mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 19:14:23 +00:00
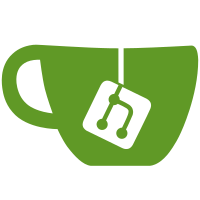
* feat: smtp templates poc * feat: add isActive & ProviderType to SMTP backend * feat: change providertype to uint32 and fix tests * feat: minimal smtp provider component * feat: woking on diiferent providers * feat: keep working on providers * feat: initial stepper for new provider * fix: settings list and working on stepper * feat: step 1 and 2 form inputs * feat: starter for smtp test step * fix: misspelled SMPT * fix: remove tests for now * feat: add tls toggle remove old google provider * feat: working on add smtp and table * fix: duplicated identifiers * fix: settings list * fix: add missing smtp config properties * fix: add configID to smtp config table * fix: working on listproviders * feat: working in listSMTPConfigs * fix: add count to listsmtpconfigs * fix: getting empty results from listSMTPConfigs * feat: table now shows real data * fix: remaining styles for smtp-table * fix: remove old notification-smtp-provider-component * feat: delete smtp configuration * feat: deactivate smtp config * feat: replace isActive with state for smtp config * feat: activate smtp config * fix: remaining errors after main merge * fix: list smtp providers panic and material mdc * feat: refactor to only one provider component * feat: current provider details view * fix: refactor AddSMTPConfig and ChangeSMTPConfig * fix: smtp config reduce issue * fix: recover domain in NewIAMSMTPConfigWriteModel * fix: add code needed by SetUpInstance * fix: go tests and warn about passing context to InstanceAggregateFromWriteModel * fix: i18n and add missing trans for fr, it, zh * fix: add e2e tests * docs: add smtp templates * fix: remove provider_type, add description * fix: remaining error from merge main * fix: add @stebenz change for primary key * fix: inactive placed after removed to prevent deleted configs to show as inactive * fix: smtp provider id can be empty (migrated) * feat: add mailchimp transactional template * feat: add Brevo (Sendinblue) template * feat: change brevo logo, add color to tls icon * fix: queries use resourceowner, id must not be empty * fix: deal with old smtp settings and tests * fix: resourceOwner is the instanceID * fix: remove aggregate_id, rename SMTPConfigByAggregateID with SMTPConfigActive * fix: add tests for multiple configs with different IDs * fix: conflict * fix: remove notification-smtp-provider * fix: add @peintnermax suggestions, rename module and fix e2e tests * fix: remove material legacy modules * fix: remove ctx as parameter for InstanceAggregateFromWriteModel * fix: add Id to SMTPConfigToPb * fix: change InstanceAggregateFromWriteModel to avoid linter errors * fix import * rm unused package-lock * update yarn lock --------- Co-authored-by: Elio Bischof <elio@zitadel.com> Co-authored-by: Max Peintner <max@caos.ch> Co-authored-by: Stefan Benz <46600784+stebenz@users.noreply.github.com>
245 lines
6.9 KiB
Go
245 lines
6.9 KiB
Go
package command
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
"github.com/zitadel/zitadel/internal/repository/instance"
|
|
)
|
|
|
|
type IAMSMTPConfigWriteModel struct {
|
|
eventstore.WriteModel
|
|
|
|
ID string
|
|
Description string
|
|
TLS bool
|
|
Host string
|
|
User string
|
|
Password *crypto.CryptoValue
|
|
SenderAddress string
|
|
SenderName string
|
|
ReplyToAddress string
|
|
State domain.SMTPConfigState
|
|
|
|
domain string
|
|
domainState domain.InstanceDomainState
|
|
smtpSenderAddressMatchesInstanceDomain bool
|
|
}
|
|
|
|
func NewIAMSMTPConfigWriteModel(instanceID, id, domain string) *IAMSMTPConfigWriteModel {
|
|
return &IAMSMTPConfigWriteModel{
|
|
WriteModel: eventstore.WriteModel{
|
|
AggregateID: instanceID,
|
|
ResourceOwner: instanceID,
|
|
InstanceID: instanceID,
|
|
},
|
|
ID: id,
|
|
domain: domain,
|
|
}
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) AppendEvents(events ...eventstore.Event) {
|
|
for _, event := range events {
|
|
switch e := event.(type) {
|
|
case *instance.DomainAddedEvent:
|
|
if e.Domain != wm.domain {
|
|
continue
|
|
}
|
|
wm.WriteModel.AppendEvents(e)
|
|
case *instance.DomainRemovedEvent:
|
|
if e.Domain != wm.domain {
|
|
continue
|
|
}
|
|
wm.WriteModel.AppendEvents(e)
|
|
default:
|
|
wm.WriteModel.AppendEvents(e)
|
|
}
|
|
|
|
}
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) Reduce() error {
|
|
for _, event := range wm.Events {
|
|
switch e := event.(type) {
|
|
case *instance.SMTPConfigAddedEvent:
|
|
if wm.ID != e.ID {
|
|
continue
|
|
}
|
|
wm.reduceSMTPConfigAddedEvent(e)
|
|
case *instance.SMTPConfigChangedEvent:
|
|
if wm.ID != e.ID {
|
|
continue
|
|
}
|
|
wm.reduceSMTPConfigChangedEvent(e)
|
|
case *instance.SMTPConfigRemovedEvent:
|
|
if wm.ID != e.ID {
|
|
continue
|
|
}
|
|
wm.reduceSMTPConfigRemovedEvent(e)
|
|
case *instance.SMTPConfigActivatedEvent:
|
|
if wm.ID != e.ID {
|
|
continue
|
|
}
|
|
wm.State = domain.SMTPConfigStateActive
|
|
case *instance.SMTPConfigDeactivatedEvent:
|
|
if wm.ID != e.ID {
|
|
continue
|
|
}
|
|
wm.State = domain.SMTPConfigStateInactive
|
|
case *instance.DomainAddedEvent:
|
|
wm.domainState = domain.InstanceDomainStateActive
|
|
case *instance.DomainRemovedEvent:
|
|
wm.domainState = domain.InstanceDomainStateRemoved
|
|
case *instance.DomainPolicyAddedEvent:
|
|
wm.smtpSenderAddressMatchesInstanceDomain = e.SMTPSenderAddressMatchesInstanceDomain
|
|
case *instance.DomainPolicyChangedEvent:
|
|
if e.SMTPSenderAddressMatchesInstanceDomain != nil {
|
|
wm.smtpSenderAddressMatchesInstanceDomain = *e.SMTPSenderAddressMatchesInstanceDomain
|
|
}
|
|
}
|
|
}
|
|
return wm.WriteModel.Reduce()
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) Query() *eventstore.SearchQueryBuilder {
|
|
// If ID equals ResourceOwner we're dealing with the old and unique smtp settings
|
|
// Let's set the empty ID for the query
|
|
if wm.ID == wm.ResourceOwner {
|
|
wm.ID = ""
|
|
}
|
|
|
|
return eventstore.NewSearchQueryBuilder(eventstore.ColumnsEvent).
|
|
ResourceOwner(wm.ResourceOwner).
|
|
AddQuery().
|
|
AggregateTypes(instance.AggregateType).
|
|
AggregateIDs(wm.AggregateID).
|
|
EventTypes(
|
|
instance.SMTPConfigAddedEventType,
|
|
instance.SMTPConfigRemovedEventType,
|
|
instance.SMTPConfigChangedEventType,
|
|
instance.SMTPConfigPasswordChangedEventType,
|
|
instance.SMTPConfigActivatedEventType,
|
|
instance.SMTPConfigDeactivatedEventType,
|
|
instance.SMTPConfigRemovedEventType,
|
|
instance.InstanceDomainAddedEventType,
|
|
instance.InstanceDomainRemovedEventType,
|
|
instance.DomainPolicyAddedEventType,
|
|
instance.DomainPolicyChangedEventType).
|
|
Builder()
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) NewChangedEvent(ctx context.Context, aggregate *eventstore.Aggregate, id, description string, tls bool, fromAddress, fromName, replyToAddress, smtpHost, smtpUser string, smtpPassword *crypto.CryptoValue) (*instance.SMTPConfigChangedEvent, bool, error) {
|
|
changes := make([]instance.SMTPConfigChanges, 0)
|
|
var err error
|
|
|
|
if wm.ID != id {
|
|
changes = append(changes, instance.ChangeSMTPConfigID(id))
|
|
}
|
|
if wm.Description != description {
|
|
changes = append(changes, instance.ChangeSMTPConfigDescription(description))
|
|
}
|
|
if wm.TLS != tls {
|
|
changes = append(changes, instance.ChangeSMTPConfigTLS(tls))
|
|
}
|
|
if wm.SenderAddress != fromAddress {
|
|
changes = append(changes, instance.ChangeSMTPConfigFromAddress(fromAddress))
|
|
}
|
|
if wm.SenderName != fromName {
|
|
changes = append(changes, instance.ChangeSMTPConfigFromName(fromName))
|
|
}
|
|
if wm.ReplyToAddress != replyToAddress {
|
|
changes = append(changes, instance.ChangeSMTPConfigReplyToAddress(replyToAddress))
|
|
}
|
|
if wm.Host != smtpHost {
|
|
changes = append(changes, instance.ChangeSMTPConfigSMTPHost(smtpHost))
|
|
}
|
|
if wm.User != smtpUser {
|
|
changes = append(changes, instance.ChangeSMTPConfigSMTPUser(smtpUser))
|
|
}
|
|
if smtpPassword != nil {
|
|
changes = append(changes, instance.ChangeSMTPConfigSMTPPassword(smtpPassword))
|
|
}
|
|
if len(changes) == 0 {
|
|
return nil, false, nil
|
|
}
|
|
changeEvent, err := instance.NewSMTPConfigChangeEvent(ctx, aggregate, id, changes)
|
|
if err != nil {
|
|
return nil, false, err
|
|
}
|
|
return changeEvent, true, nil
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) reduceSMTPConfigAddedEvent(e *instance.SMTPConfigAddedEvent) {
|
|
wm.Description = e.Description
|
|
wm.TLS = e.TLS
|
|
wm.Host = e.Host
|
|
wm.User = e.User
|
|
wm.Password = e.Password
|
|
wm.SenderAddress = e.SenderAddress
|
|
wm.SenderName = e.SenderName
|
|
wm.ReplyToAddress = e.ReplyToAddress
|
|
wm.State = domain.SMTPConfigStateInactive
|
|
// If ID has empty value we're dealing with the old and unique smtp settings
|
|
// These would be the default values for ID and State
|
|
if e.ID == "" {
|
|
wm.Description = "generic"
|
|
wm.ID = e.Aggregate().ResourceOwner
|
|
wm.State = domain.SMTPConfigStateActive
|
|
}
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) reduceSMTPConfigChangedEvent(e *instance.SMTPConfigChangedEvent) {
|
|
if e.Description != nil {
|
|
wm.Description = *e.Description
|
|
}
|
|
if e.TLS != nil {
|
|
wm.TLS = *e.TLS
|
|
}
|
|
if e.Host != nil {
|
|
wm.Host = *e.Host
|
|
}
|
|
if e.User != nil {
|
|
wm.User = *e.User
|
|
}
|
|
if e.Password != nil {
|
|
wm.Password = e.Password
|
|
}
|
|
if e.FromAddress != nil {
|
|
wm.SenderAddress = *e.FromAddress
|
|
}
|
|
if e.FromName != nil {
|
|
wm.SenderName = *e.FromName
|
|
}
|
|
if e.ReplyToAddress != nil {
|
|
wm.ReplyToAddress = *e.ReplyToAddress
|
|
}
|
|
|
|
// If ID has empty value we're dealing with the old and unique smtp settings
|
|
// These would be the default values for ID and State
|
|
if e.ID == "" {
|
|
wm.Description = "generic"
|
|
wm.ID = e.Aggregate().ResourceOwner
|
|
wm.State = domain.SMTPConfigStateActive
|
|
}
|
|
}
|
|
|
|
func (wm *IAMSMTPConfigWriteModel) reduceSMTPConfigRemovedEvent(e *instance.SMTPConfigRemovedEvent) {
|
|
wm.Description = ""
|
|
wm.TLS = false
|
|
wm.SenderName = ""
|
|
wm.SenderAddress = ""
|
|
wm.ReplyToAddress = ""
|
|
wm.Host = ""
|
|
wm.User = ""
|
|
wm.Password = nil
|
|
wm.State = domain.SMTPConfigStateRemoved
|
|
|
|
// If ID has empty value we're dealing with the old and unique smtp settings
|
|
// These would be the default values for ID and State
|
|
if e.ID == "" {
|
|
wm.ID = e.Aggregate().ResourceOwner
|
|
}
|
|
}
|