mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
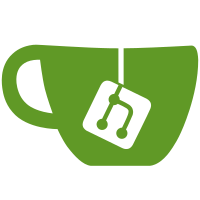
The docs say: Note that while correct uses of TryLock do exist, they are rare, and use of TryLock is often a sign of a deeper problem in a particular use of mutexes. Rare code! Or bad code! Who can tell! Signed-off-by: Josh Bleecher Snyder <josh@tailscale.com>
34 lines
698 B
Go
34 lines
698 B
Go
// Copyright (c) 2020 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package syncs
|
|
|
|
import (
|
|
"sync"
|
|
)
|
|
|
|
// AssertLocked panics if m is not locked.
|
|
func AssertLocked(m *sync.Mutex) {
|
|
if m.TryLock() {
|
|
m.Unlock()
|
|
panic("mutex is not locked")
|
|
}
|
|
}
|
|
|
|
// AssertRLocked panics if rw is not locked for reading or writing.
|
|
func AssertRLocked(rw *sync.RWMutex) {
|
|
if rw.TryLock() {
|
|
rw.Unlock()
|
|
panic("mutex is not locked")
|
|
}
|
|
}
|
|
|
|
// AssertWLocked panics if rw is not locked for writing.
|
|
func AssertWLocked(rw *sync.RWMutex) {
|
|
if rw.TryRLock() {
|
|
rw.RUnlock()
|
|
panic("mutex is not rlocked")
|
|
}
|
|
}
|