mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
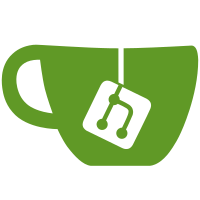
* chore: rename package errors to zerrors * rename package errors to gerrors * fix error related linting issues * fix zitadel error assertion * fix gosimple linting issues * fix deprecated linting issues * resolve gci linting issues * fix import structure --------- Co-authored-by: Elio Bischof <elio@zitadel.com>
38 lines
1.1 KiB
Go
38 lines
1.1 KiB
Go
package domain
|
|
|
|
import (
|
|
"encoding/base64"
|
|
"strings"
|
|
|
|
"github.com/zitadel/zitadel/internal/crypto"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
)
|
|
|
|
func NewRefreshToken(userID, tokenID string, algorithm crypto.EncryptionAlgorithm) (string, error) {
|
|
return RefreshToken(userID, tokenID, tokenID, algorithm)
|
|
}
|
|
|
|
func RefreshToken(userID, tokenID, token string, algorithm crypto.EncryptionAlgorithm) (string, error) {
|
|
encrypted, err := algorithm.Encrypt([]byte(userID + ":" + tokenID + ":" + token))
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
return base64.RawURLEncoding.EncodeToString(encrypted), nil
|
|
}
|
|
|
|
func FromRefreshToken(refreshToken string, algorithm crypto.EncryptionAlgorithm) (userID, tokenID, token string, err error) {
|
|
decoded, err := base64.RawURLEncoding.DecodeString(refreshToken)
|
|
if err != nil {
|
|
return "", "", "", err
|
|
}
|
|
decrypted, err := algorithm.Decrypt(decoded, algorithm.EncryptionKeyID())
|
|
if err != nil {
|
|
return "", "", "", err
|
|
}
|
|
split := strings.Split(string(decrypted), ":")
|
|
if len(split) != 3 {
|
|
return "", "", "", zerrors.ThrowInternal(nil, "DOMAIN-BGDhn", "Errors.User.RefreshToken.Invalid")
|
|
}
|
|
return split[0], split[1], split[2], nil
|
|
}
|