mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
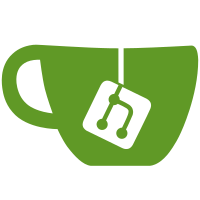
On some platforms (notably macOS and iOS) we look up the default interface to bind outgoing connections to. This is both duplicated work and results in logspam when the default interface is not available (i.e. when a phone has no connectivity, we log an error and thus cause more things that we will try to upload and fail). Fixed by passing around a netmon.Monitor to more places, so that we can use its cached interface state. Fixes #7850 Updates #7621 Signed-off-by: Mihai Parparita <mihai@tailscale.com>
79 lines
2.3 KiB
Go
79 lines
2.3 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Package netns contains the common code for using the Go net package
|
|
// in a logical "network namespace" to avoid routing loops where
|
|
// Tailscale-created packets would otherwise loop back through
|
|
// Tailscale routes.
|
|
//
|
|
// Despite the name netns, the exact mechanism used differs by
|
|
// operating system, and perhaps even by version of the OS.
|
|
//
|
|
// The netns package also handles connecting via SOCKS proxies when
|
|
// configured by the environment.
|
|
package netns
|
|
|
|
import (
|
|
"flag"
|
|
"testing"
|
|
)
|
|
|
|
var extNetwork = flag.Bool("use-external-network", false, "use the external network in tests")
|
|
|
|
func TestDial(t *testing.T) {
|
|
if !*extNetwork {
|
|
t.Skip("skipping test without --use-external-network")
|
|
}
|
|
d := NewDialer(t.Logf, nil)
|
|
c, err := d.Dial("tcp", "google.com:80")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
defer c.Close()
|
|
t.Logf("got addr %v", c.RemoteAddr())
|
|
|
|
c, err = d.Dial("tcp4", "google.com:80")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
defer c.Close()
|
|
t.Logf("got addr %v", c.RemoteAddr())
|
|
}
|
|
|
|
func TestIsLocalhost(t *testing.T) {
|
|
tests := []struct {
|
|
name string
|
|
host string
|
|
want bool
|
|
}{
|
|
{"IPv4 loopback", "127.0.0.1", true},
|
|
{"IPv4 !loopback", "192.168.0.1", false},
|
|
{"IPv4 loopback with port", "127.0.0.1:1", true},
|
|
{"IPv4 !loopback with port", "192.168.0.1:1", false},
|
|
{"IPv4 unspecified", "0.0.0.0", false},
|
|
{"IPv4 unspecified with port", "0.0.0.0:1", false},
|
|
{"IPv6 loopback", "::1", true},
|
|
{"IPv6 !loopback", "2001:4860:4860::8888", false},
|
|
{"IPv6 loopback with port", "[::1]:1", true},
|
|
{"IPv6 !loopback with port", "[2001:4860:4860::8888]:1", false},
|
|
{"IPv6 unspecified", "::", false},
|
|
{"IPv6 unspecified with port", "[::]:1", false},
|
|
{"empty", "", false},
|
|
{"hostname", "example.com", false},
|
|
{"localhost", "localhost", true},
|
|
{"localhost6", "localhost6", true},
|
|
{"localhost with port", "localhost:1", true},
|
|
{"localhost6 with port", "localhost6:1", true},
|
|
{"ip6-localhost", "ip6-localhost", true},
|
|
{"ip6-localhost with port", "ip6-localhost:1", true},
|
|
{"ip6-loopback", "ip6-loopback", true},
|
|
{"ip6-loopback with port", "ip6-loopback:1", true},
|
|
}
|
|
|
|
for _, test := range tests {
|
|
if got := isLocalhost(test.host); got != test.want {
|
|
t.Errorf("isLocalhost(%q) = %v, want %v", test.name, got, test.want)
|
|
}
|
|
}
|
|
}
|