mirror of
https://github.com/tailscale/tailscale.git
synced 2025-04-25 02:04:38 +00:00
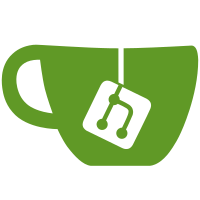
goupnp is an existing upnp client for go, which provides all the functionality we need, licensed under BSD-2-Clause, so we can copy it over and modify parts of it for our case. Specifically, we add contexts to all the methods so we can better handle timeouts, remove the dependency on large charsets, and (eventually) trim out extra components we don't need. Signed-off-by: julianknodt <julianknodt@gmail.com>
65 lines
1.8 KiB
Go
65 lines
1.8 KiB
Go
// Command to generate DCP package source from the XML specification.
|
|
package main
|
|
|
|
import (
|
|
"flag"
|
|
"fmt"
|
|
"log"
|
|
"os"
|
|
"path/filepath"
|
|
)
|
|
|
|
var (
|
|
deviceURNPrefix = "urn:schemas-upnp-org:device:"
|
|
serviceURNPrefix = "urn:schemas-upnp-org:service:"
|
|
)
|
|
|
|
func main() {
|
|
var (
|
|
dcpName = flag.String("dcp_name", "", "Name of the DCP to generate.")
|
|
specsDir = flag.String("specs_dir", ".", "Path to the specification storage directory. "+
|
|
"This is used to find (and download if not present) the specification ZIP files.")
|
|
useGofmt = flag.Bool("gofmt", true, "Pass the generated code through gofmt. "+
|
|
"Disable this if debugging code generation and needing to see the generated code "+
|
|
"prior to being passed through gofmt.")
|
|
)
|
|
flag.Parse()
|
|
|
|
if err := run(*dcpName, *specsDir, *useGofmt); err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
}
|
|
|
|
func run(dcpName, specsDir string, useGofmt bool) error {
|
|
if err := os.MkdirAll(specsDir, os.ModePerm); err != nil {
|
|
return fmt.Errorf("could not create specs-dir %q: %v", specsDir, err)
|
|
}
|
|
|
|
for _, d := range dcpMetadata {
|
|
if d.Name != dcpName {
|
|
continue
|
|
}
|
|
specFilename := filepath.Join(specsDir, d.Name+".zip")
|
|
err := acquireFile(specFilename, d.XMLSpecURL)
|
|
if err != nil {
|
|
return fmt.Errorf("could not acquire spec for %s: %v", d.Name, err)
|
|
}
|
|
dcp := newDCP(d)
|
|
if err := dcp.processZipFile(specFilename); err != nil {
|
|
return fmt.Errorf("error processing spec for %s in file %q: %v", d.Name, specFilename, err)
|
|
}
|
|
for i, hack := range d.Hacks {
|
|
if err := hack(dcp); err != nil {
|
|
return fmt.Errorf("error with Hack[%d] for %s: %v", i, d.Name, err)
|
|
}
|
|
}
|
|
if err := dcp.writeCode(d.Name+".go", useGofmt); err != nil {
|
|
return fmt.Errorf("error writing package %q: %v", dcp.Metadata.Name, err)
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
return fmt.Errorf("could not find DCP with name %q", dcpName)
|
|
}
|