mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 11:35:35 +00:00
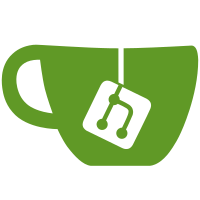
Instead of modeling remote WebDAV servers as actual webdav.FS instances, we now just proxy traffic to them. This not only simplifies the code, but it also allows WebDAV locking to work correctly by making sure locks are handled by the servers that need to (i.e. the ones actually serving the files). Updates tailscale/corp#16827 Signed-off-by: Percy Wegmann <percy@tailscale.com>
51 lines
1.3 KiB
Go
51 lines
1.3 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
package shared
|
|
|
|
import (
|
|
"path"
|
|
"strings"
|
|
)
|
|
|
|
// This file provides utility functions for working with URL paths. These are
|
|
// similar to functions in package path in the standard library, but differ in
|
|
// ways that are documented on the relevant functions.
|
|
|
|
const (
|
|
sepString = "/"
|
|
sepStringAndDot = "/."
|
|
sep = '/'
|
|
)
|
|
|
|
// CleanAndSplit cleans the provided path p and splits it into its constituent
|
|
// parts. This is different from path.Split which just splits a path into prefix
|
|
// and suffix.
|
|
func CleanAndSplit(p string) []string {
|
|
return strings.Split(strings.Trim(path.Clean(p), sepStringAndDot), sepString)
|
|
}
|
|
|
|
// Join behaves like path.Join() but also includes a leading slash.
|
|
func Join(parts ...string) string {
|
|
fullParts := make([]string, 0, len(parts))
|
|
fullParts = append(fullParts, sepString)
|
|
for _, part := range parts {
|
|
fullParts = append(fullParts, part)
|
|
}
|
|
return path.Join(fullParts...)
|
|
}
|
|
|
|
// IsRoot determines whether a given path p is the root path, defined as either
|
|
// empty or "/".
|
|
func IsRoot(p string) bool {
|
|
return p == "" || p == sepString
|
|
}
|
|
|
|
// Base is like path.Base except that it returns "" for the root folder
|
|
func Base(p string) string {
|
|
if IsRoot(p) {
|
|
return ""
|
|
}
|
|
return path.Base(p)
|
|
}
|