mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
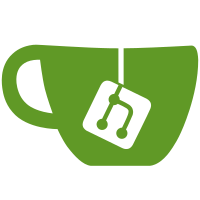
The node and domain audit log IDs are provided in the map response, but are ultimately going to be used in wgengine since that's the layer that manages the tstun.Wrapper. Do the plumbing work to get this field passed down the stack. Signed-off-by: Joe Tsai <joetsai@digital-static.net>
67 lines
1.7 KiB
Go
67 lines
1.7 KiB
Go
// Copyright (c) 2022 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// Code generated by tailscale.com/cmd/cloner; DO NOT EDIT.
|
|
|
|
package wgcfg
|
|
|
|
import (
|
|
"net/netip"
|
|
|
|
"tailscale.com/logtail"
|
|
"tailscale.com/types/key"
|
|
)
|
|
|
|
// Clone makes a deep copy of Config.
|
|
// The result aliases no memory with the original.
|
|
func (src *Config) Clone() *Config {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Config)
|
|
*dst = *src
|
|
dst.Addresses = append(src.Addresses[:0:0], src.Addresses...)
|
|
dst.DNS = append(src.DNS[:0:0], src.DNS...)
|
|
dst.Peers = make([]Peer, len(src.Peers))
|
|
for i := range dst.Peers {
|
|
dst.Peers[i] = *src.Peers[i].Clone()
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _ConfigCloneNeedsRegeneration = Config(struct {
|
|
Name string
|
|
PrivateKey key.NodePrivate
|
|
Addresses []netip.Prefix
|
|
MTU uint16
|
|
DNS []netip.Addr
|
|
Peers []Peer
|
|
NetworkLogging struct {
|
|
NodeID logtail.PrivateID
|
|
DomainID logtail.PrivateID
|
|
}
|
|
}{})
|
|
|
|
// Clone makes a deep copy of Peer.
|
|
// The result aliases no memory with the original.
|
|
func (src *Peer) Clone() *Peer {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Peer)
|
|
*dst = *src
|
|
dst.AllowedIPs = append(src.AllowedIPs[:0:0], src.AllowedIPs...)
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _PeerCloneNeedsRegeneration = Peer(struct {
|
|
PublicKey key.NodePublic
|
|
DiscoKey key.DiscoPublic
|
|
AllowedIPs []netip.Prefix
|
|
PersistentKeepalive uint16
|
|
WGEndpoint key.NodePublic
|
|
}{})
|