mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
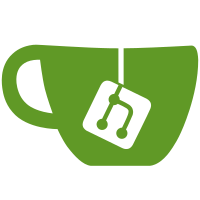
It was requested by the first customer 4-5 years ago and only used for a brief moment of time. We later added netmap visibility trimming which removes the need for this. It's been hidden by the CLI for quite some time and never documented anywhere else. This keeps the CLI flag, though, out of caution. It just returns an error if it's set to anything but true (its default). Fixes #12058 Change-Id: I7514ba572e7b82519b04ed603ff9f3bdbaecfda7 Signed-off-by: Brad Fitzpatrick <bradfitz@tailscale.com>
170 lines
4.6 KiB
Go
170 lines
4.6 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Code generated by tailscale.com/cmd/cloner; DO NOT EDIT.
|
|
|
|
package ipn
|
|
|
|
import (
|
|
"maps"
|
|
"net/netip"
|
|
|
|
"tailscale.com/drive"
|
|
"tailscale.com/tailcfg"
|
|
"tailscale.com/types/opt"
|
|
"tailscale.com/types/persist"
|
|
"tailscale.com/types/preftype"
|
|
)
|
|
|
|
// Clone makes a deep copy of Prefs.
|
|
// The result aliases no memory with the original.
|
|
func (src *Prefs) Clone() *Prefs {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Prefs)
|
|
*dst = *src
|
|
dst.AdvertiseTags = append(src.AdvertiseTags[:0:0], src.AdvertiseTags...)
|
|
dst.AdvertiseRoutes = append(src.AdvertiseRoutes[:0:0], src.AdvertiseRoutes...)
|
|
if src.DriveShares != nil {
|
|
dst.DriveShares = make([]*drive.Share, len(src.DriveShares))
|
|
for i := range dst.DriveShares {
|
|
dst.DriveShares[i] = src.DriveShares[i].Clone()
|
|
}
|
|
}
|
|
dst.Persist = src.Persist.Clone()
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _PrefsCloneNeedsRegeneration = Prefs(struct {
|
|
ControlURL string
|
|
RouteAll bool
|
|
ExitNodeID tailcfg.StableNodeID
|
|
ExitNodeIP netip.Addr
|
|
InternalExitNodePrior tailcfg.StableNodeID
|
|
ExitNodeAllowLANAccess bool
|
|
CorpDNS bool
|
|
RunSSH bool
|
|
RunWebClient bool
|
|
WantRunning bool
|
|
LoggedOut bool
|
|
ShieldsUp bool
|
|
AdvertiseTags []string
|
|
Hostname string
|
|
NotepadURLs bool
|
|
ForceDaemon bool
|
|
Egg bool
|
|
AdvertiseRoutes []netip.Prefix
|
|
NoSNAT bool
|
|
NoStatefulFiltering opt.Bool
|
|
NetfilterMode preftype.NetfilterMode
|
|
OperatorUser string
|
|
ProfileName string
|
|
AutoUpdate AutoUpdatePrefs
|
|
AppConnector AppConnectorPrefs
|
|
PostureChecking bool
|
|
NetfilterKind string
|
|
DriveShares []*drive.Share
|
|
AllowSingleHosts marshalAsTrueInJSON
|
|
Persist *persist.Persist
|
|
}{})
|
|
|
|
// Clone makes a deep copy of ServeConfig.
|
|
// The result aliases no memory with the original.
|
|
func (src *ServeConfig) Clone() *ServeConfig {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(ServeConfig)
|
|
*dst = *src
|
|
if dst.TCP != nil {
|
|
dst.TCP = map[uint16]*TCPPortHandler{}
|
|
for k, v := range src.TCP {
|
|
dst.TCP[k] = v.Clone()
|
|
}
|
|
}
|
|
if dst.Web != nil {
|
|
dst.Web = map[HostPort]*WebServerConfig{}
|
|
for k, v := range src.Web {
|
|
dst.Web[k] = v.Clone()
|
|
}
|
|
}
|
|
dst.AllowFunnel = maps.Clone(src.AllowFunnel)
|
|
if dst.Foreground != nil {
|
|
dst.Foreground = map[string]*ServeConfig{}
|
|
for k, v := range src.Foreground {
|
|
dst.Foreground[k] = v.Clone()
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _ServeConfigCloneNeedsRegeneration = ServeConfig(struct {
|
|
TCP map[uint16]*TCPPortHandler
|
|
Web map[HostPort]*WebServerConfig
|
|
AllowFunnel map[HostPort]bool
|
|
Foreground map[string]*ServeConfig
|
|
ETag string
|
|
}{})
|
|
|
|
// Clone makes a deep copy of TCPPortHandler.
|
|
// The result aliases no memory with the original.
|
|
func (src *TCPPortHandler) Clone() *TCPPortHandler {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(TCPPortHandler)
|
|
*dst = *src
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _TCPPortHandlerCloneNeedsRegeneration = TCPPortHandler(struct {
|
|
HTTPS bool
|
|
HTTP bool
|
|
TCPForward string
|
|
TerminateTLS string
|
|
}{})
|
|
|
|
// Clone makes a deep copy of HTTPHandler.
|
|
// The result aliases no memory with the original.
|
|
func (src *HTTPHandler) Clone() *HTTPHandler {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(HTTPHandler)
|
|
*dst = *src
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _HTTPHandlerCloneNeedsRegeneration = HTTPHandler(struct {
|
|
Path string
|
|
Proxy string
|
|
Text string
|
|
}{})
|
|
|
|
// Clone makes a deep copy of WebServerConfig.
|
|
// The result aliases no memory with the original.
|
|
func (src *WebServerConfig) Clone() *WebServerConfig {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(WebServerConfig)
|
|
*dst = *src
|
|
if dst.Handlers != nil {
|
|
dst.Handlers = map[string]*HTTPHandler{}
|
|
for k, v := range src.Handlers {
|
|
dst.Handlers[k] = v.Clone()
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _WebServerConfigCloneNeedsRegeneration = WebServerConfig(struct {
|
|
Handlers map[string]*HTTPHandler
|
|
}{})
|