mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
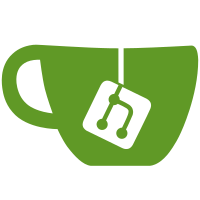
This way we can do that once (out of band, in the GitHub action), instead of increasing the time of each deploy that uses the package. .wasm is removed from the list of automatically pre-compressed extensions, an OSS bump and small change on the corp side is needed to make use of this change. Signed-off-by: Mihai Parparita <mihai@tailscale.com>
87 lines
2.2 KiB
Go
87 lines
2.2 KiB
Go
// Copyright (c) 2022 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package main
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"log"
|
|
"os"
|
|
"path"
|
|
|
|
"github.com/tailscale/hujson"
|
|
"tailscale.com/util/precompress"
|
|
"tailscale.com/version"
|
|
)
|
|
|
|
func runBuildPkg() {
|
|
buildOptions, err := commonPkgSetup(prodMode)
|
|
if err != nil {
|
|
log.Fatalf("Cannot setup: %v", err)
|
|
}
|
|
|
|
log.Printf("Linting...\n")
|
|
if err := runYarn("lint"); err != nil {
|
|
log.Fatalf("Linting failed: %v", err)
|
|
}
|
|
|
|
if err := cleanDir(*pkgDir, "package.json"); err != nil {
|
|
log.Fatalf("Cannot clean %s: %v", *pkgDir, err)
|
|
}
|
|
|
|
buildOptions.Write = true
|
|
buildOptions.MinifyWhitespace = true
|
|
buildOptions.MinifyIdentifiers = true
|
|
buildOptions.MinifySyntax = true
|
|
|
|
runEsbuild(*buildOptions)
|
|
|
|
if err := precompressWasm(); err != nil {
|
|
log.Fatalf("Could not pre-recompress wasm: %v", err)
|
|
}
|
|
|
|
log.Printf("Generating types...\n")
|
|
if err := runYarn("pkg-types"); err != nil {
|
|
log.Fatalf("Type generation failed: %v", err)
|
|
}
|
|
|
|
if err := updateVersion(); err != nil {
|
|
log.Fatalf("Cannot update version: %v", err)
|
|
}
|
|
|
|
log.Printf("Built package version %s", version.Long)
|
|
}
|
|
|
|
func precompressWasm() error {
|
|
log.Printf("Pre-compressing main.wasm...\n")
|
|
return precompress.Precompress(path.Join(*pkgDir, "main.wasm"), precompress.Options{
|
|
FastCompression: *fastCompression,
|
|
})
|
|
}
|
|
|
|
func updateVersion() error {
|
|
packageJSONBytes, err := os.ReadFile("package.json.tmpl")
|
|
if err != nil {
|
|
return fmt.Errorf("Could not read package.json: %w", err)
|
|
}
|
|
|
|
var packageJSON map[string]any
|
|
packageJSONBytes, err = hujson.Standardize(packageJSONBytes)
|
|
if err != nil {
|
|
return fmt.Errorf("Could not standardize template package.json: %w", err)
|
|
}
|
|
if err := json.Unmarshal(packageJSONBytes, &packageJSON); err != nil {
|
|
return fmt.Errorf("Could not unmarshal package.json: %w", err)
|
|
}
|
|
packageJSON["version"] = version.Long
|
|
|
|
packageJSONBytes, err = json.MarshalIndent(packageJSON, "", " ")
|
|
if err != nil {
|
|
return fmt.Errorf("Could not marshal package.json: %w", err)
|
|
}
|
|
|
|
return os.WriteFile(path.Join(*pkgDir, "package.json"), packageJSONBytes, 0644)
|
|
}
|