mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
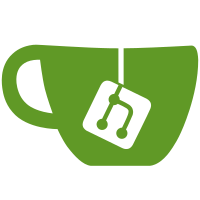
Synology and QNAP both run the web client as a CGI script. The old web client didn't care too much about requests paths, since there was only a single GET and POST handler. The new client serves assets on different paths, so now we need to care. First, enforce that the CGI script is always accessed from its full path, including a trailing slash (e.g. /cgi-bin/tailscale/index.cgi/). Then, strip that prefix off before passing the request along to the main serve handler. This allows for properly serving both static files and the API handler in a CGI environment. Also add a CGIPath option to allow other CGI environments to specify a custom path. Finally, update vite and one "api/data" call to no longer assume that we are always serving at the root path of "/". Updates tailscale/corp#13775 Signed-off-by: Will Norris <will@tailscale.com>
70 lines
2.2 KiB
TypeScript
70 lines
2.2 KiB
TypeScript
/// <reference types="vitest" />
|
|
import { createLogger, defineConfig } from "vite"
|
|
import rewrite from "vite-plugin-rewrite-all"
|
|
import svgr from "vite-plugin-svgr"
|
|
import paths from "vite-tsconfig-paths"
|
|
|
|
// Use a custom logger that filters out Vite's logging of server URLs, since
|
|
// they are an attractive nuisance (we run a proxy in front of Vite, and the
|
|
// tailscale web client should be accessed through that).
|
|
// Unfortunately there's no option to disable this logging, so the best we can
|
|
// do it to ignore calls from a specific function.
|
|
const filteringLogger = createLogger(undefined, { allowClearScreen: false })
|
|
const originalInfoLog = filteringLogger.info
|
|
filteringLogger.info = (...args) => {
|
|
if (new Error("ignored").stack?.includes("printServerUrls")) {
|
|
return
|
|
}
|
|
originalInfoLog.apply(filteringLogger, args)
|
|
}
|
|
|
|
// https://vitejs.dev/config/
|
|
export default defineConfig({
|
|
base: "./",
|
|
plugins: [
|
|
paths(),
|
|
svgr(),
|
|
// By default, the Vite dev server doesn't handle dots
|
|
// in path names and treats them as static files.
|
|
// This plugin changes Vite's routing logic to fix this.
|
|
// See: https://github.com/vitejs/vite/issues/2415
|
|
rewrite(),
|
|
],
|
|
build: {
|
|
outDir: "build",
|
|
sourcemap: true,
|
|
},
|
|
esbuild: {
|
|
logOverride: {
|
|
// Silence a warning about `this` being undefined in ESM when at the
|
|
// top-level. The way JSX is transpiled causes this to happen, but it
|
|
// isn't a problem.
|
|
// See: https://github.com/vitejs/vite/issues/8644
|
|
"this-is-undefined-in-esm": "silent",
|
|
},
|
|
},
|
|
server: {
|
|
// This needs to be 127.0.0.1 instead of localhost, because of how our
|
|
// Go proxy connects to it.
|
|
host: "127.0.0.1",
|
|
// If you change the port, be sure to update the proxy in adminhttp.go too.
|
|
port: 4000,
|
|
// Don't proxy the WebSocket connection used for live reloading by running
|
|
// it on a separate port.
|
|
hmr: {
|
|
protocol: "ws",
|
|
port: 4001,
|
|
},
|
|
},
|
|
test: {
|
|
exclude: ["**/node_modules/**", "**/dist/**"],
|
|
testTimeout: 20000,
|
|
environment: "jsdom",
|
|
deps: {
|
|
inline: ["date-fns", /\.wasm\?url$/],
|
|
},
|
|
},
|
|
clearScreen: false,
|
|
customLogger: filteringLogger,
|
|
})
|