mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-27 03:55:36 +00:00
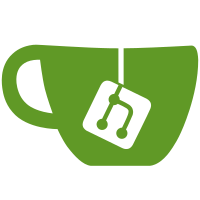
We add package defining interfaces for policy stores, enabling creation of policy sources and reading settings from them. It includes a Windows-specific PlatformPolicyStore for GP and MDM policies stored in the Registry, and an in-memory TestStore for testing purposes. We also include an internal package that tracks and reports policy usage metrics when a policy setting is read from a store. Initially, it will be used only on Windows and Android, as macOS, iOS, and tvOS report their own metrics. However, we plan to use it across all platforms eventually. Updates #12687 Signed-off-by: Nick Khyl <nickk@tailscale.com>
47 lines
1.2 KiB
Go
47 lines
1.2 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Package loggerx provides logging functions to the rest of the syspolicy packages.
|
|
package loggerx
|
|
|
|
import (
|
|
"log"
|
|
|
|
"tailscale.com/types/lazy"
|
|
"tailscale.com/types/logger"
|
|
"tailscale.com/util/syspolicy/internal"
|
|
)
|
|
|
|
const (
|
|
errorPrefix = "syspolicy: "
|
|
verbosePrefix = "syspolicy: [v2] "
|
|
)
|
|
|
|
var (
|
|
lazyErrorf lazy.SyncValue[logger.Logf]
|
|
lazyVerbosef lazy.SyncValue[logger.Logf]
|
|
)
|
|
|
|
// Errorf formats and writes an error message to the log.
|
|
func Errorf(format string, args ...any) {
|
|
errorf := lazyErrorf.Get(func() logger.Logf {
|
|
return logger.WithPrefix(log.Printf, errorPrefix)
|
|
})
|
|
errorf(format, args...)
|
|
}
|
|
|
|
// Verbosef formats and writes an optional, verbose message to the log.
|
|
func Verbosef(format string, args ...any) {
|
|
verbosef := lazyVerbosef.Get(func() logger.Logf {
|
|
return logger.WithPrefix(log.Printf, verbosePrefix)
|
|
})
|
|
verbosef(format, args...)
|
|
}
|
|
|
|
// SetForTest sets the specified errorf and verbosef functions for the duration
|
|
// of tb and its subtests.
|
|
func SetForTest(tb internal.TB, errorf, verbosef logger.Logf) {
|
|
lazyErrorf.SetForTest(tb, errorf, nil)
|
|
lazyVerbosef.SetForTest(tb, verbosef, nil)
|
|
}
|