mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
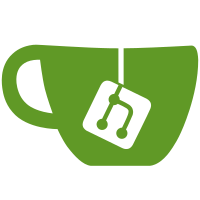
This implementation increases parallel write capabilities of the eventstore. Please have a look at the technical advisories: [05](https://zitadel.com/docs/support/advisory/a10005) and [06](https://zitadel.com/docs/support/advisory/a10006). The implementation of eventstore.push is rewritten and stored events are migrated to a new table `eventstore.events2`. If you are using cockroach: make sure that the database user of ZITADEL has `VIEWACTIVITY` grant. This is used to query events.
41 lines
876 B
Go
41 lines
876 B
Go
package models
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/zitadel/zitadel/internal/eventstore"
|
|
)
|
|
|
|
type ObjectRoot struct {
|
|
AggregateID string `json:"-"`
|
|
Sequence uint64 `json:"-"`
|
|
ResourceOwner string `json:"-"`
|
|
InstanceID string `json:"-"`
|
|
CreationDate time.Time `json:"-"`
|
|
ChangeDate time.Time `json:"-"`
|
|
}
|
|
|
|
func (o *ObjectRoot) AppendEvent(event eventstore.Event) {
|
|
if o.AggregateID == "" {
|
|
o.AggregateID = event.Aggregate().ID
|
|
} else if o.AggregateID != event.Aggregate().ID {
|
|
return
|
|
}
|
|
if o.ResourceOwner == "" {
|
|
o.ResourceOwner = event.Aggregate().ResourceOwner
|
|
}
|
|
if o.InstanceID == "" {
|
|
o.InstanceID = event.Aggregate().InstanceID
|
|
}
|
|
|
|
o.ChangeDate = event.CreatedAt()
|
|
if o.CreationDate.IsZero() {
|
|
o.CreationDate = o.ChangeDate
|
|
}
|
|
|
|
o.Sequence = event.Sequence()
|
|
}
|
|
func (o *ObjectRoot) IsZero() bool {
|
|
return o.AggregateID == ""
|
|
}
|