mirror of
https://github.com/zitadel/zitadel.git
synced 2024-12-12 11:04:25 +00:00
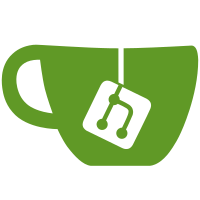
* feat(api): feature API proto definitions * update proto based on discussion with @livio-a * cleanup old feature flag stuff * authz instance queries * align defaults * projection definitions * define commands and event reducers * implement system and instance setter APIs * api getter implementation * unit test repository package * command unit tests * unit test Get queries * grpc converter unit tests * migrate the V1 features * migrate oidc to dynamic features * projection unit test * fix instance by host * fix instance by id data type in sql * fix linting errors * add system projection test * fix behavior inversion * resolve proto file comments * rename SystemDefaultLoginInstanceEventType to SystemLoginDefaultOrgEventType so it's consistent with the instance level event * use write models and conditional set events * system features integration tests * instance features integration tests * error on empty request * documentation entry * typo in feature.proto * fix start unit tests * solve linting error on key case switch * remove system defaults after discussion with @eliobischof * fix system feature projection * resolve comments in defaults.yaml --------- Co-authored-by: Livio Spring <livio.a@gmail.com>
38 lines
1.2 KiB
Go
38 lines
1.2 KiB
Go
package system
|
|
|
|
import (
|
|
"context"
|
|
|
|
object_pb "github.com/zitadel/zitadel/internal/api/grpc/object"
|
|
"github.com/zitadel/zitadel/internal/command"
|
|
"github.com/zitadel/zitadel/internal/domain"
|
|
"github.com/zitadel/zitadel/internal/zerrors"
|
|
system_pb "github.com/zitadel/zitadel/pkg/grpc/system"
|
|
)
|
|
|
|
func (s *Server) SetInstanceFeature(ctx context.Context, req *system_pb.SetInstanceFeatureRequest) (*system_pb.SetInstanceFeatureResponse, error) {
|
|
details, err := s.setInstanceFeature(ctx, req)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &system_pb.SetInstanceFeatureResponse{
|
|
Details: object_pb.DomainToChangeDetailsPb(details),
|
|
}, nil
|
|
|
|
}
|
|
|
|
func (s *Server) setInstanceFeature(ctx context.Context, req *system_pb.SetInstanceFeatureRequest) (*domain.ObjectDetails, error) {
|
|
feat := domain.Feature(req.FeatureId)
|
|
if feat != domain.FeatureLoginDefaultOrg {
|
|
return nil, zerrors.ThrowInvalidArgument(nil, "SYST-SGV45", "Errors.Feature.NotExisting")
|
|
}
|
|
switch t := req.Value.(type) {
|
|
case *system_pb.SetInstanceFeatureRequest_Bool:
|
|
return s.command.SetInstanceFeatures(ctx, &command.InstanceFeatures{
|
|
LoginDefaultOrg: &t.Bool,
|
|
})
|
|
default:
|
|
return nil, zerrors.ThrowInvalidArgument(nil, "SYST-dag5g", "Errors.Feature.TypeNotSupported")
|
|
}
|
|
}
|