mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 11:35:35 +00:00
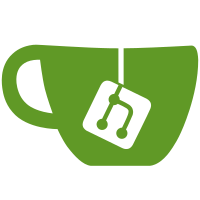
In rare circumstances (tailscale/corp#3016), the PublicKey and Endpoints can diverge. This by itself doesn't cause any harm, but our early exit in response did, because it prevented us from recovering from it. Remove the early exit. Signed-off-by: Josh Bleecher Snyder <josh@tailscale.com>
62 lines
1.7 KiB
Go
62 lines
1.7 KiB
Go
// Copyright (c) 2020 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Code generated by tailscale.com/cmd/cloner; DO NOT EDIT.
|
|
//go:generate go run tailscale.com/cmd/cloner -type=Config,Peer -output=clone.go
|
|
|
|
package wgcfg
|
|
|
|
import (
|
|
"inet.af/netaddr"
|
|
"tailscale.com/types/key"
|
|
)
|
|
|
|
// Clone makes a deep copy of Config.
|
|
// The result aliases no memory with the original.
|
|
func (src *Config) Clone() *Config {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Config)
|
|
*dst = *src
|
|
dst.Addresses = append(src.Addresses[:0:0], src.Addresses...)
|
|
dst.DNS = append(src.DNS[:0:0], src.DNS...)
|
|
dst.Peers = make([]Peer, len(src.Peers))
|
|
for i := range dst.Peers {
|
|
dst.Peers[i] = *src.Peers[i].Clone()
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _ConfigCloneNeedsRegeneration = Config(struct {
|
|
Name string
|
|
PrivateKey key.NodePrivate
|
|
Addresses []netaddr.IPPrefix
|
|
MTU uint16
|
|
DNS []netaddr.IP
|
|
Peers []Peer
|
|
}{})
|
|
|
|
// Clone makes a deep copy of Peer.
|
|
// The result aliases no memory with the original.
|
|
func (src *Peer) Clone() *Peer {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Peer)
|
|
*dst = *src
|
|
dst.AllowedIPs = append(src.AllowedIPs[:0:0], src.AllowedIPs...)
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _PeerCloneNeedsRegeneration = Peer(struct {
|
|
PublicKey key.NodePublic
|
|
DiscoKey key.DiscoPublic
|
|
AllowedIPs []netaddr.IPPrefix
|
|
PersistentKeepalive uint16
|
|
WGEndpoint key.NodePublic
|
|
}{})
|