mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
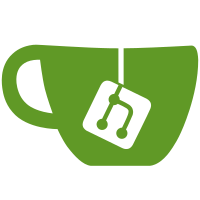
* add token exchange feature flag * allow setting reason and actor to access tokens * impersonation * set token types and scopes in response * upgrade oidc to working draft state * fix tests * audience and scope validation * id toke and jwt as input * return id tokens * add grant type token exchange to app config * add integration tests * check and deny actors in api calls * fix instance setting tests by triggering projection on write and cleanup * insert sleep statements again * solve linting issues * add translations * pin oidc v3.15.0 * resolve comments, add event translation * fix refreshtoken test * use ValidateAuthReqScopes from oidc * apparently the linter can't make up its mind * persist actor thru refresh tokens and check in tests * remove unneeded triggers
58 lines
1.3 KiB
Go
58 lines
1.3 KiB
Go
package integration
|
|
|
|
import (
|
|
"context"
|
|
"strings"
|
|
"time"
|
|
|
|
"google.golang.org/protobuf/types/known/timestamppb"
|
|
|
|
"github.com/zitadel/zitadel/pkg/grpc/admin"
|
|
"github.com/zitadel/zitadel/pkg/grpc/management"
|
|
)
|
|
|
|
func (s *Tester) CreateMachineUserPATWithMembership(ctx context.Context, roles ...string) (id, pat string, err error) {
|
|
user := s.CreateMachineUser(ctx)
|
|
|
|
patResp, err := s.Client.Mgmt.AddPersonalAccessToken(ctx, &management.AddPersonalAccessTokenRequest{
|
|
UserId: user.GetUserId(),
|
|
ExpirationDate: timestamppb.New(time.Now().Add(24 * time.Hour)),
|
|
})
|
|
if err != nil {
|
|
return "", "", err
|
|
}
|
|
|
|
orgRoles := make([]string, 0, len(roles))
|
|
iamRoles := make([]string, 0, len(roles))
|
|
|
|
for _, role := range roles {
|
|
if strings.HasPrefix(role, "ORG_") {
|
|
orgRoles = append(orgRoles, role)
|
|
}
|
|
if strings.HasPrefix(role, "IAM_") {
|
|
iamRoles = append(iamRoles, role)
|
|
}
|
|
}
|
|
|
|
if len(orgRoles) > 0 {
|
|
_, err := s.Client.Mgmt.AddOrgMember(ctx, &management.AddOrgMemberRequest{
|
|
UserId: user.GetUserId(),
|
|
Roles: orgRoles,
|
|
})
|
|
if err != nil {
|
|
return "", "", err
|
|
}
|
|
}
|
|
if len(iamRoles) > 0 {
|
|
_, err := s.Client.Admin.AddIAMMember(ctx, &admin.AddIAMMemberRequest{
|
|
UserId: user.GetUserId(),
|
|
Roles: iamRoles,
|
|
})
|
|
if err != nil {
|
|
return "", "", err
|
|
}
|
|
}
|
|
|
|
return user.GetUserId(), patResp.GetToken(), nil
|
|
}
|