mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 19:45:35 +00:00
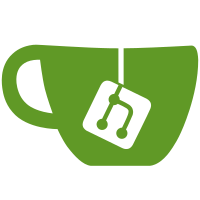
This updates all source files to use a new standard header for copyright and license declaration. Notably, copyright no longer includes a date, and we now use the standard SPDX-License-Identifier header. This commit was done almost entirely mechanically with perl, and then some minimal manual fixes. Updates #6865 Signed-off-by: Will Norris <will@tailscale.com>
63 lines
1.3 KiB
Go
63 lines
1.3 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// testwrapper is a wrapper for retrying flaky tests, using the -exec flag of
|
|
// 'go test'. Tests that are flaky can use the 'flakytest' subpackage to mark
|
|
// themselves as flaky and be retried on failure.
|
|
package main
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"log"
|
|
"os"
|
|
"os/exec"
|
|
)
|
|
|
|
const (
|
|
retryStatus = 123
|
|
maxIterations = 3
|
|
)
|
|
|
|
func main() {
|
|
ctx := context.Background()
|
|
debug := os.Getenv("TS_TESTWRAPPER_DEBUG") != ""
|
|
|
|
log.SetPrefix("testwrapper: ")
|
|
if !debug {
|
|
log.SetFlags(0)
|
|
}
|
|
|
|
for i := 1; i <= maxIterations; i++ {
|
|
if i > 1 {
|
|
log.Printf("retrying flaky tests (%d of %d)", i, maxIterations)
|
|
}
|
|
cmd := exec.CommandContext(ctx, os.Args[1], os.Args[2:]...)
|
|
cmd.Stdout = os.Stdout
|
|
cmd.Stderr = os.Stderr
|
|
cmd.Env = append(os.Environ(), "TS_IN_TESTWRAPPER=1")
|
|
err := cmd.Run()
|
|
if err == nil {
|
|
return
|
|
}
|
|
|
|
var exitErr *exec.ExitError
|
|
if !errors.As(err, &exitErr) {
|
|
if debug {
|
|
log.Printf("error isn't an ExitError")
|
|
}
|
|
os.Exit(1)
|
|
}
|
|
|
|
if code := exitErr.ExitCode(); code != retryStatus {
|
|
if debug {
|
|
log.Printf("code (%d) != retryStatus (%d)", code, retryStatus)
|
|
}
|
|
os.Exit(code)
|
|
}
|
|
}
|
|
|
|
log.Printf("test did not pass in %d iterations", maxIterations)
|
|
os.Exit(1)
|
|
}
|