mirror of
https://github.com/tailscale/tailscale.git
synced 2024-11-25 19:15:34 +00:00
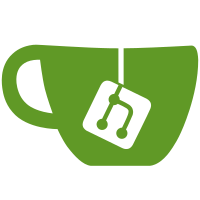
This adds a new bool that can be sent down from control to do jailing on the client side. Previously this would only be done from control by modifying the packet filter we sent down to clients. This would result in a lot of additional work/CPU on control, we could instead just do this on the client. This has always been a TODO which we keep putting off, might as well do it now. Updates tailscale/corp#19623 Signed-off-by: Maisem Ali <maisem@tailscale.com>
81 lines
2.0 KiB
Go
81 lines
2.0 KiB
Go
// Copyright (c) Tailscale Inc & AUTHORS
|
|
// SPDX-License-Identifier: BSD-3-Clause
|
|
|
|
// Code generated by tailscale.com/cmd/cloner; DO NOT EDIT.
|
|
|
|
package wgcfg
|
|
|
|
import (
|
|
"net/netip"
|
|
|
|
"tailscale.com/tailcfg"
|
|
"tailscale.com/types/key"
|
|
"tailscale.com/types/logid"
|
|
"tailscale.com/types/ptr"
|
|
)
|
|
|
|
// Clone makes a deep copy of Config.
|
|
// The result aliases no memory with the original.
|
|
func (src *Config) Clone() *Config {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Config)
|
|
*dst = *src
|
|
dst.Addresses = append(src.Addresses[:0:0], src.Addresses...)
|
|
dst.DNS = append(src.DNS[:0:0], src.DNS...)
|
|
if src.Peers != nil {
|
|
dst.Peers = make([]Peer, len(src.Peers))
|
|
for i := range dst.Peers {
|
|
dst.Peers[i] = *src.Peers[i].Clone()
|
|
}
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _ConfigCloneNeedsRegeneration = Config(struct {
|
|
Name string
|
|
NodeID tailcfg.StableNodeID
|
|
PrivateKey key.NodePrivate
|
|
Addresses []netip.Prefix
|
|
MTU uint16
|
|
DNS []netip.Addr
|
|
Peers []Peer
|
|
NetworkLogging struct {
|
|
NodeID logid.PrivateID
|
|
DomainID logid.PrivateID
|
|
LogExitFlowEnabled bool
|
|
}
|
|
}{})
|
|
|
|
// Clone makes a deep copy of Peer.
|
|
// The result aliases no memory with the original.
|
|
func (src *Peer) Clone() *Peer {
|
|
if src == nil {
|
|
return nil
|
|
}
|
|
dst := new(Peer)
|
|
*dst = *src
|
|
dst.AllowedIPs = append(src.AllowedIPs[:0:0], src.AllowedIPs...)
|
|
if dst.V4MasqAddr != nil {
|
|
dst.V4MasqAddr = ptr.To(*src.V4MasqAddr)
|
|
}
|
|
if dst.V6MasqAddr != nil {
|
|
dst.V6MasqAddr = ptr.To(*src.V6MasqAddr)
|
|
}
|
|
return dst
|
|
}
|
|
|
|
// A compilation failure here means this code must be regenerated, with the command at the top of this file.
|
|
var _PeerCloneNeedsRegeneration = Peer(struct {
|
|
PublicKey key.NodePublic
|
|
DiscoKey key.DiscoPublic
|
|
AllowedIPs []netip.Prefix
|
|
V4MasqAddr *netip.Addr
|
|
V6MasqAddr *netip.Addr
|
|
IsJailed bool
|
|
PersistentKeepalive uint16
|
|
WGEndpoint key.NodePublic
|
|
}{})
|