mirror of
https://github.com/zitadel/zitadel.git
synced 2025-03-01 02:07:24 +00:00
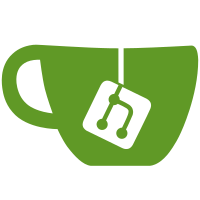
# Which Problems Are Solved Setup fails to push all role permission events when running Zitadel with CockroachDB. `TransactionRetryError`s were visible in logs which finally times out the setup job with `timeout: context deadline exceeded` # How the Problems Are Solved As suggested in the [Cockroach documentation](timeout: context deadline exceeded), _"break down larger transactions"_. The commands to be pushed for the role permissions are chunked in 50 events per push. This chunking is only done with CockroachDB. # Additional Changes - gci run fixed some unrelated imports - access to `command.Commands` for the setup job, so we can reuse the sync logic. # Additional Context Closes #9293 --------- Co-authored-by: Silvan <27845747+adlerhurst@users.noreply.github.com>
140 lines
4.0 KiB
Go
140 lines
4.0 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
"database/sql"
|
|
"database/sql/driver"
|
|
_ "embed"
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/zitadel/zitadel/internal/api/authz"
|
|
"github.com/zitadel/zitadel/internal/database"
|
|
"github.com/zitadel/zitadel/internal/database/mock"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/repository/permission"
|
|
)
|
|
|
|
func Test_rolePermissionMappingsToDatabaseMap(t *testing.T) {
|
|
type args struct {
|
|
mappings []authz.RoleMapping
|
|
system bool
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
args args
|
|
want database.Map[[]string]
|
|
}{
|
|
{
|
|
name: "instance",
|
|
args: args{
|
|
mappings: []authz.RoleMapping{
|
|
{Role: "role1", Permissions: []string{"permission1", "permission2"}},
|
|
{Role: "role2", Permissions: []string{"permission3", "permission4"}},
|
|
{Role: "SYSTEM_ROLE", Permissions: []string{"permission5", "permission6"}},
|
|
},
|
|
system: false,
|
|
},
|
|
want: database.Map[[]string]{
|
|
"role1": []string{"permission1", "permission2"},
|
|
"role2": []string{"permission3", "permission4"},
|
|
},
|
|
},
|
|
{
|
|
name: "system",
|
|
args: args{
|
|
mappings: []authz.RoleMapping{
|
|
{Role: "role1", Permissions: []string{"permission1", "permission2"}},
|
|
{Role: "role2", Permissions: []string{"permission3", "permission4"}},
|
|
{Role: "SYSTEM_ROLE", Permissions: []string{"permission5", "permission6"}},
|
|
},
|
|
system: true,
|
|
},
|
|
want: database.Map[[]string]{
|
|
"SYSTEM_ROLE": []string{"permission5", "permission6"},
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
got := rolePermissionMappingsToDatabaseMap(tt.args.mappings, tt.args.system)
|
|
assert.Equal(t, tt.want, got)
|
|
})
|
|
}
|
|
}
|
|
|
|
func Test_synchronizeRolePermissionCommands(t *testing.T) {
|
|
const aggregateID = "aggregateID"
|
|
aggregate := permission.NewAggregate(aggregateID)
|
|
target := database.Map[[]string]{
|
|
"role1": []string{"permission1", "permission2"},
|
|
"role2": []string{"permission3", "permission4"},
|
|
}
|
|
tests := []struct {
|
|
name string
|
|
mock func(*testing.T) *mock.SQLMock
|
|
wantCmds []eventstore.Command
|
|
wantErr error
|
|
}{
|
|
{
|
|
name: "query error",
|
|
mock: func(t *testing.T) *mock.SQLMock {
|
|
return mock.NewSQLMock(t,
|
|
mock.ExpectQuery(instanceRolePermissionsSyncQuery,
|
|
mock.WithQueryArgs(aggregateID, target),
|
|
mock.WithQueryErr(sql.ErrConnDone),
|
|
),
|
|
)
|
|
},
|
|
wantErr: sql.ErrConnDone,
|
|
},
|
|
{
|
|
name: "no rows",
|
|
mock: func(t *testing.T) *mock.SQLMock {
|
|
return mock.NewSQLMock(t,
|
|
mock.ExpectQuery(instanceRolePermissionsSyncQuery,
|
|
mock.WithQueryArgs(aggregateID, target),
|
|
mock.WithQueryResult([]string{"operation", "role", "permission"}, [][]driver.Value{}),
|
|
),
|
|
)
|
|
},
|
|
},
|
|
{
|
|
name: "add and remove operations",
|
|
mock: func(t *testing.T) *mock.SQLMock {
|
|
return mock.NewSQLMock(t,
|
|
mock.ExpectQuery(instanceRolePermissionsSyncQuery,
|
|
mock.WithQueryArgs(aggregateID, target),
|
|
mock.WithQueryResult([]string{"operation", "role", "permission"}, [][]driver.Value{
|
|
{"add", "role1", "permission1"},
|
|
{"add", "role1", "permission2"},
|
|
{"remove", "role3", "permission5"},
|
|
{"remove", "role3", "permission6"},
|
|
}),
|
|
),
|
|
)
|
|
},
|
|
wantCmds: []eventstore.Command{
|
|
permission.NewAddedEvent(context.Background(), aggregate, "role1", "permission1"),
|
|
permission.NewAddedEvent(context.Background(), aggregate, "role1", "permission2"),
|
|
permission.NewRemovedEvent(context.Background(), aggregate, "role3", "permission5"),
|
|
permission.NewRemovedEvent(context.Background(), aggregate, "role3", "permission6"),
|
|
},
|
|
},
|
|
}
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
mock := tt.mock(t)
|
|
defer mock.Assert(t)
|
|
db := &database.DB{
|
|
DB: mock.DB,
|
|
}
|
|
gotCmds, err := synchronizeRolePermissionCommands(context.Background(), db, aggregateID, target)
|
|
require.ErrorIs(t, err, tt.wantErr)
|
|
assert.Equal(t, tt.wantCmds, gotCmds)
|
|
})
|
|
}
|
|
}
|