mirror of
https://github.com/zitadel/zitadel.git
synced 2025-03-01 08:47:23 +00:00
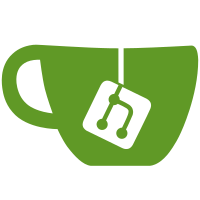
# Which Problems Are Solved The recently introduced notification queue have potential race conditions. # How the Problems Are Solved Current code is refactored to use the queue package, which is safe in regards of concurrency. # Additional Changes - the queue is included in startup - improved code quality of queue # Additional Context - closes https://github.com/zitadel/zitadel/issues/9278
30 lines
1.0 KiB
Go
30 lines
1.0 KiB
Go
package handlers
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
)
|
|
|
|
const NotifyUserID = "NOTIFICATION" //TODO: system?
|
|
|
|
func HandlerContext(event *eventstore.Aggregate) context.Context {
|
|
ctx := authz.WithInstanceID(context.Background(), event.InstanceID)
|
|
return authz.SetCtxData(ctx, authz.CtxData{UserID: NotifyUserID, OrgID: event.ResourceOwner})
|
|
}
|
|
|
|
func ContextWithNotifier(ctx context.Context, aggregate *eventstore.Aggregate) context.Context {
|
|
return authz.WithInstanceID(authz.SetCtxData(ctx, authz.CtxData{UserID: NotifyUserID, OrgID: aggregate.ResourceOwner}), aggregate.InstanceID)
|
|
}
|
|
|
|
func (n *NotificationQueries) HandlerContext(event *eventstore.Aggregate) (context.Context, error) {
|
|
ctx := context.Background()
|
|
instance, err := n.InstanceByID(ctx, event.InstanceID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
ctx = authz.WithInstance(ctx, instance)
|
|
return authz.SetCtxData(ctx, authz.CtxData{UserID: NotifyUserID, OrgID: event.ResourceOwner}), nil
|
|
}
|