
|
|
// Use Attachment.newBuilder() to construct.
|
|
private Attachment(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private Attachment(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final Attachment defaultInstance;
|
|
public static Attachment getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public Attachment getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private Attachment(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 8: {
|
|
bitField0_ |= 0x00000001;
|
|
rowId_ = input.readUInt64();
|
|
break;
|
|
}
|
|
case 16: {
|
|
bitField0_ |= 0x00000002;
|
|
attachmentId_ = input.readUInt64();
|
|
break;
|
|
}
|
|
case 24: {
|
|
bitField0_ |= 0x00000004;
|
|
length_ = input.readUInt32();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Attachment_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Attachment_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment.class, org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<Attachment> PARSER =
|
|
new com.google.protobuf.AbstractParser<Attachment>() {
|
|
public Attachment parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new Attachment(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<Attachment> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional uint64 rowId = 1;
|
|
public static final int ROWID_FIELD_NUMBER = 1;
|
|
private long rowId_;
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public boolean hasRowId() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public long getRowId() {
|
|
return rowId_;
|
|
}
|
|
|
|
// optional uint64 attachmentId = 2;
|
|
public static final int ATTACHMENTID_FIELD_NUMBER = 2;
|
|
private long attachmentId_;
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
public boolean hasAttachmentId() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
public long getAttachmentId() {
|
|
return attachmentId_;
|
|
}
|
|
|
|
// optional uint32 length = 3;
|
|
public static final int LENGTH_FIELD_NUMBER = 3;
|
|
private int length_;
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
public boolean hasLength() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
public int getLength() {
|
|
return length_;
|
|
}
|
|
|
|
private void initFields() {
|
|
rowId_ = 0L;
|
|
attachmentId_ = 0L;
|
|
length_ = 0;
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeUInt64(1, rowId_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeUInt64(2, attachmentId_);
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
output.writeUInt32(3, length_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt64Size(1, rowId_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt64Size(2, attachmentId_);
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt32Size(3, length_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Attachment parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.Attachment prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Attachment}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Attachment_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Attachment_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment.class, org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.Attachment.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
rowId_ = 0L;
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
attachmentId_ = 0L;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
length_ = 0;
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Attachment_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Attachment getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Attachment build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Attachment buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment result = new org.thoughtcrime.securesms.backup.BackupProtos.Attachment(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.rowId_ = rowId_;
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
result.attachmentId_ = attachmentId_;
|
|
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
|
|
to_bitField0_ |= 0x00000004;
|
|
}
|
|
result.length_ = length_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.Attachment) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.Attachment)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.Attachment other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance()) return this;
|
|
if (other.hasRowId()) {
|
|
setRowId(other.getRowId());
|
|
}
|
|
if (other.hasAttachmentId()) {
|
|
setAttachmentId(other.getAttachmentId());
|
|
}
|
|
if (other.hasLength()) {
|
|
setLength(other.getLength());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.Attachment) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional uint64 rowId = 1;
|
|
private long rowId_ ;
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public boolean hasRowId() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public long getRowId() {
|
|
return rowId_;
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public Builder setRowId(long value) {
|
|
bitField0_ |= 0x00000001;
|
|
rowId_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public Builder clearRowId() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
rowId_ = 0L;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional uint64 attachmentId = 2;
|
|
private long attachmentId_ ;
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
public boolean hasAttachmentId() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
public long getAttachmentId() {
|
|
return attachmentId_;
|
|
}
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
public Builder setAttachmentId(long value) {
|
|
bitField0_ |= 0x00000002;
|
|
attachmentId_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint64 attachmentId = 2;</code>
|
|
*/
|
|
public Builder clearAttachmentId() {
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
attachmentId_ = 0L;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional uint32 length = 3;
|
|
private int length_ ;
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
public boolean hasLength() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
public int getLength() {
|
|
return length_;
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
public Builder setLength(int value) {
|
|
bitField0_ |= 0x00000004;
|
|
length_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 3;</code>
|
|
*/
|
|
public Builder clearLength() {
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
length_ = 0;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.Attachment)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new Attachment(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.Attachment)
|
|
}
|
|
|
|
public interface StickerOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional uint64 rowId = 1;
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
boolean hasRowId();
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
long getRowId();
|
|
|
|
// optional uint32 length = 2;
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
boolean hasLength();
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
int getLength();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Sticker}
|
|
*/
|
|
public static final class Sticker extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements StickerOrBuilder {
|
|
// Use Sticker.newBuilder() to construct.
|
|
private Sticker(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private Sticker(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final Sticker defaultInstance;
|
|
public static Sticker getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public Sticker getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private Sticker(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 8: {
|
|
bitField0_ |= 0x00000001;
|
|
rowId_ = input.readUInt64();
|
|
break;
|
|
}
|
|
case 16: {
|
|
bitField0_ |= 0x00000002;
|
|
length_ = input.readUInt32();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Sticker_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Sticker_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker.class, org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<Sticker> PARSER =
|
|
new com.google.protobuf.AbstractParser<Sticker>() {
|
|
public Sticker parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new Sticker(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<Sticker> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional uint64 rowId = 1;
|
|
public static final int ROWID_FIELD_NUMBER = 1;
|
|
private long rowId_;
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public boolean hasRowId() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public long getRowId() {
|
|
return rowId_;
|
|
}
|
|
|
|
// optional uint32 length = 2;
|
|
public static final int LENGTH_FIELD_NUMBER = 2;
|
|
private int length_;
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public boolean hasLength() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public int getLength() {
|
|
return length_;
|
|
}
|
|
|
|
private void initFields() {
|
|
rowId_ = 0L;
|
|
length_ = 0;
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeUInt64(1, rowId_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeUInt32(2, length_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt64Size(1, rowId_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt32Size(2, length_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Sticker parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.Sticker prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Sticker}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Sticker_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Sticker_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker.class, org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.Sticker.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
rowId_ = 0L;
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
length_ = 0;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Sticker_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Sticker getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Sticker build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Sticker buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker result = new org.thoughtcrime.securesms.backup.BackupProtos.Sticker(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.rowId_ = rowId_;
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
result.length_ = length_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.Sticker) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.Sticker)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.Sticker other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance()) return this;
|
|
if (other.hasRowId()) {
|
|
setRowId(other.getRowId());
|
|
}
|
|
if (other.hasLength()) {
|
|
setLength(other.getLength());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.Sticker) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional uint64 rowId = 1;
|
|
private long rowId_ ;
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public boolean hasRowId() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public long getRowId() {
|
|
return rowId_;
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public Builder setRowId(long value) {
|
|
bitField0_ |= 0x00000001;
|
|
rowId_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint64 rowId = 1;</code>
|
|
*/
|
|
public Builder clearRowId() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
rowId_ = 0L;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional uint32 length = 2;
|
|
private int length_ ;
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public boolean hasLength() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public int getLength() {
|
|
return length_;
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public Builder setLength(int value) {
|
|
bitField0_ |= 0x00000002;
|
|
length_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public Builder clearLength() {
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
length_ = 0;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.Sticker)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new Sticker(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.Sticker)
|
|
}
|
|
|
|
public interface AvatarOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional string name = 1;
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
boolean hasName();
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
java.lang.String getName();
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
com.google.protobuf.ByteString
|
|
getNameBytes();
|
|
|
|
// optional uint32 length = 2;
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
boolean hasLength();
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
int getLength();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Avatar}
|
|
*/
|
|
public static final class Avatar extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements AvatarOrBuilder {
|
|
// Use Avatar.newBuilder() to construct.
|
|
private Avatar(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private Avatar(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final Avatar defaultInstance;
|
|
public static Avatar getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public Avatar getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private Avatar(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 10: {
|
|
bitField0_ |= 0x00000001;
|
|
name_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 16: {
|
|
bitField0_ |= 0x00000002;
|
|
length_ = input.readUInt32();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Avatar_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Avatar_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar.class, org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<Avatar> PARSER =
|
|
new com.google.protobuf.AbstractParser<Avatar>() {
|
|
public Avatar parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new Avatar(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<Avatar> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional string name = 1;
|
|
public static final int NAME_FIELD_NUMBER = 1;
|
|
private java.lang.Object name_;
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public boolean hasName() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public java.lang.String getName() {
|
|
java.lang.Object ref = name_;
|
|
if (ref instanceof java.lang.String) {
|
|
return (java.lang.String) ref;
|
|
} else {
|
|
com.google.protobuf.ByteString bs =
|
|
(com.google.protobuf.ByteString) ref;
|
|
java.lang.String s = bs.toStringUtf8();
|
|
if (bs.isValidUtf8()) {
|
|
name_ = s;
|
|
}
|
|
return s;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getNameBytes() {
|
|
java.lang.Object ref = name_;
|
|
if (ref instanceof java.lang.String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
name_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
|
|
// optional uint32 length = 2;
|
|
public static final int LENGTH_FIELD_NUMBER = 2;
|
|
private int length_;
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public boolean hasLength() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public int getLength() {
|
|
return length_;
|
|
}
|
|
|
|
private void initFields() {
|
|
name_ = "";
|
|
length_ = 0;
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeBytes(1, getNameBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeUInt32(2, length_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(1, getNameBytes());
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt32Size(2, length_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Avatar parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.Avatar prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Avatar}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Avatar_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Avatar_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar.class, org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.Avatar.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
name_ = "";
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
length_ = 0;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Avatar_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Avatar getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Avatar build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Avatar buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar result = new org.thoughtcrime.securesms.backup.BackupProtos.Avatar(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.name_ = name_;
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
result.length_ = length_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.Avatar) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.Avatar)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.Avatar other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance()) return this;
|
|
if (other.hasName()) {
|
|
bitField0_ |= 0x00000001;
|
|
name_ = other.name_;
|
|
onChanged();
|
|
}
|
|
if (other.hasLength()) {
|
|
setLength(other.getLength());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.Avatar) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional string name = 1;
|
|
private java.lang.Object name_ = "";
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public boolean hasName() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public java.lang.String getName() {
|
|
java.lang.Object ref = name_;
|
|
if (!(ref instanceof java.lang.String)) {
|
|
java.lang.String s = ((com.google.protobuf.ByteString) ref)
|
|
.toStringUtf8();
|
|
name_ = s;
|
|
return s;
|
|
} else {
|
|
return (java.lang.String) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString
|
|
getNameBytes() {
|
|
java.lang.Object ref = name_;
|
|
if (ref instanceof String) {
|
|
com.google.protobuf.ByteString b =
|
|
com.google.protobuf.ByteString.copyFromUtf8(
|
|
(java.lang.String) ref);
|
|
name_ = b;
|
|
return b;
|
|
} else {
|
|
return (com.google.protobuf.ByteString) ref;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public Builder setName(
|
|
java.lang.String value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
name_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public Builder clearName() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
name_ = getDefaultInstance().getName();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional string name = 1;</code>
|
|
*/
|
|
public Builder setNameBytes(
|
|
com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
name_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional uint32 length = 2;
|
|
private int length_ ;
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public boolean hasLength() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public int getLength() {
|
|
return length_;
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public Builder setLength(int value) {
|
|
bitField0_ |= 0x00000002;
|
|
length_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint32 length = 2;</code>
|
|
*/
|
|
public Builder clearLength() {
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
length_ = 0;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.Avatar)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new Avatar(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.Avatar)
|
|
}
|
|
|
|
public interface DatabaseVersionOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional uint32 version = 1;
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
boolean hasVersion();
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
int getVersion();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.DatabaseVersion}
|
|
*/
|
|
public static final class DatabaseVersion extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements DatabaseVersionOrBuilder {
|
|
// Use DatabaseVersion.newBuilder() to construct.
|
|
private DatabaseVersion(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private DatabaseVersion(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final DatabaseVersion defaultInstance;
|
|
public static DatabaseVersion getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public DatabaseVersion getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private DatabaseVersion(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 8: {
|
|
bitField0_ |= 0x00000001;
|
|
version_ = input.readUInt32();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_DatabaseVersion_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_DatabaseVersion_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.class, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<DatabaseVersion> PARSER =
|
|
new com.google.protobuf.AbstractParser<DatabaseVersion>() {
|
|
public DatabaseVersion parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new DatabaseVersion(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<DatabaseVersion> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional uint32 version = 1;
|
|
public static final int VERSION_FIELD_NUMBER = 1;
|
|
private int version_;
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
public boolean hasVersion() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
public int getVersion() {
|
|
return version_;
|
|
}
|
|
|
|
private void initFields() {
|
|
version_ = 0;
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeUInt32(1, version_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeUInt32Size(1, version_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.DatabaseVersion}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_DatabaseVersion_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_DatabaseVersion_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.class, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
version_ = 0;
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_DatabaseVersion_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion result = new org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.version_ = version_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance()) return this;
|
|
if (other.hasVersion()) {
|
|
setVersion(other.getVersion());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional uint32 version = 1;
|
|
private int version_ ;
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
public boolean hasVersion() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
public int getVersion() {
|
|
return version_;
|
|
}
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
public Builder setVersion(int value) {
|
|
bitField0_ |= 0x00000001;
|
|
version_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional uint32 version = 1;</code>
|
|
*/
|
|
public Builder clearVersion() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
version_ = 0;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.DatabaseVersion)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new DatabaseVersion(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.DatabaseVersion)
|
|
}
|
|
|
|
public interface HeaderOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional bytes iv = 1;
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
boolean hasIv();
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
com.google.protobuf.ByteString getIv();
|
|
|
|
// optional bytes salt = 2;
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
boolean hasSalt();
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
com.google.protobuf.ByteString getSalt();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Header}
|
|
*/
|
|
public static final class Header extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements HeaderOrBuilder {
|
|
// Use Header.newBuilder() to construct.
|
|
private Header(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private Header(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final Header defaultInstance;
|
|
public static Header getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public Header getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private Header(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 10: {
|
|
bitField0_ |= 0x00000001;
|
|
iv_ = input.readBytes();
|
|
break;
|
|
}
|
|
case 18: {
|
|
bitField0_ |= 0x00000002;
|
|
salt_ = input.readBytes();
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Header_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Header_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header.class, org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<Header> PARSER =
|
|
new com.google.protobuf.AbstractParser<Header>() {
|
|
public Header parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new Header(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<Header> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional bytes iv = 1;
|
|
public static final int IV_FIELD_NUMBER = 1;
|
|
private com.google.protobuf.ByteString iv_;
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
public boolean hasIv() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString getIv() {
|
|
return iv_;
|
|
}
|
|
|
|
// optional bytes salt = 2;
|
|
public static final int SALT_FIELD_NUMBER = 2;
|
|
private com.google.protobuf.ByteString salt_;
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
public boolean hasSalt() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString getSalt() {
|
|
return salt_;
|
|
}
|
|
|
|
private void initFields() {
|
|
iv_ = com.google.protobuf.ByteString.EMPTY;
|
|
salt_ = com.google.protobuf.ByteString.EMPTY;
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeBytes(1, iv_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeBytes(2, salt_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(1, iv_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBytesSize(2, salt_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.Header parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.Header prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.Header}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Header_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Header_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header.class, org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.Header.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
iv_ = com.google.protobuf.ByteString.EMPTY;
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
salt_ = com.google.protobuf.ByteString.EMPTY;
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_Header_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Header getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Header build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Header buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header result = new org.thoughtcrime.securesms.backup.BackupProtos.Header(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
result.iv_ = iv_;
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
result.salt_ = salt_;
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.Header) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.Header)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.Header other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance()) return this;
|
|
if (other.hasIv()) {
|
|
setIv(other.getIv());
|
|
}
|
|
if (other.hasSalt()) {
|
|
setSalt(other.getSalt());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.Header) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional bytes iv = 1;
|
|
private com.google.protobuf.ByteString iv_ = com.google.protobuf.ByteString.EMPTY;
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
public boolean hasIv() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString getIv() {
|
|
return iv_;
|
|
}
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
public Builder setIv(com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
iv_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional bytes iv = 1;</code>
|
|
*/
|
|
public Builder clearIv() {
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
iv_ = getDefaultInstance().getIv();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional bytes salt = 2;
|
|
private com.google.protobuf.ByteString salt_ = com.google.protobuf.ByteString.EMPTY;
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
public boolean hasSalt() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
public com.google.protobuf.ByteString getSalt() {
|
|
return salt_;
|
|
}
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
public Builder setSalt(com.google.protobuf.ByteString value) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
salt_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional bytes salt = 2;</code>
|
|
*/
|
|
public Builder clearSalt() {
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
salt_ = getDefaultInstance().getSalt();
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.Header)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new Header(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.Header)
|
|
}
|
|
|
|
public interface BackupFrameOrBuilder
|
|
extends com.google.protobuf.MessageOrBuilder {
|
|
|
|
// optional .signal.Header header = 1;
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
boolean hasHeader();
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header getHeader();
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder getHeaderOrBuilder();
|
|
|
|
// optional .signal.SqlStatement statement = 2;
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
boolean hasStatement();
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement getStatement();
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder getStatementOrBuilder();
|
|
|
|
// optional .signal.SharedPreference preference = 3;
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
boolean hasPreference();
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference getPreference();
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder getPreferenceOrBuilder();
|
|
|
|
// optional .signal.Attachment attachment = 4;
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
boolean hasAttachment();
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment getAttachment();
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder getAttachmentOrBuilder();
|
|
|
|
// optional .signal.DatabaseVersion version = 5;
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
boolean hasVersion();
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion getVersion();
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder getVersionOrBuilder();
|
|
|
|
// optional bool end = 6;
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
boolean hasEnd();
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
boolean getEnd();
|
|
|
|
// optional .signal.Avatar avatar = 7;
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
boolean hasAvatar();
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar getAvatar();
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder getAvatarOrBuilder();
|
|
|
|
// optional .signal.Sticker sticker = 8;
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
boolean hasSticker();
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker getSticker();
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder getStickerOrBuilder();
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.BackupFrame}
|
|
*/
|
|
public static final class BackupFrame extends
|
|
com.google.protobuf.GeneratedMessage
|
|
implements BackupFrameOrBuilder {
|
|
// Use BackupFrame.newBuilder() to construct.
|
|
private BackupFrame(com.google.protobuf.GeneratedMessage.Builder<?> builder) {
|
|
super(builder);
|
|
this.unknownFields = builder.getUnknownFields();
|
|
}
|
|
private BackupFrame(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
|
|
|
|
private static final BackupFrame defaultInstance;
|
|
public static BackupFrame getDefaultInstance() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
public BackupFrame getDefaultInstanceForType() {
|
|
return defaultInstance;
|
|
}
|
|
|
|
private final com.google.protobuf.UnknownFieldSet unknownFields;
|
|
@java.lang.Override
|
|
public final com.google.protobuf.UnknownFieldSet
|
|
getUnknownFields() {
|
|
return this.unknownFields;
|
|
}
|
|
private BackupFrame(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
initFields();
|
|
int mutable_bitField0_ = 0;
|
|
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
|
|
com.google.protobuf.UnknownFieldSet.newBuilder();
|
|
try {
|
|
boolean done = false;
|
|
while (!done) {
|
|
int tag = input.readTag();
|
|
switch (tag) {
|
|
case 0:
|
|
done = true;
|
|
break;
|
|
default: {
|
|
if (!parseUnknownField(input, unknownFields,
|
|
extensionRegistry, tag)) {
|
|
done = true;
|
|
}
|
|
break;
|
|
}
|
|
case 10: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
subBuilder = header_.toBuilder();
|
|
}
|
|
header_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.Header.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(header_);
|
|
header_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
break;
|
|
}
|
|
case 18: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
subBuilder = statement_.toBuilder();
|
|
}
|
|
statement_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(statement_);
|
|
statement_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
break;
|
|
}
|
|
case 26: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
subBuilder = preference_.toBuilder();
|
|
}
|
|
preference_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(preference_);
|
|
preference_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000004;
|
|
break;
|
|
}
|
|
case 34: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000008) == 0x00000008)) {
|
|
subBuilder = attachment_.toBuilder();
|
|
}
|
|
attachment_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.Attachment.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(attachment_);
|
|
attachment_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000008;
|
|
break;
|
|
}
|
|
case 42: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000010) == 0x00000010)) {
|
|
subBuilder = version_.toBuilder();
|
|
}
|
|
version_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(version_);
|
|
version_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000010;
|
|
break;
|
|
}
|
|
case 48: {
|
|
bitField0_ |= 0x00000020;
|
|
end_ = input.readBool();
|
|
break;
|
|
}
|
|
case 58: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000040) == 0x00000040)) {
|
|
subBuilder = avatar_.toBuilder();
|
|
}
|
|
avatar_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.Avatar.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(avatar_);
|
|
avatar_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000040;
|
|
break;
|
|
}
|
|
case 66: {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder subBuilder = null;
|
|
if (((bitField0_ & 0x00000080) == 0x00000080)) {
|
|
subBuilder = sticker_.toBuilder();
|
|
}
|
|
sticker_ = input.readMessage(org.thoughtcrime.securesms.backup.BackupProtos.Sticker.PARSER, extensionRegistry);
|
|
if (subBuilder != null) {
|
|
subBuilder.mergeFrom(sticker_);
|
|
sticker_ = subBuilder.buildPartial();
|
|
}
|
|
bitField0_ |= 0x00000080;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
throw e.setUnfinishedMessage(this);
|
|
} catch (java.io.IOException e) {
|
|
throw new com.google.protobuf.InvalidProtocolBufferException(
|
|
e.getMessage()).setUnfinishedMessage(this);
|
|
} finally {
|
|
this.unknownFields = unknownFields.build();
|
|
makeExtensionsImmutable();
|
|
}
|
|
}
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_BackupFrame_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_BackupFrame_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.class, org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.Builder.class);
|
|
}
|
|
|
|
public static com.google.protobuf.Parser<BackupFrame> PARSER =
|
|
new com.google.protobuf.AbstractParser<BackupFrame>() {
|
|
public BackupFrame parsePartialFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return new BackupFrame(input, extensionRegistry);
|
|
}
|
|
};
|
|
|
|
@java.lang.Override
|
|
public com.google.protobuf.Parser<BackupFrame> getParserForType() {
|
|
return PARSER;
|
|
}
|
|
|
|
private int bitField0_;
|
|
// optional .signal.Header header = 1;
|
|
public static final int HEADER_FIELD_NUMBER = 1;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Header header_;
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public boolean hasHeader() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Header getHeader() {
|
|
return header_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder getHeaderOrBuilder() {
|
|
return header_;
|
|
}
|
|
|
|
// optional .signal.SqlStatement statement = 2;
|
|
public static final int STATEMENT_FIELD_NUMBER = 2;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement statement_;
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public boolean hasStatement() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement getStatement() {
|
|
return statement_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder getStatementOrBuilder() {
|
|
return statement_;
|
|
}
|
|
|
|
// optional .signal.SharedPreference preference = 3;
|
|
public static final int PREFERENCE_FIELD_NUMBER = 3;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference preference_;
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public boolean hasPreference() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference getPreference() {
|
|
return preference_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder getPreferenceOrBuilder() {
|
|
return preference_;
|
|
}
|
|
|
|
// optional .signal.Attachment attachment = 4;
|
|
public static final int ATTACHMENT_FIELD_NUMBER = 4;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Attachment attachment_;
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public boolean hasAttachment() {
|
|
return ((bitField0_ & 0x00000008) == 0x00000008);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Attachment getAttachment() {
|
|
return attachment_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder getAttachmentOrBuilder() {
|
|
return attachment_;
|
|
}
|
|
|
|
// optional .signal.DatabaseVersion version = 5;
|
|
public static final int VERSION_FIELD_NUMBER = 5;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion version_;
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public boolean hasVersion() {
|
|
return ((bitField0_ & 0x00000010) == 0x00000010);
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion getVersion() {
|
|
return version_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder getVersionOrBuilder() {
|
|
return version_;
|
|
}
|
|
|
|
// optional bool end = 6;
|
|
public static final int END_FIELD_NUMBER = 6;
|
|
private boolean end_;
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
public boolean hasEnd() {
|
|
return ((bitField0_ & 0x00000020) == 0x00000020);
|
|
}
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
public boolean getEnd() {
|
|
return end_;
|
|
}
|
|
|
|
// optional .signal.Avatar avatar = 7;
|
|
public static final int AVATAR_FIELD_NUMBER = 7;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Avatar avatar_;
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public boolean hasAvatar() {
|
|
return ((bitField0_ & 0x00000040) == 0x00000040);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Avatar getAvatar() {
|
|
return avatar_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder getAvatarOrBuilder() {
|
|
return avatar_;
|
|
}
|
|
|
|
// optional .signal.Sticker sticker = 8;
|
|
public static final int STICKER_FIELD_NUMBER = 8;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Sticker sticker_;
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public boolean hasSticker() {
|
|
return ((bitField0_ & 0x00000080) == 0x00000080);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Sticker getSticker() {
|
|
return sticker_;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder getStickerOrBuilder() {
|
|
return sticker_;
|
|
}
|
|
|
|
private void initFields() {
|
|
header_ = org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance();
|
|
statement_ = org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance();
|
|
preference_ = org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance();
|
|
attachment_ = org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance();
|
|
version_ = org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance();
|
|
end_ = false;
|
|
avatar_ = org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance();
|
|
sticker_ = org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance();
|
|
}
|
|
private byte memoizedIsInitialized = -1;
|
|
public final boolean isInitialized() {
|
|
byte isInitialized = memoizedIsInitialized;
|
|
if (isInitialized != -1) return isInitialized == 1;
|
|
|
|
memoizedIsInitialized = 1;
|
|
return true;
|
|
}
|
|
|
|
public void writeTo(com.google.protobuf.CodedOutputStream output)
|
|
throws java.io.IOException {
|
|
getSerializedSize();
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
output.writeMessage(1, header_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
output.writeMessage(2, statement_);
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
output.writeMessage(3, preference_);
|
|
}
|
|
if (((bitField0_ & 0x00000008) == 0x00000008)) {
|
|
output.writeMessage(4, attachment_);
|
|
}
|
|
if (((bitField0_ & 0x00000010) == 0x00000010)) {
|
|
output.writeMessage(5, version_);
|
|
}
|
|
if (((bitField0_ & 0x00000020) == 0x00000020)) {
|
|
output.writeBool(6, end_);
|
|
}
|
|
if (((bitField0_ & 0x00000040) == 0x00000040)) {
|
|
output.writeMessage(7, avatar_);
|
|
}
|
|
if (((bitField0_ & 0x00000080) == 0x00000080)) {
|
|
output.writeMessage(8, sticker_);
|
|
}
|
|
getUnknownFields().writeTo(output);
|
|
}
|
|
|
|
private int memoizedSerializedSize = -1;
|
|
public int getSerializedSize() {
|
|
int size = memoizedSerializedSize;
|
|
if (size != -1) return size;
|
|
|
|
size = 0;
|
|
if (((bitField0_ & 0x00000001) == 0x00000001)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(1, header_);
|
|
}
|
|
if (((bitField0_ & 0x00000002) == 0x00000002)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(2, statement_);
|
|
}
|
|
if (((bitField0_ & 0x00000004) == 0x00000004)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(3, preference_);
|
|
}
|
|
if (((bitField0_ & 0x00000008) == 0x00000008)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(4, attachment_);
|
|
}
|
|
if (((bitField0_ & 0x00000010) == 0x00000010)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(5, version_);
|
|
}
|
|
if (((bitField0_ & 0x00000020) == 0x00000020)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeBoolSize(6, end_);
|
|
}
|
|
if (((bitField0_ & 0x00000040) == 0x00000040)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(7, avatar_);
|
|
}
|
|
if (((bitField0_ & 0x00000080) == 0x00000080)) {
|
|
size += com.google.protobuf.CodedOutputStream
|
|
.computeMessageSize(8, sticker_);
|
|
}
|
|
size += getUnknownFields().getSerializedSize();
|
|
memoizedSerializedSize = size;
|
|
return size;
|
|
}
|
|
|
|
private static final long serialVersionUID = 0L;
|
|
@java.lang.Override
|
|
protected java.lang.Object writeReplace()
|
|
throws java.io.ObjectStreamException {
|
|
return super.writeReplace();
|
|
}
|
|
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(
|
|
com.google.protobuf.ByteString data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(
|
|
com.google.protobuf.ByteString data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(byte[] data)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(
|
|
byte[] data,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws com.google.protobuf.InvalidProtocolBufferException {
|
|
return PARSER.parseFrom(data, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseDelimitedFrom(java.io.InputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseDelimitedFrom(
|
|
java.io.InputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseDelimitedFrom(input, extensionRegistry);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(
|
|
com.google.protobuf.CodedInputStream input)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input);
|
|
}
|
|
public static org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parseFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
return PARSER.parseFrom(input, extensionRegistry);
|
|
}
|
|
|
|
public static Builder newBuilder() { return Builder.create(); }
|
|
public Builder newBuilderForType() { return newBuilder(); }
|
|
public static Builder newBuilder(org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame prototype) {
|
|
return newBuilder().mergeFrom(prototype);
|
|
}
|
|
public Builder toBuilder() { return newBuilder(this); }
|
|
|
|
@java.lang.Override
|
|
protected Builder newBuilderForType(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
Builder builder = new Builder(parent);
|
|
return builder;
|
|
}
|
|
/**
|
|
* Protobuf type {@code signal.BackupFrame}
|
|
*/
|
|
public static final class Builder extends
|
|
com.google.protobuf.GeneratedMessage.Builder<Builder>
|
|
implements org.thoughtcrime.securesms.backup.BackupProtos.BackupFrameOrBuilder {
|
|
public static final com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptor() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_BackupFrame_descriptor;
|
|
}
|
|
|
|
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internalGetFieldAccessorTable() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_BackupFrame_fieldAccessorTable
|
|
.ensureFieldAccessorsInitialized(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.class, org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.Builder.class);
|
|
}
|
|
|
|
// Construct using org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.newBuilder()
|
|
private Builder() {
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
|
|
private Builder(
|
|
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
|
|
super(parent);
|
|
maybeForceBuilderInitialization();
|
|
}
|
|
private void maybeForceBuilderInitialization() {
|
|
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
|
|
getHeaderFieldBuilder();
|
|
getStatementFieldBuilder();
|
|
getPreferenceFieldBuilder();
|
|
getAttachmentFieldBuilder();
|
|
getVersionFieldBuilder();
|
|
getAvatarFieldBuilder();
|
|
getStickerFieldBuilder();
|
|
}
|
|
}
|
|
private static Builder create() {
|
|
return new Builder();
|
|
}
|
|
|
|
public Builder clear() {
|
|
super.clear();
|
|
if (headerBuilder_ == null) {
|
|
header_ = org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance();
|
|
} else {
|
|
headerBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
if (statementBuilder_ == null) {
|
|
statement_ = org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance();
|
|
} else {
|
|
statementBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
if (preferenceBuilder_ == null) {
|
|
preference_ = org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance();
|
|
} else {
|
|
preferenceBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
if (attachmentBuilder_ == null) {
|
|
attachment_ = org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance();
|
|
} else {
|
|
attachmentBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000008);
|
|
if (versionBuilder_ == null) {
|
|
version_ = org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance();
|
|
} else {
|
|
versionBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000010);
|
|
end_ = false;
|
|
bitField0_ = (bitField0_ & ~0x00000020);
|
|
if (avatarBuilder_ == null) {
|
|
avatar_ = org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance();
|
|
} else {
|
|
avatarBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000040);
|
|
if (stickerBuilder_ == null) {
|
|
sticker_ = org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance();
|
|
} else {
|
|
stickerBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000080);
|
|
return this;
|
|
}
|
|
|
|
public Builder clone() {
|
|
return create().mergeFrom(buildPartial());
|
|
}
|
|
|
|
public com.google.protobuf.Descriptors.Descriptor
|
|
getDescriptorForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.internal_static_signal_BackupFrame_descriptor;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame getDefaultInstanceForType() {
|
|
return org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.getDefaultInstance();
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame build() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame result = buildPartial();
|
|
if (!result.isInitialized()) {
|
|
throw newUninitializedMessageException(result);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame buildPartial() {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame result = new org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame(this);
|
|
int from_bitField0_ = bitField0_;
|
|
int to_bitField0_ = 0;
|
|
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
|
|
to_bitField0_ |= 0x00000001;
|
|
}
|
|
if (headerBuilder_ == null) {
|
|
result.header_ = header_;
|
|
} else {
|
|
result.header_ = headerBuilder_.build();
|
|
}
|
|
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
|
|
to_bitField0_ |= 0x00000002;
|
|
}
|
|
if (statementBuilder_ == null) {
|
|
result.statement_ = statement_;
|
|
} else {
|
|
result.statement_ = statementBuilder_.build();
|
|
}
|
|
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
|
|
to_bitField0_ |= 0x00000004;
|
|
}
|
|
if (preferenceBuilder_ == null) {
|
|
result.preference_ = preference_;
|
|
} else {
|
|
result.preference_ = preferenceBuilder_.build();
|
|
}
|
|
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
|
|
to_bitField0_ |= 0x00000008;
|
|
}
|
|
if (attachmentBuilder_ == null) {
|
|
result.attachment_ = attachment_;
|
|
} else {
|
|
result.attachment_ = attachmentBuilder_.build();
|
|
}
|
|
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
|
|
to_bitField0_ |= 0x00000010;
|
|
}
|
|
if (versionBuilder_ == null) {
|
|
result.version_ = version_;
|
|
} else {
|
|
result.version_ = versionBuilder_.build();
|
|
}
|
|
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
|
|
to_bitField0_ |= 0x00000020;
|
|
}
|
|
result.end_ = end_;
|
|
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
|
|
to_bitField0_ |= 0x00000040;
|
|
}
|
|
if (avatarBuilder_ == null) {
|
|
result.avatar_ = avatar_;
|
|
} else {
|
|
result.avatar_ = avatarBuilder_.build();
|
|
}
|
|
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
|
|
to_bitField0_ |= 0x00000080;
|
|
}
|
|
if (stickerBuilder_ == null) {
|
|
result.sticker_ = sticker_;
|
|
} else {
|
|
result.sticker_ = stickerBuilder_.build();
|
|
}
|
|
result.bitField0_ = to_bitField0_;
|
|
onBuilt();
|
|
return result;
|
|
}
|
|
|
|
public Builder mergeFrom(com.google.protobuf.Message other) {
|
|
if (other instanceof org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame) {
|
|
return mergeFrom((org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame)other);
|
|
} else {
|
|
super.mergeFrom(other);
|
|
return this;
|
|
}
|
|
}
|
|
|
|
public Builder mergeFrom(org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame other) {
|
|
if (other == org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame.getDefaultInstance()) return this;
|
|
if (other.hasHeader()) {
|
|
mergeHeader(other.getHeader());
|
|
}
|
|
if (other.hasStatement()) {
|
|
mergeStatement(other.getStatement());
|
|
}
|
|
if (other.hasPreference()) {
|
|
mergePreference(other.getPreference());
|
|
}
|
|
if (other.hasAttachment()) {
|
|
mergeAttachment(other.getAttachment());
|
|
}
|
|
if (other.hasVersion()) {
|
|
mergeVersion(other.getVersion());
|
|
}
|
|
if (other.hasEnd()) {
|
|
setEnd(other.getEnd());
|
|
}
|
|
if (other.hasAvatar()) {
|
|
mergeAvatar(other.getAvatar());
|
|
}
|
|
if (other.hasSticker()) {
|
|
mergeSticker(other.getSticker());
|
|
}
|
|
this.mergeUnknownFields(other.getUnknownFields());
|
|
return this;
|
|
}
|
|
|
|
public final boolean isInitialized() {
|
|
return true;
|
|
}
|
|
|
|
public Builder mergeFrom(
|
|
com.google.protobuf.CodedInputStream input,
|
|
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
|
|
throws java.io.IOException {
|
|
org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame parsedMessage = null;
|
|
try {
|
|
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
|
|
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
|
|
parsedMessage = (org.thoughtcrime.securesms.backup.BackupProtos.BackupFrame) e.getUnfinishedMessage();
|
|
throw e;
|
|
} finally {
|
|
if (parsedMessage != null) {
|
|
mergeFrom(parsedMessage);
|
|
}
|
|
}
|
|
return this;
|
|
}
|
|
private int bitField0_;
|
|
|
|
// optional .signal.Header header = 1;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Header header_ = org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header, org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder, org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder> headerBuilder_;
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public boolean hasHeader() {
|
|
return ((bitField0_ & 0x00000001) == 0x00000001);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Header getHeader() {
|
|
if (headerBuilder_ == null) {
|
|
return header_;
|
|
} else {
|
|
return headerBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public Builder setHeader(org.thoughtcrime.securesms.backup.BackupProtos.Header value) {
|
|
if (headerBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
header_ = value;
|
|
onChanged();
|
|
} else {
|
|
headerBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public Builder setHeader(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder builderForValue) {
|
|
if (headerBuilder_ == null) {
|
|
header_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
headerBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public Builder mergeHeader(org.thoughtcrime.securesms.backup.BackupProtos.Header value) {
|
|
if (headerBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000001) == 0x00000001) &&
|
|
header_ != org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance()) {
|
|
header_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header.newBuilder(header_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
header_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
headerBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000001;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public Builder clearHeader() {
|
|
if (headerBuilder_ == null) {
|
|
header_ = org.thoughtcrime.securesms.backup.BackupProtos.Header.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
headerBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000001);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder getHeaderBuilder() {
|
|
bitField0_ |= 0x00000001;
|
|
onChanged();
|
|
return getHeaderFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder getHeaderOrBuilder() {
|
|
if (headerBuilder_ != null) {
|
|
return headerBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return header_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Header header = 1;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header, org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder, org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder>
|
|
getHeaderFieldBuilder() {
|
|
if (headerBuilder_ == null) {
|
|
headerBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Header, org.thoughtcrime.securesms.backup.BackupProtos.Header.Builder, org.thoughtcrime.securesms.backup.BackupProtos.HeaderOrBuilder>(
|
|
header_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
header_ = null;
|
|
}
|
|
return headerBuilder_;
|
|
}
|
|
|
|
// optional .signal.SqlStatement statement = 2;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement statement_ = org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder> statementBuilder_;
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public boolean hasStatement() {
|
|
return ((bitField0_ & 0x00000002) == 0x00000002);
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement getStatement() {
|
|
if (statementBuilder_ == null) {
|
|
return statement_;
|
|
} else {
|
|
return statementBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public Builder setStatement(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement value) {
|
|
if (statementBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
statement_ = value;
|
|
onChanged();
|
|
} else {
|
|
statementBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public Builder setStatement(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder builderForValue) {
|
|
if (statementBuilder_ == null) {
|
|
statement_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
statementBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public Builder mergeStatement(org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement value) {
|
|
if (statementBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000002) == 0x00000002) &&
|
|
statement_ != org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance()) {
|
|
statement_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.newBuilder(statement_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
statement_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
statementBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000002;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public Builder clearStatement() {
|
|
if (statementBuilder_ == null) {
|
|
statement_ = org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
statementBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000002);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder getStatementBuilder() {
|
|
bitField0_ |= 0x00000002;
|
|
onChanged();
|
|
return getStatementFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder getStatementOrBuilder() {
|
|
if (statementBuilder_ != null) {
|
|
return statementBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return statement_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.SqlStatement statement = 2;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder>
|
|
getStatementFieldBuilder() {
|
|
if (statementBuilder_ == null) {
|
|
statementBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatement.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SqlStatementOrBuilder>(
|
|
statement_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
statement_ = null;
|
|
}
|
|
return statementBuilder_;
|
|
}
|
|
|
|
// optional .signal.SharedPreference preference = 3;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference preference_ = org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder> preferenceBuilder_;
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public boolean hasPreference() {
|
|
return ((bitField0_ & 0x00000004) == 0x00000004);
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference getPreference() {
|
|
if (preferenceBuilder_ == null) {
|
|
return preference_;
|
|
} else {
|
|
return preferenceBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public Builder setPreference(org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference value) {
|
|
if (preferenceBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
preference_ = value;
|
|
onChanged();
|
|
} else {
|
|
preferenceBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000004;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public Builder setPreference(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder builderForValue) {
|
|
if (preferenceBuilder_ == null) {
|
|
preference_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
preferenceBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000004;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public Builder mergePreference(org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference value) {
|
|
if (preferenceBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000004) == 0x00000004) &&
|
|
preference_ != org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance()) {
|
|
preference_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.newBuilder(preference_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
preference_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
preferenceBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000004;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public Builder clearPreference() {
|
|
if (preferenceBuilder_ == null) {
|
|
preference_ = org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
preferenceBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000004);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder getPreferenceBuilder() {
|
|
bitField0_ |= 0x00000004;
|
|
onChanged();
|
|
return getPreferenceFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder getPreferenceOrBuilder() {
|
|
if (preferenceBuilder_ != null) {
|
|
return preferenceBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return preference_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.SharedPreference preference = 3;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder>
|
|
getPreferenceFieldBuilder() {
|
|
if (preferenceBuilder_ == null) {
|
|
preferenceBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreference.Builder, org.thoughtcrime.securesms.backup.BackupProtos.SharedPreferenceOrBuilder>(
|
|
preference_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
preference_ = null;
|
|
}
|
|
return preferenceBuilder_;
|
|
}
|
|
|
|
// optional .signal.Attachment attachment = 4;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Attachment attachment_ = org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment, org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder, org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder> attachmentBuilder_;
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public boolean hasAttachment() {
|
|
return ((bitField0_ & 0x00000008) == 0x00000008);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Attachment getAttachment() {
|
|
if (attachmentBuilder_ == null) {
|
|
return attachment_;
|
|
} else {
|
|
return attachmentBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public Builder setAttachment(org.thoughtcrime.securesms.backup.BackupProtos.Attachment value) {
|
|
if (attachmentBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
attachment_ = value;
|
|
onChanged();
|
|
} else {
|
|
attachmentBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000008;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public Builder setAttachment(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder builderForValue) {
|
|
if (attachmentBuilder_ == null) {
|
|
attachment_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
attachmentBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000008;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public Builder mergeAttachment(org.thoughtcrime.securesms.backup.BackupProtos.Attachment value) {
|
|
if (attachmentBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000008) == 0x00000008) &&
|
|
attachment_ != org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance()) {
|
|
attachment_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment.newBuilder(attachment_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
attachment_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
attachmentBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000008;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public Builder clearAttachment() {
|
|
if (attachmentBuilder_ == null) {
|
|
attachment_ = org.thoughtcrime.securesms.backup.BackupProtos.Attachment.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
attachmentBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000008);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder getAttachmentBuilder() {
|
|
bitField0_ |= 0x00000008;
|
|
onChanged();
|
|
return getAttachmentFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder getAttachmentOrBuilder() {
|
|
if (attachmentBuilder_ != null) {
|
|
return attachmentBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return attachment_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Attachment attachment = 4;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment, org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder, org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder>
|
|
getAttachmentFieldBuilder() {
|
|
if (attachmentBuilder_ == null) {
|
|
attachmentBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Attachment, org.thoughtcrime.securesms.backup.BackupProtos.Attachment.Builder, org.thoughtcrime.securesms.backup.BackupProtos.AttachmentOrBuilder>(
|
|
attachment_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
attachment_ = null;
|
|
}
|
|
return attachmentBuilder_;
|
|
}
|
|
|
|
// optional .signal.DatabaseVersion version = 5;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion version_ = org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder> versionBuilder_;
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public boolean hasVersion() {
|
|
return ((bitField0_ & 0x00000010) == 0x00000010);
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion getVersion() {
|
|
if (versionBuilder_ == null) {
|
|
return version_;
|
|
} else {
|
|
return versionBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public Builder setVersion(org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion value) {
|
|
if (versionBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
version_ = value;
|
|
onChanged();
|
|
} else {
|
|
versionBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000010;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public Builder setVersion(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder builderForValue) {
|
|
if (versionBuilder_ == null) {
|
|
version_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
versionBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000010;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public Builder mergeVersion(org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion value) {
|
|
if (versionBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000010) == 0x00000010) &&
|
|
version_ != org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance()) {
|
|
version_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.newBuilder(version_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
version_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
versionBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000010;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public Builder clearVersion() {
|
|
if (versionBuilder_ == null) {
|
|
version_ = org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
versionBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000010);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder getVersionBuilder() {
|
|
bitField0_ |= 0x00000010;
|
|
onChanged();
|
|
return getVersionFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder getVersionOrBuilder() {
|
|
if (versionBuilder_ != null) {
|
|
return versionBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return version_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.DatabaseVersion version = 5;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder>
|
|
getVersionFieldBuilder() {
|
|
if (versionBuilder_ == null) {
|
|
versionBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersion.Builder, org.thoughtcrime.securesms.backup.BackupProtos.DatabaseVersionOrBuilder>(
|
|
version_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
version_ = null;
|
|
}
|
|
return versionBuilder_;
|
|
}
|
|
|
|
// optional bool end = 6;
|
|
private boolean end_ ;
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
public boolean hasEnd() {
|
|
return ((bitField0_ & 0x00000020) == 0x00000020);
|
|
}
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
public boolean getEnd() {
|
|
return end_;
|
|
}
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
public Builder setEnd(boolean value) {
|
|
bitField0_ |= 0x00000020;
|
|
end_ = value;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional bool end = 6;</code>
|
|
*/
|
|
public Builder clearEnd() {
|
|
bitField0_ = (bitField0_ & ~0x00000020);
|
|
end_ = false;
|
|
onChanged();
|
|
return this;
|
|
}
|
|
|
|
// optional .signal.Avatar avatar = 7;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Avatar avatar_ = org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar, org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder, org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder> avatarBuilder_;
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public boolean hasAvatar() {
|
|
return ((bitField0_ & 0x00000040) == 0x00000040);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Avatar getAvatar() {
|
|
if (avatarBuilder_ == null) {
|
|
return avatar_;
|
|
} else {
|
|
return avatarBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public Builder setAvatar(org.thoughtcrime.securesms.backup.BackupProtos.Avatar value) {
|
|
if (avatarBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
avatar_ = value;
|
|
onChanged();
|
|
} else {
|
|
avatarBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000040;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public Builder setAvatar(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder builderForValue) {
|
|
if (avatarBuilder_ == null) {
|
|
avatar_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
avatarBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000040;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public Builder mergeAvatar(org.thoughtcrime.securesms.backup.BackupProtos.Avatar value) {
|
|
if (avatarBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000040) == 0x00000040) &&
|
|
avatar_ != org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance()) {
|
|
avatar_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar.newBuilder(avatar_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
avatar_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
avatarBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000040;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public Builder clearAvatar() {
|
|
if (avatarBuilder_ == null) {
|
|
avatar_ = org.thoughtcrime.securesms.backup.BackupProtos.Avatar.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
avatarBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000040);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder getAvatarBuilder() {
|
|
bitField0_ |= 0x00000040;
|
|
onChanged();
|
|
return getAvatarFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder getAvatarOrBuilder() {
|
|
if (avatarBuilder_ != null) {
|
|
return avatarBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return avatar_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Avatar avatar = 7;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar, org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder, org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder>
|
|
getAvatarFieldBuilder() {
|
|
if (avatarBuilder_ == null) {
|
|
avatarBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Avatar, org.thoughtcrime.securesms.backup.BackupProtos.Avatar.Builder, org.thoughtcrime.securesms.backup.BackupProtos.AvatarOrBuilder>(
|
|
avatar_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
avatar_ = null;
|
|
}
|
|
return avatarBuilder_;
|
|
}
|
|
|
|
// optional .signal.Sticker sticker = 8;
|
|
private org.thoughtcrime.securesms.backup.BackupProtos.Sticker sticker_ = org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance();
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker, org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder, org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder> stickerBuilder_;
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public boolean hasSticker() {
|
|
return ((bitField0_ & 0x00000080) == 0x00000080);
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Sticker getSticker() {
|
|
if (stickerBuilder_ == null) {
|
|
return sticker_;
|
|
} else {
|
|
return stickerBuilder_.getMessage();
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public Builder setSticker(org.thoughtcrime.securesms.backup.BackupProtos.Sticker value) {
|
|
if (stickerBuilder_ == null) {
|
|
if (value == null) {
|
|
throw new NullPointerException();
|
|
}
|
|
sticker_ = value;
|
|
onChanged();
|
|
} else {
|
|
stickerBuilder_.setMessage(value);
|
|
}
|
|
bitField0_ |= 0x00000080;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public Builder setSticker(
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder builderForValue) {
|
|
if (stickerBuilder_ == null) {
|
|
sticker_ = builderForValue.build();
|
|
onChanged();
|
|
} else {
|
|
stickerBuilder_.setMessage(builderForValue.build());
|
|
}
|
|
bitField0_ |= 0x00000080;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public Builder mergeSticker(org.thoughtcrime.securesms.backup.BackupProtos.Sticker value) {
|
|
if (stickerBuilder_ == null) {
|
|
if (((bitField0_ & 0x00000080) == 0x00000080) &&
|
|
sticker_ != org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance()) {
|
|
sticker_ =
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker.newBuilder(sticker_).mergeFrom(value).buildPartial();
|
|
} else {
|
|
sticker_ = value;
|
|
}
|
|
onChanged();
|
|
} else {
|
|
stickerBuilder_.mergeFrom(value);
|
|
}
|
|
bitField0_ |= 0x00000080;
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public Builder clearSticker() {
|
|
if (stickerBuilder_ == null) {
|
|
sticker_ = org.thoughtcrime.securesms.backup.BackupProtos.Sticker.getDefaultInstance();
|
|
onChanged();
|
|
} else {
|
|
stickerBuilder_.clear();
|
|
}
|
|
bitField0_ = (bitField0_ & ~0x00000080);
|
|
return this;
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder getStickerBuilder() {
|
|
bitField0_ |= 0x00000080;
|
|
onChanged();
|
|
return getStickerFieldBuilder().getBuilder();
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
public org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder getStickerOrBuilder() {
|
|
if (stickerBuilder_ != null) {
|
|
return stickerBuilder_.getMessageOrBuilder();
|
|
} else {
|
|
return sticker_;
|
|
}
|
|
}
|
|
/**
|
|
* <code>optional .signal.Sticker sticker = 8;</code>
|
|
*/
|
|
private com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker, org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder, org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder>
|
|
getStickerFieldBuilder() {
|
|
if (stickerBuilder_ == null) {
|
|
stickerBuilder_ = new com.google.protobuf.SingleFieldBuilder<
|
|
org.thoughtcrime.securesms.backup.BackupProtos.Sticker, org.thoughtcrime.securesms.backup.BackupProtos.Sticker.Builder, org.thoughtcrime.securesms.backup.BackupProtos.StickerOrBuilder>(
|
|
sticker_,
|
|
getParentForChildren(),
|
|
isClean());
|
|
sticker_ = null;
|
|
}
|
|
return stickerBuilder_;
|
|
}
|
|
|
|
// @@protoc_insertion_point(builder_scope:signal.BackupFrame)
|
|
}
|
|
|
|
static {
|
|
defaultInstance = new BackupFrame(true);
|
|
defaultInstance.initFields();
|
|
}
|
|
|
|
// @@protoc_insertion_point(class_scope:signal.BackupFrame)
|
|
}
|
|
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_SqlStatement_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_SqlStatement_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_SqlStatement_SqlParameter_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_SqlStatement_SqlParameter_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_SharedPreference_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_SharedPreference_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_Attachment_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_Attachment_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_Sticker_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_Sticker_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_Avatar_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_Avatar_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_DatabaseVersion_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_DatabaseVersion_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_Header_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_Header_fieldAccessorTable;
|
|
private static com.google.protobuf.Descriptors.Descriptor
|
|
internal_static_signal_BackupFrame_descriptor;
|
|
private static
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable
|
|
internal_static_signal_BackupFrame_fieldAccessorTable;
|
|
|
|
public static com.google.protobuf.Descriptors.FileDescriptor
|
|
getDescriptor() {
|
|
return descriptor;
|
|
}
|
|
private static com.google.protobuf.Descriptors.FileDescriptor
|
|
descriptor;
|
|
static {
|
|
java.lang.String[] descriptorData = {
|
|
"\n\rBackups.proto\022\006signal\"\342\001\n\014SqlStatement" +
|
|
"\022\021\n\tstatement\030\001 \001(\t\0225\n\nparameters\030\002 \003(\0132" +
|
|
"!.signal.SqlStatement.SqlParameter\032\207\001\n\014S" +
|
|
"qlParameter\022\026\n\016stringParamter\030\001 \001(\t\022\030\n\020i" +
|
|
"ntegerParameter\030\002 \001(\004\022\027\n\017doubleParameter" +
|
|
"\030\003 \001(\001\022\025\n\rblobParameter\030\004 \001(\014\022\025\n\rnullpar" +
|
|
"ameter\030\005 \001(\010\"<\n\020SharedPreference\022\014\n\004file" +
|
|
"\030\001 \001(\t\022\013\n\003key\030\002 \001(\t\022\r\n\005value\030\003 \001(\t\"A\n\nAt" +
|
|
"tachment\022\r\n\005rowId\030\001 \001(\004\022\024\n\014attachmentId\030" +
|
|
"\002 \001(\004\022\016\n\006length\030\003 \001(\r\"(\n\007Sticker\022\r\n\005rowI",
|
|
"d\030\001 \001(\004\022\016\n\006length\030\002 \001(\r\"&\n\006Avatar\022\014\n\004nam" +
|
|
"e\030\001 \001(\t\022\016\n\006length\030\002 \001(\r\"\"\n\017DatabaseVersi" +
|
|
"on\022\017\n\007version\030\001 \001(\r\"\"\n\006Header\022\n\n\002iv\030\001 \001(" +
|
|
"\014\022\014\n\004salt\030\002 \001(\014\"\245\002\n\013BackupFrame\022\036\n\006heade" +
|
|
"r\030\001 \001(\0132\016.signal.Header\022\'\n\tstatement\030\002 \001" +
|
|
"(\0132\024.signal.SqlStatement\022,\n\npreference\030\003" +
|
|
" \001(\0132\030.signal.SharedPreference\022&\n\nattach" +
|
|
"ment\030\004 \001(\0132\022.signal.Attachment\022(\n\007versio" +
|
|
"n\030\005 \001(\0132\027.signal.DatabaseVersion\022\013\n\003end\030" +
|
|
"\006 \001(\010\022\036\n\006avatar\030\007 \001(\0132\016.signal.Avatar\022 \n",
|
|
"\007sticker\030\010 \001(\0132\017.signal.StickerB1\n!org.t" +
|
|
"houghtcrime.securesms.backupB\014BackupProt" +
|
|
"os"
|
|
};
|
|
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
|
|
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
|
|
public com.google.protobuf.ExtensionRegistry assignDescriptors(
|
|
com.google.protobuf.Descriptors.FileDescriptor root) {
|
|
descriptor = root;
|
|
internal_static_signal_SqlStatement_descriptor =
|
|
getDescriptor().getMessageTypes().get(0);
|
|
internal_static_signal_SqlStatement_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_SqlStatement_descriptor,
|
|
new java.lang.String[] { "Statement", "Parameters", });
|
|
internal_static_signal_SqlStatement_SqlParameter_descriptor =
|
|
internal_static_signal_SqlStatement_descriptor.getNestedTypes().get(0);
|
|
internal_static_signal_SqlStatement_SqlParameter_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_SqlStatement_SqlParameter_descriptor,
|
|
new java.lang.String[] { "StringParamter", "IntegerParameter", "DoubleParameter", "BlobParameter", "Nullparameter", });
|
|
internal_static_signal_SharedPreference_descriptor =
|
|
getDescriptor().getMessageTypes().get(1);
|
|
internal_static_signal_SharedPreference_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_SharedPreference_descriptor,
|
|
new java.lang.String[] { "File", "Key", "Value", });
|
|
internal_static_signal_Attachment_descriptor =
|
|
getDescriptor().getMessageTypes().get(2);
|
|
internal_static_signal_Attachment_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_Attachment_descriptor,
|
|
new java.lang.String[] { "RowId", "AttachmentId", "Length", });
|
|
internal_static_signal_Sticker_descriptor =
|
|
getDescriptor().getMessageTypes().get(3);
|
|
internal_static_signal_Sticker_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_Sticker_descriptor,
|
|
new java.lang.String[] { "RowId", "Length", });
|
|
internal_static_signal_Avatar_descriptor =
|
|
getDescriptor().getMessageTypes().get(4);
|
|
internal_static_signal_Avatar_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_Avatar_descriptor,
|
|
new java.lang.String[] { "Name", "Length", });
|
|
internal_static_signal_DatabaseVersion_descriptor =
|
|
getDescriptor().getMessageTypes().get(5);
|
|
internal_static_signal_DatabaseVersion_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_DatabaseVersion_descriptor,
|
|
new java.lang.String[] { "Version", });
|
|
internal_static_signal_Header_descriptor =
|
|
getDescriptor().getMessageTypes().get(6);
|
|
internal_static_signal_Header_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_Header_descriptor,
|
|
new java.lang.String[] { "Iv", "Salt", });
|
|
internal_static_signal_BackupFrame_descriptor =
|
|
getDescriptor().getMessageTypes().get(7);
|
|
internal_static_signal_BackupFrame_fieldAccessorTable = new
|
|
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
|
|
internal_static_signal_BackupFrame_descriptor,
|
|
new java.lang.String[] { "Header", "Statement", "Preference", "Attachment", "Version", "End", "Avatar", "Sticker", });
|
|
return null;
|
|
}
|
|
};
|
|
com.google.protobuf.Descriptors.FileDescriptor
|
|
.internalBuildGeneratedFileFrom(descriptorData,
|
|
new com.google.protobuf.Descriptors.FileDescriptor[] {
|
|
}, assigner);
|
|
}
|
|
|
|
// @@protoc_insertion_point(outer_class_scope)
|
|
}
|