mirror of
https://github.com/tailscale/tailscale.git
synced 2025-04-03 23:05:50 +00:00
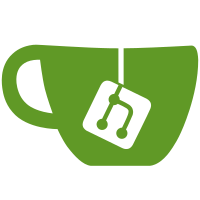
This PR adds some custom logic for reading and writing kube store values that are TLS certs and keys: 1) when store is initialized, lookup additional TLS Secrets for this node and if found, load TLS certs from there 2) if the node runs in certs 'read only' mode and TLS cert and key are not found in the in-memory store, look those up in a Secret 3) if the node runs in certs 'read only' mode, run a daily TLS certs reload to memory to get any renewed certs Updates tailscale/corp#24795 Signed-off-by: Irbe Krumina <irbe@tailscale.com>
55 lines
2.0 KiB
Go
55 lines
2.0 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
package kubeclient
|
|
|
|
import (
|
|
"context"
|
|
"net"
|
|
|
|
"tailscale.com/kube/kubeapi"
|
|
)
|
|
|
|
var _ Client = &FakeClient{}
|
|
|
|
type FakeClient struct {
|
|
GetSecretImpl func(context.Context, string) (*kubeapi.Secret, error)
|
|
CheckSecretPermissionsImpl func(ctx context.Context, name string) (bool, bool, error)
|
|
CreateSecretImpl func(context.Context, *kubeapi.Secret) error
|
|
UpdateSecretImpl func(context.Context, *kubeapi.Secret) error
|
|
JSONPatchResourceImpl func(context.Context, string, string, []JSONPatch) error
|
|
ListSecretsImpl func(context.Context, map[string]string) (*kubeapi.SecretList, error)
|
|
}
|
|
|
|
func (fc *FakeClient) CheckSecretPermissions(ctx context.Context, name string) (bool, bool, error) {
|
|
return fc.CheckSecretPermissionsImpl(ctx, name)
|
|
}
|
|
func (fc *FakeClient) GetSecret(ctx context.Context, name string) (*kubeapi.Secret, error) {
|
|
return fc.GetSecretImpl(ctx, name)
|
|
}
|
|
func (fc *FakeClient) SetURL(_ string) {}
|
|
func (fc *FakeClient) SetDialer(dialer func(ctx context.Context, network, addr string) (net.Conn, error)) {
|
|
}
|
|
func (fc *FakeClient) StrategicMergePatchSecret(context.Context, string, *kubeapi.Secret, string) error {
|
|
return nil
|
|
}
|
|
func (fc *FakeClient) Event(context.Context, string, string, string) error {
|
|
return nil
|
|
}
|
|
|
|
func (fc *FakeClient) JSONPatchResource(ctx context.Context, resource, name string, patches []JSONPatch) error {
|
|
return fc.JSONPatchResourceImpl(ctx, resource, name, patches)
|
|
}
|
|
func (fc *FakeClient) UpdateSecret(ctx context.Context, secret *kubeapi.Secret) error {
|
|
return fc.UpdateSecretImpl(ctx, secret)
|
|
}
|
|
func (fc *FakeClient) CreateSecret(ctx context.Context, secret *kubeapi.Secret) error {
|
|
return fc.CreateSecretImpl(ctx, secret)
|
|
}
|
|
func (fc *FakeClient) ListSecrets(ctx context.Context, selector map[string]string) (*kubeapi.SecretList, error) {
|
|
if fc.ListSecretsImpl != nil {
|
|
return fc.ListSecretsImpl(ctx, selector)
|
|
}
|
|
return nil, nil
|
|
}
|