mirror of
https://github.com/tailscale/tailscale.git
synced 2025-02-28 19:27:41 +00:00
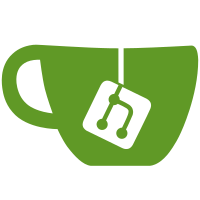
The context carries additional information about the actor, such as the request reason, and is canceled when the actor is done. Additionally, we implement three new ipn.Actor types that wrap other actors to modify their behavior: - WithRequestReason, which adds a request reason to the actor; - WithoutClose, which narrows the actor's interface to prevent it from being closed; - WithPolicyChecks, which adds policy checks to the actor's CheckProfileAccess method. Updates #14823 Signed-off-by: Nick Khyl <nickk@tailscale.com>
49 lines
1.7 KiB
Go
49 lines
1.7 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
package ipnauth
|
|
|
|
import (
|
|
"cmp"
|
|
"context"
|
|
"errors"
|
|
|
|
"tailscale.com/ipn"
|
|
)
|
|
|
|
var _ Actor = (*TestActor)(nil)
|
|
|
|
// TestActor is an [Actor] used exclusively for testing purposes.
|
|
type TestActor struct {
|
|
UID ipn.WindowsUserID // OS-specific UID of the user, if the actor represents a local Windows user
|
|
Name string // username associated with the actor, or ""
|
|
NameErr error // error to be returned by [TestActor.Username]
|
|
CID ClientID // non-zero if the actor represents a connected LocalAPI client
|
|
Ctx context.Context // context associated with the actor
|
|
LocalSystem bool // whether the actor represents the special Local System account on Windows
|
|
LocalAdmin bool // whether the actor has local admin access
|
|
}
|
|
|
|
// UserID implements [Actor].
|
|
func (a *TestActor) UserID() ipn.WindowsUserID { return a.UID }
|
|
|
|
// Username implements [Actor].
|
|
func (a *TestActor) Username() (string, error) { return a.Name, a.NameErr }
|
|
|
|
// ClientID implements [Actor].
|
|
func (a *TestActor) ClientID() (_ ClientID, ok bool) { return a.CID, a.CID != NoClientID }
|
|
|
|
// Context implements [Actor].
|
|
func (a *TestActor) Context() context.Context { return cmp.Or(a.Ctx, context.Background()) }
|
|
|
|
// CheckProfileAccess implements [Actor].
|
|
func (a *TestActor) CheckProfileAccess(profile ipn.LoginProfileView, _ ProfileAccess, _ AuditLogFunc) error {
|
|
return errors.New("profile access denied")
|
|
}
|
|
|
|
// IsLocalSystem implements [Actor].
|
|
func (a *TestActor) IsLocalSystem() bool { return a.LocalSystem }
|
|
|
|
// IsLocalAdmin implements [Actor].
|
|
func (a *TestActor) IsLocalAdmin(operatorUID string) bool { return a.LocalAdmin }
|