mirror of
https://github.com/tailscale/tailscale.git
synced 2025-07-13 09:09:06 +00:00
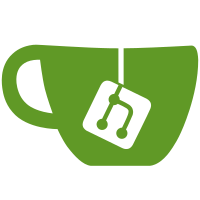
(from patchset 1, 7cdc3c3e7427c9ef69e19224d6036c09c5ea1723, of https://go-review.googlesource.com/c/go/+/229917/1) This will allow building CertPools that consume less memory. (Most certs are never accessed. Different users/programs access different ones, but not many.) This CL only adds the new internal mechanism (and uses it for the old AddCert) but does not modify any existing root pool behavior. (That is, the default Unix roots are still all slurped into memory as of this CL) Change-Id: Ib3a42e4050627b5e34413c595d8ced839c7bfa14
93 lines
2.4 KiB
Go
93 lines
2.4 KiB
Go
// Copyright 2011 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// +build aix dragonfly freebsd js,wasm linux netbsd openbsd solaris
|
|
|
|
package x509
|
|
|
|
import (
|
|
"io/ioutil"
|
|
"os"
|
|
"strings"
|
|
)
|
|
|
|
// Possible directories with certificate files; stop after successfully
|
|
// reading at least one file from a directory.
|
|
var certDirectories = []string{
|
|
"/etc/ssl/certs", // SLES10/SLES11, https://golang.org/issue/12139
|
|
"/system/etc/security/cacerts", // Android
|
|
"/usr/local/share/certs", // FreeBSD
|
|
"/etc/pki/tls/certs", // Fedora/RHEL
|
|
"/etc/openssl/certs", // NetBSD
|
|
"/var/ssl/certs", // AIX
|
|
}
|
|
|
|
const (
|
|
// certFileEnv is the environment variable which identifies where to locate
|
|
// the SSL certificate file. If set this overrides the system default.
|
|
certFileEnv = "SSL_CERT_FILE"
|
|
|
|
// certDirEnv is the environment variable which identifies which directory
|
|
// to check for SSL certificate files. If set this overrides the system default.
|
|
// It is a colon separated list of directories.
|
|
// See https://www.openssl.org/docs/man1.0.2/man1/c_rehash.html.
|
|
certDirEnv = "SSL_CERT_DIR"
|
|
)
|
|
|
|
func (c *Certificate) systemVerify(opts *VerifyOptions) (chains [][]*Certificate, err error) {
|
|
return nil, nil
|
|
}
|
|
|
|
func loadSystemRoots() (*CertPool, error) {
|
|
roots := NewCertPool()
|
|
|
|
files := certFiles
|
|
if f := os.Getenv(certFileEnv); f != "" {
|
|
files = []string{f}
|
|
}
|
|
|
|
var firstErr error
|
|
for _, file := range files {
|
|
data, err := ioutil.ReadFile(file)
|
|
if err == nil {
|
|
roots.AppendCertsFromPEM(data)
|
|
break
|
|
}
|
|
if firstErr == nil && !os.IsNotExist(err) {
|
|
firstErr = err
|
|
}
|
|
}
|
|
|
|
dirs := certDirectories
|
|
if d := os.Getenv(certDirEnv); d != "" {
|
|
// OpenSSL and BoringSSL both use ":" as the SSL_CERT_DIR separator.
|
|
// See:
|
|
// * https://golang.org/issue/35325
|
|
// * https://www.openssl.org/docs/man1.0.2/man1/c_rehash.html
|
|
dirs = strings.Split(d, ":")
|
|
}
|
|
|
|
for _, directory := range dirs {
|
|
fis, err := ioutil.ReadDir(directory)
|
|
if err != nil {
|
|
if firstErr == nil && !os.IsNotExist(err) {
|
|
firstErr = err
|
|
}
|
|
continue
|
|
}
|
|
for _, fi := range fis {
|
|
data, err := ioutil.ReadFile(directory + "/" + fi.Name())
|
|
if err == nil {
|
|
roots.AppendCertsFromPEM(data)
|
|
}
|
|
}
|
|
}
|
|
|
|
if roots.len() > 0 || firstErr == nil {
|
|
return roots, nil
|
|
}
|
|
|
|
return nil, firstErr
|
|
}
|