mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
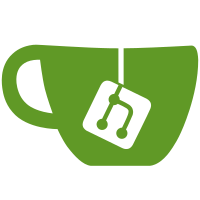
Calling both mono.Now() and time.Now() is slow and leads to unnecessary precision errors. Instead, directly compute mono.Time relative to baseMono and baseWall. This is the opposite calculation as mono.Time.WallTime. Updates tailscale/corp#8427 Signed-off-by: Joe Tsai <joetsai@digital-static.net>
64 lines
1.2 KiB
Go
64 lines
1.2 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
package mono
|
|
|
|
import (
|
|
"encoding/json"
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestNow(t *testing.T) {
|
|
start := Now()
|
|
time.Sleep(100 * time.Millisecond)
|
|
if elapsed := Since(start); elapsed < 100*time.Millisecond {
|
|
t.Errorf("short sleep: %v elapsed, want min %v", elapsed, 100*time.Millisecond)
|
|
}
|
|
}
|
|
|
|
func TestUnmarshalZero(t *testing.T) {
|
|
var tt time.Time
|
|
buf, err := json.Marshal(tt)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
var m Time
|
|
err = json.Unmarshal(buf, &m)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if !m.IsZero() {
|
|
t.Errorf("expected unmarshal of zero time to be 0, got %d (~=%v)", m, m)
|
|
}
|
|
}
|
|
|
|
func TestJSONRoundtrip(t *testing.T) {
|
|
want := Now()
|
|
b, err := want.MarshalJSON()
|
|
if err != nil {
|
|
t.Errorf("MarshalJSON error: %v", err)
|
|
}
|
|
var got Time
|
|
if err := got.UnmarshalJSON(b); err != nil {
|
|
t.Errorf("UnmarshalJSON error: %v", err)
|
|
}
|
|
if got != want {
|
|
t.Errorf("got %v, want %v", got, want)
|
|
}
|
|
}
|
|
|
|
func BenchmarkMonoNow(b *testing.B) {
|
|
b.ReportAllocs()
|
|
for i := 0; i < b.N; i++ {
|
|
Now()
|
|
}
|
|
}
|
|
|
|
func BenchmarkTimeNow(b *testing.B) {
|
|
b.ReportAllocs()
|
|
for i := 0; i < b.N; i++ {
|
|
time.Now()
|
|
}
|
|
}
|