mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-17 15:18:51 +00:00
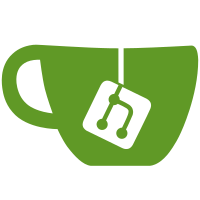
# Which Problems Are Solved Currently ZITADEL defines organization and instance member roles and permissions in defaults.yaml. The permission check is done on API call level. For example: "is this user allowed to make this call on this org". This makes sense on the V1 API where the API is permission-level shaped. For example, a search for users always happens in the context of the organization. (Either the organization the calling user belongs to, or through member ship and the x-zitadel-orgid header. However, for resource based APIs we must be able to resolve permissions by object. For example, an IAM_OWNER listing users should be able to get all users in an instance based on the query filters. Alternatively a user may have user.read permissions on one or more orgs. They should be able to read just those users. # How the Problems Are Solved ## Role permission mapping The role permission mappings defined from `defaults.yaml` or local config override are synchronized to the database on every run of `zitadel setup`: - A single query per **aggregate** builds a list of `add` and `remove` actions needed to reach the desired state or role permission mappings from the config. - The required events based on the actions are pushed to the event store. - Events define search fields so that permission checking can use the indices and is strongly consistent for both query and command sides. The migration is split in the following aggregates: - System aggregate for for roles prefixed with `SYSTEM` - Each instance for roles not prefixed with `SYSTEM`. This is in anticipation of instance level management over the API. ## Membership Current instance / org / project membership events now have field table definitions. Like the role permissions this ensures strong consistency while still being able to use the indices of the fields table. A migration is provided to fill the membership fields. ## Permission check I aimed keeping the mental overhead to the developer to a minimal. The provided implementation only provides a permission check for list queries for org level resources, for example users. In the `query` package there is a simple helper function `wherePermittedOrgs` which makes sure the underlying database function is called as part of the `SELECT` query and the permitted organizations are part of the `WHERE` clause. This makes sure results from non-permitted organizations are omitted. Under the hood: - A Pg/PlSQL function searches for a list of organization IDs the passed user has the passed permission. - When the user has the permission on instance level, it returns early with all organizations. - The functions uses a number of views. The views help mapping the fields entries into relational data and simplify the code use for the function. The views provide some pre-filters which allow proper index usage once the final `WHERE` clauses are set by the function. # Additional Changes # Additional Context Closes #9032 Closes https://github.com/zitadel/zitadel/issues/9014 https://github.com/zitadel/zitadel/issues/9188 defines follow-ups for the new permission framework based on this concept.
131 lines
4.5 KiB
Go
131 lines
4.5 KiB
Go
// Code generated by "enumer -type Key -transform snake -trimprefix Key"; DO NOT EDIT.
|
|
|
|
package feature
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
const _KeyName = "unspecifiedlogin_default_orgtrigger_introspection_projectionslegacy_introspectionuser_schematoken_exchangeactionsimproved_performanceweb_keydebug_oidc_parent_erroroidc_single_v1_session_terminationdisable_user_token_eventenable_back_channel_logoutlogin_v2permission_check_v2"
|
|
|
|
var _KeyIndex = [...]uint16{0, 11, 28, 61, 81, 92, 106, 113, 133, 140, 163, 197, 221, 247, 255, 274}
|
|
|
|
const _KeyLowerName = "unspecifiedlogin_default_orgtrigger_introspection_projectionslegacy_introspectionuser_schematoken_exchangeactionsimproved_performanceweb_keydebug_oidc_parent_erroroidc_single_v1_session_terminationdisable_user_token_eventenable_back_channel_logoutlogin_v2permission_check_v2"
|
|
|
|
func (i Key) String() string {
|
|
if i < 0 || i >= Key(len(_KeyIndex)-1) {
|
|
return fmt.Sprintf("Key(%d)", i)
|
|
}
|
|
return _KeyName[_KeyIndex[i]:_KeyIndex[i+1]]
|
|
}
|
|
|
|
// An "invalid array index" compiler error signifies that the constant values have changed.
|
|
// Re-run the stringer command to generate them again.
|
|
func _KeyNoOp() {
|
|
var x [1]struct{}
|
|
_ = x[KeyUnspecified-(0)]
|
|
_ = x[KeyLoginDefaultOrg-(1)]
|
|
_ = x[KeyTriggerIntrospectionProjections-(2)]
|
|
_ = x[KeyLegacyIntrospection-(3)]
|
|
_ = x[KeyUserSchema-(4)]
|
|
_ = x[KeyTokenExchange-(5)]
|
|
_ = x[KeyActions-(6)]
|
|
_ = x[KeyImprovedPerformance-(7)]
|
|
_ = x[KeyWebKey-(8)]
|
|
_ = x[KeyDebugOIDCParentError-(9)]
|
|
_ = x[KeyOIDCSingleV1SessionTermination-(10)]
|
|
_ = x[KeyDisableUserTokenEvent-(11)]
|
|
_ = x[KeyEnableBackChannelLogout-(12)]
|
|
_ = x[KeyLoginV2-(13)]
|
|
_ = x[KeyPermissionCheckV2-(14)]
|
|
}
|
|
|
|
var _KeyValues = []Key{KeyUnspecified, KeyLoginDefaultOrg, KeyTriggerIntrospectionProjections, KeyLegacyIntrospection, KeyUserSchema, KeyTokenExchange, KeyActions, KeyImprovedPerformance, KeyWebKey, KeyDebugOIDCParentError, KeyOIDCSingleV1SessionTermination, KeyDisableUserTokenEvent, KeyEnableBackChannelLogout, KeyLoginV2, KeyPermissionCheckV2}
|
|
|
|
var _KeyNameToValueMap = map[string]Key{
|
|
_KeyName[0:11]: KeyUnspecified,
|
|
_KeyLowerName[0:11]: KeyUnspecified,
|
|
_KeyName[11:28]: KeyLoginDefaultOrg,
|
|
_KeyLowerName[11:28]: KeyLoginDefaultOrg,
|
|
_KeyName[28:61]: KeyTriggerIntrospectionProjections,
|
|
_KeyLowerName[28:61]: KeyTriggerIntrospectionProjections,
|
|
_KeyName[61:81]: KeyLegacyIntrospection,
|
|
_KeyLowerName[61:81]: KeyLegacyIntrospection,
|
|
_KeyName[81:92]: KeyUserSchema,
|
|
_KeyLowerName[81:92]: KeyUserSchema,
|
|
_KeyName[92:106]: KeyTokenExchange,
|
|
_KeyLowerName[92:106]: KeyTokenExchange,
|
|
_KeyName[106:113]: KeyActions,
|
|
_KeyLowerName[106:113]: KeyActions,
|
|
_KeyName[113:133]: KeyImprovedPerformance,
|
|
_KeyLowerName[113:133]: KeyImprovedPerformance,
|
|
_KeyName[133:140]: KeyWebKey,
|
|
_KeyLowerName[133:140]: KeyWebKey,
|
|
_KeyName[140:163]: KeyDebugOIDCParentError,
|
|
_KeyLowerName[140:163]: KeyDebugOIDCParentError,
|
|
_KeyName[163:197]: KeyOIDCSingleV1SessionTermination,
|
|
_KeyLowerName[163:197]: KeyOIDCSingleV1SessionTermination,
|
|
_KeyName[197:221]: KeyDisableUserTokenEvent,
|
|
_KeyLowerName[197:221]: KeyDisableUserTokenEvent,
|
|
_KeyName[221:247]: KeyEnableBackChannelLogout,
|
|
_KeyLowerName[221:247]: KeyEnableBackChannelLogout,
|
|
_KeyName[247:255]: KeyLoginV2,
|
|
_KeyLowerName[247:255]: KeyLoginV2,
|
|
_KeyName[255:274]: KeyPermissionCheckV2,
|
|
_KeyLowerName[255:274]: KeyPermissionCheckV2,
|
|
}
|
|
|
|
var _KeyNames = []string{
|
|
_KeyName[0:11],
|
|
_KeyName[11:28],
|
|
_KeyName[28:61],
|
|
_KeyName[61:81],
|
|
_KeyName[81:92],
|
|
_KeyName[92:106],
|
|
_KeyName[106:113],
|
|
_KeyName[113:133],
|
|
_KeyName[133:140],
|
|
_KeyName[140:163],
|
|
_KeyName[163:197],
|
|
_KeyName[197:221],
|
|
_KeyName[221:247],
|
|
_KeyName[247:255],
|
|
_KeyName[255:274],
|
|
}
|
|
|
|
// KeyString retrieves an enum value from the enum constants string name.
|
|
// Throws an error if the param is not part of the enum.
|
|
func KeyString(s string) (Key, error) {
|
|
if val, ok := _KeyNameToValueMap[s]; ok {
|
|
return val, nil
|
|
}
|
|
|
|
if val, ok := _KeyNameToValueMap[strings.ToLower(s)]; ok {
|
|
return val, nil
|
|
}
|
|
return 0, fmt.Errorf("%s does not belong to Key values", s)
|
|
}
|
|
|
|
// KeyValues returns all values of the enum
|
|
func KeyValues() []Key {
|
|
return _KeyValues
|
|
}
|
|
|
|
// KeyStrings returns a slice of all String values of the enum
|
|
func KeyStrings() []string {
|
|
strs := make([]string, len(_KeyNames))
|
|
copy(strs, _KeyNames)
|
|
return strs
|
|
}
|
|
|
|
// IsAKey returns "true" if the value is listed in the enum definition. "false" otherwise
|
|
func (i Key) IsAKey() bool {
|
|
for _, v := range _KeyValues {
|
|
if i == v {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|