mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-26 03:25:35 +00:00
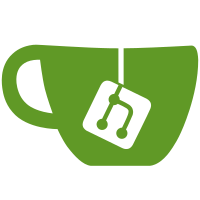
I missed connecting some controlknobs.Knobs pieces in 4e91cf20a8
resulting in that breaking control knobs entirely.
Whoops.
The fix in ipn/ipnlocal (where it makes a new controlclient) but to
atone, I also added integration tests. Those integration tests use
a new "tailscale debug control-knobs" which by itself might be useful
for future debugging.
Updates #9351
Change-Id: Id9c89c8637746d879d5da67b9ac4e0d2367a3f0d
Signed-off-by: Brad Fitzpatrick <bradfitz@tailscale.com>
58 lines
1.8 KiB
Go
58 lines
1.8 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Package controlknobs contains client options configurable from control which can be turned on
|
|
// or off. The ability to turn options on and off is for incrementally adding features in.
|
|
package controlknobs
|
|
|
|
import (
|
|
"sync/atomic"
|
|
|
|
"tailscale.com/syncs"
|
|
"tailscale.com/types/opt"
|
|
)
|
|
|
|
// Knobs is the set of knobs that the control plane's coordination server can
|
|
// adjust at runtime.
|
|
type Knobs struct {
|
|
// DisableUPnP indicates whether to attempt UPnP mapping.
|
|
DisableUPnP atomic.Bool
|
|
|
|
// DisableDRPO is whether control says to disable the
|
|
// DERP route optimization (Issue 150).
|
|
DisableDRPO atomic.Bool
|
|
|
|
// KeepFullWGConfig is whether we should disable the lazy wireguard
|
|
// programming and instead give WireGuard the full netmap always, even for
|
|
// idle peers.
|
|
KeepFullWGConfig atomic.Bool
|
|
|
|
// RandomizeClientPort is whether control says we should randomize
|
|
// the client port.
|
|
RandomizeClientPort atomic.Bool
|
|
|
|
// OneCGNAT is whether the the node should make one big CGNAT route
|
|
// in the OS rather than one /32 per peer.
|
|
OneCGNAT syncs.AtomicValue[opt.Bool]
|
|
|
|
// ForceBackgroundSTUN forces netcheck STUN queries to keep
|
|
// running in magicsock, even when idle.
|
|
ForceBackgroundSTUN atomic.Bool
|
|
}
|
|
|
|
// AsDebugJSON returns k as something that can be marshalled with json.Marshal
|
|
// for debug.
|
|
func (k *Knobs) AsDebugJSON() map[string]any {
|
|
if k == nil {
|
|
return nil
|
|
}
|
|
return map[string]any{
|
|
"DisableUPnP": k.DisableUPnP.Load(),
|
|
"DisableDRPO": k.DisableDRPO.Load(),
|
|
"KeepFullWGConfig": k.KeepFullWGConfig.Load(),
|
|
"RandomizeClientPort": k.RandomizeClientPort.Load(),
|
|
"OneCGNAT": k.OneCGNAT.Load(),
|
|
"ForceBackgroundSTUN": k.ForceBackgroundSTUN.Load(),
|
|
}
|
|
}
|