mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 11:35:35 +00:00
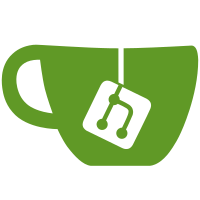
Adds a new TailscaleProxyReady condition type for use in corev1.Service conditions. Also switch our CRDs to use metav1.Condition instead of ConnectorCondition. The Go structs are seralized identically, but it updates some descriptions and validation rules. Update k8s controller-tools and controller-runtime deps to fix the documentation generation for metav1.Condition so that it excludes comments and TODOs. Stop expecting the fake client to populate TypeMeta in tests. See kubernetes-sigs/controller-runtime#2633 for details of the change. Finally, make some minor improvements to validation for service hostnames. Fixes #12216 Co-authored-by: Irbe Krumina <irbe@tailscale.com> Signed-off-by: Tom Proctor <tomhjp@users.noreply.github.com>
624 lines
17 KiB
Go
624 lines
17 KiB
Go
//go:build !ignore_autogenerated && !plan9
|
|
|
|
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Code generated by controller-gen. DO NOT EDIT.
|
|
|
|
package v1alpha1
|
|
|
|
import (
|
|
corev1 "k8s.io/api/core/v1"
|
|
"k8s.io/apimachinery/pkg/apis/meta/v1"
|
|
"k8s.io/apimachinery/pkg/runtime"
|
|
)
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Connector) DeepCopyInto(out *Connector) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Connector.
|
|
func (in *Connector) DeepCopy() *Connector {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Connector)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *Connector) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ConnectorList) DeepCopyInto(out *ConnectorList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]Connector, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ConnectorList.
|
|
func (in *ConnectorList) DeepCopy() *ConnectorList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ConnectorList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ConnectorList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ConnectorSpec) DeepCopyInto(out *ConnectorSpec) {
|
|
*out = *in
|
|
if in.Tags != nil {
|
|
in, out := &in.Tags, &out.Tags
|
|
*out = make(Tags, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
if in.SubnetRouter != nil {
|
|
in, out := &in.SubnetRouter, &out.SubnetRouter
|
|
*out = new(SubnetRouter)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ConnectorSpec.
|
|
func (in *ConnectorSpec) DeepCopy() *ConnectorSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ConnectorSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ConnectorStatus) DeepCopyInto(out *ConnectorStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
if in.TailnetIPs != nil {
|
|
in, out := &in.TailnetIPs, &out.TailnetIPs
|
|
*out = make([]string, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ConnectorStatus.
|
|
func (in *ConnectorStatus) DeepCopy() *ConnectorStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ConnectorStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Container) DeepCopyInto(out *Container) {
|
|
*out = *in
|
|
if in.Env != nil {
|
|
in, out := &in.Env, &out.Env
|
|
*out = make([]Env, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
in.Resources.DeepCopyInto(&out.Resources)
|
|
if in.SecurityContext != nil {
|
|
in, out := &in.SecurityContext, &out.SecurityContext
|
|
*out = new(corev1.SecurityContext)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Container.
|
|
func (in *Container) DeepCopy() *Container {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Container)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfig) DeepCopyInto(out *DNSConfig) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfig.
|
|
func (in *DNSConfig) DeepCopy() *DNSConfig {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfig)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *DNSConfig) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfigList) DeepCopyInto(out *DNSConfigList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]DNSConfig, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfigList.
|
|
func (in *DNSConfigList) DeepCopy() *DNSConfigList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfigList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *DNSConfigList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfigSpec) DeepCopyInto(out *DNSConfigSpec) {
|
|
*out = *in
|
|
if in.Nameserver != nil {
|
|
in, out := &in.Nameserver, &out.Nameserver
|
|
*out = new(Nameserver)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfigSpec.
|
|
func (in *DNSConfigSpec) DeepCopy() *DNSConfigSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfigSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfigStatus) DeepCopyInto(out *DNSConfigStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
if in.Nameserver != nil {
|
|
in, out := &in.Nameserver, &out.Nameserver
|
|
*out = new(NameserverStatus)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfigStatus.
|
|
func (in *DNSConfigStatus) DeepCopy() *DNSConfigStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfigStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Env) DeepCopyInto(out *Env) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Env.
|
|
func (in *Env) DeepCopy() *Env {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Env)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Image) DeepCopyInto(out *Image) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Image.
|
|
func (in *Image) DeepCopy() *Image {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Image)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Metrics) DeepCopyInto(out *Metrics) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Metrics.
|
|
func (in *Metrics) DeepCopy() *Metrics {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Metrics)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Nameserver) DeepCopyInto(out *Nameserver) {
|
|
*out = *in
|
|
if in.Image != nil {
|
|
in, out := &in.Image, &out.Image
|
|
*out = new(Image)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Nameserver.
|
|
func (in *Nameserver) DeepCopy() *Nameserver {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Nameserver)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *NameserverStatus) DeepCopyInto(out *NameserverStatus) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new NameserverStatus.
|
|
func (in *NameserverStatus) DeepCopy() *NameserverStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(NameserverStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Pod) DeepCopyInto(out *Pod) {
|
|
*out = *in
|
|
if in.Labels != nil {
|
|
in, out := &in.Labels, &out.Labels
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Annotations != nil {
|
|
in, out := &in.Annotations, &out.Annotations
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Affinity != nil {
|
|
in, out := &in.Affinity, &out.Affinity
|
|
*out = new(corev1.Affinity)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.TailscaleContainer != nil {
|
|
in, out := &in.TailscaleContainer, &out.TailscaleContainer
|
|
*out = new(Container)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.TailscaleInitContainer != nil {
|
|
in, out := &in.TailscaleInitContainer, &out.TailscaleInitContainer
|
|
*out = new(Container)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.SecurityContext != nil {
|
|
in, out := &in.SecurityContext, &out.SecurityContext
|
|
*out = new(corev1.PodSecurityContext)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.ImagePullSecrets != nil {
|
|
in, out := &in.ImagePullSecrets, &out.ImagePullSecrets
|
|
*out = make([]corev1.LocalObjectReference, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
if in.NodeSelector != nil {
|
|
in, out := &in.NodeSelector, &out.NodeSelector
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Tolerations != nil {
|
|
in, out := &in.Tolerations, &out.Tolerations
|
|
*out = make([]corev1.Toleration, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Pod.
|
|
func (in *Pod) DeepCopy() *Pod {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Pod)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClass) DeepCopyInto(out *ProxyClass) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClass.
|
|
func (in *ProxyClass) DeepCopy() *ProxyClass {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClass)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ProxyClass) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClassList) DeepCopyInto(out *ProxyClassList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]ProxyClass, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClassList.
|
|
func (in *ProxyClassList) DeepCopy() *ProxyClassList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClassList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ProxyClassList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClassSpec) DeepCopyInto(out *ProxyClassSpec) {
|
|
*out = *in
|
|
if in.StatefulSet != nil {
|
|
in, out := &in.StatefulSet, &out.StatefulSet
|
|
*out = new(StatefulSet)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.Metrics != nil {
|
|
in, out := &in.Metrics, &out.Metrics
|
|
*out = new(Metrics)
|
|
**out = **in
|
|
}
|
|
if in.TailscaleConfig != nil {
|
|
in, out := &in.TailscaleConfig, &out.TailscaleConfig
|
|
*out = new(TailscaleConfig)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClassSpec.
|
|
func (in *ProxyClassSpec) DeepCopy() *ProxyClassSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClassSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClassStatus) DeepCopyInto(out *ProxyClassStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClassStatus.
|
|
func (in *ProxyClassStatus) DeepCopy() *ProxyClassStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClassStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in Routes) DeepCopyInto(out *Routes) {
|
|
{
|
|
in := &in
|
|
*out = make(Routes, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Routes.
|
|
func (in Routes) DeepCopy() Routes {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Routes)
|
|
in.DeepCopyInto(out)
|
|
return *out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *StatefulSet) DeepCopyInto(out *StatefulSet) {
|
|
*out = *in
|
|
if in.Labels != nil {
|
|
in, out := &in.Labels, &out.Labels
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Annotations != nil {
|
|
in, out := &in.Annotations, &out.Annotations
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Pod != nil {
|
|
in, out := &in.Pod, &out.Pod
|
|
*out = new(Pod)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new StatefulSet.
|
|
func (in *StatefulSet) DeepCopy() *StatefulSet {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(StatefulSet)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *SubnetRouter) DeepCopyInto(out *SubnetRouter) {
|
|
*out = *in
|
|
if in.AdvertiseRoutes != nil {
|
|
in, out := &in.AdvertiseRoutes, &out.AdvertiseRoutes
|
|
*out = make(Routes, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new SubnetRouter.
|
|
func (in *SubnetRouter) DeepCopy() *SubnetRouter {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(SubnetRouter)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in Tags) DeepCopyInto(out *Tags) {
|
|
{
|
|
in := &in
|
|
*out = make(Tags, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Tags.
|
|
func (in Tags) DeepCopy() Tags {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Tags)
|
|
in.DeepCopyInto(out)
|
|
return *out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *TailscaleConfig) DeepCopyInto(out *TailscaleConfig) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new TailscaleConfig.
|
|
func (in *TailscaleConfig) DeepCopy() *TailscaleConfig {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(TailscaleConfig)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|