mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 11:35:35 +00:00
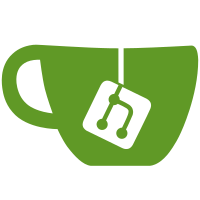
This adds support to make exit nodes and subnet routers work when in scenarios where NAT is required. It also updates the NATConfig to be generated from a `wgcfg.Config` as that handles merging prefs with the netmap, so it has the required information about whether an exit node is already configured and whether routes are accepted. Updates tailscale/corp#8020 Signed-off-by: Maisem Ali <maisem@tailscale.com>
69 lines
1.7 KiB
Go
69 lines
1.7 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Code generated by tailscale.com/cmd/cloner; DO NOT EDIT.
|
|
|
|
package wgcfg
|
|
|
|
import (
|
|
"net/netip"
|
|
|
|
"tailscale.com/tailcfg"
|
|
"tailscale.com/types/key"
|
|
"tailscale.com/types/logid"
|
|
)
|
|
|
|
// Clone makes a deep copy of Config.
|
|
// The result aliases no memory with the original.
|
|
func (src *Config) Clone() *Config {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Config)
|
|
*dst = *src
|
|
dst.Addresses = append(src.Addresses[:0:0], src.Addresses...)
|
|
dst.DNS = append(src.DNS[:0:0], src.DNS...)
|
|
dst.Peers = make([]Peer, len(src.Peers))
|
|
for i := range dst.Peers {
|
|
dst.Peers[i] = *src.Peers[i].Clone()
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _ConfigCloneNeedsRegeneration = Config(struct {
|
|
Name string
|
|
NodeID tailcfg.StableNodeID
|
|
PrivateKey key.NodePrivate
|
|
Addresses []netip.Prefix
|
|
MTU uint16
|
|
DNS []netip.Addr
|
|
Peers []Peer
|
|
NetworkLogging struct {
|
|
NodeID logid.PrivateID
|
|
DomainID logid.PrivateID
|
|
}
|
|
}{})
|
|
|
|
// Clone makes a deep copy of Peer.
|
|
// The result aliases no memory with the original.
|
|
func (src *Peer) Clone() *Peer {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Peer)
|
|
*dst = *src
|
|
dst.AllowedIPs = append(src.AllowedIPs[:0:0], src.AllowedIPs...)
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _PeerCloneNeedsRegeneration = Peer(struct {
|
|
PublicKey key.NodePublic
|
|
DiscoKey key.DiscoPublic
|
|
AllowedIPs []netip.Prefix
|
|
V4MasqAddr netip.Addr
|
|
PersistentKeepalive uint16
|
|
WGEndpoint key.NodePublic
|
|
}{})
|