mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 11:35:35 +00:00
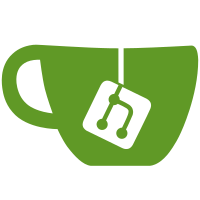
If the user's running "go test" by hand, no need to spam stderr with the sentinel marker. It already calls t.Logf (which only gets output on actual failure, or verbose mode) which is enough to tell users it's known flaky. Stderr OTOH always prints out and is distracting to manual "go test" users. Updates #cleanup Change-Id: Ie5e6881bae291787c30f75924fa132f4a28abbb2 Signed-off-by: Brad Fitzpatrick <bradfitz@tailscale.com>
45 lines
1.7 KiB
Go
45 lines
1.7 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Package flakytest contains test helpers for marking a test as flaky. For
|
|
// tests run using cmd/testwrapper, a failed flaky test will cause tests to be
|
|
// re-run a few time until they succeed or exceed our iteration limit.
|
|
package flakytest
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"regexp"
|
|
"testing"
|
|
)
|
|
|
|
// FlakyTestLogMessage is a sentinel value that is printed to stderr when a
|
|
// flaky test is marked. This is used by cmd/testwrapper to detect flaky tests
|
|
// and retry them.
|
|
const FlakyTestLogMessage = "flakytest: this is a known flaky test"
|
|
|
|
// FlakeAttemptEnv is an environment variable that is set by cmd/testwrapper
|
|
// when a flaky test is being (re)tried. It contains the attempt number,
|
|
// starting at 1.
|
|
const FlakeAttemptEnv = "TS_TESTWRAPPER_ATTEMPT"
|
|
|
|
var issueRegexp = regexp.MustCompile(`\Ahttps://github\.com/tailscale/[a-zA-Z0-9_.-]+/issues/\d+\z`)
|
|
|
|
// Mark sets the current test as a flaky test, such that if it fails, it will
|
|
// be retried a few times on failure. issue must be a GitHub issue that tracks
|
|
// the status of the flaky test being marked, of the format:
|
|
//
|
|
// https://github.com/tailscale/myRepo-H3re/issues/12345
|
|
func Mark(t testing.TB, issue string) {
|
|
if !issueRegexp.MatchString(issue) {
|
|
t.Fatalf("bad issue format: %q", issue)
|
|
}
|
|
if _, ok := os.LookupEnv(FlakeAttemptEnv); ok {
|
|
// We're being run under cmd/testwrapper so send our sentinel message
|
|
// to stderr. (We avoid doing this when the env is absent to avoid
|
|
// spamming people running tests without the wrapper)
|
|
fmt.Fprintln(os.Stderr, FlakyTestLogMessage)
|
|
}
|
|
t.Logf("flakytest: issue tracking this flaky test: %s", issue)
|
|
}
|