mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
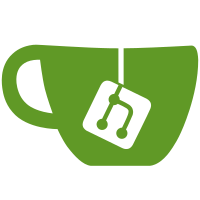
Adds csrf protection and hooks up an initial POST request from the React web client. Updates tailscale/corp#13775 Signed-off-by: Sonia Appasamy <sonia@tailscale.com>
42 lines
927 B
Go
42 lines
927 B
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
package web
|
|
|
|
import (
|
|
"net/http"
|
|
"strings"
|
|
|
|
"github.com/gorilla/csrf"
|
|
"tailscale.com/util/httpm"
|
|
)
|
|
|
|
type api struct {
|
|
s *Server
|
|
}
|
|
|
|
// ServeHTTP serves requests for the web client api.
|
|
// It should only be called by Server.ServeHTTP, via Server.apiHandler,
|
|
// which protects the handler using gorilla csrf.
|
|
func (a *api) ServeHTTP(w http.ResponseWriter, r *http.Request) {
|
|
w.Header().Set("X-CSRF-Token", csrf.Token(r))
|
|
user, err := authorize(w, r)
|
|
if err != nil {
|
|
return
|
|
}
|
|
path := strings.TrimPrefix(r.URL.Path, "/api")
|
|
switch path {
|
|
case "/data":
|
|
switch r.Method {
|
|
case httpm.GET:
|
|
a.s.serveGetNodeDataJSON(w, r, user)
|
|
case httpm.POST:
|
|
a.s.servePostNodeUpdate(w, r)
|
|
default:
|
|
http.Error(w, "method not allowed", http.StatusMethodNotAllowed)
|
|
}
|
|
return
|
|
}
|
|
http.Error(w, "invalid endpoint", http.StatusNotFound)
|
|
}
|