mirror of
https://github.com/zitadel/zitadel.git
synced 2025-01-06 13:07:52 +00:00
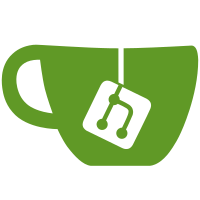
# Which Problems Are Solved Some IdP callbacks use HTTP form POST to return their data on callbacks. For handling CSRF in the login after such calls, a 302 Found to the corresponding non form callback (in ZITADEL) is sent. Depending on the size of the initial form body, this could lead to ZITADEL terminating the connection, resulting in the user not getting a response or an intermediate proxy to return them an HTTP 502. # How the Problems Are Solved - the form body is parsed and stored into the ZITADEL cache (using the configured database by default) - the redirect (302 Found) is performed with the request id - the callback retrieves the data from the cache instead of the query parameters (will fallback to latter to handle open uncached requests) # Additional Changes - fixed a typo in the default (cache) configuration: `LastUsage` -> `LastUseAge` # Additional Context - reported by a customer - needs to be backported to current cloud version (2.66.x) --------- Co-authored-by: Silvan <27845747+adlerhurst@users.noreply.github.com> (cherry picked from commit fa5e590aabda38bd346f1a41484466aebdd8f903)
91 lines
2.6 KiB
Go
91 lines
2.6 KiB
Go
// Code generated by "enumer -type Purpose -transform snake -trimprefix Purpose"; DO NOT EDIT.
|
|
|
|
package cache
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
const _PurposeName = "unspecifiedauthz_instancemilestonesorganizationid_p_form_callback"
|
|
|
|
var _PurposeIndex = [...]uint8{0, 11, 25, 35, 47, 65}
|
|
|
|
const _PurposeLowerName = "unspecifiedauthz_instancemilestonesorganizationid_p_form_callback"
|
|
|
|
func (i Purpose) String() string {
|
|
if i < 0 || i >= Purpose(len(_PurposeIndex)-1) {
|
|
return fmt.Sprintf("Purpose(%d)", i)
|
|
}
|
|
return _PurposeName[_PurposeIndex[i]:_PurposeIndex[i+1]]
|
|
}
|
|
|
|
// An "invalid array index" compiler error signifies that the constant values have changed.
|
|
// Re-run the stringer command to generate them again.
|
|
func _PurposeNoOp() {
|
|
var x [1]struct{}
|
|
_ = x[PurposeUnspecified-(0)]
|
|
_ = x[PurposeAuthzInstance-(1)]
|
|
_ = x[PurposeMilestones-(2)]
|
|
_ = x[PurposeOrganization-(3)]
|
|
_ = x[PurposeIdPFormCallback-(4)]
|
|
}
|
|
|
|
var _PurposeValues = []Purpose{PurposeUnspecified, PurposeAuthzInstance, PurposeMilestones, PurposeOrganization, PurposeIdPFormCallback}
|
|
|
|
var _PurposeNameToValueMap = map[string]Purpose{
|
|
_PurposeName[0:11]: PurposeUnspecified,
|
|
_PurposeLowerName[0:11]: PurposeUnspecified,
|
|
_PurposeName[11:25]: PurposeAuthzInstance,
|
|
_PurposeLowerName[11:25]: PurposeAuthzInstance,
|
|
_PurposeName[25:35]: PurposeMilestones,
|
|
_PurposeLowerName[25:35]: PurposeMilestones,
|
|
_PurposeName[35:47]: PurposeOrganization,
|
|
_PurposeLowerName[35:47]: PurposeOrganization,
|
|
_PurposeName[47:65]: PurposeIdPFormCallback,
|
|
_PurposeLowerName[47:65]: PurposeIdPFormCallback,
|
|
}
|
|
|
|
var _PurposeNames = []string{
|
|
_PurposeName[0:11],
|
|
_PurposeName[11:25],
|
|
_PurposeName[25:35],
|
|
_PurposeName[35:47],
|
|
_PurposeName[47:65],
|
|
}
|
|
|
|
// PurposeString retrieves an enum value from the enum constants string name.
|
|
// Throws an error if the param is not part of the enum.
|
|
func PurposeString(s string) (Purpose, error) {
|
|
if val, ok := _PurposeNameToValueMap[s]; ok {
|
|
return val, nil
|
|
}
|
|
|
|
if val, ok := _PurposeNameToValueMap[strings.ToLower(s)]; ok {
|
|
return val, nil
|
|
}
|
|
return 0, fmt.Errorf("%s does not belong to Purpose values", s)
|
|
}
|
|
|
|
// PurposeValues returns all values of the enum
|
|
func PurposeValues() []Purpose {
|
|
return _PurposeValues
|
|
}
|
|
|
|
// PurposeStrings returns a slice of all String values of the enum
|
|
func PurposeStrings() []string {
|
|
strs := make([]string, len(_PurposeNames))
|
|
copy(strs, _PurposeNames)
|
|
return strs
|
|
}
|
|
|
|
// IsAPurpose returns "true" if the value is listed in the enum definition. "false" otherwise
|
|
func (i Purpose) IsAPurpose() bool {
|
|
for _, v := range _PurposeValues {
|
|
if i == v {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|