mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
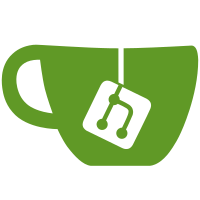
Implements the controller for the new ProxyGroup CRD, designed for running proxies in a high availability configuration. Each proxy gets its own config and state Secret, and its own tailscale node ID. We are currently mounting all of the config secrets into the container, but will stop mounting them and instead read them directly from the kube API once #13578 is implemented. Updates #13406 Signed-off-by: Tom Proctor <tomhjp@users.noreply.github.com>
1069 lines
29 KiB
Go
1069 lines
29 KiB
Go
//go:build !ignore_autogenerated && !plan9
|
|
|
|
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Code generated by controller-gen. DO NOT EDIT.
|
|
|
|
package v1alpha1
|
|
|
|
import (
|
|
corev1 "k8s.io/api/core/v1"
|
|
"k8s.io/apimachinery/pkg/apis/meta/v1"
|
|
"k8s.io/apimachinery/pkg/runtime"
|
|
)
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Connector) DeepCopyInto(out *Connector) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Connector.
|
|
func (in *Connector) DeepCopy() *Connector {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Connector)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *Connector) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ConnectorList) DeepCopyInto(out *ConnectorList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]Connector, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ConnectorList.
|
|
func (in *ConnectorList) DeepCopy() *ConnectorList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ConnectorList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ConnectorList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ConnectorSpec) DeepCopyInto(out *ConnectorSpec) {
|
|
*out = *in
|
|
if in.Tags != nil {
|
|
in, out := &in.Tags, &out.Tags
|
|
*out = make(Tags, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
if in.SubnetRouter != nil {
|
|
in, out := &in.SubnetRouter, &out.SubnetRouter
|
|
*out = new(SubnetRouter)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ConnectorSpec.
|
|
func (in *ConnectorSpec) DeepCopy() *ConnectorSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ConnectorSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ConnectorStatus) DeepCopyInto(out *ConnectorStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
if in.TailnetIPs != nil {
|
|
in, out := &in.TailnetIPs, &out.TailnetIPs
|
|
*out = make([]string, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ConnectorStatus.
|
|
func (in *ConnectorStatus) DeepCopy() *ConnectorStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ConnectorStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Container) DeepCopyInto(out *Container) {
|
|
*out = *in
|
|
if in.Env != nil {
|
|
in, out := &in.Env, &out.Env
|
|
*out = make([]Env, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
in.Resources.DeepCopyInto(&out.Resources)
|
|
if in.SecurityContext != nil {
|
|
in, out := &in.SecurityContext, &out.SecurityContext
|
|
*out = new(corev1.SecurityContext)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Container.
|
|
func (in *Container) DeepCopy() *Container {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Container)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfig) DeepCopyInto(out *DNSConfig) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfig.
|
|
func (in *DNSConfig) DeepCopy() *DNSConfig {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfig)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *DNSConfig) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfigList) DeepCopyInto(out *DNSConfigList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]DNSConfig, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfigList.
|
|
func (in *DNSConfigList) DeepCopy() *DNSConfigList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfigList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *DNSConfigList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfigSpec) DeepCopyInto(out *DNSConfigSpec) {
|
|
*out = *in
|
|
if in.Nameserver != nil {
|
|
in, out := &in.Nameserver, &out.Nameserver
|
|
*out = new(Nameserver)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfigSpec.
|
|
func (in *DNSConfigSpec) DeepCopy() *DNSConfigSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfigSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *DNSConfigStatus) DeepCopyInto(out *DNSConfigStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
if in.Nameserver != nil {
|
|
in, out := &in.Nameserver, &out.Nameserver
|
|
*out = new(NameserverStatus)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new DNSConfigStatus.
|
|
func (in *DNSConfigStatus) DeepCopy() *DNSConfigStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(DNSConfigStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Env) DeepCopyInto(out *Env) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Env.
|
|
func (in *Env) DeepCopy() *Env {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Env)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Metrics) DeepCopyInto(out *Metrics) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Metrics.
|
|
func (in *Metrics) DeepCopy() *Metrics {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Metrics)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Nameserver) DeepCopyInto(out *Nameserver) {
|
|
*out = *in
|
|
if in.Image != nil {
|
|
in, out := &in.Image, &out.Image
|
|
*out = new(NameserverImage)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Nameserver.
|
|
func (in *Nameserver) DeepCopy() *Nameserver {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Nameserver)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *NameserverImage) DeepCopyInto(out *NameserverImage) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new NameserverImage.
|
|
func (in *NameserverImage) DeepCopy() *NameserverImage {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(NameserverImage)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *NameserverStatus) DeepCopyInto(out *NameserverStatus) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new NameserverStatus.
|
|
func (in *NameserverStatus) DeepCopy() *NameserverStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(NameserverStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Pod) DeepCopyInto(out *Pod) {
|
|
*out = *in
|
|
if in.Labels != nil {
|
|
in, out := &in.Labels, &out.Labels
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Annotations != nil {
|
|
in, out := &in.Annotations, &out.Annotations
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Affinity != nil {
|
|
in, out := &in.Affinity, &out.Affinity
|
|
*out = new(corev1.Affinity)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.TailscaleContainer != nil {
|
|
in, out := &in.TailscaleContainer, &out.TailscaleContainer
|
|
*out = new(Container)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.TailscaleInitContainer != nil {
|
|
in, out := &in.TailscaleInitContainer, &out.TailscaleInitContainer
|
|
*out = new(Container)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.SecurityContext != nil {
|
|
in, out := &in.SecurityContext, &out.SecurityContext
|
|
*out = new(corev1.PodSecurityContext)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.ImagePullSecrets != nil {
|
|
in, out := &in.ImagePullSecrets, &out.ImagePullSecrets
|
|
*out = make([]corev1.LocalObjectReference, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
if in.NodeSelector != nil {
|
|
in, out := &in.NodeSelector, &out.NodeSelector
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Tolerations != nil {
|
|
in, out := &in.Tolerations, &out.Tolerations
|
|
*out = make([]corev1.Toleration, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Pod.
|
|
func (in *Pod) DeepCopy() *Pod {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Pod)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClass) DeepCopyInto(out *ProxyClass) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClass.
|
|
func (in *ProxyClass) DeepCopy() *ProxyClass {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClass)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ProxyClass) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClassList) DeepCopyInto(out *ProxyClassList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]ProxyClass, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClassList.
|
|
func (in *ProxyClassList) DeepCopy() *ProxyClassList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClassList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ProxyClassList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClassSpec) DeepCopyInto(out *ProxyClassSpec) {
|
|
*out = *in
|
|
if in.StatefulSet != nil {
|
|
in, out := &in.StatefulSet, &out.StatefulSet
|
|
*out = new(StatefulSet)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.Metrics != nil {
|
|
in, out := &in.Metrics, &out.Metrics
|
|
*out = new(Metrics)
|
|
**out = **in
|
|
}
|
|
if in.TailscaleConfig != nil {
|
|
in, out := &in.TailscaleConfig, &out.TailscaleConfig
|
|
*out = new(TailscaleConfig)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClassSpec.
|
|
func (in *ProxyClassSpec) DeepCopy() *ProxyClassSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClassSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyClassStatus) DeepCopyInto(out *ProxyClassStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyClassStatus.
|
|
func (in *ProxyClassStatus) DeepCopy() *ProxyClassStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyClassStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyGroup) DeepCopyInto(out *ProxyGroup) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyGroup.
|
|
func (in *ProxyGroup) DeepCopy() *ProxyGroup {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyGroup)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ProxyGroup) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyGroupList) DeepCopyInto(out *ProxyGroupList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]ProxyGroup, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyGroupList.
|
|
func (in *ProxyGroupList) DeepCopy() *ProxyGroupList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyGroupList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *ProxyGroupList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyGroupSpec) DeepCopyInto(out *ProxyGroupSpec) {
|
|
*out = *in
|
|
if in.Tags != nil {
|
|
in, out := &in.Tags, &out.Tags
|
|
*out = make(Tags, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
if in.Replicas != nil {
|
|
in, out := &in.Replicas, &out.Replicas
|
|
*out = new(int32)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyGroupSpec.
|
|
func (in *ProxyGroupSpec) DeepCopy() *ProxyGroupSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyGroupSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *ProxyGroupStatus) DeepCopyInto(out *ProxyGroupStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
if in.Devices != nil {
|
|
in, out := &in.Devices, &out.Devices
|
|
*out = make([]TailnetDevice, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new ProxyGroupStatus.
|
|
func (in *ProxyGroupStatus) DeepCopy() *ProxyGroupStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(ProxyGroupStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Recorder) DeepCopyInto(out *Recorder) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ObjectMeta.DeepCopyInto(&out.ObjectMeta)
|
|
in.Spec.DeepCopyInto(&out.Spec)
|
|
in.Status.DeepCopyInto(&out.Status)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Recorder.
|
|
func (in *Recorder) DeepCopy() *Recorder {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Recorder)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *Recorder) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderContainer) DeepCopyInto(out *RecorderContainer) {
|
|
*out = *in
|
|
if in.Env != nil {
|
|
in, out := &in.Env, &out.Env
|
|
*out = make([]Env, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
in.Resources.DeepCopyInto(&out.Resources)
|
|
if in.SecurityContext != nil {
|
|
in, out := &in.SecurityContext, &out.SecurityContext
|
|
*out = new(corev1.SecurityContext)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderContainer.
|
|
func (in *RecorderContainer) DeepCopy() *RecorderContainer {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderContainer)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderList) DeepCopyInto(out *RecorderList) {
|
|
*out = *in
|
|
out.TypeMeta = in.TypeMeta
|
|
in.ListMeta.DeepCopyInto(&out.ListMeta)
|
|
if in.Items != nil {
|
|
in, out := &in.Items, &out.Items
|
|
*out = make([]Recorder, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderList.
|
|
func (in *RecorderList) DeepCopy() *RecorderList {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderList)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyObject is an autogenerated deepcopy function, copying the receiver, creating a new runtime.Object.
|
|
func (in *RecorderList) DeepCopyObject() runtime.Object {
|
|
if c := in.DeepCopy(); c != nil {
|
|
return c
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderPod) DeepCopyInto(out *RecorderPod) {
|
|
*out = *in
|
|
if in.Labels != nil {
|
|
in, out := &in.Labels, &out.Labels
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Annotations != nil {
|
|
in, out := &in.Annotations, &out.Annotations
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Affinity != nil {
|
|
in, out := &in.Affinity, &out.Affinity
|
|
*out = new(corev1.Affinity)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
in.Container.DeepCopyInto(&out.Container)
|
|
if in.SecurityContext != nil {
|
|
in, out := &in.SecurityContext, &out.SecurityContext
|
|
*out = new(corev1.PodSecurityContext)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
if in.ImagePullSecrets != nil {
|
|
in, out := &in.ImagePullSecrets, &out.ImagePullSecrets
|
|
*out = make([]corev1.LocalObjectReference, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
if in.NodeSelector != nil {
|
|
in, out := &in.NodeSelector, &out.NodeSelector
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Tolerations != nil {
|
|
in, out := &in.Tolerations, &out.Tolerations
|
|
*out = make([]corev1.Toleration, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderPod.
|
|
func (in *RecorderPod) DeepCopy() *RecorderPod {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderPod)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderSpec) DeepCopyInto(out *RecorderSpec) {
|
|
*out = *in
|
|
in.StatefulSet.DeepCopyInto(&out.StatefulSet)
|
|
if in.Tags != nil {
|
|
in, out := &in.Tags, &out.Tags
|
|
*out = make(Tags, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
in.Storage.DeepCopyInto(&out.Storage)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderSpec.
|
|
func (in *RecorderSpec) DeepCopy() *RecorderSpec {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderSpec)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderStatefulSet) DeepCopyInto(out *RecorderStatefulSet) {
|
|
*out = *in
|
|
if in.Labels != nil {
|
|
in, out := &in.Labels, &out.Labels
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Annotations != nil {
|
|
in, out := &in.Annotations, &out.Annotations
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
in.Pod.DeepCopyInto(&out.Pod)
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderStatefulSet.
|
|
func (in *RecorderStatefulSet) DeepCopy() *RecorderStatefulSet {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderStatefulSet)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderStatus) DeepCopyInto(out *RecorderStatus) {
|
|
*out = *in
|
|
if in.Conditions != nil {
|
|
in, out := &in.Conditions, &out.Conditions
|
|
*out = make([]v1.Condition, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
if in.Devices != nil {
|
|
in, out := &in.Devices, &out.Devices
|
|
*out = make([]RecorderTailnetDevice, len(*in))
|
|
for i := range *in {
|
|
(*in)[i].DeepCopyInto(&(*out)[i])
|
|
}
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderStatus.
|
|
func (in *RecorderStatus) DeepCopy() *RecorderStatus {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderStatus)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *RecorderTailnetDevice) DeepCopyInto(out *RecorderTailnetDevice) {
|
|
*out = *in
|
|
if in.TailnetIPs != nil {
|
|
in, out := &in.TailnetIPs, &out.TailnetIPs
|
|
*out = make([]string, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new RecorderTailnetDevice.
|
|
func (in *RecorderTailnetDevice) DeepCopy() *RecorderTailnetDevice {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(RecorderTailnetDevice)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in Routes) DeepCopyInto(out *Routes) {
|
|
{
|
|
in := &in
|
|
*out = make(Routes, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Routes.
|
|
func (in Routes) DeepCopy() Routes {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Routes)
|
|
in.DeepCopyInto(out)
|
|
return *out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *S3) DeepCopyInto(out *S3) {
|
|
*out = *in
|
|
out.Credentials = in.Credentials
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new S3.
|
|
func (in *S3) DeepCopy() *S3 {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(S3)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *S3Credentials) DeepCopyInto(out *S3Credentials) {
|
|
*out = *in
|
|
out.Secret = in.Secret
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new S3Credentials.
|
|
func (in *S3Credentials) DeepCopy() *S3Credentials {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(S3Credentials)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *S3Secret) DeepCopyInto(out *S3Secret) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new S3Secret.
|
|
func (in *S3Secret) DeepCopy() *S3Secret {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(S3Secret)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *StatefulSet) DeepCopyInto(out *StatefulSet) {
|
|
*out = *in
|
|
if in.Labels != nil {
|
|
in, out := &in.Labels, &out.Labels
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Annotations != nil {
|
|
in, out := &in.Annotations, &out.Annotations
|
|
*out = make(map[string]string, len(*in))
|
|
for key, val := range *in {
|
|
(*out)[key] = val
|
|
}
|
|
}
|
|
if in.Pod != nil {
|
|
in, out := &in.Pod, &out.Pod
|
|
*out = new(Pod)
|
|
(*in).DeepCopyInto(*out)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new StatefulSet.
|
|
func (in *StatefulSet) DeepCopy() *StatefulSet {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(StatefulSet)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *Storage) DeepCopyInto(out *Storage) {
|
|
*out = *in
|
|
if in.S3 != nil {
|
|
in, out := &in.S3, &out.S3
|
|
*out = new(S3)
|
|
**out = **in
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Storage.
|
|
func (in *Storage) DeepCopy() *Storage {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Storage)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *SubnetRouter) DeepCopyInto(out *SubnetRouter) {
|
|
*out = *in
|
|
if in.AdvertiseRoutes != nil {
|
|
in, out := &in.AdvertiseRoutes, &out.AdvertiseRoutes
|
|
*out = make(Routes, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new SubnetRouter.
|
|
func (in *SubnetRouter) DeepCopy() *SubnetRouter {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(SubnetRouter)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in Tags) DeepCopyInto(out *Tags) {
|
|
{
|
|
in := &in
|
|
*out = make(Tags, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new Tags.
|
|
func (in Tags) DeepCopy() Tags {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(Tags)
|
|
in.DeepCopyInto(out)
|
|
return *out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *TailnetDevice) DeepCopyInto(out *TailnetDevice) {
|
|
*out = *in
|
|
if in.TailnetIPs != nil {
|
|
in, out := &in.TailnetIPs, &out.TailnetIPs
|
|
*out = make([]string, len(*in))
|
|
copy(*out, *in)
|
|
}
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new TailnetDevice.
|
|
func (in *TailnetDevice) DeepCopy() *TailnetDevice {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(TailnetDevice)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|
|
|
|
// DeepCopyInto is an autogenerated deepcopy function, copying the receiver, writing into out. in must be non-nil.
|
|
func (in *TailscaleConfig) DeepCopyInto(out *TailscaleConfig) {
|
|
*out = *in
|
|
}
|
|
|
|
// DeepCopy is an autogenerated deepcopy function, copying the receiver, creating a new TailscaleConfig.
|
|
func (in *TailscaleConfig) DeepCopy() *TailscaleConfig {
|
|
if in == nil {
|
|
return nil
|
|
}
|
|
out := new(TailscaleConfig)
|
|
in.DeepCopyInto(out)
|
|
return out
|
|
}
|