mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
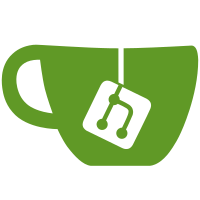
For now this just deletes the net/socks5/tssocks implementation (and the DNSMap stuff from wgengine/netstack) and moves it into net/tsdial. Then initialize a Dialer early in tailscaled, currently only use for the outbound and SOCKS5 proxies. It will be plumbed more later. Notably, it needs to get down into the DNS forwarder for exit node DNS forwading in netstack mode. But it will also absorb all the peerapi setsockopt and netns Dial and tlsdial complexity too. Updates #1713 Change-Id: Ibc6d56ae21a22655b2fa1002d8fc3f2b2ae8b6df Signed-off-by: Brad Fitzpatrick <bradfitz@tailscale.com>
113 lines
2.4 KiB
Go
113 lines
2.4 KiB
Go
// Copyright (c) 2021 Tailscale Inc & AUTHORS All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package tsdial
|
|
|
|
import (
|
|
"reflect"
|
|
"testing"
|
|
|
|
"inet.af/netaddr"
|
|
"tailscale.com/tailcfg"
|
|
"tailscale.com/types/netmap"
|
|
)
|
|
|
|
func TestDNSMapFromNetworkMap(t *testing.T) {
|
|
pfx := netaddr.MustParseIPPrefix
|
|
ip := netaddr.MustParseIP
|
|
tests := []struct {
|
|
name string
|
|
nm *netmap.NetworkMap
|
|
want DNSMap
|
|
}{
|
|
{
|
|
name: "self",
|
|
nm: &netmap.NetworkMap{
|
|
Name: "foo.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100.102.103.104/32"),
|
|
pfx("100::123/128"),
|
|
},
|
|
},
|
|
want: DNSMap{
|
|
"foo": ip("100.102.103.104"),
|
|
"foo.tailnet": ip("100.102.103.104"),
|
|
},
|
|
},
|
|
{
|
|
name: "self_and_peers",
|
|
nm: &netmap.NetworkMap{
|
|
Name: "foo.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100.102.103.104/32"),
|
|
pfx("100::123/128"),
|
|
},
|
|
Peers: []*tailcfg.Node{
|
|
{
|
|
Name: "a.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100.0.0.201/32"),
|
|
pfx("100::201/128"),
|
|
},
|
|
},
|
|
{
|
|
Name: "b.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100::202/128"),
|
|
},
|
|
},
|
|
},
|
|
},
|
|
want: DNSMap{
|
|
"foo": ip("100.102.103.104"),
|
|
"foo.tailnet": ip("100.102.103.104"),
|
|
"a": ip("100.0.0.201"),
|
|
"a.tailnet": ip("100.0.0.201"),
|
|
"b": ip("100::202"),
|
|
"b.tailnet": ip("100::202"),
|
|
},
|
|
},
|
|
{
|
|
name: "self_has_v6_only",
|
|
nm: &netmap.NetworkMap{
|
|
Name: "foo.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100::123/128"),
|
|
},
|
|
Peers: []*tailcfg.Node{
|
|
{
|
|
Name: "a.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100.0.0.201/32"),
|
|
pfx("100::201/128"),
|
|
},
|
|
},
|
|
{
|
|
Name: "b.tailnet",
|
|
Addresses: []netaddr.IPPrefix{
|
|
pfx("100::202/128"),
|
|
},
|
|
},
|
|
},
|
|
},
|
|
want: DNSMap{
|
|
"foo": ip("100::123"),
|
|
"foo.tailnet": ip("100::123"),
|
|
"a": ip("100::201"),
|
|
"a.tailnet": ip("100::201"),
|
|
"b": ip("100::202"),
|
|
"b.tailnet": ip("100::202"),
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got := DNSMapFromNetworkMap(tt.nm)
|
|
if !reflect.DeepEqual(got, tt.want) {
|
|
t.Errorf("mismatch:\n got %v\nwant %v\n", got, tt.want)
|
|
}
|
|
})
|
|
}
|
|
}
|