mirror of
https://github.com/tailscale/tailscale.git
synced 2025-03-28 03:52:35 +00:00
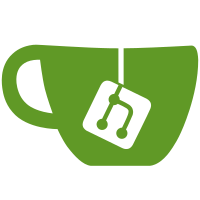
Reduces the amount of boilerplate to render the UI and makes it easier to respond to state changes (e.g. machine getting authorized, netmap changing, etc.) Preact adds ~13K to our bundle size (5K after Brotli) thus is a neglibible size contribution. We mitigate the delay in rendering the UI by having a static placeholder in the HTML. Required bumping the esbuild version to pick up evanw/esbuild#2349, which makes it easier to support Preact's JSX code generation. Fixes #5137 Fixes #5273 Signed-off-by: Mihai Parparita <mihai@tailscale.com>
30 lines
940 B
TypeScript
30 lines
940 B
TypeScript
// Copyright (c) 2022 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
import "./wasm_exec"
|
|
import wasmUrl from "./main.wasm"
|
|
import { sessionStateStorage } from "./js-state-store"
|
|
import { renderApp } from "./app"
|
|
|
|
async function main() {
|
|
const app = await renderApp()
|
|
const go = new Go()
|
|
const wasmInstance = await WebAssembly.instantiateStreaming(
|
|
fetch(`./dist/${wasmUrl}`),
|
|
go.importObject
|
|
)
|
|
// The Go process should never exit, if it does then it's an unhandled panic.
|
|
go.run(wasmInstance.instance).then(() =>
|
|
app.handleGoPanic("Unexpected shutdown")
|
|
)
|
|
const ipn = newIPN({
|
|
// Persist IPN state in sessionStorage in development, so that we don't need
|
|
// to re-authorize every time we reload the page.
|
|
stateStorage: DEBUG ? sessionStateStorage : undefined,
|
|
})
|
|
app.runWithIPN(ipn)
|
|
}
|
|
|
|
main()
|