mirror of
https://github.com/zitadel/zitadel.git
synced 2025-03-03 21:45:13 +00:00
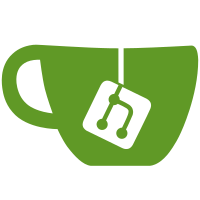
# Which Problems Are Solved - Microsoft Entra invokes the user patch endpoint with `"active": "True"` / `"active": "False"` when patching a user. This is a well-known bug in MS Entra (see [here](https://learn.microsoft.com/en-us/entra/identity/app-provisioning/application-provisioning-config-problem-scim-compatibility)), but the bug fix has not landed yet and/or the feature flag does not work. # How the Problems Are Solved - To ensure compatibility with MS Entra, the parsing of the the boolean active flag of the scim user is relaxed and accepts strings in any casing that resolve to `true` or `false` as well as raw boolean values. # Additional Context Part of https://github.com/zitadel/zitadel/issues/8140
42 lines
1.0 KiB
Go
42 lines
1.0 KiB
Go
package schemas
|
|
|
|
import (
|
|
"encoding/json"
|
|
"strings"
|
|
|
|
"github.com/muhlemmer/gu"
|
|
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
// RelaxedBool a bool which is more relaxed when it comes to json (un)marshaling.
|
|
// This ensures compatibility with some bugged scim providers,
|
|
// such as Microsoft Entry, which sends booleans as "True" or "False".
|
|
// See also https://learn.microsoft.com/en-us/entra/identity/app-provisioning/application-provisioning-config-problem-scim-compatibility.
|
|
type RelaxedBool bool
|
|
|
|
func NewRelaxedBool(value bool) *RelaxedBool {
|
|
return gu.Ptr(RelaxedBool(value))
|
|
}
|
|
|
|
func (b *RelaxedBool) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(bool(*b))
|
|
}
|
|
|
|
func (b *RelaxedBool) UnmarshalJSON(bytes []byte) error {
|
|
str := strings.ToLower(string(bytes))
|
|
switch {
|
|
case str == "true" || str == "\"true\"":
|
|
*b = true
|
|
case str == "false" || str == "\"false\"":
|
|
*b = false
|
|
default:
|
|
return zerrors.ThrowInvalidArgumentf(nil, "SCIM-BOO1", "bool expected, got %v", str)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (b *RelaxedBool) Bool() bool {
|
|
return bool(*b)
|
|
}
|