mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-13 13:58:32 +00:00
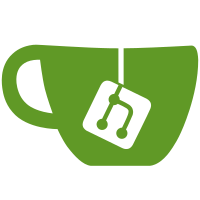
# Which Problems Are Solved The recently introduced notification queue have potential race conditions. # How the Problems Are Solved Current code is refactored to use the queue package, which is safe in regards of concurrency. # Additional Changes - the queue is included in startup - improved code quality of queue # Additional Context - closes https://github.com/zitadel/zitadel/issues/9278
35 lines
1.5 KiB
Go
35 lines
1.5 KiB
Go
package notification
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
)
|
|
|
|
const (
|
|
QueueName = "notification"
|
|
)
|
|
|
|
type Request struct {
|
|
Aggregate *eventstore.Aggregate `json:"aggregate"`
|
|
UserID string `json:"userID"`
|
|
UserResourceOwner string `json:"userResourceOwner"`
|
|
TriggeredAtOrigin string `json:"triggeredAtOrigin"`
|
|
EventType eventstore.EventType `json:"eventType"`
|
|
MessageType string `json:"messageType"`
|
|
NotificationType domain.NotificationType `json:"notificationType"`
|
|
URLTemplate string `json:"urlTemplate,omitempty"`
|
|
CodeExpiry time.Duration `json:"codeExpiry,omitempty"`
|
|
Code *crypto.CryptoValue `json:"code,omitempty"`
|
|
UnverifiedNotificationChannel bool `json:"unverifiedNotificationChannel,omitempty"`
|
|
IsOTP bool `json:"isOTP,omitempty"`
|
|
RequiresPreviousDomain bool `json:"requiresPreviousDomain,omitempty"`
|
|
Args *domain.NotificationArguments `json:"args,omitempty"`
|
|
}
|
|
|
|
func (e *Request) Kind() string {
|
|
return "notification_request"
|
|
}
|