mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
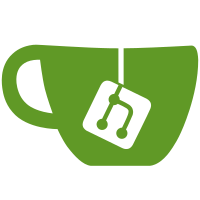
The remove hook implementation was copy/pasted from the line above and I didn't change the body, resulting in packet forwarding routes never being removed. Fortunately we weren't using this path yet, but it led to stats being off, and (very) slow memory growth.
46 lines
1.1 KiB
Go
46 lines
1.1 KiB
Go
// Copyright (c) 2020 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"log"
|
|
"strings"
|
|
|
|
"tailscale.com/derp"
|
|
"tailscale.com/derp/derphttp"
|
|
"tailscale.com/types/key"
|
|
"tailscale.com/types/logger"
|
|
)
|
|
|
|
func startMesh(s *derp.Server) error {
|
|
if *meshWith == "" {
|
|
return nil
|
|
}
|
|
if !s.HasMeshKey() {
|
|
return errors.New("--mesh-with requires --mesh-psk-file")
|
|
}
|
|
for _, host := range strings.Split(*meshWith, ",") {
|
|
if err := startMeshWithHost(s, host); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func startMeshWithHost(s *derp.Server, host string) error {
|
|
logf := logger.WithPrefix(log.Printf, fmt.Sprintf("mesh(%q): ", host))
|
|
c, err := derphttp.NewClient(s.PrivateKey(), "https://"+host+"/derp", logf)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
c.MeshKey = s.MeshKey()
|
|
add := func(k key.Public) { s.AddPacketForwarder(k, c) }
|
|
remove := func(k key.Public) { s.RemovePacketForwarder(k, c) }
|
|
go c.RunWatchConnectionLoop(s.PublicKey(), add, remove)
|
|
return nil
|
|
}
|