mirror of
https://github.com/zitadel/zitadel.git
synced 2025-07-02 17:58:36 +00:00
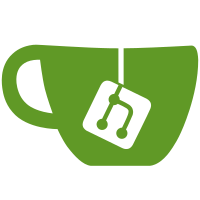
# Which Problems Are Solved Extends load tests by testing session creation. # How the Problems Are Solved The test creates a session including a check for user id. # Additional Context - part of https://github.com/zitadel/zitadel/issues/7639
43 lines
1.1 KiB
TypeScript
43 lines
1.1 KiB
TypeScript
import { Trend } from 'k6/metrics';
|
|
import { Org } from './org';
|
|
import http from 'k6/http';
|
|
import url from './url';
|
|
import { check } from 'k6';
|
|
import { User } from './user';
|
|
|
|
export type Session = {
|
|
id: string;
|
|
};
|
|
|
|
const addSessionTrend = new Trend('session_add_session_duration', true);
|
|
export function createSession(user: User, org: Org, accessToken: string): Promise<Session> {
|
|
return new Promise((resolve, reject) => {
|
|
let response = http.asyncRequest(
|
|
'POST',
|
|
url('/v2beta/sessions'),
|
|
JSON.stringify({
|
|
checks: {
|
|
user: {
|
|
userId: user.userId,
|
|
}
|
|
}
|
|
}),
|
|
{
|
|
headers: {
|
|
authorization: `Bearer ${accessToken}`,
|
|
'Content-Type': 'application/json',
|
|
'x-zitadel-orgid': org.organizationId,
|
|
},
|
|
},
|
|
);
|
|
response.then((res) => {
|
|
check(res, {
|
|
'add Session status ok': (r) => r.status === 201,
|
|
}) || reject(`unable to add Session status: ${res.status} body: ${res.body}`);
|
|
|
|
addSessionTrend.add(res.timings.duration);
|
|
resolve(res.json() as Session);
|
|
});
|
|
});
|
|
}
|