mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
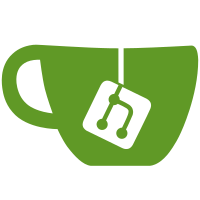
* fix: styling type on idp * fix: google styling * fix: google styling * fix: google styling * fix: remove logo src from angular * fix: pr requests * fix drop column migration * fix: drop column migration * fix: grant id
15213 lines
370 KiB
Go
15213 lines
370 KiB
Go
// Code generated by protoc-gen-validate. DO NOT EDIT.
|
|
// source: management.proto
|
|
|
|
package management
|
|
|
|
import (
|
|
"bytes"
|
|
"errors"
|
|
"fmt"
|
|
"net"
|
|
"net/mail"
|
|
"net/url"
|
|
"regexp"
|
|
"strings"
|
|
"time"
|
|
"unicode/utf8"
|
|
|
|
"github.com/golang/protobuf/ptypes"
|
|
)
|
|
|
|
// ensure the imports are used
|
|
var (
|
|
_ = bytes.MinRead
|
|
_ = errors.New("")
|
|
_ = fmt.Print
|
|
_ = utf8.UTFMax
|
|
_ = (*regexp.Regexp)(nil)
|
|
_ = (*strings.Reader)(nil)
|
|
_ = net.IPv4len
|
|
_ = time.Duration(0)
|
|
_ = (*url.URL)(nil)
|
|
_ = (*mail.Address)(nil)
|
|
_ = ptypes.DynamicAny{}
|
|
)
|
|
|
|
// define the regex for a UUID once up-front
|
|
var _management_uuidPattern = regexp.MustCompile("^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$")
|
|
|
|
// Validate checks the field values on ZitadelDocs with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ZitadelDocs) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Issuer
|
|
|
|
// no validation rules for DiscoveryEndpoint
|
|
|
|
return nil
|
|
}
|
|
|
|
// ZitadelDocsValidationError is the validation error returned by
|
|
// ZitadelDocs.Validate if the designated constraints aren't met.
|
|
type ZitadelDocsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ZitadelDocsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ZitadelDocsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ZitadelDocsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ZitadelDocsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ZitadelDocsValidationError) ErrorName() string { return "ZitadelDocsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ZitadelDocsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sZitadelDocs.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ZitadelDocsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ZitadelDocsValidationError{}
|
|
|
|
// Validate checks the field values on Iam with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Iam) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for GlobalOrgId
|
|
|
|
// no validation rules for IamProjectId
|
|
|
|
// no validation rules for SetUpDone
|
|
|
|
// no validation rules for SetUpStarted
|
|
|
|
return nil
|
|
}
|
|
|
|
// IamValidationError is the validation error returned by Iam.Validate if the
|
|
// designated constraints aren't met.
|
|
type IamValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IamValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IamValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IamValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IamValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IamValidationError) ErrorName() string { return "IamValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IamValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIam.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IamValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IamValidationError{}
|
|
|
|
// Validate checks the field values on ChangeRequest with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ChangeRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for SecId
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for SequenceOffset
|
|
|
|
// no validation rules for Asc
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangeRequestValidationError is the validation error returned by
|
|
// ChangeRequest.Validate if the designated constraints aren't met.
|
|
type ChangeRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangeRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangeRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangeRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangeRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangeRequestValidationError) ErrorName() string { return "ChangeRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangeRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChangeRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangeRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangeRequestValidationError{}
|
|
|
|
// Validate checks the field values on Changes with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Changes) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetChanges() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangesValidationError{
|
|
field: fmt.Sprintf("Changes[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangesValidationError is the validation error returned by Changes.Validate
|
|
// if the designated constraints aren't met.
|
|
type ChangesValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangesValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangesValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangesValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangesValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangesValidationError) ErrorName() string { return "ChangesValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangesValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChanges.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangesValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangesValidationError{}
|
|
|
|
// Validate checks the field values on Change with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Change) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangeValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetEventType()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangeValidationError{
|
|
field: "EventType",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for EditorId
|
|
|
|
// no validation rules for Editor
|
|
|
|
if v, ok := interface{}(m.GetData()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ChangeValidationError{
|
|
field: "Data",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangeValidationError is the validation error returned by Change.Validate if
|
|
// the designated constraints aren't met.
|
|
type ChangeValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangeValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangeValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangeValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangeValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangeValidationError) ErrorName() string { return "ChangeValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangeValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChange.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangeValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangeValidationError{}
|
|
|
|
// Validate checks the field values on ApplicationID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ApplicationID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ApplicationIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ApplicationIDValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationIDValidationError is the validation error returned by
|
|
// ApplicationID.Validate if the designated constraints aren't met.
|
|
type ApplicationIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationIDValidationError) ErrorName() string { return "ApplicationIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplicationID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationIDValidationError{}
|
|
|
|
// Validate checks the field values on ProjectID with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *ProjectID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectIDValidationError is the validation error returned by
|
|
// ProjectID.Validate if the designated constraints aren't met.
|
|
type ProjectIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectIDValidationError) ErrorName() string { return "ProjectIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectIDValidationError{}
|
|
|
|
// Validate checks the field values on UserID with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return UserIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserIDValidationError is the validation error returned by UserID.Validate if
|
|
// the designated constraints aren't met.
|
|
type UserIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserIDValidationError) ErrorName() string { return "UserIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserIDValidationError{}
|
|
|
|
// Validate checks the field values on LoginName with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *LoginName) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetLoginName()) < 1 {
|
|
return LoginNameValidationError{
|
|
field: "LoginName",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// LoginNameValidationError is the validation error returned by
|
|
// LoginName.Validate if the designated constraints aren't met.
|
|
type LoginNameValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e LoginNameValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e LoginNameValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e LoginNameValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e LoginNameValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e LoginNameValidationError) ErrorName() string { return "LoginNameValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e LoginNameValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sLoginName.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = LoginNameValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = LoginNameValidationError{}
|
|
|
|
// Validate checks the field values on UniqueUserRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UniqueUserRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if !_UniqueUserRequest_UserName_Pattern.MatchString(m.GetUserName()) {
|
|
return UniqueUserRequestValidationError{
|
|
field: "UserName",
|
|
reason: "value does not match regex pattern \"^[^[:space:]]{1,200}$\"",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetEmail()); l < 1 || l > 200 {
|
|
return UniqueUserRequestValidationError{
|
|
field: "Email",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UniqueUserRequestValidationError is the validation error returned by
|
|
// UniqueUserRequest.Validate if the designated constraints aren't met.
|
|
type UniqueUserRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UniqueUserRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UniqueUserRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UniqueUserRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UniqueUserRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UniqueUserRequestValidationError) ErrorName() string {
|
|
return "UniqueUserRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UniqueUserRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUniqueUserRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UniqueUserRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UniqueUserRequestValidationError{}
|
|
|
|
var _UniqueUserRequest_UserName_Pattern = regexp.MustCompile("^[^[:space:]]{1,200}$")
|
|
|
|
// Validate checks the field values on UniqueUserResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UniqueUserResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IsUnique
|
|
|
|
return nil
|
|
}
|
|
|
|
// UniqueUserResponseValidationError is the validation error returned by
|
|
// UniqueUserResponse.Validate if the designated constraints aren't met.
|
|
type UniqueUserResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UniqueUserResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UniqueUserResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UniqueUserResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UniqueUserResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UniqueUserResponseValidationError) ErrorName() string {
|
|
return "UniqueUserResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UniqueUserResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUniqueUserResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UniqueUserResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UniqueUserResponseValidationError{}
|
|
|
|
// Validate checks the field values on CreateUserRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *CreateUserRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if !_CreateUserRequest_UserName_Pattern.MatchString(m.GetUserName()) {
|
|
return CreateUserRequestValidationError{
|
|
field: "UserName",
|
|
reason: "value does not match regex pattern \"^[^[:space:]]{1,200}$\"",
|
|
}
|
|
}
|
|
|
|
switch m.User.(type) {
|
|
|
|
case *CreateUserRequest_Human:
|
|
|
|
if v, ok := interface{}(m.GetHuman()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return CreateUserRequestValidationError{
|
|
field: "Human",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
case *CreateUserRequest_Machine:
|
|
|
|
if v, ok := interface{}(m.GetMachine()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return CreateUserRequestValidationError{
|
|
field: "Machine",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
default:
|
|
return CreateUserRequestValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// CreateUserRequestValidationError is the validation error returned by
|
|
// CreateUserRequest.Validate if the designated constraints aren't met.
|
|
type CreateUserRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateUserRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateUserRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateUserRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateUserRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateUserRequestValidationError) ErrorName() string {
|
|
return "CreateUserRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateUserRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateUserRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateUserRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateUserRequestValidationError{}
|
|
|
|
var _CreateUserRequest_UserName_Pattern = regexp.MustCompile("^[^[:space:]]{1,200}$")
|
|
|
|
// Validate checks the field values on CreateHumanRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *CreateHumanRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetFirstName()); l < 1 || l > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "FirstName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetLastName()); l < 1 || l > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "LastName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetNickName()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "NickName",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPreferredLanguage()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "PreferredLanguage",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Gender
|
|
|
|
if l := utf8.RuneCountInString(m.GetEmail()); l < 1 || l > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Email",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if err := m._validateEmail(m.GetEmail()); err != nil {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Email",
|
|
reason: "value must be a valid email address",
|
|
cause: err,
|
|
}
|
|
}
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
if utf8.RuneCountInString(m.GetPhone()) > 20 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Phone",
|
|
reason: "value length must be at most 20 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
if utf8.RuneCountInString(m.GetCountry()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Country",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetLocality()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Locality",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPostalCode()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "PostalCode",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetRegion()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Region",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetStreetAddress()) > 200 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "StreetAddress",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPassword()) > 72 {
|
|
return CreateHumanRequestValidationError{
|
|
field: "Password",
|
|
reason: "value length must be at most 72 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (m *CreateHumanRequest) _validateHostname(host string) error {
|
|
s := strings.ToLower(strings.TrimSuffix(host, "."))
|
|
|
|
if len(host) > 253 {
|
|
return errors.New("hostname cannot exceed 253 characters")
|
|
}
|
|
|
|
for _, part := range strings.Split(s, ".") {
|
|
if l := len(part); l == 0 || l > 63 {
|
|
return errors.New("hostname part must be non-empty and cannot exceed 63 characters")
|
|
}
|
|
|
|
if part[0] == '-' {
|
|
return errors.New("hostname parts cannot begin with hyphens")
|
|
}
|
|
|
|
if part[len(part)-1] == '-' {
|
|
return errors.New("hostname parts cannot end with hyphens")
|
|
}
|
|
|
|
for _, r := range part {
|
|
if (r < 'a' || r > 'z') && (r < '0' || r > '9') && r != '-' {
|
|
return fmt.Errorf("hostname parts can only contain alphanumeric characters or hyphens, got %q", string(r))
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func (m *CreateHumanRequest) _validateEmail(addr string) error {
|
|
a, err := mail.ParseAddress(addr)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
addr = a.Address
|
|
|
|
if len(addr) > 254 {
|
|
return errors.New("email addresses cannot exceed 254 characters")
|
|
}
|
|
|
|
parts := strings.SplitN(addr, "@", 2)
|
|
|
|
if len(parts[0]) > 64 {
|
|
return errors.New("email address local phrase cannot exceed 64 characters")
|
|
}
|
|
|
|
return m._validateHostname(parts[1])
|
|
}
|
|
|
|
// CreateHumanRequestValidationError is the validation error returned by
|
|
// CreateHumanRequest.Validate if the designated constraints aren't met.
|
|
type CreateHumanRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateHumanRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateHumanRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateHumanRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateHumanRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateHumanRequestValidationError) ErrorName() string {
|
|
return "CreateHumanRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateHumanRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateHumanRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateHumanRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateHumanRequestValidationError{}
|
|
|
|
// Validate checks the field values on CreateMachineRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *CreateMachineRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return CreateMachineRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDescription()) > 500 {
|
|
return CreateMachineRequestValidationError{
|
|
field: "Description",
|
|
reason: "value length must be at most 500 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// CreateMachineRequestValidationError is the validation error returned by
|
|
// CreateMachineRequest.Validate if the designated constraints aren't met.
|
|
type CreateMachineRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e CreateMachineRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e CreateMachineRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e CreateMachineRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e CreateMachineRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e CreateMachineRequestValidationError) ErrorName() string {
|
|
return "CreateMachineRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e CreateMachineRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sCreateMachineRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = CreateMachineRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = CreateMachineRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserResponse with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for UserName
|
|
|
|
switch m.User.(type) {
|
|
|
|
case *UserResponse_Human:
|
|
|
|
if v, ok := interface{}(m.GetHuman()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "Human",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
case *UserResponse_Machine:
|
|
|
|
if v, ok := interface{}(m.GetMachine()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserResponseValidationError{
|
|
field: "Machine",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
default:
|
|
return UserResponseValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserResponseValidationError is the validation error returned by
|
|
// UserResponse.Validate if the designated constraints aren't met.
|
|
type UserResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserResponseValidationError) ErrorName() string { return "UserResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for PreferredLoginName
|
|
|
|
if v, ok := interface{}(m.GetLastLogin()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "LastLogin",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for ResourceOwner
|
|
|
|
// no validation rules for UserName
|
|
|
|
switch m.User.(type) {
|
|
|
|
case *UserView_Human:
|
|
|
|
if v, ok := interface{}(m.GetHuman()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "Human",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
case *UserView_Machine:
|
|
|
|
if v, ok := interface{}(m.GetMachine()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserViewValidationError{
|
|
field: "Machine",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
default:
|
|
return UserViewValidationError{
|
|
field: "User",
|
|
reason: "value is required",
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserViewValidationError is the validation error returned by
|
|
// UserView.Validate if the designated constraints aren't met.
|
|
type UserViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserViewValidationError) ErrorName() string { return "UserViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserViewValidationError{}
|
|
|
|
// Validate checks the field values on HumanResponse with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *HumanResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
return nil
|
|
}
|
|
|
|
// HumanResponseValidationError is the validation error returned by
|
|
// HumanResponse.Validate if the designated constraints aren't met.
|
|
type HumanResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e HumanResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e HumanResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e HumanResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e HumanResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e HumanResponseValidationError) ErrorName() string { return "HumanResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e HumanResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sHumanResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = HumanResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = HumanResponseValidationError{}
|
|
|
|
// Validate checks the field values on HumanView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *HumanView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetPasswordChanged()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return HumanViewValidationError{
|
|
field: "PasswordChanged",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
return nil
|
|
}
|
|
|
|
// HumanViewValidationError is the validation error returned by
|
|
// HumanView.Validate if the designated constraints aren't met.
|
|
type HumanViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e HumanViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e HumanViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e HumanViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e HumanViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e HumanViewValidationError) ErrorName() string { return "HumanViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e HumanViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sHumanView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = HumanViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = HumanViewValidationError{}
|
|
|
|
// Validate checks the field values on MachineResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *MachineResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Description
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineResponseValidationError is the validation error returned by
|
|
// MachineResponse.Validate if the designated constraints aren't met.
|
|
type MachineResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineResponseValidationError) ErrorName() string { return "MachineResponseValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineResponseValidationError{}
|
|
|
|
// Validate checks the field values on MachineView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MachineView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetLastKeyAdded()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineViewValidationError{
|
|
field: "LastKeyAdded",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Description
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineViewValidationError is the validation error returned by
|
|
// MachineView.Validate if the designated constraints aren't met.
|
|
type MachineViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineViewValidationError) ErrorName() string { return "MachineViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateMachineRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateMachineRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return UpdateMachineRequestValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDescription()) > 500 {
|
|
return UpdateMachineRequestValidationError{
|
|
field: "Description",
|
|
reason: "value length must be at most 500 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateMachineRequestValidationError is the validation error returned by
|
|
// UpdateMachineRequest.Validate if the designated constraints aren't met.
|
|
type UpdateMachineRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateMachineRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateMachineRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateMachineRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateMachineRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateMachineRequestValidationError) ErrorName() string {
|
|
return "UpdateMachineRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateMachineRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateMachineRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateMachineRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateMachineRequestValidationError{}
|
|
|
|
// Validate checks the field values on AddMachineKeyRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *AddMachineKeyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return AddMachineKeyRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if _, ok := _AddMachineKeyRequest_Type_NotInLookup[m.GetType()]; ok {
|
|
return AddMachineKeyRequestValidationError{
|
|
field: "Type",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetExpirationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return AddMachineKeyRequestValidationError{
|
|
field: "ExpirationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// AddMachineKeyRequestValidationError is the validation error returned by
|
|
// AddMachineKeyRequest.Validate if the designated constraints aren't met.
|
|
type AddMachineKeyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e AddMachineKeyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e AddMachineKeyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e AddMachineKeyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e AddMachineKeyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e AddMachineKeyRequestValidationError) ErrorName() string {
|
|
return "AddMachineKeyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e AddMachineKeyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sAddMachineKeyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = AddMachineKeyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = AddMachineKeyRequestValidationError{}
|
|
|
|
var _AddMachineKeyRequest_Type_NotInLookup = map[MachineKeyType]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on AddMachineKeyResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *AddMachineKeyResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return AddMachineKeyResponseValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for Type
|
|
|
|
if v, ok := interface{}(m.GetExpirationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return AddMachineKeyResponseValidationError{
|
|
field: "ExpirationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for KeyDetails
|
|
|
|
return nil
|
|
}
|
|
|
|
// AddMachineKeyResponseValidationError is the validation error returned by
|
|
// AddMachineKeyResponse.Validate if the designated constraints aren't met.
|
|
type AddMachineKeyResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e AddMachineKeyResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e AddMachineKeyResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e AddMachineKeyResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e AddMachineKeyResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e AddMachineKeyResponseValidationError) ErrorName() string {
|
|
return "AddMachineKeyResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e AddMachineKeyResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sAddMachineKeyResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = AddMachineKeyResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = AddMachineKeyResponseValidationError{}
|
|
|
|
// Validate checks the field values on MachineKeyIDRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MachineKeyIDRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return MachineKeyIDRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetKeyId()) < 1 {
|
|
return MachineKeyIDRequestValidationError{
|
|
field: "KeyId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineKeyIDRequestValidationError is the validation error returned by
|
|
// MachineKeyIDRequest.Validate if the designated constraints aren't met.
|
|
type MachineKeyIDRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineKeyIDRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineKeyIDRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineKeyIDRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineKeyIDRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineKeyIDRequestValidationError) ErrorName() string {
|
|
return "MachineKeyIDRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineKeyIDRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineKeyIDRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineKeyIDRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineKeyIDRequestValidationError{}
|
|
|
|
// Validate checks the field values on MachineKeyView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MachineKeyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Type
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetExpirationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeyViewValidationError{
|
|
field: "ExpirationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineKeyViewValidationError is the validation error returned by
|
|
// MachineKeyView.Validate if the designated constraints aren't met.
|
|
type MachineKeyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineKeyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineKeyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineKeyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineKeyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineKeyViewValidationError) ErrorName() string { return "MachineKeyViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineKeyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineKeyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineKeyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineKeyViewValidationError{}
|
|
|
|
// Validate checks the field values on MachineKeySearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MachineKeySearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for Asc
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return MachineKeySearchRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineKeySearchRequestValidationError is the validation error returned by
|
|
// MachineKeySearchRequest.Validate if the designated constraints aren't met.
|
|
type MachineKeySearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineKeySearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineKeySearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineKeySearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineKeySearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineKeySearchRequestValidationError) ErrorName() string {
|
|
return "MachineKeySearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineKeySearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineKeySearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineKeySearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineKeySearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on MachineKeySearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *MachineKeySearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeySearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MachineKeySearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MachineKeySearchResponseValidationError is the validation error returned by
|
|
// MachineKeySearchResponse.Validate if the designated constraints aren't met.
|
|
type MachineKeySearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MachineKeySearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MachineKeySearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MachineKeySearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MachineKeySearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MachineKeySearchResponseValidationError) ErrorName() string {
|
|
return "MachineKeySearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MachineKeySearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMachineKeySearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MachineKeySearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MachineKeySearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserSearchRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for SortingColumn
|
|
|
|
// no validation rules for Asc
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserSearchRequestValidationError is the validation error returned by
|
|
// UserSearchRequest.Validate if the designated constraints aren't met.
|
|
type UserSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserSearchRequestValidationError) ErrorName() string {
|
|
return "UserSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserSearchQuery with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _UserSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return UserSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserSearchQueryValidationError is the validation error returned by
|
|
// UserSearchQuery.Validate if the designated constraints aren't met.
|
|
type UserSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserSearchQueryValidationError) ErrorName() string { return "UserSearchQueryValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserSearchQueryValidationError{}
|
|
|
|
var _UserSearchQuery_Key_NotInLookup = map[UserSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on UserSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserSearchResponseValidationError is the validation error returned by
|
|
// UserSearchResponse.Validate if the designated constraints aren't met.
|
|
type UserSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserSearchResponseValidationError) ErrorName() string {
|
|
return "UserSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserProfile with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserProfile) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserProfileValidationError is the validation error returned by
|
|
// UserProfile.Validate if the designated constraints aren't met.
|
|
type UserProfileValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserProfileValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserProfileValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserProfileValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserProfileValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserProfileValidationError) ErrorName() string { return "UserProfileValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserProfileValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserProfile.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserProfileValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserProfileValidationError{}
|
|
|
|
// Validate checks the field values on UserProfileView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserProfileView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for NickName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for PreferredLanguage
|
|
|
|
// no validation rules for Gender
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserProfileViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for PreferredLoginName
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserProfileViewValidationError is the validation error returned by
|
|
// UserProfileView.Validate if the designated constraints aren't met.
|
|
type UserProfileViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserProfileViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserProfileViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserProfileViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserProfileViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserProfileViewValidationError) ErrorName() string { return "UserProfileViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserProfileViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserProfileView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserProfileViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserProfileViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserProfileRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserProfileRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
if l := utf8.RuneCountInString(m.GetFirstName()); l < 1 || l > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "FirstName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetLastName()); l < 1 || l > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "LastName",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetNickName()) > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "NickName",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPreferredLanguage()) > 200 {
|
|
return UpdateUserProfileRequestValidationError{
|
|
field: "PreferredLanguage",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Gender
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserProfileRequestValidationError is the validation error returned by
|
|
// UpdateUserProfileRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserProfileRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserProfileRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserProfileRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserProfileRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserProfileRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserProfileRequestValidationError) ErrorName() string {
|
|
return "UpdateUserProfileRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserProfileRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserProfileRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserProfileRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserProfileRequestValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserUserNameRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserUserNameRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
if !_UpdateUserUserNameRequest_UserName_Pattern.MatchString(m.GetUserName()) {
|
|
return UpdateUserUserNameRequestValidationError{
|
|
field: "UserName",
|
|
reason: "value does not match regex pattern \"^[^[:space:]]{1,200}$\"",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserUserNameRequestValidationError is the validation error returned by
|
|
// UpdateUserUserNameRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserUserNameRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserUserNameRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserUserNameRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserUserNameRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserUserNameRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserUserNameRequestValidationError) ErrorName() string {
|
|
return "UpdateUserUserNameRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserUserNameRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserUserNameRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserUserNameRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserUserNameRequestValidationError{}
|
|
|
|
var _UpdateUserUserNameRequest_UserName_Pattern = regexp.MustCompile("^[^[:space:]]{1,200}$")
|
|
|
|
// Validate checks the field values on UserEmail with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserEmail) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserEmailValidationError is the validation error returned by
|
|
// UserEmail.Validate if the designated constraints aren't met.
|
|
type UserEmailValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserEmailValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserEmailValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserEmailValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserEmailValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserEmailValidationError) ErrorName() string { return "UserEmailValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserEmailValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserEmail.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserEmailValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserEmailValidationError{}
|
|
|
|
// Validate checks the field values on UserEmailView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserEmailView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserEmailViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserEmailViewValidationError is the validation error returned by
|
|
// UserEmailView.Validate if the designated constraints aren't met.
|
|
type UserEmailViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserEmailViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserEmailViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserEmailViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserEmailViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserEmailViewValidationError) ErrorName() string { return "UserEmailViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserEmailViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserEmailView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserEmailViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserEmailViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserEmailRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserEmailRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
if l := utf8.RuneCountInString(m.GetEmail()); l < 1 || l > 200 {
|
|
return UpdateUserEmailRequestValidationError{
|
|
field: "Email",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IsEmailVerified
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserEmailRequestValidationError is the validation error returned by
|
|
// UpdateUserEmailRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserEmailRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserEmailRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserEmailRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserEmailRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserEmailRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserEmailRequestValidationError) ErrorName() string {
|
|
return "UpdateUserEmailRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserEmailRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserEmailRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserEmailRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserEmailRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserPhone with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserPhone) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserPhoneValidationError is the validation error returned by
|
|
// UserPhone.Validate if the designated constraints aren't met.
|
|
type UserPhoneValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserPhoneValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserPhoneValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserPhoneValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserPhoneValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserPhoneValidationError) ErrorName() string { return "UserPhoneValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserPhoneValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserPhone.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserPhoneValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserPhoneValidationError{}
|
|
|
|
// Validate checks the field values on UserPhoneView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserPhoneView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Phone
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserPhoneViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserPhoneViewValidationError is the validation error returned by
|
|
// UserPhoneView.Validate if the designated constraints aren't met.
|
|
type UserPhoneViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserPhoneViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserPhoneViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserPhoneViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserPhoneViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserPhoneViewValidationError) ErrorName() string { return "UserPhoneViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserPhoneViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserPhoneView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserPhoneViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserPhoneViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserPhoneRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserPhoneRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return UpdateUserPhoneRequestValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetPhone()); l < 1 || l > 20 {
|
|
return UpdateUserPhoneRequestValidationError{
|
|
field: "Phone",
|
|
reason: "value length must be between 1 and 20 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IsPhoneVerified
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserPhoneRequestValidationError is the validation error returned by
|
|
// UpdateUserPhoneRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserPhoneRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserPhoneRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserPhoneRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserPhoneRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserPhoneRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserPhoneRequestValidationError) ErrorName() string {
|
|
return "UpdateUserPhoneRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserPhoneRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserPhoneRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserPhoneRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserPhoneRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserAddress with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserAddress) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserAddressValidationError is the validation error returned by
|
|
// UserAddress.Validate if the designated constraints aren't met.
|
|
type UserAddressValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserAddressValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserAddressValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserAddressValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserAddressValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserAddressValidationError) ErrorName() string { return "UserAddressValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserAddressValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserAddress.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserAddressValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserAddressValidationError{}
|
|
|
|
// Validate checks the field values on UserAddressView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserAddressView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Country
|
|
|
|
// no validation rules for Locality
|
|
|
|
// no validation rules for PostalCode
|
|
|
|
// no validation rules for Region
|
|
|
|
// no validation rules for StreetAddress
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserAddressViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserAddressViewValidationError is the validation error returned by
|
|
// UserAddressView.Validate if the designated constraints aren't met.
|
|
type UserAddressViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserAddressViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserAddressViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserAddressViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserAddressViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserAddressViewValidationError) ErrorName() string { return "UserAddressViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserAddressViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserAddressView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserAddressViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserAddressViewValidationError{}
|
|
|
|
// Validate checks the field values on UpdateUserAddressRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UpdateUserAddressRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetCountry()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Country",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetLocality()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Locality",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetPostalCode()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "PostalCode",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetRegion()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "Region",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetStreetAddress()) > 200 {
|
|
return UpdateUserAddressRequestValidationError{
|
|
field: "StreetAddress",
|
|
reason: "value length must be at most 200 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UpdateUserAddressRequestValidationError is the validation error returned by
|
|
// UpdateUserAddressRequest.Validate if the designated constraints aren't met.
|
|
type UpdateUserAddressRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UpdateUserAddressRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UpdateUserAddressRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UpdateUserAddressRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UpdateUserAddressRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UpdateUserAddressRequestValidationError) ErrorName() string {
|
|
return "UpdateUserAddressRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UpdateUserAddressRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUpdateUserAddressRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UpdateUserAddressRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UpdateUserAddressRequestValidationError{}
|
|
|
|
// Validate checks the field values on MultiFactors with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MultiFactors) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetMfas() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return MultiFactorsValidationError{
|
|
field: fmt.Sprintf("Mfas[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// MultiFactorsValidationError is the validation error returned by
|
|
// MultiFactors.Validate if the designated constraints aren't met.
|
|
type MultiFactorsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MultiFactorsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MultiFactorsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MultiFactorsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MultiFactorsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MultiFactorsValidationError) ErrorName() string { return "MultiFactorsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MultiFactorsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMultiFactors.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MultiFactorsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MultiFactorsValidationError{}
|
|
|
|
// Validate checks the field values on MultiFactor with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *MultiFactor) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Type
|
|
|
|
// no validation rules for State
|
|
|
|
return nil
|
|
}
|
|
|
|
// MultiFactorValidationError is the validation error returned by
|
|
// MultiFactor.Validate if the designated constraints aren't met.
|
|
type MultiFactorValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e MultiFactorValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e MultiFactorValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e MultiFactorValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e MultiFactorValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e MultiFactorValidationError) ErrorName() string { return "MultiFactorValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e MultiFactorValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sMultiFactor.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = MultiFactorValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = MultiFactorValidationError{}
|
|
|
|
// Validate checks the field values on PasswordRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *PasswordRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return PasswordRequestValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetPassword()); l < 1 || l > 72 {
|
|
return PasswordRequestValidationError{
|
|
field: "Password",
|
|
reason: "value length must be between 1 and 72 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordRequestValidationError is the validation error returned by
|
|
// PasswordRequest.Validate if the designated constraints aren't met.
|
|
type PasswordRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordRequestValidationError) ErrorName() string { return "PasswordRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordRequestValidationError{}
|
|
|
|
// Validate checks the field values on SetPasswordNotificationRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *SetPasswordNotificationRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return SetPasswordNotificationRequestValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Type
|
|
|
|
return nil
|
|
}
|
|
|
|
// SetPasswordNotificationRequestValidationError is the validation error
|
|
// returned by SetPasswordNotificationRequest.Validate if the designated
|
|
// constraints aren't met.
|
|
type SetPasswordNotificationRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e SetPasswordNotificationRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e SetPasswordNotificationRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e SetPasswordNotificationRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e SetPasswordNotificationRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e SetPasswordNotificationRequestValidationError) ErrorName() string {
|
|
return "SetPasswordNotificationRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e SetPasswordNotificationRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sSetPasswordNotificationRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = SetPasswordNotificationRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = SetPasswordNotificationRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgIamPolicyView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgIamPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserLoginMustBeDomain
|
|
|
|
// no validation rules for Default
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgIamPolicyViewValidationError is the validation error returned by
|
|
// OrgIamPolicyView.Validate if the designated constraints aren't met.
|
|
type OrgIamPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgIamPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgIamPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgIamPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgIamPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgIamPolicyViewValidationError) ErrorName() string { return "OrgIamPolicyViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgIamPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgIamPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgIamPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgIamPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on OrgCreateRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OrgCreateRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return OrgCreateRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgCreateRequestValidationError is the validation error returned by
|
|
// OrgCreateRequest.Validate if the designated constraints aren't met.
|
|
type OrgCreateRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgCreateRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgCreateRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgCreateRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgCreateRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgCreateRequestValidationError) ErrorName() string { return "OrgCreateRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgCreateRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgCreateRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgCreateRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgCreateRequestValidationError{}
|
|
|
|
// Validate checks the field values on Org with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Org) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgValidationError is the validation error returned by Org.Validate if the
|
|
// designated constraints aren't met.
|
|
type OrgValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgValidationError) ErrorName() string { return "OrgValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrg.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgValidationError{}
|
|
|
|
// Validate checks the field values on OrgView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *OrgView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgViewValidationError is the validation error returned by OrgView.Validate
|
|
// if the designated constraints aren't met.
|
|
type OrgViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgViewValidationError) ErrorName() string { return "OrgViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgViewValidationError{}
|
|
|
|
// Validate checks the field values on Domain with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Domain) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDomain()) < 1 {
|
|
return DomainValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// DomainValidationError is the validation error returned by Domain.Validate if
|
|
// the designated constraints aren't met.
|
|
type DomainValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e DomainValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e DomainValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e DomainValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e DomainValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e DomainValidationError) ErrorName() string { return "DomainValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e DomainValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sDomain.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = DomainValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = DomainValidationError{}
|
|
|
|
// Validate checks the field values on OrgDomain with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *OrgDomain) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for OrgId
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Domain
|
|
|
|
// no validation rules for Verified
|
|
|
|
// no validation rules for Primary
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainValidationError is the validation error returned by
|
|
// OrgDomain.Validate if the designated constraints aren't met.
|
|
type OrgDomainValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainValidationError) ErrorName() string { return "OrgDomainValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomain.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainValidationError{}
|
|
|
|
// Validate checks the field values on OrgDomainView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OrgDomainView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for OrgId
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Domain
|
|
|
|
// no validation rules for Verified
|
|
|
|
// no validation rules for Primary
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for ValidationType
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainViewValidationError is the validation error returned by
|
|
// OrgDomainView.Validate if the designated constraints aren't met.
|
|
type OrgDomainViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainViewValidationError) ErrorName() string { return "OrgDomainViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomainView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainViewValidationError{}
|
|
|
|
// Validate checks the field values on AddOrgDomainRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *AddOrgDomainRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetDomain()); l < 1 || l > 200 {
|
|
return AddOrgDomainRequestValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// AddOrgDomainRequestValidationError is the validation error returned by
|
|
// AddOrgDomainRequest.Validate if the designated constraints aren't met.
|
|
type AddOrgDomainRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e AddOrgDomainRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e AddOrgDomainRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e AddOrgDomainRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e AddOrgDomainRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e AddOrgDomainRequestValidationError) ErrorName() string {
|
|
return "AddOrgDomainRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e AddOrgDomainRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sAddOrgDomainRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = AddOrgDomainRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = AddOrgDomainRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgDomainValidationRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgDomainValidationRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetDomain()); l < 1 || l > 200 {
|
|
return OrgDomainValidationRequestValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if _, ok := _OrgDomainValidationRequest_Type_NotInLookup[m.GetType()]; ok {
|
|
return OrgDomainValidationRequestValidationError{
|
|
field: "Type",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainValidationRequestValidationError is the validation error returned
|
|
// by OrgDomainValidationRequest.Validate if the designated constraints aren't met.
|
|
type OrgDomainValidationRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainValidationRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainValidationRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainValidationRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainValidationRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainValidationRequestValidationError) ErrorName() string {
|
|
return "OrgDomainValidationRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainValidationRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomainValidationRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainValidationRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainValidationRequestValidationError{}
|
|
|
|
var _OrgDomainValidationRequest_Type_NotInLookup = map[OrgDomainValidationType]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on OrgDomainValidationResponse with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgDomainValidationResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Token
|
|
|
|
// no validation rules for Url
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainValidationResponseValidationError is the validation error returned
|
|
// by OrgDomainValidationResponse.Validate if the designated constraints
|
|
// aren't met.
|
|
type OrgDomainValidationResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainValidationResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainValidationResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainValidationResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainValidationResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainValidationResponseValidationError) ErrorName() string {
|
|
return "OrgDomainValidationResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainValidationResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomainValidationResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainValidationResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainValidationResponseValidationError{}
|
|
|
|
// Validate checks the field values on ValidateOrgDomainRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ValidateOrgDomainRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetDomain()); l < 1 || l > 200 {
|
|
return ValidateOrgDomainRequestValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ValidateOrgDomainRequestValidationError is the validation error returned by
|
|
// ValidateOrgDomainRequest.Validate if the designated constraints aren't met.
|
|
type ValidateOrgDomainRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ValidateOrgDomainRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ValidateOrgDomainRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ValidateOrgDomainRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ValidateOrgDomainRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ValidateOrgDomainRequestValidationError) ErrorName() string {
|
|
return "ValidateOrgDomainRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ValidateOrgDomainRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sValidateOrgDomainRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ValidateOrgDomainRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ValidateOrgDomainRequestValidationError{}
|
|
|
|
// Validate checks the field values on PrimaryOrgDomainRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PrimaryOrgDomainRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDomain()) < 1 {
|
|
return PrimaryOrgDomainRequestValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PrimaryOrgDomainRequestValidationError is the validation error returned by
|
|
// PrimaryOrgDomainRequest.Validate if the designated constraints aren't met.
|
|
type PrimaryOrgDomainRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PrimaryOrgDomainRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PrimaryOrgDomainRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PrimaryOrgDomainRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PrimaryOrgDomainRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PrimaryOrgDomainRequestValidationError) ErrorName() string {
|
|
return "PrimaryOrgDomainRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PrimaryOrgDomainRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPrimaryOrgDomainRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PrimaryOrgDomainRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PrimaryOrgDomainRequestValidationError{}
|
|
|
|
// Validate checks the field values on RemoveOrgDomainRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *RemoveOrgDomainRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetDomain()) < 1 {
|
|
return RemoveOrgDomainRequestValidationError{
|
|
field: "Domain",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// RemoveOrgDomainRequestValidationError is the validation error returned by
|
|
// RemoveOrgDomainRequest.Validate if the designated constraints aren't met.
|
|
type RemoveOrgDomainRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e RemoveOrgDomainRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e RemoveOrgDomainRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e RemoveOrgDomainRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e RemoveOrgDomainRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e RemoveOrgDomainRequestValidationError) ErrorName() string {
|
|
return "RemoveOrgDomainRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e RemoveOrgDomainRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sRemoveOrgDomainRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = RemoveOrgDomainRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = RemoveOrgDomainRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgDomainSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgDomainSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainSearchResponseValidationError is the validation error returned by
|
|
// OrgDomainSearchResponse.Validate if the designated constraints aren't met.
|
|
type OrgDomainSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainSearchResponseValidationError) ErrorName() string {
|
|
return "OrgDomainSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomainSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on OrgDomainSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgDomainSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgDomainSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainSearchRequestValidationError is the validation error returned by
|
|
// OrgDomainSearchRequest.Validate if the designated constraints aren't met.
|
|
type OrgDomainSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainSearchRequestValidationError) ErrorName() string {
|
|
return "OrgDomainSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomainSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgDomainSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgDomainSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _OrgDomainSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return OrgDomainSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgDomainSearchQueryValidationError is the validation error returned by
|
|
// OrgDomainSearchQuery.Validate if the designated constraints aren't met.
|
|
type OrgDomainSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgDomainSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgDomainSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgDomainSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgDomainSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgDomainSearchQueryValidationError) ErrorName() string {
|
|
return "OrgDomainSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgDomainSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgDomainSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgDomainSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgDomainSearchQueryValidationError{}
|
|
|
|
var _OrgDomainSearchQuery_Key_NotInLookup = map[OrgDomainSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on OrgMemberRoles with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OrgMemberRoles) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgMemberRolesValidationError is the validation error returned by
|
|
// OrgMemberRoles.Validate if the designated constraints aren't met.
|
|
type OrgMemberRolesValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgMemberRolesValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgMemberRolesValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgMemberRolesValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgMemberRolesValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgMemberRolesValidationError) ErrorName() string { return "OrgMemberRolesValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgMemberRolesValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgMemberRoles.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgMemberRolesValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgMemberRolesValidationError{}
|
|
|
|
// Validate checks the field values on OrgMember with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *OrgMember) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgMemberValidationError is the validation error returned by
|
|
// OrgMember.Validate if the designated constraints aren't met.
|
|
type OrgMemberValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgMemberValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgMemberValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgMemberValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgMemberValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgMemberValidationError) ErrorName() string { return "OrgMemberValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgMemberValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgMember.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgMemberValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgMemberValidationError{}
|
|
|
|
// Validate checks the field values on AddOrgMemberRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *AddOrgMemberRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return AddOrgMemberRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// AddOrgMemberRequestValidationError is the validation error returned by
|
|
// AddOrgMemberRequest.Validate if the designated constraints aren't met.
|
|
type AddOrgMemberRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e AddOrgMemberRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e AddOrgMemberRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e AddOrgMemberRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e AddOrgMemberRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e AddOrgMemberRequestValidationError) ErrorName() string {
|
|
return "AddOrgMemberRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e AddOrgMemberRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sAddOrgMemberRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = AddOrgMemberRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = AddOrgMemberRequestValidationError{}
|
|
|
|
// Validate checks the field values on ChangeOrgMemberRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ChangeOrgMemberRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ChangeOrgMemberRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ChangeOrgMemberRequestValidationError is the validation error returned by
|
|
// ChangeOrgMemberRequest.Validate if the designated constraints aren't met.
|
|
type ChangeOrgMemberRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ChangeOrgMemberRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ChangeOrgMemberRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ChangeOrgMemberRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ChangeOrgMemberRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ChangeOrgMemberRequestValidationError) ErrorName() string {
|
|
return "ChangeOrgMemberRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ChangeOrgMemberRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sChangeOrgMemberRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ChangeOrgMemberRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ChangeOrgMemberRequestValidationError{}
|
|
|
|
// Validate checks the field values on RemoveOrgMemberRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *RemoveOrgMemberRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return RemoveOrgMemberRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// RemoveOrgMemberRequestValidationError is the validation error returned by
|
|
// RemoveOrgMemberRequest.Validate if the designated constraints aren't met.
|
|
type RemoveOrgMemberRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e RemoveOrgMemberRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e RemoveOrgMemberRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e RemoveOrgMemberRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e RemoveOrgMemberRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e RemoveOrgMemberRequestValidationError) ErrorName() string {
|
|
return "RemoveOrgMemberRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e RemoveOrgMemberRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sRemoveOrgMemberRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = RemoveOrgMemberRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = RemoveOrgMemberRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgMemberSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgMemberSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgMemberSearchResponseValidationError is the validation error returned by
|
|
// OrgMemberSearchResponse.Validate if the designated constraints aren't met.
|
|
type OrgMemberSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgMemberSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgMemberSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgMemberSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgMemberSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgMemberSearchResponseValidationError) ErrorName() string {
|
|
return "OrgMemberSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgMemberSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgMemberSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgMemberSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgMemberSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on OrgMemberView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OrgMemberView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for UserName
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgMemberViewValidationError is the validation error returned by
|
|
// OrgMemberView.Validate if the designated constraints aren't met.
|
|
type OrgMemberViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgMemberViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgMemberViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgMemberViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgMemberViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgMemberViewValidationError) ErrorName() string { return "OrgMemberViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgMemberViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgMemberView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgMemberViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgMemberViewValidationError{}
|
|
|
|
// Validate checks the field values on OrgMemberSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgMemberSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OrgMemberSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgMemberSearchRequestValidationError is the validation error returned by
|
|
// OrgMemberSearchRequest.Validate if the designated constraints aren't met.
|
|
type OrgMemberSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgMemberSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgMemberSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgMemberSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgMemberSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgMemberSearchRequestValidationError) ErrorName() string {
|
|
return "OrgMemberSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgMemberSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgMemberSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgMemberSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgMemberSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on OrgMemberSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OrgMemberSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _OrgMemberSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return OrgMemberSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// OrgMemberSearchQueryValidationError is the validation error returned by
|
|
// OrgMemberSearchQuery.Validate if the designated constraints aren't met.
|
|
type OrgMemberSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OrgMemberSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OrgMemberSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OrgMemberSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OrgMemberSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OrgMemberSearchQueryValidationError) ErrorName() string {
|
|
return "OrgMemberSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OrgMemberSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOrgMemberSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OrgMemberSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OrgMemberSearchQueryValidationError{}
|
|
|
|
var _OrgMemberSearchQuery_Key_NotInLookup = map[OrgMemberSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on ProjectCreateRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectCreateRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return ProjectCreateRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for ProjectRoleAssertion
|
|
|
|
// no validation rules for ProjectRoleCheck
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectCreateRequestValidationError is the validation error returned by
|
|
// ProjectCreateRequest.Validate if the designated constraints aren't met.
|
|
type ProjectCreateRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectCreateRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectCreateRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectCreateRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectCreateRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectCreateRequestValidationError) ErrorName() string {
|
|
return "ProjectCreateRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectCreateRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectCreateRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectCreateRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectCreateRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectUpdateRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectUpdateRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectUpdateRequestValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return ProjectUpdateRequestValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for ProjectRoleAssertion
|
|
|
|
// no validation rules for ProjectRoleCheck
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectUpdateRequestValidationError is the validation error returned by
|
|
// ProjectUpdateRequest.Validate if the designated constraints aren't met.
|
|
type ProjectUpdateRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectUpdateRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectUpdateRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectUpdateRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectUpdateRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectUpdateRequestValidationError) ErrorName() string {
|
|
return "ProjectUpdateRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectUpdateRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectUpdateRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectUpdateRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectUpdateRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectSearchResponseValidationError is the validation error returned by
|
|
// ProjectSearchResponse.Validate if the designated constraints aren't met.
|
|
type ProjectSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectSearchResponseValidationError) ErrorName() string {
|
|
return "ProjectSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ProjectView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ProjectView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for ResourceOwner
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for ProjectRoleAssertion
|
|
|
|
// no validation rules for ProjectRoleCheck
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectViewValidationError is the validation error returned by
|
|
// ProjectView.Validate if the designated constraints aren't met.
|
|
type ProjectViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectViewValidationError) ErrorName() string { return "ProjectViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectViewValidationError{}
|
|
|
|
// Validate checks the field values on ProjectSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectSearchRequestValidationError is the validation error returned by
|
|
// ProjectSearchRequest.Validate if the designated constraints aren't met.
|
|
type ProjectSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectSearchRequestValidationError) ErrorName() string {
|
|
return "ProjectSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _ProjectSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return ProjectSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectSearchQueryValidationError is the validation error returned by
|
|
// ProjectSearchQuery.Validate if the designated constraints aren't met.
|
|
type ProjectSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectSearchQueryValidationError) ErrorName() string {
|
|
return "ProjectSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectSearchQueryValidationError{}
|
|
|
|
var _ProjectSearchQuery_Key_NotInLookup = map[ProjectSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on Projects with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Projects) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
for idx, item := range m.GetProjects() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectsValidationError{
|
|
field: fmt.Sprintf("Projects[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectsValidationError is the validation error returned by
|
|
// Projects.Validate if the designated constraints aren't met.
|
|
type ProjectsValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectsValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectsValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectsValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectsValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectsValidationError) ErrorName() string { return "ProjectsValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectsValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjects.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectsValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectsValidationError{}
|
|
|
|
// Validate checks the field values on Project with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Project) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for ProjectRoleAssertion
|
|
|
|
// no validation rules for ProjectRoleCheck
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectValidationError is the validation error returned by Project.Validate
|
|
// if the designated constraints aren't met.
|
|
type ProjectValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectValidationError) ErrorName() string { return "ProjectValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProject.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberRoles with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectMemberRoles) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberRolesValidationError is the validation error returned by
|
|
// ProjectMemberRoles.Validate if the designated constraints aren't met.
|
|
type ProjectMemberRolesValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberRolesValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberRolesValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberRolesValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberRolesValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberRolesValidationError) ErrorName() string {
|
|
return "ProjectMemberRolesValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberRolesValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberRoles.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberRolesValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberRolesValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMember with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ProjectMember) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberValidationError is the validation error returned by
|
|
// ProjectMember.Validate if the designated constraints aren't met.
|
|
type ProjectMemberValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberValidationError) ErrorName() string { return "ProjectMemberValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMember.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberAdd with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ProjectMemberAdd) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectMemberAddValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ProjectMemberAddValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberAddValidationError is the validation error returned by
|
|
// ProjectMemberAdd.Validate if the designated constraints aren't met.
|
|
type ProjectMemberAddValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberAddValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberAddValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberAddValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberAddValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberAddValidationError) ErrorName() string { return "ProjectMemberAddValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberAddValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberAdd.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberAddValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberAddValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberChange with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectMemberChange) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectMemberChangeValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ProjectMemberChangeValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberChangeValidationError is the validation error returned by
|
|
// ProjectMemberChange.Validate if the designated constraints aren't met.
|
|
type ProjectMemberChangeValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberChangeValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberChangeValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberChangeValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberChangeValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberChangeValidationError) ErrorName() string {
|
|
return "ProjectMemberChangeValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberChangeValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberChange.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberChangeValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberChangeValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberRemove with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectMemberRemove) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectMemberRemoveValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ProjectMemberRemoveValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberRemoveValidationError is the validation error returned by
|
|
// ProjectMemberRemove.Validate if the designated constraints aren't met.
|
|
type ProjectMemberRemoveValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberRemoveValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberRemoveValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberRemoveValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberRemoveValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberRemoveValidationError) ErrorName() string {
|
|
return "ProjectMemberRemoveValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberRemoveValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberRemove.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberRemoveValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberRemoveValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleAdd with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ProjectRoleAdd) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectRoleAddValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Key
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for Group
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleAddValidationError is the validation error returned by
|
|
// ProjectRoleAdd.Validate if the designated constraints aren't met.
|
|
type ProjectRoleAddValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleAddValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleAddValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleAddValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleAddValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleAddValidationError) ErrorName() string { return "ProjectRoleAddValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleAddValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleAdd.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleAddValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleAddValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleAddBulk with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectRoleAddBulk) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectRoleAddBulkValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
for idx, item := range m.GetProjectRoles() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleAddBulkValidationError{
|
|
field: fmt.Sprintf("ProjectRoles[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleAddBulkValidationError is the validation error returned by
|
|
// ProjectRoleAddBulk.Validate if the designated constraints aren't met.
|
|
type ProjectRoleAddBulkValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleAddBulkValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleAddBulkValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleAddBulkValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleAddBulkValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleAddBulkValidationError) ErrorName() string {
|
|
return "ProjectRoleAddBulkValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleAddBulkValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleAddBulk.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleAddBulkValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleAddBulkValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleChange with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ProjectRoleChange) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectRoleChangeValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetKey()) < 1 {
|
|
return ProjectRoleChangeValidationError{
|
|
field: "Key",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for Group
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleChangeValidationError is the validation error returned by
|
|
// ProjectRoleChange.Validate if the designated constraints aren't met.
|
|
type ProjectRoleChangeValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleChangeValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleChangeValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleChangeValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleChangeValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleChangeValidationError) ErrorName() string {
|
|
return "ProjectRoleChangeValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleChangeValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleChange.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleChangeValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleChangeValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRole with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ProjectRole) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for Key
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Group
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleValidationError is the validation error returned by
|
|
// ProjectRole.Validate if the designated constraints aren't met.
|
|
type ProjectRoleValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleValidationError) ErrorName() string { return "ProjectRoleValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRole.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ProjectRoleView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for Key
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Group
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleViewValidationError is the validation error returned by
|
|
// ProjectRoleView.Validate if the designated constraints aren't met.
|
|
type ProjectRoleViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleViewValidationError) ErrorName() string { return "ProjectRoleViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleViewValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleRemove with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ProjectRoleRemove) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectRoleRemoveValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetKey()) < 1 {
|
|
return ProjectRoleRemoveValidationError{
|
|
field: "Key",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleRemoveValidationError is the validation error returned by
|
|
// ProjectRoleRemove.Validate if the designated constraints aren't met.
|
|
type ProjectRoleRemoveValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleRemoveValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleRemoveValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleRemoveValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleRemoveValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleRemoveValidationError) ErrorName() string {
|
|
return "ProjectRoleRemoveValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleRemoveValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleRemove.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleRemoveValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleRemoveValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectRoleSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleSearchResponseValidationError is the validation error returned by
|
|
// ProjectRoleSearchResponse.Validate if the designated constraints aren't met.
|
|
type ProjectRoleSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleSearchResponseValidationError) ErrorName() string {
|
|
return "ProjectRoleSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectRoleSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectRoleSearchRequestValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectRoleSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleSearchRequestValidationError is the validation error returned by
|
|
// ProjectRoleSearchRequest.Validate if the designated constraints aren't met.
|
|
type ProjectRoleSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleSearchRequestValidationError) ErrorName() string {
|
|
return "ProjectRoleSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectRoleSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectRoleSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _ProjectRoleSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return ProjectRoleSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectRoleSearchQueryValidationError is the validation error returned by
|
|
// ProjectRoleSearchQuery.Validate if the designated constraints aren't met.
|
|
type ProjectRoleSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectRoleSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectRoleSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectRoleSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectRoleSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectRoleSearchQueryValidationError) ErrorName() string {
|
|
return "ProjectRoleSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectRoleSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectRoleSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectRoleSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectRoleSearchQueryValidationError{}
|
|
|
|
var _ProjectRoleSearchQuery_Key_NotInLookup = map[ProjectRoleSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on ProjectMemberView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ProjectMemberView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for UserName
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberViewValidationError is the validation error returned by
|
|
// ProjectMemberView.Validate if the designated constraints aren't met.
|
|
type ProjectMemberViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberViewValidationError) ErrorName() string {
|
|
return "ProjectMemberViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberViewValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberSearchResponse with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectMemberSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberSearchResponseValidationError is the validation error returned
|
|
// by ProjectMemberSearchResponse.Validate if the designated constraints
|
|
// aren't met.
|
|
type ProjectMemberSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberSearchResponseValidationError) ErrorName() string {
|
|
return "ProjectMemberSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberSearchRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectMemberSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectMemberSearchRequestValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectMemberSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberSearchRequestValidationError is the validation error returned
|
|
// by ProjectMemberSearchRequest.Validate if the designated constraints aren't met.
|
|
type ProjectMemberSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberSearchRequestValidationError) ErrorName() string {
|
|
return "ProjectMemberSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectMemberSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectMemberSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _ProjectMemberSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return ProjectMemberSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectMemberSearchQueryValidationError is the validation error returned by
|
|
// ProjectMemberSearchQuery.Validate if the designated constraints aren't met.
|
|
type ProjectMemberSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectMemberSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectMemberSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectMemberSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectMemberSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectMemberSearchQueryValidationError) ErrorName() string {
|
|
return "ProjectMemberSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectMemberSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectMemberSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectMemberSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectMemberSearchQueryValidationError{}
|
|
|
|
var _ProjectMemberSearchQuery_Key_NotInLookup = map[ProjectMemberSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on Application with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *Application) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Sequence
|
|
|
|
switch m.AppConfig.(type) {
|
|
|
|
case *Application_OidcConfig:
|
|
|
|
if v, ok := interface{}(m.GetOidcConfig()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationValidationError{
|
|
field: "OidcConfig",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationValidationError is the validation error returned by
|
|
// Application.Validate if the designated constraints aren't met.
|
|
type ApplicationValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationValidationError) ErrorName() string { return "ApplicationValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplication.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationValidationError{}
|
|
|
|
// Validate checks the field values on ApplicationUpdate with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ApplicationUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ApplicationUpdateValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ApplicationUpdateValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return ApplicationUpdateValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationUpdateValidationError is the validation error returned by
|
|
// ApplicationUpdate.Validate if the designated constraints aren't met.
|
|
type ApplicationUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationUpdateValidationError) ErrorName() string {
|
|
return "ApplicationUpdateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplicationUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationUpdateValidationError{}
|
|
|
|
// Validate checks the field values on OIDCConfig with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *OIDCConfig) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ApplicationType
|
|
|
|
// no validation rules for ClientId
|
|
|
|
// no validation rules for ClientSecret
|
|
|
|
// no validation rules for AuthMethodType
|
|
|
|
// no validation rules for Version
|
|
|
|
// no validation rules for NoneCompliant
|
|
|
|
for idx, item := range m.GetComplianceProblems() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return OIDCConfigValidationError{
|
|
field: fmt.Sprintf("ComplianceProblems[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for DevMode
|
|
|
|
// no validation rules for AccessTokenType
|
|
|
|
// no validation rules for AccessTokenRoleAssertion
|
|
|
|
// no validation rules for IdTokenRoleAssertion
|
|
|
|
return nil
|
|
}
|
|
|
|
// OIDCConfigValidationError is the validation error returned by
|
|
// OIDCConfig.Validate if the designated constraints aren't met.
|
|
type OIDCConfigValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OIDCConfigValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OIDCConfigValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OIDCConfigValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OIDCConfigValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OIDCConfigValidationError) ErrorName() string { return "OIDCConfigValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OIDCConfigValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOIDCConfig.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OIDCConfigValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OIDCConfigValidationError{}
|
|
|
|
// Validate checks the field values on OIDCApplicationCreate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OIDCApplicationCreate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return OIDCApplicationCreateValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return OIDCApplicationCreateValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for ApplicationType
|
|
|
|
// no validation rules for AuthMethodType
|
|
|
|
// no validation rules for Version
|
|
|
|
// no validation rules for DevMode
|
|
|
|
// no validation rules for AccessTokenType
|
|
|
|
// no validation rules for AccessTokenRoleAssertion
|
|
|
|
// no validation rules for IdTokenRoleAssertion
|
|
|
|
return nil
|
|
}
|
|
|
|
// OIDCApplicationCreateValidationError is the validation error returned by
|
|
// OIDCApplicationCreate.Validate if the designated constraints aren't met.
|
|
type OIDCApplicationCreateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OIDCApplicationCreateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OIDCApplicationCreateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OIDCApplicationCreateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OIDCApplicationCreateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OIDCApplicationCreateValidationError) ErrorName() string {
|
|
return "OIDCApplicationCreateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OIDCApplicationCreateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOIDCApplicationCreate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OIDCApplicationCreateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OIDCApplicationCreateValidationError{}
|
|
|
|
// Validate checks the field values on OIDCConfigUpdate with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OIDCConfigUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return OIDCConfigUpdateValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetApplicationId()) < 1 {
|
|
return OIDCConfigUpdateValidationError{
|
|
field: "ApplicationId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for ApplicationType
|
|
|
|
// no validation rules for AuthMethodType
|
|
|
|
// no validation rules for DevMode
|
|
|
|
// no validation rules for AccessTokenType
|
|
|
|
// no validation rules for AccessTokenRoleAssertion
|
|
|
|
// no validation rules for IdTokenRoleAssertion
|
|
|
|
return nil
|
|
}
|
|
|
|
// OIDCConfigUpdateValidationError is the validation error returned by
|
|
// OIDCConfigUpdate.Validate if the designated constraints aren't met.
|
|
type OIDCConfigUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OIDCConfigUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OIDCConfigUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OIDCConfigUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OIDCConfigUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OIDCConfigUpdateValidationError) ErrorName() string { return "OIDCConfigUpdateValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OIDCConfigUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOIDCConfigUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OIDCConfigUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OIDCConfigUpdateValidationError{}
|
|
|
|
// Validate checks the field values on ClientSecret with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ClientSecret) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ClientSecret
|
|
|
|
return nil
|
|
}
|
|
|
|
// ClientSecretValidationError is the validation error returned by
|
|
// ClientSecret.Validate if the designated constraints aren't met.
|
|
type ClientSecretValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ClientSecretValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ClientSecretValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ClientSecretValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ClientSecretValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ClientSecretValidationError) ErrorName() string { return "ClientSecretValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ClientSecretValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sClientSecret.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ClientSecretValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ClientSecretValidationError{}
|
|
|
|
// Validate checks the field values on ApplicationView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ApplicationView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Sequence
|
|
|
|
switch m.AppConfig.(type) {
|
|
|
|
case *ApplicationView_OidcConfig:
|
|
|
|
if v, ok := interface{}(m.GetOidcConfig()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationViewValidationError{
|
|
field: "OidcConfig",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationViewValidationError is the validation error returned by
|
|
// ApplicationView.Validate if the designated constraints aren't met.
|
|
type ApplicationViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationViewValidationError) ErrorName() string { return "ApplicationViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplicationView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationViewValidationError{}
|
|
|
|
// Validate checks the field values on ApplicationSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ApplicationSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationSearchResponseValidationError is the validation error returned by
|
|
// ApplicationSearchResponse.Validate if the designated constraints aren't met.
|
|
type ApplicationSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationSearchResponseValidationError) ErrorName() string {
|
|
return "ApplicationSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplicationSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ApplicationSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ApplicationSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ApplicationSearchRequestValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ApplicationSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationSearchRequestValidationError is the validation error returned by
|
|
// ApplicationSearchRequest.Validate if the designated constraints aren't met.
|
|
type ApplicationSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationSearchRequestValidationError) ErrorName() string {
|
|
return "ApplicationSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplicationSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ApplicationSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ApplicationSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _ApplicationSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return ApplicationSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// ApplicationSearchQueryValidationError is the validation error returned by
|
|
// ApplicationSearchQuery.Validate if the designated constraints aren't met.
|
|
type ApplicationSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ApplicationSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ApplicationSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ApplicationSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ApplicationSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ApplicationSearchQueryValidationError) ErrorName() string {
|
|
return "ApplicationSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ApplicationSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sApplicationSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ApplicationSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ApplicationSearchQueryValidationError{}
|
|
|
|
var _ApplicationSearchQuery_Key_NotInLookup = map[ApplicationSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on ProjectGrant with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ProjectGrant) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for GrantedOrgId
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantValidationError is the validation error returned by
|
|
// ProjectGrant.Validate if the designated constraints aren't met.
|
|
type ProjectGrantValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantValidationError) ErrorName() string { return "ProjectGrantValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrant.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantCreate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantCreate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantCreateValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetGrantedOrgId()) < 1 {
|
|
return ProjectGrantCreateValidationError{
|
|
field: "GrantedOrgId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantCreateValidationError is the validation error returned by
|
|
// ProjectGrantCreate.Validate if the designated constraints aren't met.
|
|
type ProjectGrantCreateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantCreateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantCreateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantCreateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantCreateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantCreateValidationError) ErrorName() string {
|
|
return "ProjectGrantCreateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantCreateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantCreate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantCreateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantCreateValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantUpdate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantUpdateValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectGrantUpdateValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantUpdateValidationError is the validation error returned by
|
|
// ProjectGrantUpdate.Validate if the designated constraints aren't met.
|
|
type ProjectGrantUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantUpdateValidationError) ErrorName() string {
|
|
return "ProjectGrantUpdateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantUpdateValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *ProjectGrantID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantIDValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return ProjectGrantIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantIDValidationError is the validation error returned by
|
|
// ProjectGrantID.Validate if the designated constraints aren't met.
|
|
type ProjectGrantIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantIDValidationError) ErrorName() string { return "ProjectGrantIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantIDValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ProjectGrantView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for GrantedOrgId
|
|
|
|
// no validation rules for GrantedOrgName
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for ProjectName
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for ResourceOwner
|
|
|
|
// no validation rules for ResourceOwnerName
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantViewValidationError is the validation error returned by
|
|
// ProjectGrantView.Validate if the designated constraints aren't met.
|
|
type ProjectGrantViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantViewValidationError) ErrorName() string { return "ProjectGrantViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantViewValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantSearchResponse with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantSearchResponseValidationError is the validation error returned
|
|
// by ProjectGrantSearchResponse.Validate if the designated constraints aren't met.
|
|
type ProjectGrantSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantSearchResponseValidationError) ErrorName() string {
|
|
return "ProjectGrantSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on GrantedProjectSearchRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *GrantedProjectSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return GrantedProjectSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// GrantedProjectSearchRequestValidationError is the validation error returned
|
|
// by GrantedProjectSearchRequest.Validate if the designated constraints
|
|
// aren't met.
|
|
type GrantedProjectSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e GrantedProjectSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e GrantedProjectSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e GrantedProjectSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e GrantedProjectSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e GrantedProjectSearchRequestValidationError) ErrorName() string {
|
|
return "GrantedProjectSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e GrantedProjectSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sGrantedProjectSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = GrantedProjectSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = GrantedProjectSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantSearchRequestValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantSearchRequestValidationError is the validation error returned by
|
|
// ProjectGrantSearchRequest.Validate if the designated constraints aren't met.
|
|
type ProjectGrantSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantSearchRequestValidationError) ErrorName() string {
|
|
return "ProjectGrantSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _ProjectGrantSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return ProjectGrantSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantSearchQueryValidationError is the validation error returned by
|
|
// ProjectGrantSearchQuery.Validate if the designated constraints aren't met.
|
|
type ProjectGrantSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantSearchQueryValidationError) ErrorName() string {
|
|
return "ProjectGrantSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantSearchQueryValidationError{}
|
|
|
|
var _ProjectGrantSearchQuery_Key_NotInLookup = map[ProjectGrantSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberRoles with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberRoles) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberRolesValidationError is the validation error returned by
|
|
// ProjectGrantMemberRoles.Validate if the designated constraints aren't met.
|
|
type ProjectGrantMemberRolesValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberRolesValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberRolesValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberRolesValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberRolesValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberRolesValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberRolesValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberRolesValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberRoles.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberRolesValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberRolesValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMember with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMember) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberValidationError is the validation error returned by
|
|
// ProjectGrantMember.Validate if the designated constraints aren't met.
|
|
type ProjectGrantMemberValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMember.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberAdd with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberAdd) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantMemberAddValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetGrantId()) < 1 {
|
|
return ProjectGrantMemberAddValidationError{
|
|
field: "GrantId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ProjectGrantMemberAddValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberAddValidationError is the validation error returned by
|
|
// ProjectGrantMemberAdd.Validate if the designated constraints aren't met.
|
|
type ProjectGrantMemberAddValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberAddValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberAddValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberAddValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberAddValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberAddValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberAddValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberAddValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberAdd.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberAddValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberAddValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberChange with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberChange) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantMemberChangeValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetGrantId()) < 1 {
|
|
return ProjectGrantMemberChangeValidationError{
|
|
field: "GrantId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ProjectGrantMemberChangeValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberChangeValidationError is the validation error returned by
|
|
// ProjectGrantMemberChange.Validate if the designated constraints aren't met.
|
|
type ProjectGrantMemberChangeValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberChangeValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberChangeValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberChangeValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberChangeValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberChangeValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberChangeValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberChangeValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberChange.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberChangeValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberChangeValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberRemove with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberRemove) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantMemberRemoveValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetGrantId()) < 1 {
|
|
return ProjectGrantMemberRemoveValidationError{
|
|
field: "GrantId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return ProjectGrantMemberRemoveValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberRemoveValidationError is the validation error returned by
|
|
// ProjectGrantMemberRemove.Validate if the designated constraints aren't met.
|
|
type ProjectGrantMemberRemoveValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberRemoveValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberRemoveValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberRemoveValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberRemoveValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberRemoveValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberRemoveValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberRemoveValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberRemove.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberRemoveValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberRemoveValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberView with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for UserName
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberViewValidationError is the validation error returned by
|
|
// ProjectGrantMemberView.Validate if the designated constraints aren't met.
|
|
type ProjectGrantMemberViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberViewValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberViewValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberSearchResponse with
|
|
// the rules defined in the proto definition for this message. If any rules
|
|
// are violated, an error is returned.
|
|
func (m *ProjectGrantMemberSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberSearchResponseValidationError is the validation error
|
|
// returned by ProjectGrantMemberSearchResponse.Validate if the designated
|
|
// constraints aren't met.
|
|
type ProjectGrantMemberSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberSearchResponseValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberSearchRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return ProjectGrantMemberSearchRequestValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetGrantId()) < 1 {
|
|
return ProjectGrantMemberSearchRequestValidationError{
|
|
field: "GrantId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ProjectGrantMemberSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberSearchRequestValidationError is the validation error
|
|
// returned by ProjectGrantMemberSearchRequest.Validate if the designated
|
|
// constraints aren't met.
|
|
type ProjectGrantMemberSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberSearchRequestValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ProjectGrantMemberSearchQuery with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ProjectGrantMemberSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _ProjectGrantMemberSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return ProjectGrantMemberSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// ProjectGrantMemberSearchQueryValidationError is the validation error
|
|
// returned by ProjectGrantMemberSearchQuery.Validate if the designated
|
|
// constraints aren't met.
|
|
type ProjectGrantMemberSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ProjectGrantMemberSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ProjectGrantMemberSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ProjectGrantMemberSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ProjectGrantMemberSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ProjectGrantMemberSearchQueryValidationError) ErrorName() string {
|
|
return "ProjectGrantMemberSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ProjectGrantMemberSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sProjectGrantMemberSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ProjectGrantMemberSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ProjectGrantMemberSearchQueryValidationError{}
|
|
|
|
var _ProjectGrantMemberSearchQuery_Key_NotInLookup = map[ProjectGrantMemberSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on UserGrant with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *UserGrant) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for OrgId
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for GrantId
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantValidationError is the validation error returned by
|
|
// UserGrant.Validate if the designated constraints aren't met.
|
|
type UserGrantValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantValidationError) ErrorName() string { return "UserGrantValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrant.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantCreate with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserGrantCreate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return UserGrantCreateValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetProjectId()) < 1 {
|
|
return UserGrantCreateValidationError{
|
|
field: "ProjectId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for GrantId
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantCreateValidationError is the validation error returned by
|
|
// UserGrantCreate.Validate if the designated constraints aren't met.
|
|
type UserGrantCreateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantCreateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantCreateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantCreateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantCreateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantCreateValidationError) ErrorName() string { return "UserGrantCreateValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantCreateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantCreate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantCreateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantCreateValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantUpdate with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *UserGrantUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return UserGrantUpdateValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return UserGrantUpdateValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantUpdateValidationError is the validation error returned by
|
|
// UserGrantUpdate.Validate if the designated constraints aren't met.
|
|
type UserGrantUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantUpdateValidationError) ErrorName() string { return "UserGrantUpdateValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantUpdateValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantRemoveBulk with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantRemoveBulk) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if len(m.GetIds()) < 1 {
|
|
return UserGrantRemoveBulkValidationError{
|
|
field: "Ids",
|
|
reason: "value must contain at least 1 item(s)",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantRemoveBulkValidationError is the validation error returned by
|
|
// UserGrantRemoveBulk.Validate if the designated constraints aren't met.
|
|
type UserGrantRemoveBulkValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantRemoveBulkValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantRemoveBulkValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantRemoveBulkValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantRemoveBulkValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantRemoveBulkValidationError) ErrorName() string {
|
|
return "UserGrantRemoveBulkValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantRemoveBulkValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantRemoveBulk.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantRemoveBulkValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantRemoveBulkValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserGrantID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return UserGrantIDValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return UserGrantIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantIDValidationError is the validation error returned by
|
|
// UserGrantID.Validate if the designated constraints aren't met.
|
|
type UserGrantIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantIDValidationError) ErrorName() string { return "UserGrantIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantIDValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantView with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *UserGrantView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for OrgId
|
|
|
|
// no validation rules for ProjectId
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for UserName
|
|
|
|
// no validation rules for FirstName
|
|
|
|
// no validation rules for LastName
|
|
|
|
// no validation rules for Email
|
|
|
|
// no validation rules for OrgName
|
|
|
|
// no validation rules for OrgDomain
|
|
|
|
// no validation rules for ProjectName
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for ResourceOwner
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
// no validation rules for GrantId
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantViewValidationError is the validation error returned by
|
|
// UserGrantView.Validate if the designated constraints aren't met.
|
|
type UserGrantViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantViewValidationError) ErrorName() string { return "UserGrantViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantViewValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantSearchResponseValidationError is the validation error returned by
|
|
// UserGrantSearchResponse.Validate if the designated constraints aren't met.
|
|
type UserGrantSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantSearchResponseValidationError) ErrorName() string {
|
|
return "UserGrantSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserGrantSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantSearchRequestValidationError is the validation error returned by
|
|
// UserGrantSearchRequest.Validate if the designated constraints aren't met.
|
|
type UserGrantSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantSearchRequestValidationError) ErrorName() string {
|
|
return "UserGrantSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserGrantSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserGrantSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _UserGrantSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return UserGrantSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
if _, ok := _UserGrantSearchQuery_Method_InLookup[m.GetMethod()]; !ok {
|
|
return UserGrantSearchQueryValidationError{
|
|
field: "Method",
|
|
reason: "value must be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserGrantSearchQueryValidationError is the validation error returned by
|
|
// UserGrantSearchQuery.Validate if the designated constraints aren't met.
|
|
type UserGrantSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserGrantSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserGrantSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserGrantSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserGrantSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserGrantSearchQueryValidationError) ErrorName() string {
|
|
return "UserGrantSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserGrantSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserGrantSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserGrantSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserGrantSearchQueryValidationError{}
|
|
|
|
var _UserGrantSearchQuery_Key_NotInLookup = map[UserGrantSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
var _UserGrantSearchQuery_Method_InLookup = map[SearchMethod]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on UserMembershipSearchResponse with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserMembershipSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserMembershipSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserMembershipSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserMembershipSearchResponseValidationError is the validation error returned
|
|
// by UserMembershipSearchResponse.Validate if the designated constraints
|
|
// aren't met.
|
|
type UserMembershipSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserMembershipSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserMembershipSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserMembershipSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserMembershipSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserMembershipSearchResponseValidationError) ErrorName() string {
|
|
return "UserMembershipSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserMembershipSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserMembershipSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserMembershipSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserMembershipSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on UserMembershipSearchRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserMembershipSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetUserId()) < 1 {
|
|
return UserMembershipSearchRequestValidationError{
|
|
field: "UserId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserMembershipSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserMembershipSearchRequestValidationError is the validation error returned
|
|
// by UserMembershipSearchRequest.Validate if the designated constraints
|
|
// aren't met.
|
|
type UserMembershipSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserMembershipSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserMembershipSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserMembershipSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserMembershipSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserMembershipSearchRequestValidationError) ErrorName() string {
|
|
return "UserMembershipSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserMembershipSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserMembershipSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserMembershipSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserMembershipSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on UserMembershipSearchQuery with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserMembershipSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _UserMembershipSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return UserMembershipSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
if _, ok := _UserMembershipSearchQuery_Method_InLookup[m.GetMethod()]; !ok {
|
|
return UserMembershipSearchQueryValidationError{
|
|
field: "Method",
|
|
reason: "value must be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserMembershipSearchQueryValidationError is the validation error returned by
|
|
// UserMembershipSearchQuery.Validate if the designated constraints aren't met.
|
|
type UserMembershipSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserMembershipSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserMembershipSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserMembershipSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserMembershipSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserMembershipSearchQueryValidationError) ErrorName() string {
|
|
return "UserMembershipSearchQueryValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserMembershipSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserMembershipSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserMembershipSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserMembershipSearchQueryValidationError{}
|
|
|
|
var _UserMembershipSearchQuery_Key_NotInLookup = map[UserMembershipSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
var _UserMembershipSearchQuery_Method_InLookup = map[SearchMethod]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on UserMembershipView with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *UserMembershipView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for MemberType
|
|
|
|
// no validation rules for AggregateId
|
|
|
|
// no validation rules for ObjectId
|
|
|
|
// no validation rules for DisplayName
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserMembershipViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return UserMembershipViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Sequence
|
|
|
|
// no validation rules for ResourceOwner
|
|
|
|
return nil
|
|
}
|
|
|
|
// UserMembershipViewValidationError is the validation error returned by
|
|
// UserMembershipView.Validate if the designated constraints aren't met.
|
|
type UserMembershipViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e UserMembershipViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e UserMembershipViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e UserMembershipViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e UserMembershipViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e UserMembershipViewValidationError) ErrorName() string {
|
|
return "UserMembershipViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e UserMembershipViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sUserMembershipView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = UserMembershipViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = UserMembershipViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpID with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IdpID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return IdpIDValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpIDValidationError is the validation error returned by IdpID.Validate if
|
|
// the designated constraints aren't met.
|
|
type IdpIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpIDValidationError) ErrorName() string { return "IdpIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpIDValidationError{}
|
|
|
|
// Validate checks the field values on Idp with the rules defined in the proto
|
|
// definition for this message. If any rules are violated, an error is returned.
|
|
func (m *Idp) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for StylingType
|
|
|
|
// no validation rules for Sequence
|
|
|
|
switch m.IdpConfig.(type) {
|
|
|
|
case *Idp_OidcConfig:
|
|
|
|
if v, ok := interface{}(m.GetOidcConfig()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpValidationError{
|
|
field: "OidcConfig",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpValidationError is the validation error returned by Idp.Validate if the
|
|
// designated constraints aren't met.
|
|
type IdpValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpValidationError) ErrorName() string { return "IdpValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdp.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpValidationError{}
|
|
|
|
// Validate checks the field values on IdpUpdate with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IdpUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetId()) < 1 {
|
|
return IdpUpdateValidationError{
|
|
field: "Id",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return IdpUpdateValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for StylingType
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpUpdateValidationError is the validation error returned by
|
|
// IdpUpdate.Validate if the designated constraints aren't met.
|
|
type IdpUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpUpdateValidationError) ErrorName() string { return "IdpUpdateValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpUpdateValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfig with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *OidcIdpConfig) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ClientId
|
|
|
|
// no validation rules for ClientSecret
|
|
|
|
// no validation rules for Issuer
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigValidationError is the validation error returned by
|
|
// OidcIdpConfig.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigValidationError) ErrorName() string { return "OidcIdpConfigValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfig.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfigCreate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OidcIdpConfigCreate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetName()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "Name",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for StylingType
|
|
|
|
if l := utf8.RuneCountInString(m.GetClientId()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "ClientId",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetClientSecret()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "ClientSecret",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetIssuer()); l < 1 || l > 200 {
|
|
return OidcIdpConfigCreateValidationError{
|
|
field: "Issuer",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigCreateValidationError is the validation error returned by
|
|
// OidcIdpConfigCreate.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigCreateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigCreateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigCreateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigCreateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigCreateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigCreateValidationError) ErrorName() string {
|
|
return "OidcIdpConfigCreateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigCreateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfigCreate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigCreateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigCreateValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfigUpdate with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *OidcIdpConfigUpdate) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetIdpId()) < 1 {
|
|
return OidcIdpConfigUpdateValidationError{
|
|
field: "IdpId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetClientId()); l < 1 || l > 200 {
|
|
return OidcIdpConfigUpdateValidationError{
|
|
field: "ClientId",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for ClientSecret
|
|
|
|
if l := utf8.RuneCountInString(m.GetIssuer()); l < 1 || l > 200 {
|
|
return OidcIdpConfigUpdateValidationError{
|
|
field: "Issuer",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigUpdateValidationError is the validation error returned by
|
|
// OidcIdpConfigUpdate.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigUpdateValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigUpdateValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigUpdateValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigUpdateValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigUpdateValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigUpdateValidationError) ErrorName() string {
|
|
return "OidcIdpConfigUpdateValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigUpdateValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfigUpdate.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigUpdateValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigUpdateValidationError{}
|
|
|
|
// Validate checks the field values on IdpSearchResponse with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *IdpSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpSearchResponseValidationError is the validation error returned by
|
|
// IdpSearchResponse.Validate if the designated constraints aren't met.
|
|
type IdpSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpSearchResponseValidationError) ErrorName() string {
|
|
return "IdpSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on IdpView with the rules defined in the
|
|
// proto definition for this message. If any rules are violated, an error is returned.
|
|
func (m *IdpView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Id
|
|
|
|
// no validation rules for State
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for StylingType
|
|
|
|
// no validation rules for ProviderType
|
|
|
|
// no validation rules for Sequence
|
|
|
|
switch m.IdpConfigView.(type) {
|
|
|
|
case *IdpView_OidcConfig:
|
|
|
|
if v, ok := interface{}(m.GetOidcConfig()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpViewValidationError{
|
|
field: "OidcConfig",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpViewValidationError is the validation error returned by IdpView.Validate
|
|
// if the designated constraints aren't met.
|
|
type IdpViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpViewValidationError) ErrorName() string { return "IdpViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpViewValidationError{}
|
|
|
|
// Validate checks the field values on OidcIdpConfigView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *OidcIdpConfigView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for ClientId
|
|
|
|
// no validation rules for Issuer
|
|
|
|
// no validation rules for IdpDisplayNameMapping
|
|
|
|
// no validation rules for UsernameMapping
|
|
|
|
return nil
|
|
}
|
|
|
|
// OidcIdpConfigViewValidationError is the validation error returned by
|
|
// OidcIdpConfigView.Validate if the designated constraints aren't met.
|
|
type OidcIdpConfigViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e OidcIdpConfigViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e OidcIdpConfigViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e OidcIdpConfigViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e OidcIdpConfigViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e OidcIdpConfigViewValidationError) ErrorName() string {
|
|
return "OidcIdpConfigViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e OidcIdpConfigViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sOidcIdpConfigView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = OidcIdpConfigViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = OidcIdpConfigViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpSearchRequest with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *IdpSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
for idx, item := range m.GetQueries() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpSearchRequestValidationError{
|
|
field: fmt.Sprintf("Queries[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpSearchRequestValidationError is the validation error returned by
|
|
// IdpSearchRequest.Validate if the designated constraints aren't met.
|
|
type IdpSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpSearchRequestValidationError) ErrorName() string { return "IdpSearchRequestValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on IdpSearchQuery with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IdpSearchQuery) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if _, ok := _IdpSearchQuery_Key_NotInLookup[m.GetKey()]; ok {
|
|
return IdpSearchQueryValidationError{
|
|
field: "Key",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
// no validation rules for Method
|
|
|
|
// no validation rules for Value
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpSearchQueryValidationError is the validation error returned by
|
|
// IdpSearchQuery.Validate if the designated constraints aren't met.
|
|
type IdpSearchQueryValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpSearchQueryValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpSearchQueryValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpSearchQueryValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpSearchQueryValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpSearchQueryValidationError) ErrorName() string { return "IdpSearchQueryValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpSearchQueryValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpSearchQuery.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpSearchQueryValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpSearchQueryValidationError{}
|
|
|
|
var _IdpSearchQuery_Key_NotInLookup = map[IdpSearchKey]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on LoginPolicy with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *LoginPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for AllowUsernamePassword
|
|
|
|
// no validation rules for AllowRegister
|
|
|
|
// no validation rules for AllowExternalIdp
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return LoginPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return LoginPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// LoginPolicyValidationError is the validation error returned by
|
|
// LoginPolicy.Validate if the designated constraints aren't met.
|
|
type LoginPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e LoginPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e LoginPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e LoginPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e LoginPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e LoginPolicyValidationError) ErrorName() string { return "LoginPolicyValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e LoginPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sLoginPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = LoginPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = LoginPolicyValidationError{}
|
|
|
|
// Validate checks the field values on LoginPolicyRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *LoginPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for AllowUsernamePassword
|
|
|
|
// no validation rules for AllowRegister
|
|
|
|
// no validation rules for AllowExternalIdp
|
|
|
|
return nil
|
|
}
|
|
|
|
// LoginPolicyRequestValidationError is the validation error returned by
|
|
// LoginPolicyRequest.Validate if the designated constraints aren't met.
|
|
type LoginPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e LoginPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e LoginPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e LoginPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e LoginPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e LoginPolicyRequestValidationError) ErrorName() string {
|
|
return "LoginPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e LoginPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sLoginPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = LoginPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = LoginPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderID with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IdpProviderID) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if utf8.RuneCountInString(m.GetIdpConfigId()) < 1 {
|
|
return IdpProviderIDValidationError{
|
|
field: "IdpConfigId",
|
|
reason: "value length must be at least 1 runes",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderIDValidationError is the validation error returned by
|
|
// IdpProviderID.Validate if the designated constraints aren't met.
|
|
type IdpProviderIDValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderIDValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderIDValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderIDValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderIDValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderIDValidationError) ErrorName() string { return "IdpProviderIDValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderIDValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderID.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderIDValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderIDValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderAdd with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IdpProviderAdd) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
if l := utf8.RuneCountInString(m.GetIdpConfigId()); l < 1 || l > 200 {
|
|
return IdpProviderAddValidationError{
|
|
field: "IdpConfigId",
|
|
reason: "value length must be between 1 and 200 runes, inclusive",
|
|
}
|
|
}
|
|
|
|
if _, ok := _IdpProviderAdd_IdpProviderType_NotInLookup[m.GetIdpProviderType()]; ok {
|
|
return IdpProviderAddValidationError{
|
|
field: "IdpProviderType",
|
|
reason: "value must not be in list [0]",
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderAddValidationError is the validation error returned by
|
|
// IdpProviderAdd.Validate if the designated constraints aren't met.
|
|
type IdpProviderAddValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderAddValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderAddValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderAddValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderAddValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderAddValidationError) ErrorName() string { return "IdpProviderAddValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderAddValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderAdd.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderAddValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderAddValidationError{}
|
|
|
|
var _IdpProviderAdd_IdpProviderType_NotInLookup = map[IdpProviderType]struct{}{
|
|
0: {},
|
|
}
|
|
|
|
// Validate checks the field values on IdpProvider with the rules defined in
|
|
// the proto definition for this message. If any rules are violated, an error
|
|
// is returned.
|
|
func (m *IdpProvider) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for IdpProvider_Type
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderValidationError is the validation error returned by
|
|
// IdpProvider.Validate if the designated constraints aren't met.
|
|
type IdpProviderValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderValidationError) ErrorName() string { return "IdpProviderValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProvider.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderValidationError{}
|
|
|
|
// Validate checks the field values on LoginPolicyView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *LoginPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Default
|
|
|
|
// no validation rules for AllowUsernamePassword
|
|
|
|
// no validation rules for AllowRegister
|
|
|
|
// no validation rules for AllowExternalIdp
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return LoginPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return LoginPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// LoginPolicyViewValidationError is the validation error returned by
|
|
// LoginPolicyView.Validate if the designated constraints aren't met.
|
|
type LoginPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e LoginPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e LoginPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e LoginPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e LoginPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e LoginPolicyViewValidationError) ErrorName() string { return "LoginPolicyViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e LoginPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sLoginPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = LoginPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = LoginPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *IdpProviderView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for Name
|
|
|
|
// no validation rules for Type
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderViewValidationError is the validation error returned by
|
|
// IdpProviderView.Validate if the designated constraints aren't met.
|
|
type IdpProviderViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderViewValidationError) ErrorName() string { return "IdpProviderViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderViewValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IdpProviderSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpProviderSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return IdpProviderSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderSearchResponseValidationError is the validation error returned by
|
|
// IdpProviderSearchResponse.Validate if the designated constraints aren't met.
|
|
type IdpProviderSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderSearchResponseValidationError) ErrorName() string {
|
|
return "IdpProviderSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on IdpProviderSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *IdpProviderSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
return nil
|
|
}
|
|
|
|
// IdpProviderSearchRequestValidationError is the validation error returned by
|
|
// IdpProviderSearchRequest.Validate if the designated constraints aren't met.
|
|
type IdpProviderSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e IdpProviderSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e IdpProviderSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e IdpProviderSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e IdpProviderSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e IdpProviderSearchRequestValidationError) ErrorName() string {
|
|
return "IdpProviderSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e IdpProviderSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sIdpProviderSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = IdpProviderSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = IdpProviderSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPSearchRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPSearchRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for UserId
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPSearchRequestValidationError is the validation error returned by
|
|
// ExternalIDPSearchRequest.Validate if the designated constraints aren't met.
|
|
type ExternalIDPSearchRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPSearchRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPSearchRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPSearchRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPSearchRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPSearchRequestValidationError) ErrorName() string {
|
|
return "ExternalIDPSearchRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPSearchRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPSearchRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPSearchRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPSearchRequestValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPSearchResponse with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPSearchResponse) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Offset
|
|
|
|
// no validation rules for Limit
|
|
|
|
// no validation rules for TotalResult
|
|
|
|
for idx, item := range m.GetResult() {
|
|
_, _ = idx, item
|
|
|
|
if v, ok := interface{}(item).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPSearchResponseValidationError{
|
|
field: fmt.Sprintf("Result[%v]", idx),
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
// no validation rules for ProcessedSequence
|
|
|
|
if v, ok := interface{}(m.GetViewTimestamp()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPSearchResponseValidationError{
|
|
field: "ViewTimestamp",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPSearchResponseValidationError is the validation error returned by
|
|
// ExternalIDPSearchResponse.Validate if the designated constraints aren't met.
|
|
type ExternalIDPSearchResponseValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPSearchResponseValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPSearchResponseValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPSearchResponseValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPSearchResponseValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPSearchResponseValidationError) ErrorName() string {
|
|
return "ExternalIDPSearchResponseValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPSearchResponseValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPSearchResponse.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPSearchResponseValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPSearchResponseValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPView with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *ExternalIDPView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for ExternalUserId
|
|
|
|
// no validation rules for IdpName
|
|
|
|
// no validation rules for ExternalUserDisplayName
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return ExternalIDPViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPViewValidationError is the validation error returned by
|
|
// ExternalIDPView.Validate if the designated constraints aren't met.
|
|
type ExternalIDPViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPViewValidationError) ErrorName() string { return "ExternalIDPViewValidationError" }
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPViewValidationError{}
|
|
|
|
// Validate checks the field values on ExternalIDPRemoveRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *ExternalIDPRemoveRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for UserId
|
|
|
|
// no validation rules for IdpConfigId
|
|
|
|
// no validation rules for ExternalUserId
|
|
|
|
return nil
|
|
}
|
|
|
|
// ExternalIDPRemoveRequestValidationError is the validation error returned by
|
|
// ExternalIDPRemoveRequest.Validate if the designated constraints aren't met.
|
|
type ExternalIDPRemoveRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e ExternalIDPRemoveRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e ExternalIDPRemoveRequestValidationError) ErrorName() string {
|
|
return "ExternalIDPRemoveRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e ExternalIDPRemoveRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sExternalIDPRemoveRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = ExternalIDPRemoveRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = ExternalIDPRemoveRequestValidationError{}
|
|
|
|
// Validate checks the field values on PasswordComplexityPolicy with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordComplexityPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordComplexityPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordComplexityPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordComplexityPolicyValidationError is the validation error returned by
|
|
// PasswordComplexityPolicy.Validate if the designated constraints aren't met.
|
|
type PasswordComplexityPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordComplexityPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordComplexityPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordComplexityPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordComplexityPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordComplexityPolicyValidationError) ErrorName() string {
|
|
return "PasswordComplexityPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordComplexityPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordComplexityPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordComplexityPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordComplexityPolicyValidationError{}
|
|
|
|
// Validate checks the field values on PasswordComplexityPolicyRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordComplexityPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordComplexityPolicyRequestValidationError is the validation error
|
|
// returned by PasswordComplexityPolicyRequest.Validate if the designated
|
|
// constraints aren't met.
|
|
type PasswordComplexityPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordComplexityPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordComplexityPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordComplexityPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordComplexityPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordComplexityPolicyRequestValidationError) ErrorName() string {
|
|
return "PasswordComplexityPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordComplexityPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordComplexityPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordComplexityPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordComplexityPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on PasswordComplexityPolicyView with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordComplexityPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Default
|
|
|
|
// no validation rules for MinLength
|
|
|
|
// no validation rules for HasLowercase
|
|
|
|
// no validation rules for HasUppercase
|
|
|
|
// no validation rules for HasNumber
|
|
|
|
// no validation rules for HasSymbol
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordComplexityPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordComplexityPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordComplexityPolicyViewValidationError is the validation error returned
|
|
// by PasswordComplexityPolicyView.Validate if the designated constraints
|
|
// aren't met.
|
|
type PasswordComplexityPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordComplexityPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordComplexityPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordComplexityPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordComplexityPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordComplexityPolicyViewValidationError) ErrorName() string {
|
|
return "PasswordComplexityPolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordComplexityPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordComplexityPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordComplexityPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordComplexityPolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on PasswordAgePolicy with the rules defined
|
|
// in the proto definition for this message. If any rules are violated, an
|
|
// error is returned.
|
|
func (m *PasswordAgePolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAgeDays
|
|
|
|
// no validation rules for ExpireWarnDays
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordAgePolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordAgePolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordAgePolicyValidationError is the validation error returned by
|
|
// PasswordAgePolicy.Validate if the designated constraints aren't met.
|
|
type PasswordAgePolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordAgePolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordAgePolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordAgePolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordAgePolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordAgePolicyValidationError) ErrorName() string {
|
|
return "PasswordAgePolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordAgePolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordAgePolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordAgePolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordAgePolicyValidationError{}
|
|
|
|
// Validate checks the field values on PasswordAgePolicyRequest with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordAgePolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAgeDays
|
|
|
|
// no validation rules for ExpireWarnDays
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordAgePolicyRequestValidationError is the validation error returned by
|
|
// PasswordAgePolicyRequest.Validate if the designated constraints aren't met.
|
|
type PasswordAgePolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordAgePolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordAgePolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordAgePolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordAgePolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordAgePolicyRequestValidationError) ErrorName() string {
|
|
return "PasswordAgePolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordAgePolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordAgePolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordAgePolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordAgePolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on PasswordAgePolicyView with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordAgePolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Default
|
|
|
|
// no validation rules for MaxAgeDays
|
|
|
|
// no validation rules for ExpireWarnDays
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordAgePolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordAgePolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordAgePolicyViewValidationError is the validation error returned by
|
|
// PasswordAgePolicyView.Validate if the designated constraints aren't met.
|
|
type PasswordAgePolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordAgePolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordAgePolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordAgePolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordAgePolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordAgePolicyViewValidationError) ErrorName() string {
|
|
return "PasswordAgePolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordAgePolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordAgePolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordAgePolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordAgePolicyViewValidationError{}
|
|
|
|
// Validate checks the field values on PasswordLockoutPolicy with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordLockoutPolicy) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAttempts
|
|
|
|
// no validation rules for ShowLockoutFailure
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordLockoutPolicyValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordLockoutPolicyValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordLockoutPolicyValidationError is the validation error returned by
|
|
// PasswordLockoutPolicy.Validate if the designated constraints aren't met.
|
|
type PasswordLockoutPolicyValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordLockoutPolicyValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordLockoutPolicyValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordLockoutPolicyValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordLockoutPolicyValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordLockoutPolicyValidationError) ErrorName() string {
|
|
return "PasswordLockoutPolicyValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordLockoutPolicyValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordLockoutPolicy.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordLockoutPolicyValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordLockoutPolicyValidationError{}
|
|
|
|
// Validate checks the field values on PasswordLockoutPolicyRequest with the
|
|
// rules defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordLockoutPolicyRequest) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for MaxAttempts
|
|
|
|
// no validation rules for ShowLockoutFailure
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordLockoutPolicyRequestValidationError is the validation error returned
|
|
// by PasswordLockoutPolicyRequest.Validate if the designated constraints
|
|
// aren't met.
|
|
type PasswordLockoutPolicyRequestValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordLockoutPolicyRequestValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordLockoutPolicyRequestValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordLockoutPolicyRequestValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordLockoutPolicyRequestValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordLockoutPolicyRequestValidationError) ErrorName() string {
|
|
return "PasswordLockoutPolicyRequestValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordLockoutPolicyRequestValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordLockoutPolicyRequest.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordLockoutPolicyRequestValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordLockoutPolicyRequestValidationError{}
|
|
|
|
// Validate checks the field values on PasswordLockoutPolicyView with the rules
|
|
// defined in the proto definition for this message. If any rules are
|
|
// violated, an error is returned.
|
|
func (m *PasswordLockoutPolicyView) Validate() error {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
// no validation rules for Default
|
|
|
|
// no validation rules for MaxAttempts
|
|
|
|
// no validation rules for ShowLockoutFailure
|
|
|
|
// no validation rules for Sequence
|
|
|
|
if v, ok := interface{}(m.GetCreationDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordLockoutPolicyViewValidationError{
|
|
field: "CreationDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
if v, ok := interface{}(m.GetChangeDate()).(interface{ Validate() error }); ok {
|
|
if err := v.Validate(); err != nil {
|
|
return PasswordLockoutPolicyViewValidationError{
|
|
field: "ChangeDate",
|
|
reason: "embedded message failed validation",
|
|
cause: err,
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// PasswordLockoutPolicyViewValidationError is the validation error returned by
|
|
// PasswordLockoutPolicyView.Validate if the designated constraints aren't met.
|
|
type PasswordLockoutPolicyViewValidationError struct {
|
|
field string
|
|
reason string
|
|
cause error
|
|
key bool
|
|
}
|
|
|
|
// Field function returns field value.
|
|
func (e PasswordLockoutPolicyViewValidationError) Field() string { return e.field }
|
|
|
|
// Reason function returns reason value.
|
|
func (e PasswordLockoutPolicyViewValidationError) Reason() string { return e.reason }
|
|
|
|
// Cause function returns cause value.
|
|
func (e PasswordLockoutPolicyViewValidationError) Cause() error { return e.cause }
|
|
|
|
// Key function returns key value.
|
|
func (e PasswordLockoutPolicyViewValidationError) Key() bool { return e.key }
|
|
|
|
// ErrorName returns error name.
|
|
func (e PasswordLockoutPolicyViewValidationError) ErrorName() string {
|
|
return "PasswordLockoutPolicyViewValidationError"
|
|
}
|
|
|
|
// Error satisfies the builtin error interface
|
|
func (e PasswordLockoutPolicyViewValidationError) Error() string {
|
|
cause := ""
|
|
if e.cause != nil {
|
|
cause = fmt.Sprintf(" | caused by: %v", e.cause)
|
|
}
|
|
|
|
key := ""
|
|
if e.key {
|
|
key = "key for "
|
|
}
|
|
|
|
return fmt.Sprintf(
|
|
"invalid %sPasswordLockoutPolicyView.%s: %s%s",
|
|
key,
|
|
e.field,
|
|
e.reason,
|
|
cause)
|
|
}
|
|
|
|
var _ error = PasswordLockoutPolicyViewValidationError{}
|
|
|
|
var _ interface {
|
|
Field() string
|
|
Reason() string
|
|
Key() bool
|
|
Cause() error
|
|
ErrorName() string
|
|
} = PasswordLockoutPolicyViewValidationError{}
|