mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 11:35:35 +00:00
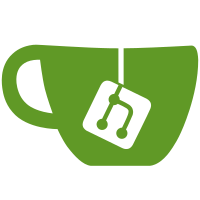
Go 1.23 updates the go/types package to produce Alias type nodes for type aliases, unless disabled with gotypesalias=0. This new default behavior breaks codegen.LookupMethod, which uses checked type assertions to types.Named and types.Interface, as only named types and interfaces have methods. In this PR, we update codegen.LookupMethod to perform method lookup on the right-hand side of the alias declaration and clearly switch on the supported type nodes types. We also improve support for various edge cases, such as when an alias is used as a type parameter constraint, and add tests for the LookupMethod function. Additionally, we update cmd/viewer/tests to include types with aliases used in type fields and generic type constraints. Updates #13224 Updates #12912 Signed-off-by: Nick Khyl <nickk@tailscale.com>
482 lines
14 KiB
Go
482 lines
14 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Code generated by tailscale.com/cmd/cloner; DO NOT EDIT.
|
|
|
|
package tests
|
|
|
|
import (
|
|
"maps"
|
|
"net/netip"
|
|
|
|
"golang.org/x/exp/constraints"
|
|
"tailscale.com/types/ptr"
|
|
"tailscale.com/types/views"
|
|
)
|
|
|
|
// Clone makes a deep copy of StructWithPtrs.
|
|
// The result aliases no memory with the original.
|
|
func (src *StructWithPtrs) Clone() *StructWithPtrs {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(StructWithPtrs)
|
|
*dst = *src
|
|
if dst.Value != nil {
|
|
dst.Value = ptr.To(*src.Value)
|
|
}
|
|
if dst.Int != nil {
|
|
dst.Int = ptr.To(*src.Int)
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _StructWithPtrsCloneNeedsRegeneration = StructWithPtrs(struct {
|
|
Value *StructWithoutPtrs
|
|
Int *int
|
|
NoCloneValue *StructWithoutPtrs
|
|
}{})
|
|
|
|
// Clone makes a deep copy of StructWithoutPtrs.
|
|
// The result aliases no memory with the original.
|
|
func (src *StructWithoutPtrs) Clone() *StructWithoutPtrs {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(StructWithoutPtrs)
|
|
*dst = *src
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _StructWithoutPtrsCloneNeedsRegeneration = StructWithoutPtrs(struct {
|
|
Int int
|
|
Pfx netip.Prefix
|
|
}{})
|
|
|
|
// Clone makes a deep copy of Map.
|
|
// The result aliases no memory with the original.
|
|
func (src *Map) Clone() *Map {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Map)
|
|
*dst = *src
|
|
dst.Int = maps.Clone(src.Int)
|
|
if dst.SliceInt != nil {
|
|
dst.SliceInt = map[string][]int{}
|
|
for k := range src.SliceInt {
|
|
dst.SliceInt[k] = append([]int{}, src.SliceInt[k]...)
|
|
}
|
|
}
|
|
if dst.StructPtrWithPtr != nil {
|
|
dst.StructPtrWithPtr = map[string]*StructWithPtrs{}
|
|
for k, v := range src.StructPtrWithPtr {
|
|
if v == nil {
|
|
dst.StructPtrWithPtr[k] = nil
|
|
} else {
|
|
dst.StructPtrWithPtr[k] = v.Clone()
|
|
}
|
|
}
|
|
}
|
|
if dst.StructPtrWithoutPtr != nil {
|
|
dst.StructPtrWithoutPtr = map[string]*StructWithoutPtrs{}
|
|
for k, v := range src.StructPtrWithoutPtr {
|
|
if v == nil {
|
|
dst.StructPtrWithoutPtr[k] = nil
|
|
} else {
|
|
dst.StructPtrWithoutPtr[k] = ptr.To(*v)
|
|
}
|
|
}
|
|
}
|
|
dst.StructWithoutPtr = maps.Clone(src.StructWithoutPtr)
|
|
if dst.SlicesWithPtrs != nil {
|
|
dst.SlicesWithPtrs = map[string][]*StructWithPtrs{}
|
|
for k := range src.SlicesWithPtrs {
|
|
dst.SlicesWithPtrs[k] = append([]*StructWithPtrs{}, src.SlicesWithPtrs[k]...)
|
|
}
|
|
}
|
|
if dst.SlicesWithoutPtrs != nil {
|
|
dst.SlicesWithoutPtrs = map[string][]*StructWithoutPtrs{}
|
|
for k := range src.SlicesWithoutPtrs {
|
|
dst.SlicesWithoutPtrs[k] = append([]*StructWithoutPtrs{}, src.SlicesWithoutPtrs[k]...)
|
|
}
|
|
}
|
|
dst.StructWithoutPtrKey = maps.Clone(src.StructWithoutPtrKey)
|
|
if dst.StructWithPtr != nil {
|
|
dst.StructWithPtr = map[string]StructWithPtrs{}
|
|
for k, v := range src.StructWithPtr {
|
|
dst.StructWithPtr[k] = *(v.Clone())
|
|
}
|
|
}
|
|
if dst.SliceIntPtr != nil {
|
|
dst.SliceIntPtr = map[string][]*int{}
|
|
for k := range src.SliceIntPtr {
|
|
dst.SliceIntPtr[k] = append([]*int{}, src.SliceIntPtr[k]...)
|
|
}
|
|
}
|
|
dst.PointerKey = maps.Clone(src.PointerKey)
|
|
dst.StructWithPtrKey = maps.Clone(src.StructWithPtrKey)
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _MapCloneNeedsRegeneration = Map(struct {
|
|
Int map[string]int
|
|
SliceInt map[string][]int
|
|
StructPtrWithPtr map[string]*StructWithPtrs
|
|
StructPtrWithoutPtr map[string]*StructWithoutPtrs
|
|
StructWithoutPtr map[string]StructWithoutPtrs
|
|
SlicesWithPtrs map[string][]*StructWithPtrs
|
|
SlicesWithoutPtrs map[string][]*StructWithoutPtrs
|
|
StructWithoutPtrKey map[StructWithoutPtrs]int
|
|
StructWithPtr map[string]StructWithPtrs
|
|
SliceIntPtr map[string][]*int
|
|
PointerKey map[*string]int
|
|
StructWithPtrKey map[StructWithPtrs]int
|
|
}{})
|
|
|
|
// Clone makes a deep copy of StructWithSlices.
|
|
// The result aliases no memory with the original.
|
|
func (src *StructWithSlices) Clone() *StructWithSlices {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(StructWithSlices)
|
|
*dst = *src
|
|
dst.Values = append(src.Values[:0:0], src.Values...)
|
|
if src.ValuePointers != nil {
|
|
dst.ValuePointers = make([]*StructWithoutPtrs, len(src.ValuePointers))
|
|
for i := range dst.ValuePointers {
|
|
if src.ValuePointers[i] == nil {
|
|
dst.ValuePointers[i] = nil
|
|
} else {
|
|
dst.ValuePointers[i] = ptr.To(*src.ValuePointers[i])
|
|
}
|
|
}
|
|
}
|
|
if src.StructPointers != nil {
|
|
dst.StructPointers = make([]*StructWithPtrs, len(src.StructPointers))
|
|
for i := range dst.StructPointers {
|
|
if src.StructPointers[i] == nil {
|
|
dst.StructPointers[i] = nil
|
|
} else {
|
|
dst.StructPointers[i] = src.StructPointers[i].Clone()
|
|
}
|
|
}
|
|
}
|
|
dst.Slice = append(src.Slice[:0:0], src.Slice...)
|
|
dst.Prefixes = append(src.Prefixes[:0:0], src.Prefixes...)
|
|
dst.Data = append(src.Data[:0:0], src.Data...)
|
|
if src.Structs != nil {
|
|
dst.Structs = make([]StructWithPtrs, len(src.Structs))
|
|
for i := range dst.Structs {
|
|
dst.Structs[i] = *src.Structs[i].Clone()
|
|
}
|
|
}
|
|
if src.Ints != nil {
|
|
dst.Ints = make([]*int, len(src.Ints))
|
|
for i := range dst.Ints {
|
|
if src.Ints[i] == nil {
|
|
dst.Ints[i] = nil
|
|
} else {
|
|
dst.Ints[i] = ptr.To(*src.Ints[i])
|
|
}
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _StructWithSlicesCloneNeedsRegeneration = StructWithSlices(struct {
|
|
Values []StructWithoutPtrs
|
|
ValuePointers []*StructWithoutPtrs
|
|
StructPointers []*StructWithPtrs
|
|
Slice []string
|
|
Prefixes []netip.Prefix
|
|
Data []byte
|
|
Structs []StructWithPtrs
|
|
Ints []*int
|
|
}{})
|
|
|
|
// Clone makes a deep copy of OnlyGetClone.
|
|
// The result aliases no memory with the original.
|
|
func (src *OnlyGetClone) Clone() *OnlyGetClone {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(OnlyGetClone)
|
|
*dst = *src
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _OnlyGetCloneCloneNeedsRegeneration = OnlyGetClone(struct {
|
|
SinViewerPorFavor bool
|
|
}{})
|
|
|
|
// Clone makes a deep copy of StructWithEmbedded.
|
|
// The result aliases no memory with the original.
|
|
func (src *StructWithEmbedded) Clone() *StructWithEmbedded {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(StructWithEmbedded)
|
|
*dst = *src
|
|
dst.A = src.A.Clone()
|
|
dst.StructWithSlices = *src.StructWithSlices.Clone()
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _StructWithEmbeddedCloneNeedsRegeneration = StructWithEmbedded(struct {
|
|
A *StructWithPtrs
|
|
StructWithSlices
|
|
}{})
|
|
|
|
// Clone makes a deep copy of GenericIntStruct.
|
|
// The result aliases no memory with the original.
|
|
func (src *GenericIntStruct[T]) Clone() *GenericIntStruct[T] {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(GenericIntStruct[T])
|
|
*dst = *src
|
|
if dst.Pointer != nil {
|
|
dst.Pointer = ptr.To(*src.Pointer)
|
|
}
|
|
dst.Slice = append(src.Slice[:0:0], src.Slice...)
|
|
dst.Map = maps.Clone(src.Map)
|
|
if src.PtrSlice != nil {
|
|
dst.PtrSlice = make([]*T, len(src.PtrSlice))
|
|
for i := range dst.PtrSlice {
|
|
if src.PtrSlice[i] == nil {
|
|
dst.PtrSlice[i] = nil
|
|
} else {
|
|
dst.PtrSlice[i] = ptr.To(*src.PtrSlice[i])
|
|
}
|
|
}
|
|
}
|
|
dst.PtrKeyMap = maps.Clone(src.PtrKeyMap)
|
|
if dst.PtrValueMap != nil {
|
|
dst.PtrValueMap = map[string]*T{}
|
|
for k, v := range src.PtrValueMap {
|
|
if v == nil {
|
|
dst.PtrValueMap[k] = nil
|
|
} else {
|
|
dst.PtrValueMap[k] = ptr.To(*v)
|
|
}
|
|
}
|
|
}
|
|
if dst.SliceMap != nil {
|
|
dst.SliceMap = map[string][]T{}
|
|
for k := range src.SliceMap {
|
|
dst.SliceMap[k] = append([]T{}, src.SliceMap[k]...)
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
func _GenericIntStructCloneNeedsRegeneration[T constraints.Integer](GenericIntStruct[T]) {
|
|
_GenericIntStructCloneNeedsRegeneration(struct {
|
|
Value T
|
|
Pointer *T
|
|
Slice []T
|
|
Map map[string]T
|
|
PtrSlice []*T
|
|
PtrKeyMap map[*T]string `json:"-"`
|
|
PtrValueMap map[string]*T
|
|
SliceMap map[string][]T
|
|
}{})
|
|
}
|
|
|
|
// Clone makes a deep copy of GenericNoPtrsStruct.
|
|
// The result aliases no memory with the original.
|
|
func (src *GenericNoPtrsStruct[T]) Clone() *GenericNoPtrsStruct[T] {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(GenericNoPtrsStruct[T])
|
|
*dst = *src
|
|
if dst.Pointer != nil {
|
|
dst.Pointer = ptr.To(*src.Pointer)
|
|
}
|
|
dst.Slice = append(src.Slice[:0:0], src.Slice...)
|
|
dst.Map = maps.Clone(src.Map)
|
|
if src.PtrSlice != nil {
|
|
dst.PtrSlice = make([]*T, len(src.PtrSlice))
|
|
for i := range dst.PtrSlice {
|
|
if src.PtrSlice[i] == nil {
|
|
dst.PtrSlice[i] = nil
|
|
} else {
|
|
dst.PtrSlice[i] = ptr.To(*src.PtrSlice[i])
|
|
}
|
|
}
|
|
}
|
|
dst.PtrKeyMap = maps.Clone(src.PtrKeyMap)
|
|
if dst.PtrValueMap != nil {
|
|
dst.PtrValueMap = map[string]*T{}
|
|
for k, v := range src.PtrValueMap {
|
|
if v == nil {
|
|
dst.PtrValueMap[k] = nil
|
|
} else {
|
|
dst.PtrValueMap[k] = ptr.To(*v)
|
|
}
|
|
}
|
|
}
|
|
if dst.SliceMap != nil {
|
|
dst.SliceMap = map[string][]T{}
|
|
for k := range src.SliceMap {
|
|
dst.SliceMap[k] = append([]T{}, src.SliceMap[k]...)
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
func _GenericNoPtrsStructCloneNeedsRegeneration[T StructWithoutPtrs | netip.Prefix | BasicType](GenericNoPtrsStruct[T]) {
|
|
_GenericNoPtrsStructCloneNeedsRegeneration(struct {
|
|
Value T
|
|
Pointer *T
|
|
Slice []T
|
|
Map map[string]T
|
|
PtrSlice []*T
|
|
PtrKeyMap map[*T]string `json:"-"`
|
|
PtrValueMap map[string]*T
|
|
SliceMap map[string][]T
|
|
}{})
|
|
}
|
|
|
|
// Clone makes a deep copy of GenericCloneableStruct.
|
|
// The result aliases no memory with the original.
|
|
func (src *GenericCloneableStruct[T, V]) Clone() *GenericCloneableStruct[T, V] {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(GenericCloneableStruct[T, V])
|
|
*dst = *src
|
|
dst.Value = src.Value.Clone()
|
|
if src.Slice != nil {
|
|
dst.Slice = make([]T, len(src.Slice))
|
|
for i := range dst.Slice {
|
|
dst.Slice[i] = src.Slice[i].Clone()
|
|
}
|
|
}
|
|
if dst.Map != nil {
|
|
dst.Map = map[string]T{}
|
|
for k, v := range src.Map {
|
|
dst.Map[k] = v.Clone()
|
|
}
|
|
}
|
|
if dst.Pointer != nil {
|
|
dst.Pointer = ptr.To((*src.Pointer).Clone())
|
|
}
|
|
if src.PtrSlice != nil {
|
|
dst.PtrSlice = make([]*T, len(src.PtrSlice))
|
|
for i := range dst.PtrSlice {
|
|
if src.PtrSlice[i] == nil {
|
|
dst.PtrSlice[i] = nil
|
|
} else {
|
|
dst.PtrSlice[i] = ptr.To((*src.PtrSlice[i]).Clone())
|
|
}
|
|
}
|
|
}
|
|
dst.PtrKeyMap = maps.Clone(src.PtrKeyMap)
|
|
if dst.PtrValueMap != nil {
|
|
dst.PtrValueMap = map[string]*T{}
|
|
for k, v := range src.PtrValueMap {
|
|
if v == nil {
|
|
dst.PtrValueMap[k] = nil
|
|
} else {
|
|
dst.PtrValueMap[k] = ptr.To((*v).Clone())
|
|
}
|
|
}
|
|
}
|
|
if dst.SliceMap != nil {
|
|
dst.SliceMap = map[string][]T{}
|
|
for k := range src.SliceMap {
|
|
dst.SliceMap[k] = append([]T{}, src.SliceMap[k]...)
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
func _GenericCloneableStructCloneNeedsRegeneration[T views.ViewCloner[T, V], V views.StructView[T]](GenericCloneableStruct[T, V]) {
|
|
_GenericCloneableStructCloneNeedsRegeneration(struct {
|
|
Value T
|
|
Slice []T
|
|
Map map[string]T
|
|
Pointer *T
|
|
PtrSlice []*T
|
|
PtrKeyMap map[*T]string `json:"-"`
|
|
PtrValueMap map[string]*T
|
|
SliceMap map[string][]T
|
|
}{})
|
|
}
|
|
|
|
// Clone makes a deep copy of StructWithContainers.
|
|
// The result aliases no memory with the original.
|
|
func (src *StructWithContainers) Clone() *StructWithContainers {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(StructWithContainers)
|
|
*dst = *src
|
|
dst.CloneableContainer = *src.CloneableContainer.Clone()
|
|
dst.CloneableGenericContainer = *src.CloneableGenericContainer.Clone()
|
|
dst.CloneableMap = *src.CloneableMap.Clone()
|
|
dst.CloneableGenericMap = *src.CloneableGenericMap.Clone()
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _StructWithContainersCloneNeedsRegeneration = StructWithContainers(struct {
|
|
IntContainer Container[int]
|
|
CloneableContainer Container[*StructWithPtrs]
|
|
BasicGenericContainer Container[GenericBasicStruct[int]]
|
|
CloneableGenericContainer Container[*GenericNoPtrsStruct[int]]
|
|
CloneableMap MapContainer[int, *StructWithPtrs]
|
|
CloneableGenericMap MapContainer[int, *GenericNoPtrsStruct[int]]
|
|
}{})
|
|
|
|
// Clone makes a deep copy of StructWithTypeAliasFields.
|
|
// The result aliases no memory with the original.
|
|
func (src *StructWithTypeAliasFields) Clone() *StructWithTypeAliasFields {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(StructWithTypeAliasFields)
|
|
*dst = *src
|
|
panic("TODO: WithPtr (*types.Struct)")
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _StructWithTypeAliasFieldsCloneNeedsRegeneration = StructWithTypeAliasFields(struct {
|
|
WithPtr StructWithPtrsAlias
|
|
WithoutPtr StructWithoutPtrsAlias
|
|
}{})
|
|
|
|
// Clone makes a deep copy of GenericTypeAliasStruct.
|
|
// The result aliases no memory with the original.
|
|
func (src *GenericTypeAliasStruct[T, T2, V2]) Clone() *GenericTypeAliasStruct[T, T2, V2] {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(GenericTypeAliasStruct[T, T2, V2])
|
|
*dst = *src
|
|
dst.Cloneable = src.Cloneable.Clone()
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
func _GenericTypeAliasStructCloneNeedsRegeneration[T integer, T2 views.ViewCloner[T2, V2], V2 views.StructView[T2]](GenericTypeAliasStruct[T, T2, V2]) {
|
|
_GenericTypeAliasStructCloneNeedsRegeneration(struct {
|
|
NonCloneable T
|
|
Cloneable T2
|
|
}{})
|
|
}
|