mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
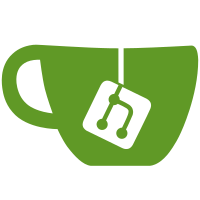
Thus new function allows constructing vizerrors that combine a message appropriate for display to users with a wrapped underlying error. Updates tailscale/corp#23781 Signed-off-by: Percy Wegmann <percy@tailscale.com>
67 lines
1.5 KiB
Go
67 lines
1.5 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
package vizerror
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"io/fs"
|
|
"testing"
|
|
)
|
|
|
|
func TestNew(t *testing.T) {
|
|
err := New("abc")
|
|
if err.Error() != "abc" {
|
|
t.Errorf(`New("abc").Error() = %q, want %q`, err.Error(), "abc")
|
|
}
|
|
}
|
|
|
|
func TestErrorf(t *testing.T) {
|
|
err := Errorf("%w", fs.ErrNotExist)
|
|
|
|
if got, want := err.Error(), "file does not exist"; got != want {
|
|
t.Errorf("Errorf().Error() = %q, want %q", got, want)
|
|
}
|
|
|
|
// ensure error wrapping still works
|
|
if !errors.Is(err, fs.ErrNotExist) {
|
|
t.Errorf("error chain does not contain fs.ErrNotExist")
|
|
}
|
|
}
|
|
|
|
func TestAs(t *testing.T) {
|
|
verr := New("visible error")
|
|
err := fmt.Errorf("wrap: %w", verr)
|
|
|
|
got, ok := As(err)
|
|
if !ok {
|
|
t.Errorf("As() return false, want true")
|
|
}
|
|
if got != verr {
|
|
t.Errorf("As() returned error %v, want %v", got, verr)
|
|
}
|
|
}
|
|
|
|
func TestWrap(t *testing.T) {
|
|
wrapped := errors.New("wrapped")
|
|
err := Wrap(wrapped)
|
|
if err.Error() != "wrapped" {
|
|
t.Errorf(`Wrap(wrapped).Error() = %q, want %q`, err.Error(), "wrapped")
|
|
}
|
|
if errors.Unwrap(err) != wrapped {
|
|
t.Errorf("Unwrap = %q, want %q", errors.Unwrap(err), wrapped)
|
|
}
|
|
}
|
|
|
|
func TestWrapWithMessage(t *testing.T) {
|
|
wrapped := errors.New("wrapped")
|
|
err := WrapWithMessage(wrapped, "safe")
|
|
if err.Error() != "safe" {
|
|
t.Errorf(`WrapWithMessage(wrapped, "safe").Error() = %q, want %q`, err.Error(), "safe")
|
|
}
|
|
if errors.Unwrap(err) != wrapped {
|
|
t.Errorf("Unwrap = %q, want %q", errors.Unwrap(err), wrapped)
|
|
}
|
|
}
|