mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
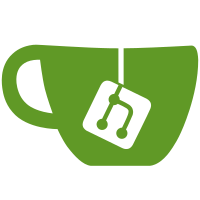
* cleanup todo * pass id token details to oidc * feat(oidc): id token for device authorization This changes updates to the newest oidc version, so the Device Authorization grant can return ID tokens when the scope `openid` is set. There is also some refactoring done, so that the eventstore can be queried directly when polling for state. The projection is cleaned up to a minimum with only data required for the login UI. * try to be explicit wit hthe timezone to fix github * pin oidc v3.8.0 * remove TBD entry
75 lines
1.6 KiB
Go
75 lines
1.6 KiB
Go
package domain
|
|
|
|
const (
|
|
OrgDomainPrimaryScope = "urn:zitadel:iam:org:domain:primary:"
|
|
OrgIDScope = "urn:zitadel:iam:org:id:"
|
|
OrgDomainPrimaryClaim = "urn:zitadel:iam:org:domain:primary"
|
|
OrgIDClaim = "urn:zitadel:iam:org:id"
|
|
ProjectIDScope = "urn:zitadel:iam:org:project:id:"
|
|
ProjectIDScopeZITADEL = "zitadel"
|
|
AudSuffix = ":aud"
|
|
SelectIDPScope = "urn:zitadel:iam:org:idp:id:"
|
|
)
|
|
|
|
// TODO: Change AuthRequest to interface and let oidcauthreqesut implement it
|
|
type Request interface {
|
|
Type() AuthRequestType
|
|
IsValid() bool
|
|
}
|
|
|
|
type AuthRequestType int32
|
|
|
|
const (
|
|
AuthRequestTypeOIDC AuthRequestType = iota
|
|
AuthRequestTypeSAML
|
|
AuthRequestTypeDevice
|
|
)
|
|
|
|
type AuthRequestOIDC struct {
|
|
Scopes []string
|
|
ResponseType OIDCResponseType
|
|
Nonce string
|
|
CodeChallenge *OIDCCodeChallenge
|
|
}
|
|
|
|
func (a *AuthRequestOIDC) Type() AuthRequestType {
|
|
return AuthRequestTypeOIDC
|
|
}
|
|
|
|
func (a *AuthRequestOIDC) IsValid() bool {
|
|
return len(a.Scopes) > 0 &&
|
|
a.CodeChallenge == nil || a.CodeChallenge != nil && a.CodeChallenge.IsValid()
|
|
}
|
|
|
|
type AuthRequestSAML struct {
|
|
ID string
|
|
BindingType string
|
|
Code string
|
|
Issuer string
|
|
IssuerName string
|
|
Destination string
|
|
}
|
|
|
|
func (a *AuthRequestSAML) Type() AuthRequestType {
|
|
return AuthRequestTypeSAML
|
|
}
|
|
|
|
func (a *AuthRequestSAML) IsValid() bool {
|
|
return true
|
|
}
|
|
|
|
type AuthRequestDevice struct {
|
|
ClientID string
|
|
DeviceCode string
|
|
UserCode string
|
|
Scopes []string
|
|
}
|
|
|
|
func (*AuthRequestDevice) Type() AuthRequestType {
|
|
return AuthRequestTypeDevice
|
|
}
|
|
|
|
func (a *AuthRequestDevice) IsValid() bool {
|
|
return a.DeviceCode != "" && a.UserCode != ""
|
|
}
|